In this tutorial, you'll learn how to create a plugin that uses Google's Static Maps API to display a map. We'll also look at the differences between enclosed and non-enclosed shortcodes.
The Google Static Maps API
Google provides two versions of it's Maps API for developers: dynamic, requiring JavaScript, and static, which simply returns an image and doesn't require JavaScript.
Dynamic maps allow you to zoom and pan the map as well as manipulate the map programatically in response to user-generated events. All of that is great, but if you simply need to drop a basic map image onto your page, you're much better off using the static map API.
Advantages Of The Static Maps API
- No need to have JavaScript enabled
- Reduction of requests to the server
- No need to write any JavaScript
- The static maps response is very fast
All You Need Is A URL
The base url for a static map is: http://maps.googleapis.com/maps/api/staticmap?
plus some parameters.
Here's a live url for Chicago, Illinois using the required parameters: center, size, zoom and sensor:
This is what you should see in your browser:
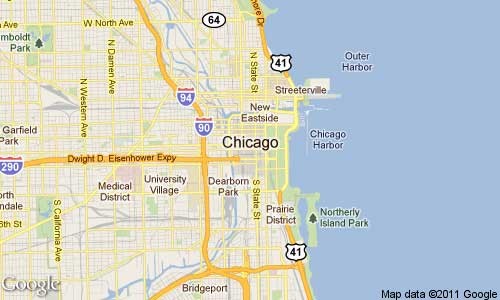
Clean and quick!
Our plugin will take arguments from a shortcode, add them to the base url, and return the full url to our posts and pages.
We'll make use of the following parameters:
- center (required) - the location of the map center as either text or as a latitude, longitude pair, example: 'chicago, illinois' or '41.88,-87.63'
- size (required) - the size of the map image in pixels, width x height, example: '400x350'
- zoom (required) - the zoom (magnification) level, 0 (the whole world) to 21 (most detailed)
- sensor (required) - true or false, see sensor parameter explanation
- maptype (optional) - the type of map: roadmap, satellite, terrain, hybrid, see maptypes parameter explanation
- scale (optional) - scaling for desktop or mobile usage , see scale parameter explanation
- format (optional) - required image format, png, jpg, gif and others, see format parameter explanation
- markers (optional) - we'll use a simple marker to show the center of our map
Let's do it.
Step 1 Set-Up the Plugin
The WordPress plugin folder is located in your WordPress installation folder at wp-content/plugins
Create a folder inside the plugins folder. Let's call it google-static-map
Now, create the plugin file itself. Let's call it google_static_map.php
The path to your plugin file should now be: wp-content/plugins/google-static-map/google_static_map.php
Every Wordpress plugin needs some header information so WordPress can identify it and make it available on your dashboard plugin page. Place this code at the top of your plugin file and save it.
<?php /* Plugin Name: Google Static Map Plugin URI: http://wp.rosselliot.co.nz/google-static-maps/ Description: Displays a map using the Google Static Maps API. Version: 1.0 License: GPLv2 Author: Ross Elliot Author URI: http://wp.rosselliot.co.nz */
You can edit this info as per your own requirements.
Now, go to your WordPress dashboard and select the Plugins menu. You'll see the plugin listed like this:

Don't activate it just yet.
Step 2 Shortcode Set-Up
Wordpress shortcodes allow you to place plugin output into your posts and pages.
The Shortcode
The WordPress shortcode will be used in posts and pages like this: [gsm_google_static_map center = 'chicago,illinois' zoom = '10' size = '400x350']
Because we're using a shortcode, we need to tell WordPress about it. To do that we use the WordPress function add_shortcode
.
Place the following code in your plugin file:
add_shortcode('gsm_google_static_map', 'gsm_google_static_map_shortcode');
The first parameter gsm_google_static_map
defines the name of the shortcode we'll use in our posts and pages. The second parameter gsm_google_static_map_shortcode
is the name of the function that is called by the shortcode.
The shortcode and plugin functions are prefixed with gsm
(google static map) to avoid any possible name collisions with any other functions that WordPress may be using. The chances are slim that WordPress or any plugin will have a function called google_static_map
but it pays to play it safe.
Step 3 The Shortcode Function
Here's the function called by gsm_google_static_map
. Place the following code in your plugin file:
function gsm_google_static_map_shortcode($atts){ $args = shortcode_atts(array( 'center' => '41.88,-87.63', 'zoom' => '14', 'size' => '400x400', 'scale' => '1', 'sensor' => 'false', 'maptype' => 'roadmap', 'format' => 'png', 'markers' => $atts['center'] ), $atts ); $map_url = '<img src="http://maps.googleapis.com/maps/api/staticmap?'; foreach($args as $arg => $value){ $map_url .= $arg . '=' . urlencode($value) . '&'; } $map_url .= '"/>'; return $map_url; }
Getting Good Data
Our shortcode function gsm_google_static_map_shortcode
accepts shortcode attributes (parameters) contained in the $atts
array. These attributes should be a rock-solid set of map parameters. But what if they're not? The shortcode API provides us with a way to provide defaults for these expected attributes, the shortcode_atts
function.
Shortcode_atts takes two arguments. The first is an array of name => value pairs. Name is the expected shortcode attribute and value is its default value. If name is not present in $atts, it is created with the default value. This allows us to make sure that our function has the expected attributes with defaults.
What we're really saying here is: compare the $atts
with the listed name => value pairs and if any of them don't exist in $atts
then create them and use the default value.
$args = shortcode_atts(array( 'center' => '41.88,-87.63', 'zoom' => '14', 'size' => '400x400', 'scale' => '1', 'sensor' => 'false', 'maptype' => 'roadmap', 'format' => 'png', 'markers' => $atts['center'] ), $atts );
Note that for the markers parameter we've assigned the center value that has been passed from the shortcode.
Make A Nice URL
Now that shortcode_atts
has made sure we have a good set of parameters, all we have to do is create a good static maps url to return to our post or page.
We use foreach
to loop over the name => value pairs in $args
concatenating them with the proper elements to make a properly formed url.
$map_url = '<img src="http://maps.googleapis.com/maps/api/staticmap?'; foreach($args as $arg => $value){ $map_url .= $arg . '=' . urlencode($value) . '&'; } $map_url .= '"/>';
The trick with this is to make sure that the supplied $args
element names (center, zoom, etc.) are exactly the same as the expected parameter names in the Google Maps request.
We use urlencode
on the values to make sure that any non-standard characters are converted safely. For instance, spaces are converted to the + character.
Step 4 Test
Activate the plugin in your Plugin page.
Add this shortcode to a post or page:
[gsm_google_static_map center = 'sydney,australia' zoom = '10' size = '600x350']
You should see this map:
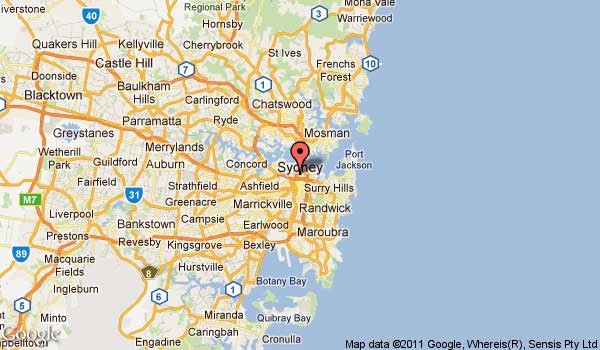
Play around with the shortcode attributes to see different maps. Perhaps this:
[gsm_google_static_map center = 'auckland,nz' zoom = '6' size = '600x350' maptype = 'terrain']
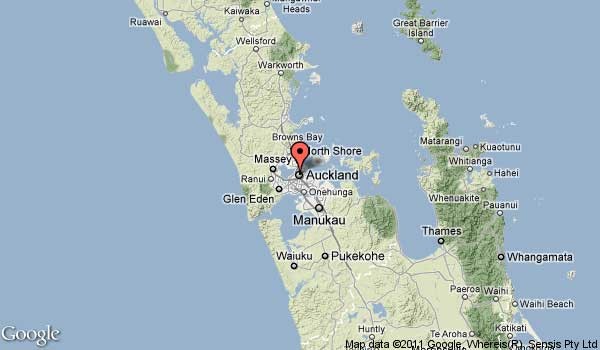
Step 5 A Better Plugin
Added Value: Enclosing Shortcodes
The shortcode form that we've used above is known as a self-closing shortcode. This means that the shortcode name and its attributes are contained between a pair of square brackets.
[gsm_google_static_map center = 'chicago,illinois' zoom = '10' size = '400x350']
But there's a variation on this form known as enclosing shortcodes.
[gsm_google_static_map center = 'chicago,illinois' zoom = '10']Map of Chicago, Illinois[/gsm_google_static_map]
The text "Map of Chicago, Illinois" is enclosed by the opening and closing tags of the shortcode. This is called the "content", and we can use it to our advantage.
Better SEO And A Fallback
The shortcode API lets us grab the content as a second parameter to the shortcode function, in our case the gsm_google_static_map_shortcode
function.
Let's update/replace that function with this:
function gsm_google_static_map_shortcode($atts, $content = NULL){ $args = shortcode_atts(array( 'center' => '41.88,-87.63', 'zoom' => '14', 'size' => '400x400', 'scale' => '1', 'sensor' => 'false', 'maptype' => 'roadmap', 'format' => 'png', 'markers' => $atts['center'] ), $atts ); $map_url = '<img title="' . $content . '" alt="' . $content . '" src="http://maps.googleapis.com/maps/api/staticmap?'; foreach($args as $arg => $value){ $map_url .= $arg . '=' . urlencode($value) . '&'; } $map_url .= '"/>'; return $map_url; }
Now we have a $content
argument that we can use. But for what? Well how about a value for our IMG tag attributes, ALT and TITLE?
The code above makes no difference to the display of the map itself, but if you hover over the map, you'll see a tooltip that displays a helpful piece of information, "Map of Chicago, Illinois". And if you view the source of the page you'll see that the ALT and TITLE attributes have the same value. This is helpful for SEO as search engine robots can't interpret images but they can, and do, read ALT and TITLE tags. Further, if images are disabled or not functioning in a user's browser, then they will at least still see a description of your map in their browser.
You could even use it to display a heading above the map by checking for $content not NULL and adding it to a heading tag:
if($content != NULL) $map_url = '<h3>' . $content . '</h3>'; $map_url .= '<img title="' . $content . '" alt="' . $content . '" src="http://maps.googleapis.com/maps/api/staticmap?'; foreach($args as $arg => $value){ $map_url .= $arg . '=' . urlencode($value) . '&'; } $map_url .= '"/>';
Shortcode for Havana, Cuba with heading: [gsm_google_static_map center="havana,cuba" zoom="11" scale="1" size="500x250"]Havana, Cuba[/gsm_google_static_map]
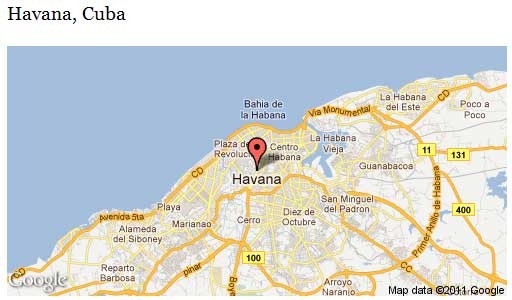
Note: the Wordpress shortcode API is very forgiving. You can use the self-closing or enclosing forms of a shortcode and the API will correct for variations. See the documentation.
Using Shortcodes In Template Files
Shortcodes are designed for use in posts and pages, but they can be used in your template files if wrapped in the do_shortcode
function.
Open the footer.php
file in your site's theme folder. Add the following just after the <div id="footer">
tag:
echo do_shortcode('[gsm_google_static_map center = 'chicago,illinois' zoom = '8' size = '300x250']');
Refresh your page and you should see a map in the footer.
Static Maps Usage Limits
From the Static Maps API Usage Limits section:
"Use of the Google Static Maps API is subject to a query limit of 1000 unique (different) image requests per viewer per day. Since this restriction is a quota per viewer, most developers should not need to worry about exceeding their quota."
Also, map sizes are restricted to 640x640, but by using the scale parameter set to 2, you can effectively double the map size.
Comments