If you are reading this, you know that chat bots are one of the biggest tech trends of 2016.
The bot revolution is not only about artificial intelligence. A bot can be a tool in your messenger with a simple chat interface that can be used to extend the functionality of sites or services or can even be a standalone application. Bots are cheaper to develop and easier to install, and another great feature is that messengers can be used on every type of device—laptops, smartphones, and tablets. That's why everybody is crazy about bots now.
And the biggest messenger with an open bot API is Telegram.
What We Are Going to Do
In this article we will create a simple stopwatch Telegram bot. I will show you how to create your bot, connect with analytics, write some code, and finally add your bot to a bot store.
By the way, I've already prepared a demo, so you can test it just by adding @stopwatchbot to your Telegram contact list.
Create a Bot With BotFather
The first step to creating a bot is to register the account of your bot in Telegram. And there is a bot for that, called the BotFather. Just add it to your contact list and you'll be able to create and set up Telegram bots, just by typing the /newbot
command and following the instructions of BotFather.

After registering your new bot, you will receive a congratulations message with an authorization token. We will use this token soon to authorize a bot and send requests to the Bot API.
Later you can use BotFather to add descriptions or photos to the profiles of your bots, regenerate tokens, set lists of commands to use, delete accounts, and so on. To get a full list of commands, just type /help
in a chat to get a list of BotFather's commands.
Connect to Botan Analytics
There is no built-in analytics in the Telegram Bots API, but it's important to know how many users you have, how they act, and which commands they trigger more. Of course, we can collect this information using our own engine, but if we want to focus on bot functionality, not metrics, we just need to use an out-of-the-box solution.
And there is a simple tool to connect your bot to analytics, called Botan. It's based on Yandex AppMetric and completely free. Using Botan, you can segment your audience, get information about user profiles, get the most used command, and get beautiful graphs right in your messenger, like this:
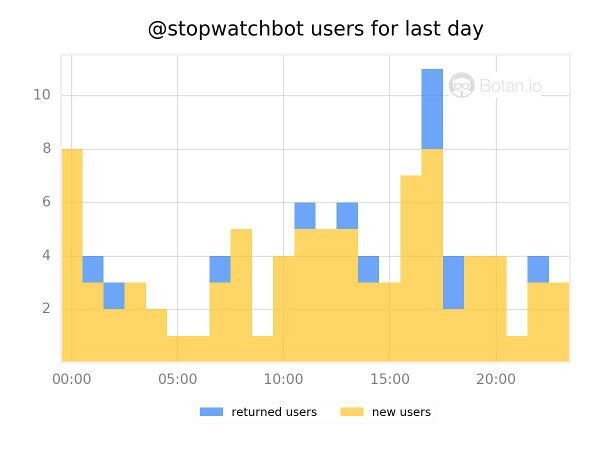
To get started, you need to register your bot in Botan and get a token. And again, you can do it with a bot, BotanioBot:

Just click the “Add bot” key on the dialog keyboard, type the nick of your bot, and you will get your bot track token. Now Botanio is ready to track your bot events, and you can get statistics by users, sessions, retention and events right in your messenger.
Create and Register an SSL Webhook
In Telegram there are two ways to get messages from your users: long polling and webhooks.
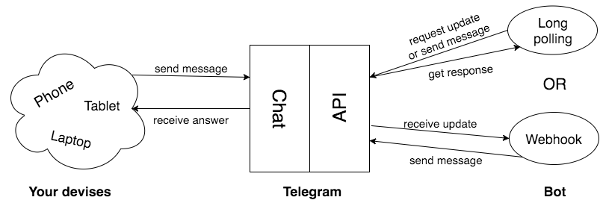
Basically, with long polling, you need to request new messages from the API, and with webhooks you are setting a callback that the Telegram API will call if a new message arrives from a user. I prefer to use webhooks because it looks like real-time communication, so in this article we will use this method too. Now we need to choose a callback URL for our webhook, which needs to be reached under the HTTPS protocol, and we need to set it really secure, so hide your script in a secret path, as the manual says:
If you'd like to make sure that the Webhook request comes from Telegram, we recommend using a secret path in the URL, e.g.https://www.example.com/<token>
. Since nobody else knows your bot‘s token, you can be pretty sure it’s us.
If your SSL certificate is trusted, all you need to do is open this URL in your browser:
https://api.telegram.org:443/bot[token]/setwebhook?url=[webhook]
Otherwise you have to generate a self-signed certificate. Here is an example of the command on Linux for it:
openssl req -newkey rsa:2048 -sha256 -nodes -keyout /path/to/certificate.key -x509 -days 365 -out /path/to/certificate.crt -subj "/C=IT/ST=state/L=location/O=description/CN=yourdomain.com"
And don't forget to open the SSL port:
sudo ufw allow 443/tcp
To get the certificate checked and set your webhook domain to trusted, you need to upload your public key certificate:
curl \ -F "url=https://yourdomain.com/path/to/script.php" \ -F "certificate=/path/to/certificate.key" \ "https://api.telegram.org/bot[token]/setwebhook"
Finally you will get a JSON reply like this:
{"ok":true,"result":true,"description":"Webhook was set"}
It says that the webhook was set and we are ready to start the engine of the bot.
Build a Database
Now we need to build a database for our timers. What do we need to store in it? When a user commands the stopwatch to start, we will take the ID of the chat and save a row with the chat ID and current Unix time, which is the number of seconds between now and the start of Unix Epoch, which is 1 January 1970 at UTC. Consequently, we will save a row with the chat ID and integer timestamp of the current Unix time.
To show the current stopwatch time, we will get the saved timestamp and compare it with the current timestamp. The difference will be the current time in seconds. If the user stops the timer, we will simply delete the row with the current chat ID.
So let's create a database and table to store the stopwatch information:
CREATE TABLE IF NOT EXISTS `stopwatch` ( `chat_id` int(10) unsigned NOT NULL, `timestamp` int(10) unsigned NOT NULL, PRIMARY KEY (`chat_id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
Create a Stopwatch Class
Finally we are ready to start coding. Let's create a class to work with the database in a file called stopwatch.php
and start with a constructor that will set two private variables, where we will store the chat ID and the current MySQL connection:
class Stopwatch { /** @var mysqli */ private $mysqli; /** @var int */ private $stopwatch_id; /** * Stopwatch constructor * @param mysqli $mysqli * @param $stopwatch_id */ public function __construct(\mysqli $mysqli, $stopwatch_id) { $this->mysqli = $mysqli; $this->stopwatch_id = intval($stopwatch_id); } }
When the user starts the timer, we will get the current Unix time and save it in a row with the chat ID, so here is the start()
method:
public function start() { $timestamp = time(); $query = " INSERT INTO `stopwatch` (`chat_id`, `timestamp`) VALUES ('$this->stopwatch_id', '$timestamp') ON DUPLICATE KEY UPDATE timestamp = '$timestamp' "; return $this->mysqli->query($query); }
If the timer stops, we need to delete a row from the database:
/** * Delete row with stopwatch id * @return bool|mysqli_result */ public function stop() { $query = " DELETE FROM `stopwatch` WHERE `chat_id` = $this->stopwatch_id "; return $this->mysqli->query($query); }
And now for the main part of the class. When the user requests the status of the timer, we need to find the row with the stopwatch from the current conversation and calculate the difference in seconds between the saved Unix time and the current time. Fortunately, Unix time is an integer, so we can just subtract one value from another. To format the resulting value as a time, we will use the gmdate
function.
/** * Find row with stopwatch id and return difference in seconds from saved Unix time and current time * @return string */ public function status() { $query = " SELECT `timestamp` FROM `stopwatch` WHERE `chat_id` = $this->stopwatch_id "; $timestamp = $this->mysqli->query($query)->fetch_row(); if (!empty($timestamp)) { return gmdate("H:i:s", time() - reset($timestamp)); } }
As you can see, if there is no value in the database, the method status()
will return nothing, and we will process a null value like a stopped timer.
Choosing a PHP Library
There are many PHP libraries that exist to work with the Telegram API, but, at least at the moment of writing this article, there's only one that supports both the Telegram Bot API wrapper and Botan tracking. And it's called PHP Telegram Bot API.
Use Composer to install this library:
composer require telegram-bot/api
If you're not interested in using analytics, try Telegram Bot API PHP SDK with Lavarel integration or PHP Telegram Bot.
Start the Webhook Script
And now the main part begins—we will create a script to process callbacks from the Telegram Bot API. Start a file called index.php
and include Composer autoload and a new Stopwatch class. Open a MySQL connection, create a new Telegram API client, and run it:
require_once 'vendor/autoload.php'; require_once 'stopwatch.php'; // connect to database $mysqli = new mysqli('database_host', 'database_user', 'database_password', 'database_name'); if (!empty($mysqli->connect_errno)) { throw new \Exception($mysqli->connect_error, $mysqli->connect_errno); } // create a bot $bot = new \TelegramBot\Api\Client('bot_token', 'botanio_token'); // run, bot, run! $bot->run();
Create Commands
Now we need to set up a bot to answer on command /start
. This command is used to start all Telegram bots, and users will be shown our welcome message when the first chat begins.
$bot->command('start', function ($message) use ($bot) { $answer = 'Howdy! Welcome to the stopwatch. Use bot commands or keyboard to control your time.'; $bot->sendMessage($message->getChat()->getId(), $answer); });
Here, in the command()
method, we defined a closure for receiving a command. This closure gets the ID of the current chat and sends a welcome message. Also, all registered commands are automatically tracked as the command name.
To start the stopwatch, we will define the /go
command:
$bot->command('go', function ($message) use ($bot, $mysqli) { $stopwatch = new Stopwatch($mysqli, $message->getChat()->getId()); $stopwatch->start(); $bot->sendMessage($message->getChat()->getId(), 'Stopwatch started. Go!'); });
This will create an instance of the Stopwatch class and start a timer calling the start()
method that we have defined already.
To define the /status
command, we need to do the same thing. Just call the status()
method and return the result. If the method returned null, tell the user that the timer is not started.
$bot->command('status', function ($message) use ($bot, $mysqli) { $stopwatch = new Stopwatch($mysqli, $message->getChat()->getId()); $answer = $stopwatch->status(); if (empty($answer)) { $answer = 'Timer is not started.'; } $bot->sendMessage($message->getChat()->getId(), $answer); });
And if the user stops the timer, we need to get the status first, show the resulting time, and stop the timer using the stop()
method.
$bot->command('stop', function ($message) use ($bot, $mysqli) { $stopwatch = new Stopwatch($mysqli, $message->getChat()->getId()); $answer = $stopwatch->status(); if (!empty($answer)) { $answer = 'Your time is ' . $answer . PHP_EOL; } $stopwatch->stop(); $bot->sendMessage($message->getChat()->getId(), $answer . 'Stopwatch stopped. Enjoy your time!'); });
That's it! Now you can upload all the files to the webhook directory and test your bot.
Adding a Keyboard
To suggest to the user which commands he or she can run, we can add a keyboard to a message. Our stopwatch can be running or stopped, and there will be two ones for each state. To show a keyboard to the user, we just need to extend the sendMessage()
method:
$keyboard = new \TelegramBot\Api\Types\ReplyKeyboardMarkup([['/go', '/status']], null, true); $bot->sendMessage($message->getChat()->getId(), $answer, false, null, null, $keyboards); });
Now you can add keyboards to every command of your bot. I will not include a full example here, but you can see it in the repository pages.
Adding Your Bot to a Store
Okay, so now we have working bot, and we want to show it to the world. The best way is to register the bot in a bot catalogue. For now, Telegram doesn't have an official catalogue like this, but there are a few unofficial ones, and the biggest is Storebot.me, where thousands of bots are already registered.
And there is a... bot to register your bot in a bot store! Add @storebot to your contact list, type the /add
command, and follow the instructions. You will be asked to enter the bot's username, name, and description, choose one of the standard categories, and confirm the bot's ownership by sending its token.

After a while, your bot will pass the submission process and appear in the Storebot charts. Now you and your users can vote, find and rate your bot in the bot store to help it rise to the top of the chart.
Conclusion
We've come a long way, from creating a baby bot to registering it in a store to be available to real users. As you can see, there are many tools that exist to make your life easier with creating and spreading your bot, and you don't need much code to start an easy bot. Now you are ready to make your own!
If you have any questions, don't hesitate to ask questions in the comments to the article.
Comments