As many stated before me: "A good WordPress citizen only loads their files where they're needed". This principle applies both to front-end and back-end (admin). There's no justification for loading CSS and JS files on every admin page when you only need them on one single page you created. Thankfully doing things the right way is only one function call away.
"Never include CSS or JS files on all admin pages. It will cause conflicts with other plugins."
There's a WordPress Function for Everything
Since almost all admin pages have a unique URL it's really not difficult to detect when a certain page is loaded and then (and only then) include JS and CSS files we need. We can use $_SERVER['REQUEST_URI']
, or in many cases, the $_GET['action']
variable. However there's a much easier, cleaner and more standardized way of doing that. Say hello to the get_current_screen
function.
Things to know about the get_current_screen
function:
- It was introduced in WordPress v3.1, so if you try to use it in older versions you'll get a "call to undefined function" error. Using the
function_exists
function to check for it is a good idea if you want to provide an alternative. - It's not available in the
admin_init
orinit
hooks because it gets initialized after those hooks are called. - The function returns a
WP_Screen
object with a lot of info but you'll mainly be interested in theid
object property. - It's not available on the front-end (because it serves no purpose there).
A Few Lines of Code Make All the Difference
Let's assume your plugin has an options page under the Settings menu which you created with:
add_options_page('My Plugin', 'My Plugin', 'manage_options', 'my_plugin', 'my_plugin_options');
You need some extra CSS and JavaScript on that page so you add this code as well:
// Bad code below! Don't copy/paste! add_action('admin_enqueue_scripts', 'my_plugin_scripts'); function my_plugin_scripts() { wp_enqueue_style('farbtastic'); wp_enqueue_script('farbtastic'); }
That's bad! Don't do that! The snippet above will include CSS and JS for Farbtastic color picker on every single admin page. If other plugins want to get rid of your includes on their pages they have to use wp_dequeue_*
functions to dequeue them. That's really unnecessary and rude of us because we can write better code!
add_action('admin_enqueue_scripts', 'my_plugin_scripts'); function my_plugin_scripts() { // Include JS/CSS only if we're on our options page if (is_my_plugin_screen()) { wp_enqueue_style('farbtastic'); wp_enqueue_script('farbtastic'); } } // Check if we're on our options page function is_my_plugin_screen() { $screen = get_current_screen(); if (is_object($screen) && $screen->id == 'settings_page_my_plugin') { return true; } else { return false; } }
It's Really Easy
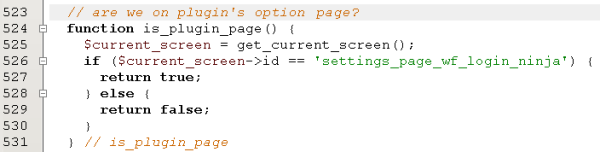
If you have a look at our improved code you can see we only added one if
statement and a simple function – is_my_plugin_screen
which checks if we're on our plugin's options page. The variable $screen
holds the WP_Screen
object which has many properties but we're only interested in the id
one. That id
consists of a prefix "settings_page_
", which is the same for all settings pages, and a string "my_plugin
" which is unique to our plugin because we defined it as the 4th parameter in the add_options_page
function call.
The code is very simple and easily adaptable to any admin screen. To see what the id is of the current screen simply dump the content of $screen
with:
echo '<pre>' . print_r(get_current_screen(), true) . '</pre>';
Things to take home:
- Never include CSS or JS files on all admin pages; it will cause conflicts with other plugins.
- Use
get_current_screen
afterinit
to find out when your admin screen is visible and only then include additional files. - You can find a list of the core admin screen IDs in the Codex under Admin Screen Reference.
- Never echo
<script>
or<style>
tags; always usewp_enqueue_*
functions. - Check the Codex to see if the script you need is included in WP core already.
Comments