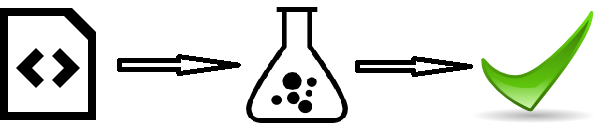
HTTP mock tests let you implement and test a feature even if dependent services are not implemented yet. Also, you can test services that have already been implemented without actually calling those services—in other words you can test them offline. You need to analyze your business needs for that application first, and write them as scenarios in your design documents.
Let's say that you are developing a NodeJS client API for a media client that is a service (there is no such service in real life, just for testing) for music, video, pictures, etc. There will be lots of features in this API, and there might be a need for some changes from time to time.
If you have no tests for this API, you will not know what problems it will cause. However, if you have tests for this API, you can detect issues by running all the tests you have written before. If you are developing a new feature, you need to add specified test cases for that. You can find an API that's already been implemented in this GitHub repository. You can download the project and run npm test
in order to run all the tests. Let’s continue the test part.
For an HTTP mock test, we will use nock, which is an HTTP mocking and expectations library for NodeJS. In HTTP mock testing, you can apply the following flow:
- Define a Request/Response mock rule.
- Create a test and use your function in the test.
- Compare the expected results with the actual results in the test callback.
Simple Testing
Let's think about a media client API. Let's say you call the function musicsList
by using an instance of the Media
library as below:
var Media = require('../lib/media'); var mediaClient = new Media("your_token_here"); mediaClient.musicsList(function(error, response) { console.logs(response); })
In this case, you will get a music list in the response
variable by making a request to https://ap.example.com/musics
inside this function. If you want to write for this, you need to mock requests in order to make your tests run offline. Let's simulate this request in nock.
describe('Music Tests', function () { it('should list music', function (done) { nock('https://api.example.com') .get('/musics') .reply(200, 'OK'); mediaClient.musicList(function (error, response) { expect(response).to.eql('OK') done() }) }) })
describe('Musics Tests', function() .....
is for grouping your tests. In the above example, we are grouping music related tests under the same group. it('should list music', function(done)....
is for testing specific actions in music-related functions. In each test, the done
callback is provided in order to check the test result inside the callback function of the real function. In the mock request, we assume that it will respond OK
if we call the musicList
function. The expected and actual result is compared inside the callback function.
Matching From a File
You can define your request and response data inside a file. You can see the below example for matching response data from a file.
it('should create music and respond as in resource file', function (done) { nock('https://api.example.com') .post('/musics', { title: 'Smoke on the water', author: 'Deep Purple', duration: '5.40 min.' }) .reply(200, function (uri, requestBody) { return fs.createReadStream(path.normalize(__dirname + '/resources/new_music_response.json', 'utf8')) }); mediaClient.musicCreate({ title: 'Smoke on the water', author: 'Deep Purple', duration: '5.40 min.' }, function (error, response) { expect(JSON.parse(response).music.id).to.eql(3164495) done() }) })
When you create a piece of music, the response should match the response from the file.
Chaining Mock Requests
You can also chain a mock request in one scope as below:
it('it should create music and then delete', function(done) { nock('https://api.example.com') .post('/musics', { title: 'Maps', author: 'Maroon5', duration: '5:00 min.' }) .reply(200, { music: { id: 3164494, title: 'Maps', author: 'Maroon5', duration: '7:00 min.' } }) .delete('/musics/' + 3164494) .reply(200, 'Music deleted') mediaClient.musicCreate({ title: 'Maps', author: 'Maroon5', duration: '5:00 min.' }, function (error, response) { var musicId = JSON.parse(response).music.id expect(musicId).to.eql(3164494) mediaClient.musicDelete(musicId, function(error, response) { expect(response).to.eql('Music deleted') done() }) }) })
As you can see, you can mock music creation and response first, and then music deletion, and finally the response to other data.
Matching Request Headers
In the media client, Content-Type: application/json
is provided in request headers. You can test a specific header like this:
it('should provide token in header', function (done) { nock('https://api.example.com', { reqheaders: { 'Content-Type': 'application/json' } }) .get('/musics') .reply(200, 'OK') mediaClient.musicList(function(error, response) { expect(response).to.eql('OK') done() }) })
When you make a request to https://api.example.com/musics
with a header Content-Type: application/json
, it will be intercepted by the nock HTTP mock above, and you will be able to test the expected and actual result. You can also test response headers in the same way just by stating the response header in the reply section:
it('should provide specific header in response', function (done) { nock('https://api.example.com') .get('/musics') .reply(200, 'OK', { 'Content-Type': 'application/json' }) mediaClient.musicList(function(error, response) { expect(response).to.eql('OK') done() }) })
Reply With Default Headers
You can specify default reply headers for the requests in one scope by using defaultReplyHeaders
as follows:
it('should provide default response header', function(done) { nock('https://api.example.com') .defaultReplyHeaders({ 'Content-Type': 'application/json' }) .get('/musics') .reply(200, 'OK, with default response headers') mediaClient.musicList(function(error, response) { expect(response).to.eql('OK, with default response headers') done() }) })
When you call the API, you will find default reply headers in the response, even if it is not specified.
HTTP Operations
HTTP operations are the fundamentals of client requests. You can intercept any HTTP operation by using intercept
:
scope('http://api.example.com') .intercept('/musics/1', 'DELETE') .reply(404);
When you try to delete music with id 1, it will respond 404. You can compare your actual result with 404 to check the test status. Also you can use GET
, POST
, DELETE
, PATCH
, PUT
, and HEAD
in the same way.
Response Repetition
Normally, mock requests made by using nock are available for the first time. You can make it available as much as you want like this:
var scope = nock('https://api.example.com') .get('/musics') .times(2) .reply(200, 'OK with music list'); http.get('https://api.example.com/musics'); // "OK with music list" http.get('https://apiexample.com/musics'); // "OK with music list" http.get('https://apiexample.com/musics'); // "Real response from api"
You are limited to doing two requests as mock HTTP requests. When you make three or more requests, you will automatically make a real request to the API.
Custom Port
You can also provide a custom port in your API URL:
it('should handle specific port', function(done) { nock('https://api.example.com:8081') .get('/') .reply(200, 'OK with custom port') request('https://api.example.com:8081', function(error, response, body) { expect(body).to.eql('OK with custom port') done() }) })
Scope Filtering
Scope filtering becomes important when you want to filter the domain, protocol, and port for your tests. For example, the below test will let you mock requests that have sub-domains like API0, API1, API2, etc.
it('should also support sub domains', function(done) { nock('http://api.example.com', { filteringScope: function(scope) { return /^http:\/\/api[0-9]*.example.com/.test(scope); } }) .get('/musics') .reply(200, 'OK with dynamic subdomains') request('http://api2.example.com/musics', function(error, response, body) { expect(body).to.eql('OK with dynamic subdomains') done() }) })
You are not limited to one URL here; you can test sub-domains specified in the regexp
.
Path Filtering
Sometimes your URL parameters may vary. For example, pagination parameters are not static. In that case, you can use the following test:
it('should support dynamic pagination', function(done) { nock('http://api.example.com') .filteringPath(/page=[^&]*/g, 'page=123') .get('/musics?page=123') .reply(200, 'Ok response with paginate') request('http://api.example.com/musics?page=13', function(error, response, body) { expect(body).to.eql('Ok response with paginate') done() }) })
Request Body Filtering
If you have varying fields in your request body, you can use filteringRequestBody
to eliminate varying fields like this:
it('should create movie with dynamic title', function(done) { nock('http://api.example.com') .filteringRequestBody(function(path) { return 'test' }) .post('/musics', 'test') .reply(201, 'OK'); var options = { url: 'http://api.example.com/musics', method: 'POST', body: 'author=test_author&title=test' } request(options, function(err, response, body) { expect(body).to.eql('OK') done() }) })
Here author
may vary, but you can intercept the request body with title=test
.
Request Header Match
In this client, a token is used to authenticate the client user. You need to check the header to see a valid token in the Authorization
header:
it('should match bearer token header', function(done) { nock('https://api.example.com') .matchHeader('Authorization', /Bearer.*/) .get('/musics') .reply(200, 'Ok response with music list') mediaClient.musicList(function(error, response) { expect(response).to.eql('Ok response with music list') done() }) })
Turn Mocking On or Off
In test cases, you can enable real HTTP requests by setting allowUnmocked
as true. Let's look at the following case:
it('should request performed to "http://api.example.com"', function(done) { var scope = nock('http://api.example.com', {allowUnmocked: true}) .get('/musics') .reply(200, 'OK'); http.get('http://api.example.com/musics'); // This will intercepted by the nock http.get('http://api.example.com/videos'); // This will make real request to service })
In a nock scenario, you can see a scenario for the /musics
URI, but also if you make any URL different from /musics
, it will be a real request to the specified URL instead of a failing test.
Other Utilities
We have covered how to write scenarios by providing a sample request, response, header, etc., and checking the actual and expected result. You can also use some expectation utilities like isDone()
, clear the scope scenario with cleanAll()
, use a nock scenario forever by using persist()
, and list pending mocks inside your test case by using pendingMocks()
.
isDone()
Let's say that you have written a nock scenario and execute a real function to a test scenario. Inside your test case, you can check whether the written scenario is executed or not as follows:
it('should request performed to "http://api.example.com"', function(done) { var musicList = nock('http://api.example.com') .get('/musics') .reply(200, 'OK with music list'); request('http://api.example.com/musics', function(error, response){ expect(musicList.isDone()).to.eql(true) done() }) })
Inside the request callback, we are expecting that URL in the nock scenario (http://api.example.com/musics) to be called.
cleanAll()
If you use a nock scenario with persist()
, it will be available forever during the execution of your test. You can clear the scenario whenever you want by using this command as shown in the following example:
it('should failed due to scope clearing', function(done) { var musicList = nock('http://api.example.com') .get('/musics') .reply(200, 'OK with music list'); nock.cleanAll(); request('http://api.example.com/musics', function(error, response, body){ expect(body).not.eql('OK with music list') done() }) })
In this test, our request and response match with the scenario. However, after nock.cleanAll()
we reset the scenario, and we are not expecting a result with 'OK with music list'
.
persist()
Every nock scenario is available only the first time. If you want to make it live forever, you can use a test like this one:
it('should be available for infinite request', function(done) { nock('http://api.example.com') .get('/musics') .reply(200, 'OK') // First call request('https://api.example.com/musics', function(error, response, body) { expect(body).to.eql('OK') done() }) // Second call request('https://api.example.com/musics', function(error, response, body) { expect(body).to.eql('OK') done() }) // ....... })
pendingMocks()
You can list the pending nock scenarios that are not done yet inside your test as follows:
it('should log pending mocks', function(done) { var scope = nock('http://api.example.com') .persist() .get('/') .reply(200, 'OK'); if (!scope.isDone()) { console.error('Waiting mocks: %j', scope.pendingMocks()); } })
You may also want to see the actions performed during test execution. You can manage to do that by using the following example:
it('should log all the request', function(done) { var musicList = nock('http://api.example.com') .log(console.log) .get('/musics') .reply(200, 'OK with music list'); request('http://api.example.com/musics', function(error, response, body){ expect(body).eql('OK with music list') done() }) })
This test will generate an output as shown below:
matching GET http://api.example.com:80/musics to GET http://api.example.com:80/musics: true
Quick Tips
Sometimes, you need to disable mocking for specific URLs. For example, you may want to call the real http://tutsplus.com
, instead of the mocked one. You can use:
nock.nock.enableNetConnect('tutsplus.com');
This will make a real request inside your tests. Also, you can globally enable/disable by using:
nock.enableNetConnect(); nock.disableNetConnect();
Note that, if you disable net connect, and try to access an unmocked URL, you will get a NetConnectNotAllowedError
exception in your test.
Conclusion
It is best practice to write your tests before real implementation, even if your dependent service is not implemented yet. Beyond this, you need to be able to run your tests successfully when you are offline. Nock lets you simulate your request and response in order to test your application.
Comments