There are several methods used to produce menus within Unity, the main two being the built in GUI system and using GameObjects with Colliders that respond to interactions with the mouse. Unity's GUI system can be tricky to work with so we're going to use the GameObject approach which I think is also a bit more fun for what we're trying to achieve here.
Final Result Preview
Step 1: Determine Your Game Resolution
Before designing a menu you should always determine what resolution you are going to serve it to.
Open the Player settings via the top menu, Edit > Project Settings > Player and enter your default screen width and height values into the inspector. I chose to leave mine as the default 600x450px as shown below.
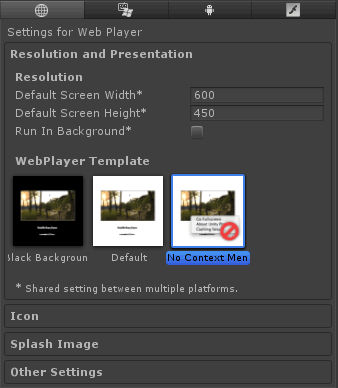
You then need to adjust the size of your Game view from the default "Free Aspect" to "Web (600 x 450)", else you could be positioning your menu items off the screen.
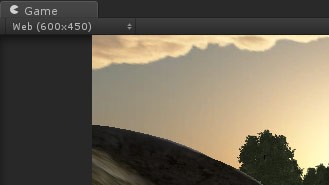
Step 2: Choosing a Menu Background
As you will have seen in the preview, our menu scene is going to have our game environment in the background so that when you click Play you enter seamlessly into the game.
To do this you need to position your player somewhere in the scene where you like the background and round up the Y rotation value. This is so it's easier to remember and to replicate later, so that the transition can be seamless from the menu into the game.
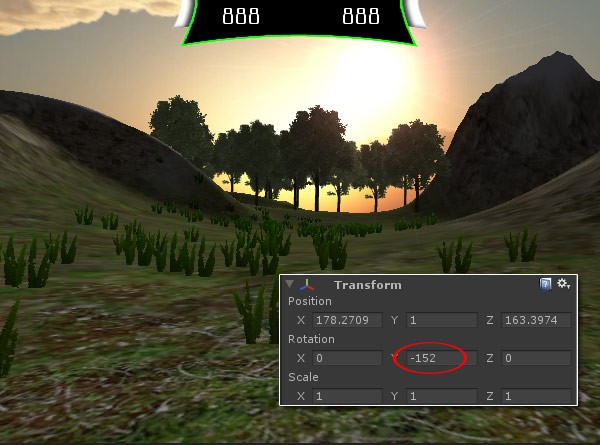
Let's now get on with the creation of the menu scene!
Step 3: Creating the Menu Scene
Make sure your scene is saved and is called "game" - you'll see why later.
Select the game scene within the Project view and duplicate it using Ctrl/Command + D, then rename the copy to "menu" and double-click it to open it.
Note: You can confirm which scene is open by checking the top of the screen, it should say something like "Unity - menu.unity - ProjectName - Web Player / Stand Alone". You don't want to start deleting parts accidently from your game scene!
Now select and delete the GUI and PickupSpawnPoints GameObjects from the Hierarchy. Expand the "Player" and drag the "Main Camera" so that it's no longer a child of the Player, then delete the Player.
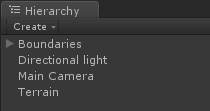
Next, select the terrain and remove the Terrain Collider Component by right-clicking and selecting Remove Component.
Finally, select the "Main Camera" and remove the MouseLook Component by right-clicking and selecting Remove Component.
If you run the game now nothing should happen and you shouldn't be able to move at all. If you can move or rotate then redo the above steps.
Step 4: Adjusting the Build Settings
Currently when you build or play your game the only level included within that build is the "game" scene. This means that the menu scene will never appear. So that we can test our menu, we'll adjust the build settings now.
From the top menu select File > Build Settings and drag the menu scene from your Project View into the Build Settings' "Scenes In Build" list as shown below
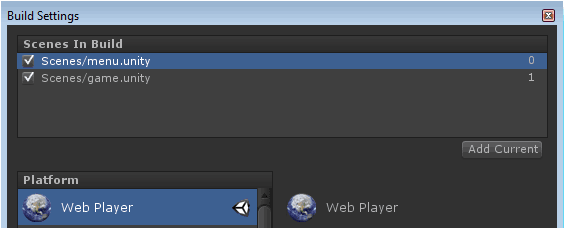
(Make sure you rearrange the scenes to put "menu.unity" at the top, so that it's the scene that's loaded first when the game is played.)
Perfect!
Step 5: Adding the Play Button
We're going to use 3D Text for our menu, so go ahead and create a 3D Text GameObject via the top menu: GameObject > Create Other > 3D Text, then rename it "PlayBT". Set the Y rotation of the PlayBT text to match the Y rotation value of your Main Camera so that it's facing directly at it and therefore easily readable.
With the PlayBT selected, change the Text Mesh text property from Hello World to "Play", reduce the Character Size to 0.1 and increase the Font Size to 160 to make the text crisper.
Note: If you want to use a font other than the default, either select the font before creating the 3D Text or drag the Font onto the 3DText's TextMesh's Font property and then drag the Fonts "Font Material" onto the Mesh Renderers Materials list, overwriting the existing Font Material. Quite a hassle I know!
Finally, add a Box Collider via the top menu: Component > Physics > Box Collider. Resize the Box Collider to fit the text if it doesn't fit it nicely.
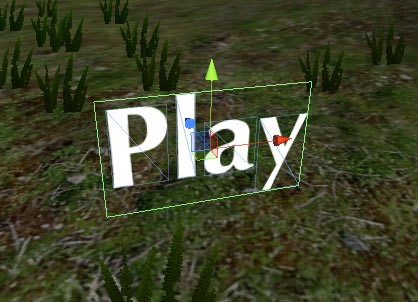
At this point in the tutorial you really need to have both the Scene and Game Views open at the same time since you are now going to move the PlayBT within the Scene View so that it's centred within your Game View as shown below. I recommend first positioning it horizontally using a top down view and then revert to using a perspective view to position it vertically using the axis handles.
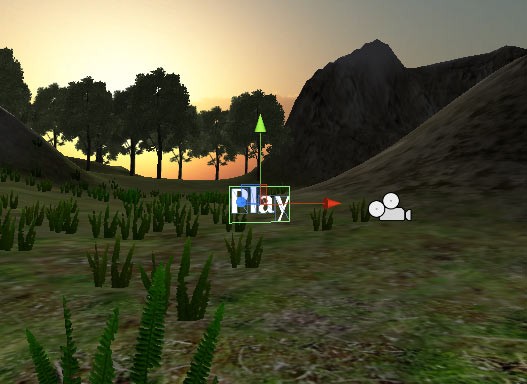
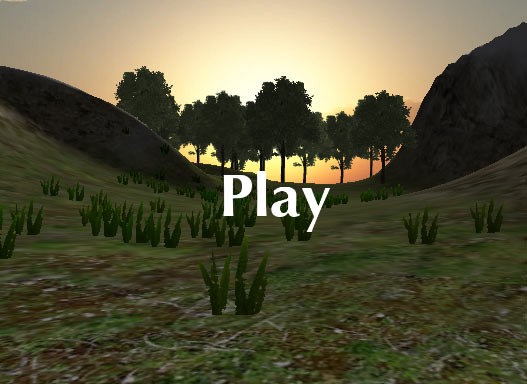
So that's our Play button all set up. Now let's make it play!
Step 6: The Mouse Handler Script
Create a new JavaScript script within your scripts folder, rename it "MenuMouseHandler" and add it as a Component of the PlayBT GameObject by either dragging in directly onto PlayBT or by selecting PlayBT and dragging the script onto it's Inspector.
Replace the default code with the following:
/** Mouse Down Event Handler */ function OnMouseDown() { // if we clicked the play button if (this.name == "PlayBT") { // load the game Application.LoadLevel("game"); } }
We're using the MonoBehaviour OnMouseDown(...) function, invoked every time the BoxCollider is clicked by the mouse. We check whether the button clicked is called "PlayBT", and if so we use Application.LoadLevel(...) to load the "game" scene.
Enough talk - go run it and watch your game come to life when you click Play!
Note: If you click Play and have found yourself with a build settings error, don't fret; you just need to check your build settings - revisit Step 4.
Step 7: Ending the Game
So the menu to start the game is great but the game technically never ends since when the timer runs out nothing happens... let's fix that now.
Open the CountdownTimer script and at the bottom of the Tick()
function add the following line:
Application.LoadLevel("menu");
Now re-run your game and when the timer runs out you'll be taken back to the menu! Easy Peasy!
There we go - a basic menu added to our game. Now let's make it a little more user friendly with a help screen to explain how to play.
Step 8: Adding the Help Button
The help button is identical to the PlayBT in practically every way, so go ahead and duplicate the PlayBT, rename it to HelpBT and position it below the Play button. Adjust the text property to say "Help" rather than "Play" and perhaps make the Font Size a little smaller as shown below - I used 100.
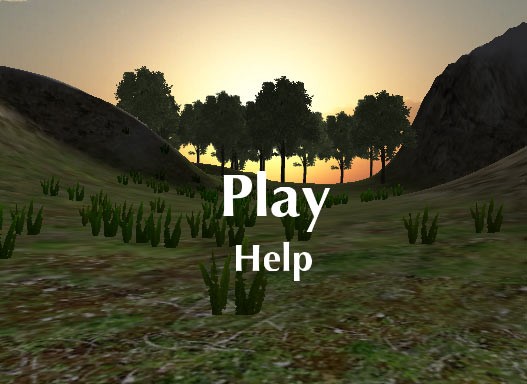
Now open the MenuMouseHandler script and add the following else if
block to your existing if
statement.
// if we clicked the help button else if (this.name == "HelpBT") { // rotate the camera to view the help "page" }
If you check the preview you'll see that when you click Help the camera rotates around to show the help menu. So, how do we do that?
Step 9: God Save iTween
Our camera rotation can all be done nice and cleanly in one line, thanks to iTween. Without iTween life wouldn't be nearly as fun. As the name may give away it's a tweening engine, built for Unity. It's also free.
Go ahead and open iTween within the Unity Asset store, then click Download/Import and import it all into your project. Once it's imported you need to move the iTween/Plugins directory into the root of your Assets folder.
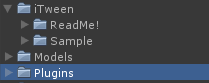
You're now all set to tween your life away!
Step 10: Rotating the Camera
Grab a piece of paper and a pen (or open a blank document) and make a note of your Main Camera's Y rotation value, as circled below.
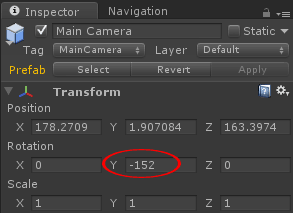
Within the scene view rotate the camera around in whichever direction you like around the Y axis so that the Play and Help text are out of view and so that you've got a decent background for your help page. You can see mine below: I rotated from -152 to -8.
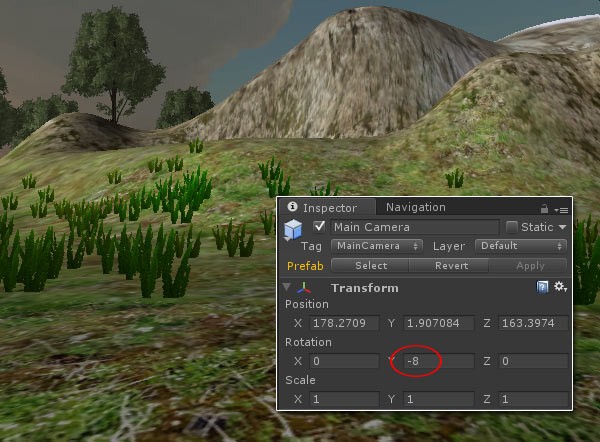
Return to your MenuMouseHandler script and add the following line within the else if
statement:
iTween.RotateTo(Camera.main.gameObject, Vector3(0, -8, 0), 1.0);
We use Camera.main to retrieve the main camera (defined by the "MainCamera" tag) from the scene and use iTween.RotateTo(...) to rotate the camera to a specific angle - in my case -8
.
(You need to replace the -8
within the above line with your camera's current rotation.)
Now go back to your scene and return your camera back to its original rotation that you wrote down at the start of this section, so that it's facing the PlayBT. Run your game, click Help and the camera should spin around. Woo!
Note: If you get an error about iTween not existing then you haven't imported it properly - revisit Step 9.
Now let's build our Help page.
Step 11: Building the Help Page
Rotate your camera back to the Y rotation of your help page - in my case -8
.
Now we're going to add a little explanation text as well as some more text to explain the different pickups and their scores. Finally, we'll add a Back button to return to the main menu. You can arrange your page in whatever way you wish so feel free to get creative. Here we go...
Duplicate the HelpBT, rename it HelpHeader, set its rotation to that of your camera, change the Anchor value to "upper center" and reduce the Font Size - I used 60.
Next, copy and paste the below paragraph into the text
property:
"Collect as many Cubes as you can within the time limit.
Watch out though, they change over time!
Note: It's worth noting that you can't type multiline text into the text property; you have to type it in another program and then copy and paste it since pressing enter/return assigns the field.
Finally remove the Box Collider and MenuMouseHandler Components within the Inspector since this text won't need to be clickable. Hopefully you end up with something like this:
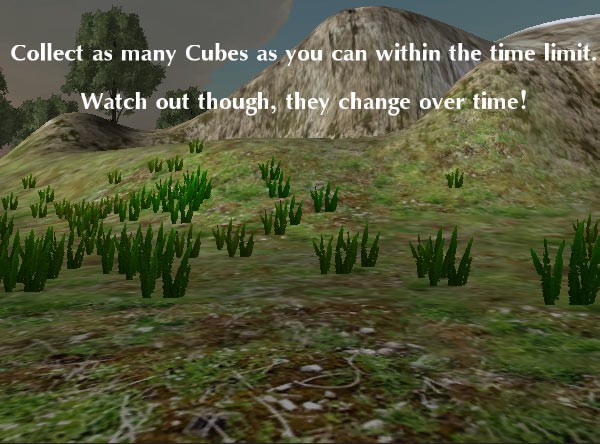
Now drag a pickup prefab into the scene and position it on the screen. I put mine as shown below.
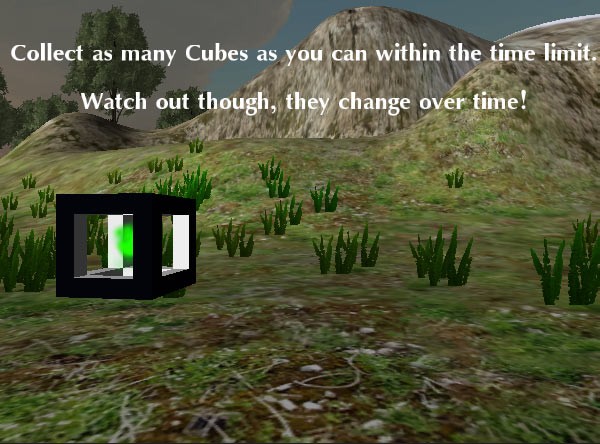
Next, duplicate the HelpHeader, rename it to HelpPowerups, change the Anchor to "upper-left" and copy and paste the below paragraph into the text field.
"Green: + 2 Points
Pink: +/- Random Points
Blue: Random Speed Boost"
Reposition it so you have something like the below.
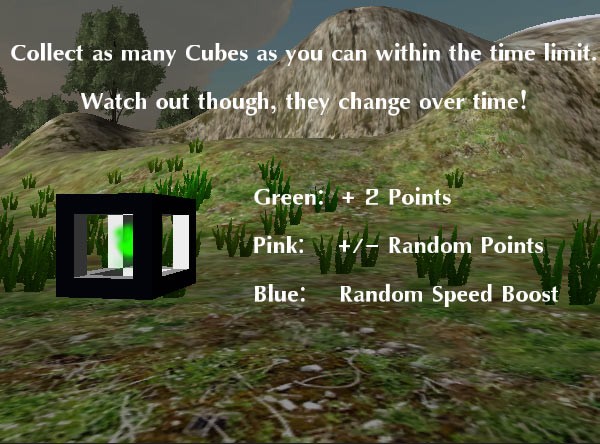
All that's left now is to add a Back button to return to the main menu.
Step 12: The Help Page Back Button
Duplicate the HelpBT, rename it BackBT, change its text to "Back", set its rotation to that of your camera and use the Scene View to reposition it within the Game View. I placed mine in the bottom corner as shown here:
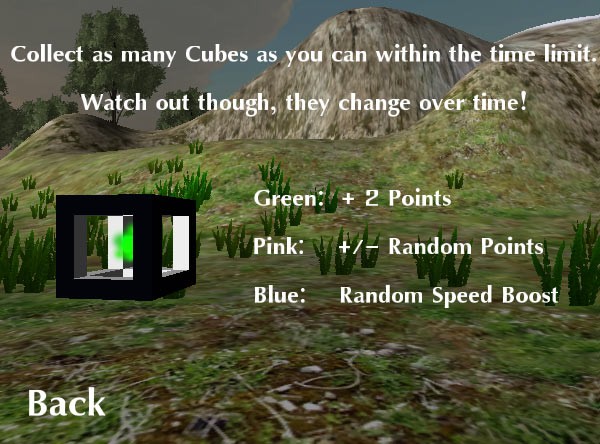
Now we just need to update our MenuMouseHandler script to handle mouse clicks from the BackBT as well. Add the following else if
block to the bottom of the OnMouseDown()
if
statements:
// if we clicked the Back button else if (this.name == "BackBT") { // rotate the camera to view the menu "page" iTween.RotateTo(Camera.main.gameObject, Vector3(0, -152, 0), 1.0); }
This is nearly identical to the previous iTween statement, the only difference being the angle the camera is rotated to - -152
in my case. You need to change this number to the original Y rotation of your camera was (the one you wrote down, remember?)
Now all you need to do it set your camera back to its original rotation - the value you just added to the iTween statement - so that it's facing the main menu again.
Run the game and your camera should spin round to reveal the help page and spin back round to the main menu. Congratulations, you've finished!
Conclusion
I hope you've enjoyed this Getting Started with Unity Session!
In this part we've covered using GameObjects as menu items and the incredibly powerful tweening library, iTween.
If you want an extra challenge, try using iTween to change the text colour on MouseOver and then back again on MouseExit. (You'll find a list of Mouse handlers on this page.)
Or add an iTween CameraFade and then fade it out when the timer runs out, then load then menu - rather than abruptly ending the game. You could then delay the call to Application.LoadLevel(...)
using yield WaitForSeconds(...)
.
Let me know how you get on in the comments!
Comments