Typically new features for web applications are tested by visiting the appropriate page in a browser, maybe filling out some form data, submitting the form, and then developers or testers hope to see their desired result. This is the natural way most web developers test their apps. We can continue with this natural testing process and improve upon it to ensure our apps are as stable as possible by using Codeception.
What Is Codeception?
Codeception is a multi-featured testing framework for PHP. It can handle unit, functional, and acceptance testing of web applications and it's powered by the already very popular PHPUnit testing framework.
Codeception allows us to test different kinds of user perspectives and site scenarios while they are visiting our app to ensure a pleasant user experience. By testing multiple scenarios, we can simulate a user's natural flow throughout our application to make sure the app is working as expecting.
Installation & Configuration
Let's start by creating a folder in our Sites
's directory (or wherever you prefer to store your web applications) to hold our sample application that we'll test with Codeception:
cd Sites mkdir codeception
Now I've already created a small sample HTML and PHP file that we can use to test with. You can just copy and paste it from below. We'll start with the toupper.html
file:
# codeception/toupper.html <!DOCTYPE html> <html> <head> <title>Convert Me!</title> </head> <body> <h1>Convert Me!</h1> <form action="toupper.php" method="post"> <label for="string">Convert to Uppercase:</label> <input type="text" name="string" id="string"> <input type="submit" value="Convert"> </form> </body> </html>
This page simply displays a form allowing a user to enter in a string of text and we'll convert it to uppercase using PHP. Next will be our PHP file which will process the form submission:
# codeception/toupper.php <?php $message = "No string entered"; if (!empty($_POST['string'])) { $message = "String converted: " . strtoupper($_POST['string']); } ?> <!DOCTYPE html> <html> <head> <title>To Upper!</title> </head> <body> <h1>To Upper!</h1> <p><?php echo $message; ?></p> <p><a href="toupper.html">Back to form</a>.</p> </body> </html>
This page creates a $message
variable to hold a default message. We then check to see if the form was submitted. If so, we replace the default message with the uppercase converted string, then we echo out the message and at the bottom of the page we have a link, linking back to the form.
A super simple PHP application, but it does allow us to try out Codeception's acceptance testing features.
Now let's download and install Codeception. Luckily it is very easy to install and configure. There are a couple of ways to install it using either Composer, Git, and installing the Phar. I prefer to use Composer, so let's create a composer.json
file in the root of our codeception
sample web app:
cd codeception touch composer.json
Next, open up composer.json
into your favorite text editor and add the following lines to download Codeception:
{ "require": { "codeception/codeception": "*" } }
Then run composer in your terminal:
composer update
To actually install it, run the following command:
./vendor/bin/codecept bootstrap
You'll notice after running the previous commands, we now have tests
and vendor
folders in our sample web app.
Next we just need to add our local application's URL into our tests/acceptance.suite.yml
file:
class_name: WebGuy modules: enabled: - PhpBrowser - WebHelper config: PhpBrowser: url: 'http://localhost/codeception/'
Ok, our sample application and Codeception should now be installed and ready to go.
Acceptance Testing
Acceptance testing allows us to test our applications using the normal website viewing process of visit a webpage, fill in a form, and submit the form to see the desired result. The difference is with Codeception, we don't have to waste time going to the browser each time we want to test a new feature out, instead we can just run our acceptance tests to see if they pass or not.
Generating an Acceptance Test
Now we need a file that we can write our tests in. Codeception makes it super simple to create different types of tests by using the codecept
generator scripts. Let's generate a Toupper
acceptance test:
./vendor/bin/codecept generate:cept acceptance Toupper
Here we run the codecept
command again and tell it to generate an acceptance test with the name of Toupper
(after file generation, the file will actually be named ToupperCept.php
).
Writing an Acceptance Test
When using Codeception you will likely come across the various different "Guys" that run the various different tasks for the framework. There's a CodeGuy, a TestGuy, and a WebGuy.
For acceptance testing, we'll be using the WebGuy. Let's open up our ToupperCept.php
file in our favorite text editor and begin by creating a new WebGuy that we can use to run our tests:
$I = new WebGuy($scenario);
Here we create a new WebGuy
object, storing it in an $I
variable. We can now use this object to test out different parts of our page to ensure everything is correct.
Let's make sure that our Toupper web page is loading up properly first, then we'll test out the form submission:
$I = new WebGuy($scenario); $I->wantTo('ensure Toupper form works'); $I->amOnPage('toupper.html'); $I->see('Convert Me!');
In the above code we use our $I
object and call several of its methods to test out our page. We start by stating what we're wanting to do. In this case, we're just wanting to make sure our Toupper form works using the wantTo
method. Then we use the amOnPage
method to ensure we're on the correct page of toupper.html
. Finally we call the see
method to ensure we see the text: Convert Me! on the webpage.
Executing Our Test
Now that we have a basic test to see if we're on the right page and that we're seeing the correct content, let's run the test using the following command:
./vendor/bin/codecept run
This will run all test suites. You should see the following output in your console, showing a successful test:
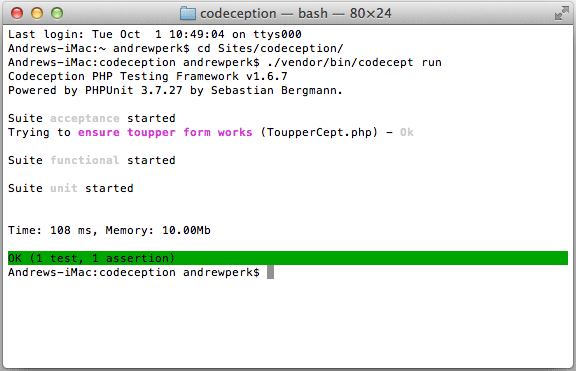
If you'd like to run only your acceptance tests, you can use the following command:
./vendor/bin/codecept run acceptance
Additionally, you can see the full list of actions performed by using the --steps
flag:
./vendor/bin/codecept run acceptance --steps
Here's what your console would look like:
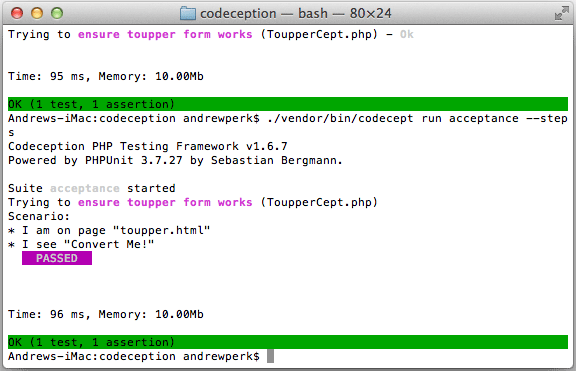
Testing Forms
Next, let's see how we can use Codeception to test out the functionality of our form. Let's add the following into our ToupperCept.php
file:
$I = new WebGuy($scenario); $I->wantTo('ensure Toupper form works'); $I->amOnPage('toupper.html'); $I->see('Convert Me!'); $I->fillField('string', "Convert me to upper"); $I->click('Convert'); $I->amOnPage('toupper.php'); $I->see('To Upper!');
Here we just continue where we left off. After ensuring we're on the right page, we then fill in the form using the fillField
method passing it the field name and the value we'd like to use and then we click the Convert button. Afterwards, we verify we are now on the toupper.php
page and that we're seeing our heading of To Upper!.
Let's run our tests again:
./vendor/bin/codecept run
Your console should have something similar to below, confirming the tests have passed:
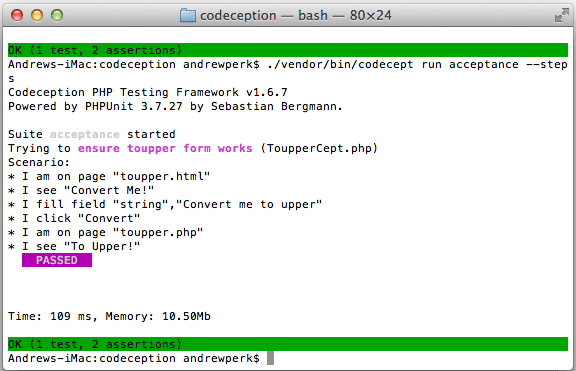
Testing Links
Now lastly, let's test out the link that's on our toupper.php
page to ensure it takes us back home:
$I = new WebGuy($scenario); $I->wantTo('ensure Toupper form works'); $I->amOnPage('toupper.html'); $I->see('Convert Me!'); $I->fillField('string', "Convert me to upper"); $I->click('Convert'); $I->amOnPage('toupper.php'); $I->see('To Upper!'); $I->click('Back to form'); $I->see('Convert Me!');
Again, we call the click
method to click on our link and to make sure we're back on the right page, we verify that we see the text Convert Me!.
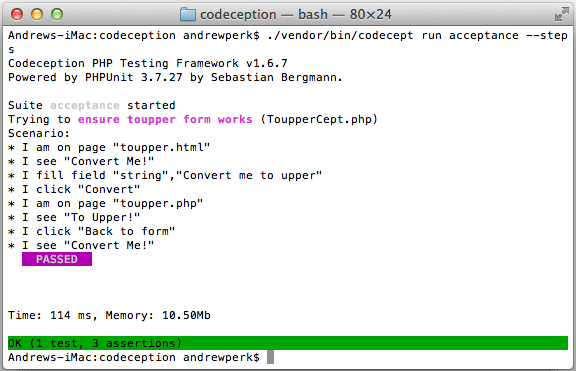
In Conclusion
Now that you know the basics of working with Codeception you should give it a try on your own. Go ahead and attempt to add a few more tests to ensure that the form displays the: No string entered error message if you submit the form empty.
Here's my final code after doing the above and the resulting passed tests:
$I = new WebGuy($scenario); $I->wantTo('ensure Toupper form works'); $I->amOnPage('toupper.html'); $I->see('Convert Me!'); $I->fillField('string', "Convert me to upper"); $I->click('Convert'); $I->amOnPage('toupper.php'); $I->see('To Upper!'); $I->click('Back to form'); $I->see('Convert Me!'); $I->fillField('string', ''); $I->click('Convert'); $I->amOnPage('toupper.php'); $I->see('No string entered');
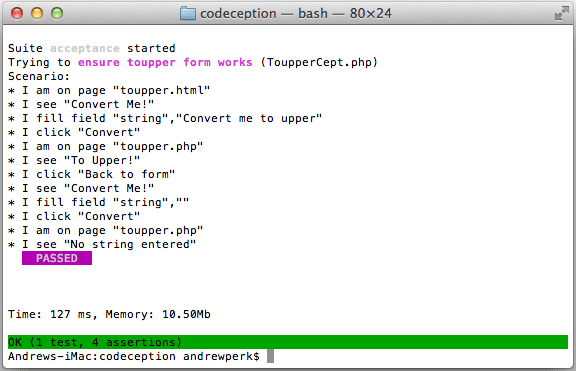
So to wrap up, Codeception is an awesome testing framework that allows you to write very readable tests, easily. Be sure to checkout the full documentation for more detailed examples and for information regarding the other testing paradigms.
Comments