Sometimes we have a page just for the sake of making it a parent of other pages. I've even seen these pages left blank! You should at least have a small paragraph for the sake of search engines and visitors alike, but what about also offering a snippet of the subpages to read similar to how your blog page does posts?
In this quick tip, we'll create a little function that will query the page for child pages, display titles, excerpts and links if it finds any, and add it to a shortcode for use from the WordPress page editor.
Create the Function
function subpage_peek() { global $post; //query subpages $args = array( 'post_parent' => $post->ID, 'post_type' => 'page' ); $subpages = new WP_query($args); // create output if ($subpages->have_posts()) : $output = '<ul>'; while ($subpages->have_posts()) : $subpages->the_post(); $output .= '<li><strong><a href="'.get_permalink().'">'.get_the_title().'</a></strong> <p>'.get_the_excerpt().'<br /> <a href="'.get_permalink().'">Continue Reading →</a></p></li>'; endwhile; $output .= '</ul>'; else : $output = '<p>No subpages found.</p>'; endif; // reset the query wp_reset_postdata(); // return something return $output; }
This code performs a simple query for the current page's children.
- Query the child pages
- If the query returns pages, loop through them and create an output with an unordered list that includes the linked title, the excerpt, and a "Continue Reading" link
- If the query doesn't return anything, set the output to say that nothing was found. You could set this to whatever would be the most useful for your application.
- Don't forget to reset the post data!
- Return the results rather than echo them so that it can be used as a shortcode
Create the Shortcode
add_shortcode('subpage_peek', 'subpage_peek');
Creating shortcodes out of functions is pretty simple with the built in WordPress function. You could also simply echo the function from within a template. If you really wanted to get creative, you could add it to a custom widget!
Conclusion
That's all, folks! This is a pretty handy way of handling subpages and offering a preview to readers. Your output should look something like this:
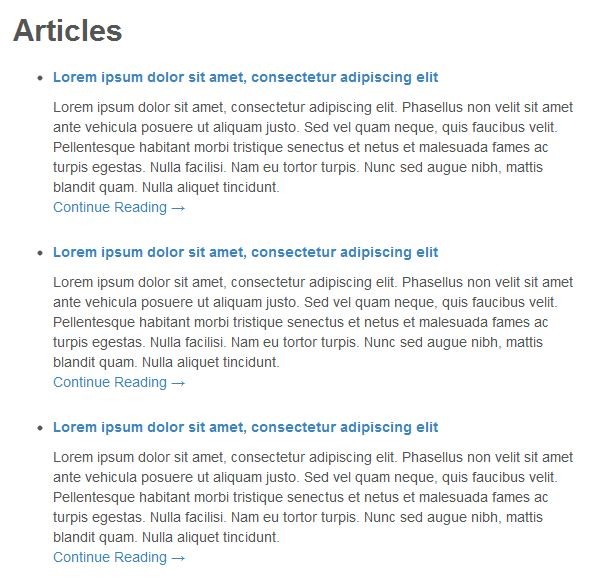
Comments