Learn how to use key codes in ActionScript 3.0 to detect when the user presses a specific key.
Final Result Preview
Let's take a look at the final result we will be working towards:
Test the responses by pressing the keys on your keyboard..
Step 1: Add Text Boxes
Open a new Flash document. Add static text boxes on the left which have the names of the keys you will detect and dynamic text boxes with the text "No" inside them.
Give your dynamic text boxes instance names with the following format: "keyname_txt
". Mine are ctrl_txt
, shift_txt
, left_txt
, up_txt
, right_txt
, down_txt
and space_text
respectively.
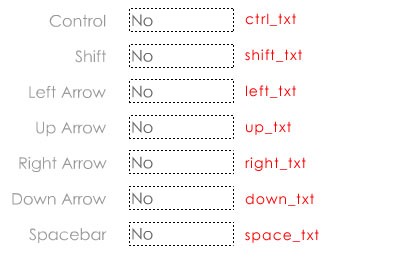
Step 2: Create Base Code
Go File 〉 New
and select Actionscript File
.
Now set up the base document class like so: (If you want to learn about document classes read Michael's Quick Tip)
package { import flash.display.MovieClip; public class KeyCodes extends MovieClip { public function KeyCodes() { } } }
Step 3: Create the Listener Events
To detect when the user presses a key with AS3 we need to add event listeners which listen for a user pressing and releasing keys. We can do this by adding the following piece of code inside our KeyCodes()
constructor function:
public function KeyCodes() { stage.addEventListener(KeyboardEvent.KEY_DOWN, onKeyPress); //Add an event listener to the stage that listens for a key being pressed stage.addEventListener(KeyboardEvent.KEY_UP, onKeyRelease); //Add an event listener to the stage that listens for a key being released }
Before we proceed we need to add a line of code to import the KeyboardEvent
. Add this line of code below where we import the MovieClip
class on line 3:
package { import flash.display.MovieClip; import flash.events.KeyboardEvent;
Step 4: Simple Test
Underneath our listeners add two functions which will be called when the user either presses or releases a key:
public function KeyCodes() { stage.addEventListener(KeyboardEvent.KEY_DOWN, onKeyPress); //Add an event listener to the stage that listens for a key being pressed stage.addEventListener(KeyboardEvent.KEY_UP, onKeyRelease); //Add an event listener to the stage that listens for a key being released function onKeyPress(e:KeyboardEvent):void { } function onKeyRelease(e:KeyboardEvent):void { } }
Now we can add a trace()
to each function so when you press a key it will trace "key pressed" into the output panel and "key released" when the key is released. To do this we can add the following code into our functions:
public function KeyCodes() { stage.addEventListener(KeyboardEvent.KEY_DOWN, onKeyPress); //Add an event listener to the stage that listens for a key being pressed stage.addEventListener(KeyboardEvent.KEY_UP, onKeyRelease); //Add an event listener to the stage that listens for a key being released function onKeyPress(e:KeyboardEvent):void { trace("key pressed"); } function onKeyRelease(e:KeyboardEvent):void { trace("key released"); } }
Test your movie (Ctrl+Enter). When you press a key it should trace "key pressed" into your output panel and "key released" when you release it.
Step 5: Detect Keycodes
You can detect which key has been pressed by tracing the keycode. Change your trace from trace("key pressed")
to trace(e.keyCode)
and remove the key released trace. Your code should now look like this:
public function KeyCodes() { stage.addEventListener(KeyboardEvent.KEY_DOWN, onKeyPress); //Add an event listener to the stage that listens for a key being pressed stage.addEventListener(KeyboardEvent.KEY_UP, onKeyRelease); //Add an event listener to the stage that listens for a key being released function onKeyPress(e:KeyboardEvent):void { trace(e.keyCode); } function onKeyRelease(e:KeyboardEvent):void { } }
Now when you press a key it will trace out the code that relates to that specific key. If I press the left arrow it will trace 37
into the output panel and the spacebar will trace 32
.
With this information literally at your fingertips you can execute different events for different keys, all with one event listener. Try adding this code to your function and see what happens when you press the spacebar:
function onKeyPress(e:KeyboardEvent):void { trace(e.keyCode); if(e.keyCode == 32) { //If the keycode is equal to 32 (spacebar) trace("spacebar pressed"); } }
Now if you press the spacebar it will not only trace out 32, it will trace "spacebar pressed". You can use this to deal with many different keys separately within the one function.
Step 6: Change Text on Key Press
Go ahead and delete the trace inside the "if" statement. Replace it with this:
function onKeyPress(e:KeyboardEvent):void { trace(e.keyCode); if(e.keyCode == 32) { //If the keycode is equal to 32 (spacebar) space_txt.text = "Yes"; //Change the spacebar text box to "Yes" } }
Now when you press the spacebar, you should see the spacebar label change from "No" to "Yes".
Do this for all of the keys except control and shift, as they have special ways of being detected.
function onKeyPress(e:KeyboardEvent):void { if(e.keyCode == 37){ //37 is keycode for left arrow left_txt.text = "Yes"; } if(e.keyCode == 38){ //38 is keycode for up arrow up_txt.text = "Yes"; } if(e.keyCode == 39){ //39 is keycode for right arrow right_txt.text = "Yes"; } if(e.keyCode == 40){ //40 is keycode for down arrow down_txt.text = "Yes"; } if(e.keyCode == 32){ //32 is keycode for spacebar space_txt.text = "Yes"; } }
Step 7: Detect Control and Shift
How do we detect whether the control or shift buttons are pressed? Each one has an easy built-in variable which is automatically changed when the keys are pressed. You can detect them using the following code. Put this code below the other "if" statements in onKeyPress()
.
if(e.ctrlKey == true) { ctrl_txt.text = "Yes"; } if(e.shiftKey == true) { shift_txt.text = "Yes"; }
Note: there is also altKey
, which will detect whether the alt key is pressed. This will only work in Adobe AIR applications as pressing alt while focused in a Flash file will almost always take focus from the SWF and therefore not work.
Step 8: Write the Release Function
To create the release function all we need to do is copy the code inside the onKeyPress()
function and just change a few things.
We need to change all the text to say "No" instead of "Yes" and check if ctrlKey
and shiftKey
are false
not true
. This is what the final code should look like:
package { import flash.display.MovieClip; import flash.events.KeyboardEvent; public class KeyCodes extends MovieClip { public function KeyCodes() { stage.addEventListener(KeyboardEvent.KEY_DOWN, onKeyPress); //Add an event listener to the stage that listens for a key being pressed stage.addEventListener(KeyboardEvent.KEY_UP, onKeyRelease); //Add an event listener to the stage that listens for a key being released function onKeyPress(e:KeyboardEvent):void { if(e.keyCode == 37){ //37 is keycode for left arrow left_txt.text = "Yes"; } if(e.keyCode == 38){ //38 is keycode for up arrow up_txt.text = "Yes"; } if(e.keyCode == 39){ //39 is keycode for right arrow right_txt.text = "Yes"; } if(e.keyCode == 40){ //40 is keycode for down arrow down_txt.text = "Yes"; } if(e.keyCode == 32){ //32 is keycode for spacebar space_txt.text = "Yes"; } if(e.ctrlKey == true) { ctrl_txt.text = "Yes"; } if(e.shiftKey == true) { shift_txt.text = "Yes"; } } function onKeyRelease(e:KeyboardEvent):void { if(e.keyCode == 37){ //37 is keycode for left arrow left_txt.text = "No"; } if(e.keyCode == 38){ //38 is keycode for up arrow up_txt.text = "No"; } if(e.keyCode == 39){ //39 is keycode for right arrow right_txt.text = "No"; } if(e.keyCode == 40){ //40 is keycode for down arrow down_txt.text = "No"; } if(e.keyCode == 32){ //32 is keycode for spacebar space_txt.text = "No"; } if(e.ctrlKey == false) { ctrl_txt.text = "No"; } if(e.shiftKey == false) { shift_txt.text = "No"; } } } } }
Declare the functions inside the constructor means that they'll be garbage collected if the event listeners are removed. If you'd rather this didn't happen, you can declare them as methods, like so:
package { import flash.display.MovieClip; import flash.events.KeyboardEvent; public class KeyCodes extends MovieClip { public function KeyCodes() { stage.addEventListener(KeyboardEvent.KEY_DOWN, onKeyPress); //Add an event listener to the stage that listens for a key being pressed stage.addEventListener(KeyboardEvent.KEY_UP, onKeyRelease); //Add an event listener to the stage that listens for a key being released } public function onKeyPress(e:KeyboardEvent):void { if(e.keyCode == 37){ //37 is keycode for left arrow left_txt.text = "Yes"; } if(e.keyCode == 38){ //38 is keycode for up arrow up_txt.text = "Yes"; } if(e.keyCode == 39){ //39 is keycode for right arrow right_txt.text = "Yes"; } if(e.keyCode == 40){ //40 is keycode for down arrow down_txt.text = "Yes"; } if(e.keyCode == 32){ //32 is keycode for spacebar space_txt.text = "Yes"; } if(e.ctrlKey == true) { ctrl_txt.text = "Yes"; } if(e.shiftKey == true) { shift_txt.text = "Yes"; } } public function onKeyRelease(e:KeyboardEvent):void { if(e.keyCode == 37){ //37 is keycode for left arrow left_txt.text = "No"; } if(e.keyCode == 38){ //38 is keycode for up arrow up_txt.text = "No"; } if(e.keyCode == 39){ //39 is keycode for right arrow right_txt.text = "No"; } if(e.keyCode == 40){ //40 is keycode for down arrow down_txt.text = "No"; } if(e.keyCode == 32){ //32 is keycode for spacebar space_txt.text = "No"; } if(e.ctrlKey == false) { ctrl_txt.text = "No"; } if(e.shiftKey == false) { shift_txt.text = "No"; } } } }
Conclusion
Test your movie and all should be well! If you have any comments or issues just post them in the comments section and I (or someone else) will answer your question.
Thanks for reading and I hope it helped you learn more about key presses in Flash.
Comments