These three components all deal with numbers in ranges. In this Quick Tip we'll look at how to use them.
The numeric stepper allows you to set a number within a certain range using the keyboard or by clicking arrow keys; the slider allows you to drag a thumb to pick a number from a range in a more graphical manner, and the progress bar shows how far through a range a certain number is.
Preview
In the preview SWF you'll see two Labels, two NumericSteppers, two Buttons, a blue Circle and a Slider. The NumericSteppers are used to set a range of numbers which we will generate a random number from. When the button is clicked the Label will change to show the random number that was generated.The Slider is used to change the size of the Circle; dragging the slider right increases the Circle's size, while dragging Left decreases the Circle's size.
The other button is used to load a SWF; press the button and a ProgressBar is added to the stage to show the Loading Progress. Once the Loader loads its content it is added to the stage and the ProgressBar is removed.
Step 1: Setting Up The Document
Open a new Flash Document and set the following properties
- Document Size: 550*360
- Background Color:#FFFFFF
Step 2: Add Components To The Stage
Open the components window by going to Menu > Window > Components or pressing CTRL+F7.
Drag two Buttons, two Labels, two Numeric Steppers, and one Slider to the stage.
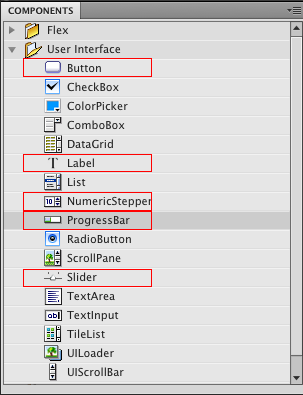
In the Properties panel give the first label the instance name "StepperLabel"
(If the Properties panel is not showing go to Menu > Window > Components or press CTRL+F3)
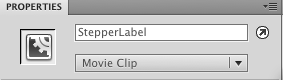
Set the label's X to 19 and its Y to 9.
Using the Properties panel:
- Give the second label the instance name "sliderLabel" X:19,Y:140
- Give the first NumericStepper the instance name "fromStepper" X:19,Y:144
- Give the second NumericStepper the instance name "toStepper" x:130,Y:44
- Give the Slider the instance name "slider" X:19,Y:223
- Give the first Button the instance name "randomNumButton" X:60,Y:82
- Give the second Button the instance name "loaderButton
Draw a blue circle with the oval tool (hold shift will dragging to make it conform to a circle) and convert it to a MovieClip by selecting it, right-clicking, and then choosing "Convert To Symbol". Give it an instance name of "theCircle".
Step 3: Preparing the AS File and Importing the Classes
Create a new ActionScript file and give it the name Main.as
We will be declaring our components in Main.as so we need to turn off "auto declare stage instances". The benefit of doing this is that you get code hinting for the instance.
Go to Menu > File > Publish Settings
Click on Settings next to the Script[ActionScript 3]

Uncheck "automatically declare stage instances"
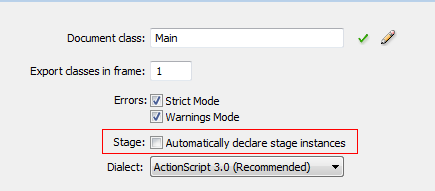
In Main.as open the package declaration and import the classes we will be using.
Add the following to the Main.as.
package { //Our Onstage Componets import fl.controls.NumericStepper; import fl.controls.Slider; import fl.controls.ProgressBar; import fl.controls.Label; import fl.controls.Button; //Needed to extend the Class import flash.display.MovieClip; //Needed to autosize our Label's Text import flash.text.TextFieldAutoSize; //The events we'l need in this project import flash.events.MouseEvent; import flash.events.Event; import fl.events.SliderEvent; import flash.events.ProgressEvent; //Needed to load the .swf file import flash.net.URLRequest; import flash.display.Loader;
Step 4: Set Up the Main Class
Let's add the Class, make it extend Movie Clip, and set up our constructor function.
Add the following to the Main.as:
public class Main extends MovieClip { public var StepperLabel:Label; public var sliderLabel:Label; public var fromStepper:NumericStepper; public var toStepper:NumericStepper; public var randomNumButton:Button; public var loaderButton:Button; public var slider:Slider; public var progressBar:ProgressBar; public var theLoader:Loader; public var theCircle:Circle; public function Main() { setupLabels(); setupSteppers(); setupButtons(); setupSlider(); }
Step 5: Main Constructor Functions
Here we define the functions that are used in our constructor.
In setupLabels()
function we do some basic setup on our Labels by using autoSize
so that each Label will automatically resize to hold all text passed to it.
In setupSteppers() function we set up our steppers' "minimum" and "maximum" properties. These are used to restrict the numbers available within the stepper. We are not using a event listener with the sliders here (though you can use Event.CHANGE
for the sliders to detect when their value is altered).
In setupButtons()
we add our text to the buttons via the label
property and add event listeners to the buttons.
In setupSliders()
we set the minimum
and maximum
for the sliders' values. The snapInterval
sets how much the slider's value changes when dragging; here we use .1. The liveDragging
is set to true
so that the value of the slider is available while dragging -- if this were set to false
we would not be able to get the value until the user stopped dragging. Finally, we add a listener to the slider.
Add the following to Main.as:
private function setupLabels():void { StepperLabel.text = "Click Button For A Random Number"; //Autosizes the label to hold all the text StepperLabel.autoSize = StepperLabel.autoSize = TextFieldAutoSize.LEFT; sliderLabel.text = "Drag Slider To Change Circle Size"; //Autosizes the label to hold all the text sliderLabel.autoSize = sliderLabel.autoSize = TextFieldAutoSize.LEFT; } private function setupSteppers():void { //Minumum values of the steppers fromStepper.minimum = 0; toStepper.minimum = 1; //Maximum values of the steppers fromStepper.maximum = 99; toStepper.maximum = 100; //Set the steppers value here fromStepper.value = 0; toStepper.value = 45; } private function setupButtons():void { randomNumButton.label = "Click"; randomNumButton.addEventListener(MouseEvent.CLICK,getrandomNumer); loaderButton.label = "Load Swf"; loaderButton.addEventListener(MouseEvent.CLICK,loadSwf); } private function setupSlider():void { //Minimum and maximum values for the slider slider.minimum = 1; slider.maximum = 3; //This set how much the slider "increments" by slider.snapInterval = .1; //liveDragging means the steppers value are available while dragging //if set to false we not not get the value till we stopped dragging slider.liveDragging = true; slider.addEventListener(SliderEvent.CHANGE,scaleCircle); }
Step 6 Add the Random Functions
Here we add the remaining functions.
In the getrandomNumber()
function we call another function, generateRandomNumber()
, which is from a tutorial here on Activetuts+ by Carlos Yanez. Go ahead and check out that tutorial to see how this works!
The scaleCircle()
function gets the value of the slider and sets the scaleX
and scaleY
of the theCircle
movieClip. The scaleX
and scaleY
are used to change the size (scale) of the movie clip.
The loadSwf()
function sets up a Loader which we use to load an external SWF. We then add an event listener to the Loader's contentLoaderInfo
; the contentLoaderInfo
holds information about what the loader is loading (here, a SWF).
We then set up a ProgressBar
and add it to the stage. We set the ProgressBar's source
to thecontentLoaderInfo
of the Loader, and since the contentInfoLoader
holds information about what the loader is loading (including the bytesLoaded
and bytesTotal
) this is how the ProgressBar can update itself to reflect how much of the SWF has loaded.
The finishedLoading()
function sets the x- and y-position of the Loader's content (i.e. the SWF) and then adds it to the stage. At this point we are done with the ProgressBar, so we remove it form the stage.
Then we close out the class and the package.
private function getrandomNumer(e:Event):void { //Here we pass in two numbers (the steppers' values) generateRandomNumber(fromStepper.value,toStepper.value); } private function generateRandomNumber(minNum:Number, maxNum:Number):void { //Holds the generated number var randomNumber = (Math.floor(Math.random() * (maxNum - minNum + 1)) + minNum); //Here we cast random number to a string so we can use it in the labels text StepperLabel.text = "Your random number is " + String(randomNumber); } private function scaleCircle(e:Event):void { //scaleX and scaleY increase or decrease a MovieClip's size //here we use the slider's value from "1-3" theCircle.scaleX = e.target.value; theCircle.scaleY = e.target.value; } private function loadSwf(e:Event):void { theLoader = new Loader(); theLoader.x = 319.00; theLoader.y = 31.00; theLoader.load(new URLRequest("dummy.swf")); //Here we get the loader's contentLoaderInfo which is what the loader //is loading (here a swf) theLoader.contentLoaderInfo.addEventListener(Event.COMPLETE, finishedLoading); progressBar = new ProgressBar(); progressBar.x = 323; progressBar.y = 244; addChild(progressBar); //The progress bar's source is set to the loader's contentLoaderInfo //contentLoaderInfo holds the information about the SWF's bytesLoaded/bytesTotal progressBar.source = theLoader.contentLoaderInfo; } public function finishedLoading(e:Event):void { //Here we set the loader content.x and .y //I.E. the swf's .x and .y theLoader.content.x=290; theLoader.content.y=20; //The we add the loaders content I.E. the swf addChild(theLoader.content); //Since it loader is done loading we don't need the progress bar anymore //So we remove it removeChild(progressBar); } } //close out the class }//close out the package
Conclusion
Again using components is a great way to add functionality to your Flash movies without having to build them from scratch
You'll notice in the Components Parameters panel of the components that you can check and select certain properties.
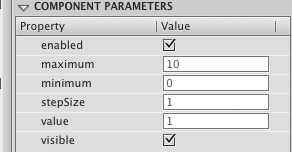
The above image is for the NumericStepper component
The properties are as follows for the NumericStepper component:
- enabled: a Boolean value that indicates whether the component can accept user input
- maximum: the maximum value in the sequence of numeric values.
- minimum: the minimum value in the sequence of numeric values.
- stepSize: nonzero number that describes the unit of change between values.
- value: the current value of the NumericStepper component.
- visible: a Boolean value that indicates whether the component instance is visible
The properties for the Slider are as follows
-
direction: direction of the slider. Acceptable values are
SliderDirection.HORIZONTAL
and SliderDirection.VERTICAL. - enabled: a Boolean value that indicates whether the component can accept user input
- maximum: The maximum allowed value on the Slider component instance.
- minimum: The minimum allowed value on the Slider component instance.
- snapInterval: the increment by which the value is increased or decreased as the user moves the slider thumb.
- tickInterval: spacing of the tick marks relative to the maximum value of the component.
- value: the current value of the Slider component.
- visible: a Boolean value that indicates whether the component instance is visible
The properties for the ProgressBar are as follows
-
direction: Indicates the fill direction for the progress bar. A value of
ProgressBarDirection.RIGHT
indicates that the progress bar is filled from left to right. A value ofProgressBarDirection.LEFT
indicates that the progress bar is filled from right to left. - enabled: a Boolean value that indicates whether the component can accept user input
-
mode: Gets or sets the method to be used to update the progress bar. The following values are valid for this property:
ProgressBarMode.EVENT
,ProgressBarMode.POLLED
,ProgressBarMode.MANUAL
- source: a reference to the content that is being loaded and for which the ProgressBar is measuring the progress of the load operation.
- visible: a Boolean value that indicates whether the component instance is visible
To see the properties for the labels and buttons be sure to check out the Quick Tip on labels and buttons.
The help files are also a great place to learn more about the component properties you can set in the parameters panel.
Thanks for reading and be look out for more upcoming component introductions!
Comments