In this tutorial, you will learn the basics of the Lua programming language and I will help you getting started with writing applications for iOS and Android. Excited? Let's dive right in.
Introduction
In this tutorial, we will take a look at the Corona SDK and the Lua programming language. Even though Lua isn't difficult to pick up, it is recommended to have some experience with other languages, such as JavaScript, PHP, Java, or Ruby. We will cover the very basics of the Corona SDK and Lua to get you familiar with developing for the Corona platform. You will see that it takes very little effort and code to get up and running. I'm ready when you are.
1. Introducing Lua and the Corona SDK
In the past, mobile developers faced a difficult predicament. Should they develop applications for iOS or Android? Most iOS developers use Objective-C, while Android developers use Java. Fortunately, we've got the Corona SDK and the Lua programming language, which enables cross platform mobile development. To put it simply, it means you can develop an application once and build it for iOS, Android, Kindle, and Nook.
The programming language we use when developing with the Corona SDK is Lua, which is moon in Portuguese. One of the main beneits of Lua, especially in combination with the Corona SDK, is that Lua is cross-platform as the language is written in C. Lua is not difficult to learn as you'll find out in this tutorial. Lua was created in 1993 by a small group of people at the Pontifical Catholic University of Rio de Janeiro, Brazil. Lua is open source software so you can freely use it in your projects. It is distributed under the MIT license.
The Corona SDK is developed and maintained by Corona Labs and is a commercial platform. There are several pricing plans to choose from. There's a free starter plan and paid plans starting from $19 per month.
For this tutorial, however, we'll be using the starter plan. Even though you can develop Corona applications on several platforms, in this tutorial I'll show you how to build applications using Windows and we'll build for the Android platform.
2. Setting Up Lua and the Corona SDK
Are you ready to get started with cross platform mobile development? Visit the developer portal of the Corona SDK, create an account, and download the Corona SDK. As I mentioned, in this tutorial I'll be using Windows, but you can follow along on OS X just as well. After the installation of the Corona SDK, open the Start menu and navigate to Corona SDK > Corona Simulator. You should see two windows as shown below.
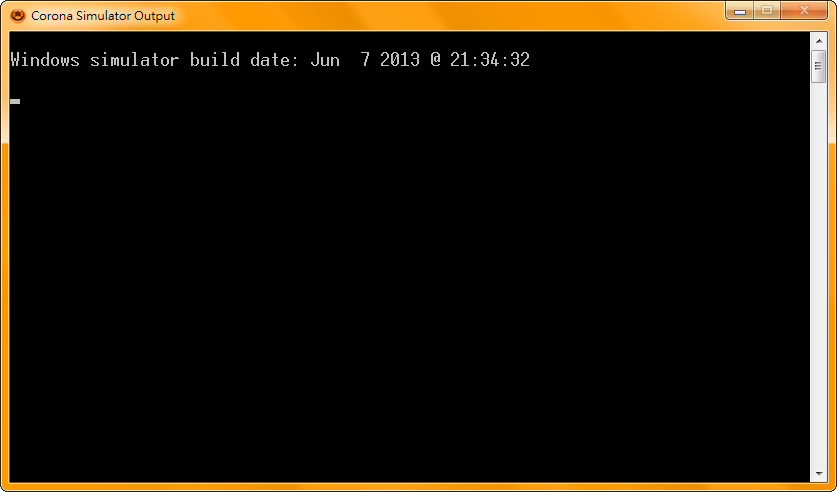
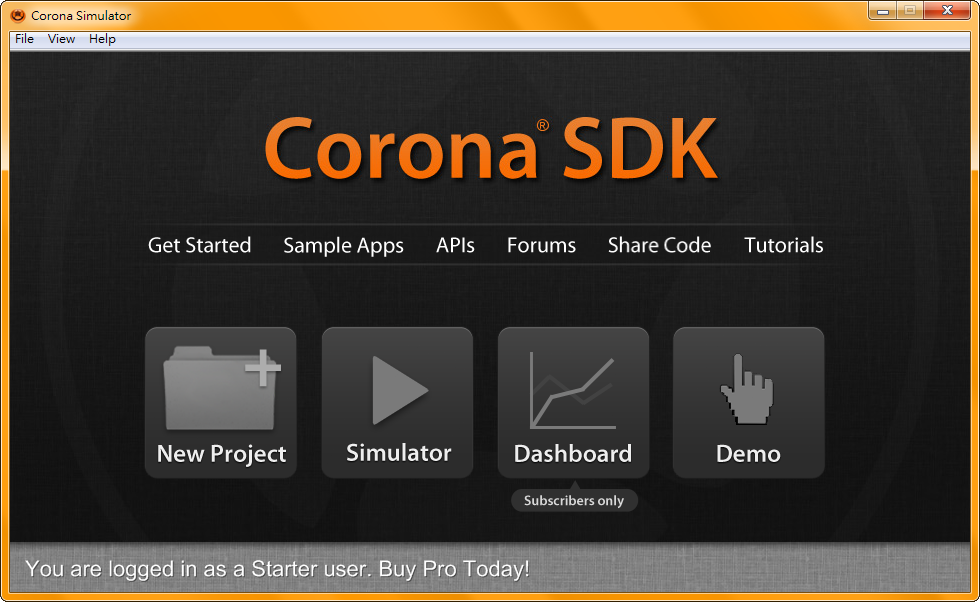
3. Text Editors
Now that we have the Corona Simulator up and running, we need to get a text editor to write and edit Lua. I recommend Sublime Text 2, which is a free download. It's a great and popular text editor that support syntax highlighting and a boatload of other useful features. This is especially useful if you're writing large and complex applications. It supports Lua along with 43 other programming languages. Did I tell you Sublime Text if available on Windows, OS X as well as Linux? You can't go wrong with Sublime Text 2.
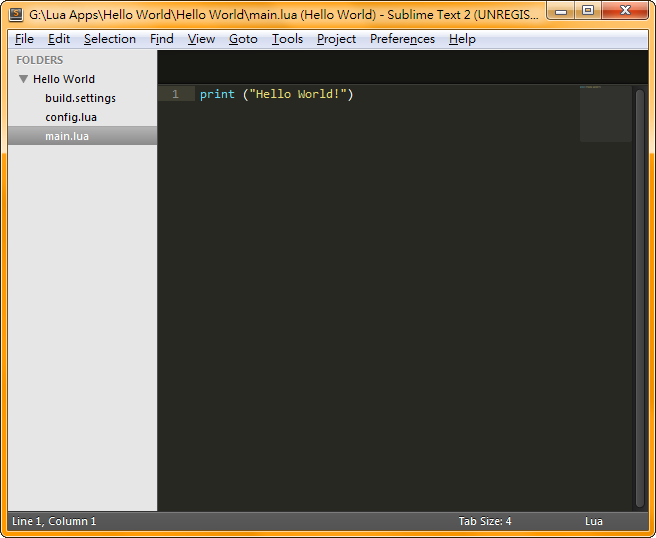
4. Writing a Hello World Application
Head back to the Corona Simulator, hit New Project, and choose a directory to store your project's files in. Select Blank as the project's template, Phone Preset for Upright Screen Size, and Upright as the Default Orientation. Click OK to finalize the project setup and navigate to the directory where you created your new project. You should find three files, build.settings, config.lua, and main.lua. The only file we'll need to edit is Main.lua. Open this file with your editor of choice and replace the file's contents with the code snippet below.
print("Hello World!");
Save the changes by pressing Ctrl+S
and open the Corona Simulator window. Wait a minute. Nothing happened. That's perfectly normal. We need to check the other window that looks like command prompt. It should display Hello World! as shown below.
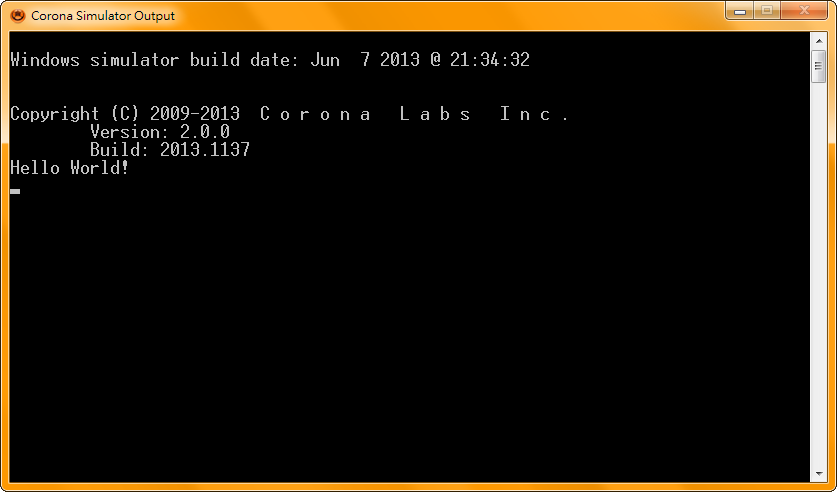
The reason why the text was only displayed in the Corona Terminal and not in the Corona Simulator is, because the print
command is only used for the Lua programming language. It cannot be used to display the words on the screen of the Corona Simulator or a physical device. However, this basic print
command will still be useful when we develop application, especially for debugging purposes.
5. Hello World - Take 2
We're going to create another Hello World! application. However, this time, we'll make it display the words in the Corona Simulator itself. Delete the contents of main.lua and replace it with the code snippet shown below.
display.newText("Hello World!", 0, 0, native.systemFont, 16);
You may have noticed that this snippet was a bit longer than the previous one. Let's see what this piece of code does for us.
-
display
is the object we're talking to. -
newText
is the function that we use to display the text on the screen. -
"Hello@ World!"
is the text we want to display. -
0, 0
are thex
andy
coordinates respectively. -
native.systemFont
is the font we use for the text and16
is the font's size.
If you save the changes and relaunch the Corona Simulator, you should see the following.
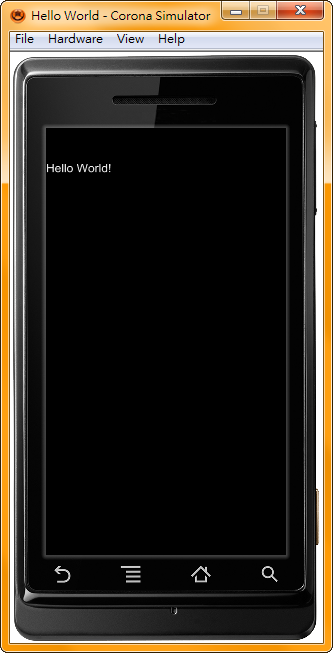
6. Variables and Math
What if you wanted to store a number as a variable for later use? The following code snippet shows how variables are declared in Lua.
local num1 = 3 + 3;
-
local
is the keyword for declaring a variable. -
num1
is the name of the variable.
If we combine this with the previous code snippet, we get the following.
local num1 = 3 + 3; display.newText(num1, 0, 0, native.systemFont, 32);
The Corona Simulator should now display the number 6
, which is the result of adding 3
and 3
. Let's try another example using math. Using the following code snippet, the Corona Simulator should display the number 18
.
local num1 = 3 + 3; display.newText(num1 * 3, 0, 0, native.systemFont, 32);
As you can see, it is perfectly possible to perform mathematical operations on a variable. In the above code snippet, we multiplied num1
by 3
using * 3
. I'm sure you've already figured out that the asterisk is the multiplication operator in Lua.
-
+
for addition -
-
for subtraction and negative numbers -
*
for multiplication -
/
for division
7. Images
Displaying images isn't difficult either. To display an image, you need to add the image to the directory where main.lua sits. It is fine to create a subdirectory to keep the project's resources separated and organized. Let's do that now. Create a new directory in your project folder and name it images. Use the images directory to store your project's images. The image I'd like to use for this tutorial is logo1.png and I've placed it in the images directory we created a moment ago.
As you can see in the following code snippet, displaying an image is almost as easy as displaying text. I dare to say it's even easier as you don't need to specify a font.
local photo1 = display.newImage("images/logo1.png", 0, 0);
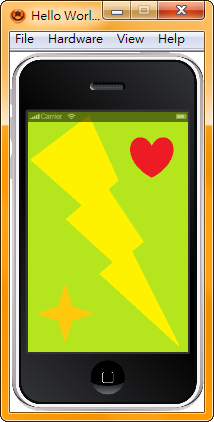
8. Status Bar
If you look closely at the previous screenshot, you'll notice that there's a status bar at the top of the screen displaying the carrier, battery life, etc. Have you ever noticed that sometimes, when you open an application, games in particular, the status bar automatically disappears? Hiding the status bar is as simple as adding one line of code to main.lua. It's a simple as that. Update your project and take a look at the result in the Corona Simulator.
display.setStatusBar(display.HiddenStatusBar);
It is useful to know that the status bar can have different styles. The names of the styles speak for themselves. For many applications, especially games, using HiddenStatusBar
is most suitable.
display.setStatusBar(display.DefaultStatusBar);
display.setStatusBar(display.DarkStatusBar);
display.setStatusBar(display.TranslucentStatusBar);
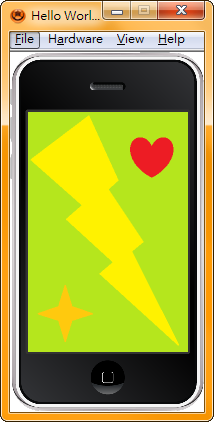
9. Rectangles, Borders, and Colors
Let's move on with shapes. The first shape we'll display is a rectangle. Let's see what it takes to display a rectangle on the screen.
local rect1 = display.newRect(10, 20, 150, 50);
-
local rect1
declares a variable for the rectangle. -
display.newRect
creates the rectangle shape. -
(10, 20, 150, 50)
define the x and y coordinates and the width and height, respectively.
Let's add some color to the rectangle.
rect1:setFillColor(51, 255, 0);
Hmmm. What does this mean?
-
rect1
is the variable we declared earlier. -
setFillColor
is the method we use to fill the rectangle with a color. -
(51, 255, 0)
specify the red (51
), green (255
), and blue (0
) value of the color we use.
Let's expand this example with a border or stroke as shown in the following code snippet.
rect1.strokeWidth = 8; rect1:setStrokeColor(80, 200, 130);
-
rect1.strokeWidth = 8
sets thestrokeWidth
property of the rectangle to8
. -
rect1.setStrokeColor(80, 200, 130)
sets thestrokeColor
property of the rectangle to the color specified by the values80
,200
, and130
as we saw earlier.
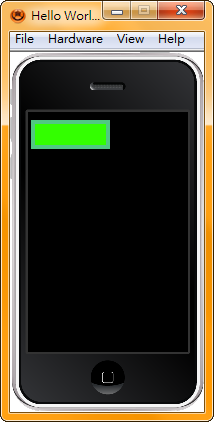
10. Comments
Comments may seem trivial and even obsolete, but they are important and even more so when you work in a team. Comments are very useful for documenting code and this applies both for you as your colleagues. It improves the readability of code for other people in your team. In Lua, comments are easy to use. Take a look at the following example.
--this is a comment
Comments have no effect on your application in terms of how it works. They are only there for the developer. The following code snippet will not print Hello World! to the terminal.
--print("Hello World!")
You can also write comments that span several lines, which is useful if you need to explain how a particularly complex piece of code works or if you want to write an introduction to an application or project.
--[[ This is comment spans several lines. ]]
Conclusion
In this tutorial, you've learned the basics of Lua and the Corona SDK. We installed and set up the Corona SDK, downloaded and used a text editor for editing Lua, wrote several applications, and ran them in the Corona Simulator. We also learned how to use variables to store pieces of data, display images on the screen, configure the status bar, and draw shapes to the screen. And, last but not least, we saw how to use comments and why you should use comments in your code. I hope that this tutorial was helpful to you. Stay tuned for more.
If you'd like to learn more about the Corona SDK, I recommend visiting the Corona Labs developer website. It is filled with resources and guides to get you started. You can also explore the example applications that are included in the Corona SDK that you downloaded and installed earlier.
The Lua programming language also has its own website. It contains everything you need to know about the language, including a getting started guide and a very, very detailed manual. Make sure to visit the Lua website if you decide to continue with Corona development.
Comments