This tutorial will demonstrate how to create a Default Post Thumbnail from the core. Many tutorials demonstrate how to check if a thumbnail exists in a post and then render one if no thumbnail exists. This tutorial will show you how to avoid saving a post without a thumbnail, in the first place.
Usual Way to Get Default Thumbnail
As mentioned before, some tutorials suggest making an If ... Else
statement in the theme to show a default thumbnail. An example is as follows:
if (has_post_thumbnail()) { the_post_thumbnail(); } else { echo '<img src="' . get_bloginfo('template_directory') . '/images/thumb-default.png' . '" width="100" height="100" alt="thumbnail" />'; }
That simple statement will work, but it would require modifying third-party plugins that do not have default thumbnail features. Which is why, this tutorial will save a default thumbnail to the database.
Step 1 Getting the Thumbnail ID
The first thing you have to do is fetch the ID of the uploaded thumbnail you will be using. You do this by visiting the Media Library, selecting your image, and collecting the ID.
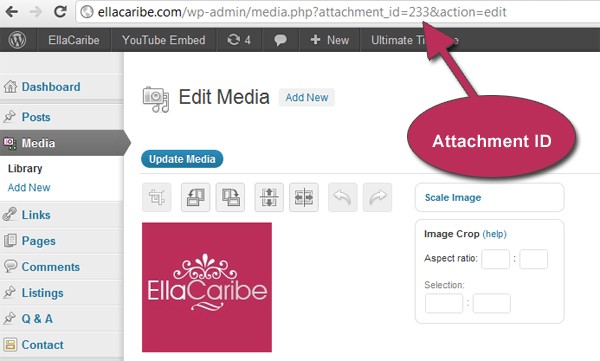
In the screenshot, one collects the ID integer from the address bar where "attachment_id=
".
Step 2 Coding the Function
To add the default thumbnail feature, you can add the following snippet to your functions.php file in your theme folder.
add_action( 'save_post', 'wptuts_save_thumbnail' ); function wptuts_save_thumbnail( $post_id ) { // Get Thumbnail $post_thumbnail = get_post_meta( $post_id, $key = '_thumbnail_id', $single = true ); // Verify that post is not a revision if ( !wp_is_post_revision( $post_id ) ) { // Check if Thumbnail exists if ( empty( $post_thumbnail ) ) { // Add thumbnail to post update_post_meta( $post_id, $meta_key = '_thumbnail_id', $meta_value = '233' ); } } }
We use the save_post
hook to trigger our function and get the recently added post. Once we are referencing the recently added post, we use get_post_meta
to get the value from the database for thumbnail ID where the post ID is the last inserted. Next we use the wp_is_post_revision
function to check whether the saved post is a revision or a new post. If the post is a revision, then we will void the rest of the function. Using an If
statement, we check to see if any meta data for the thumbnail exists. If none exists, then we use the add_post_meta
function to add the default thumbnail's ID to the last inserted post.
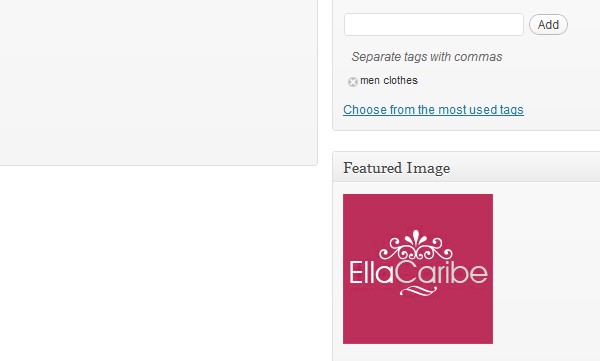
Voila! During a save on draft save, the default thumbnail will be saved to the database and is now available for use in Widgets or Plugins that use thumbnails. If you are building a theme, you can use this method and replace the $meta_value
with an image from your theme file. Happy Coding!
Comments