Welcome back to the second of a two part tutorial series on building native, social applications for mobile devices. In part 1, we created a basic application that utilized the Facebook authentication library to allow a user to sign in with their facebook credentials. The result was access to the user's Facebook data through a session token that we received when the user signed in.
Once we have this token, the Facebook Graph API can be used to create a variety of applications. When an end user signs in, the application is responsible for passing along the necessary permissions that are required for it to run. The user can either permit or not permit the application from accessing his/her data from within Facebook. This attempts to at least notify the end user what an application might do with their data in hopes of creating more transparency.
For part 2 of this tutorial, we are going to modify our original mobile Facebook application (which admittedly did not do much), to create a neat collage of a user's friends' profile pictures. The FB Graph API is primarily based on JSON objects so we will make use of a Lua JSON wrapper library that can be found on the Ansca Mobile code exchange.
To start, we need to add the following library inclusion to the top of our main.lua file:
require("Json")
Again, this library can be found in the code exchange on anscamobile.com. Once included, we have access to the library methods through a variable entitled Json. More on this later.
Before in our listener method, we were listening for events that were being fired from the Facebook login box that was being displayed upon clicking the button in the center screen. Upon a successful login, we accessed a personal data object through the API to prove that the connection was successful. This code can be seen here:
if ( "login" == event.phase ) then facebook.request( "me" ) end
Since our app is primarily concerned with accessing our friend's profile pictures, we are going to modify the code to pull the "Friends" data object from the API in the same manner. We will replace the above code snippet with the following:
if ( "login" == event.phase ) then facebook.request( "me/friends", "GET" ) end
The documentation for this "Friends" object can be viewed here in the FB Graph API user doc (See Connections -> friends). You'll notice that we are passing a secondary parameter of "GET" to the facebook.request method. Since the Graph API is more or less "REST" compliant, the type of request we are making matters greatly. "GET" is by nature a read request so we are "getting" data about the logged-in user's friends. If we were doing a write based call to the API (e.g. Posting to a Wall), we might pass along "PUT" to specify that.
Once the "facebook.request" method is called, the listener will capture new events that will be fired upon this API call's return. In part 1 of the tutorial, we watched for an event type of "request" to determine this was a result of our post login API call. The code was as follows:
elseif ( "request" == event.type ) then local response = event.response print( response ) end
This basically got the response back from the Facebook API and printed it out. This is the part of the code that we are going to replace in order to create our collage of friend profile photos. The above code will be replaced with the following:
elseif ( "request" == event.type ) then local response = Json.Decode( event.response ) local data = response.data local function showImage( event ) event.target.alpha = 0 event.target.xScale = 0.25 event.target.yScale = 0.25 transition.to( event.target, { alpha = 1.0 , xScale = 1, yScale = 1} ) end for i=1,#data do display.loadRemoteImage("http://graph.facebook.com/".. data[i].id .."/picture", "GET", showImage, "friend"..i..".png", system.TemporaryDirectory, math.random(0,display.contentWidth), math.random(0,display.contentHeight) ) end end
Let's break this down so we can understand each part:
local response = Json.Decode( event.response ) local data = response.data
In this part of the code we are simply decoding the JSON response that we are receiving from the Facebook API. The response is stored as an attribute of the event object (event.response) that is being passed to the listener. When the JSON object is decoded, it is translated into a Lua object which we are storing in a variable called "response." Since the key of root element of this JSON object is "data", once it is decoded, we are able to drill down into the object by calling "response.data."
local function showImage( event ) event.target.alpha = 0 event.target.xScale = 0.25 event.target.yScale = 0.25 transition.to( event.target, { alpha = 1.0 , xScale = 1, yScale = 1} ) end
This method is a callback that will be fired upon the loading of a remote image from a URL. The next section will describe how this occurs. Once this method is fired, it expects an event object which will contain the display object of the a profile image inside the attribute "target" (event.target). As you can see we are setting the base attributes of "alpha" to 0 or invisible as well the scale to 1/4 the original size of the image.
The transition.to method accepts 2 parameters: first being the object that it will act upon and the second a table object of the parameters and values it is transitioning to. The effect of this transition will be to gradually display the image and modify it to go from 1/4 its size and invisible to it's full dimensions (scale=1) and visible (alpha = 1). This makes for a poor man's kind of math based, animation effect on each image as it is displayed. This will make more sense when the application is run.
for i=1,#data do display.loadRemoteImage("http://graph.facebook.com/".. data[i].id .."/picture", "GET", showImage, "friend"..i..".png", system.TemporaryDirectory, math.random(0,display.contentWidth), math.random(0,display.contentHeight) ) end
Since the "Friends" object we are receiving from the Faceobok API is an array, we need to loop through it to get the ID's of each user. This ID is used to construct a URL that will serve up a small square version of a user's profile picture. Using the display.loadRemoteImage method, we can call an URL and it will handle downloading the image data and storing it locally before displaying it on the screen.
The first parameter we pass:
"http://graph.facebook.com/".. data[i].id .."/picture"
is the constructed URL which includes the user's unique Facebook ID (data[i].id).
"GET", showImage, "friend"..i..".png", system.TemporaryDirectory,
The following 4 parameters are:
- the type of request, "GET" in this case
- our showImage callback method that will be fired when the image is downloaded.
- a unique name for the image file we are downloading
- a temporary file space to store the downloaded images accessed through the "system.TemporaryDirectory" method
Finally, the last 2 parameters we pass are the X and Y coordinates that we want to display the image at:
math.random(0,display.contentWidth), math.random(0,display.contentHeight)
Since we are creating a collage, we can place the images randomly all over the screen. The "math.random" call takes a starting value and an ending value and returns a random number between the 2. In this case, we need random numbers that are anywhere between 0 and the width/height of the screen. Any more or less and the image would not be visible.
And there we have it! Check out the result below:
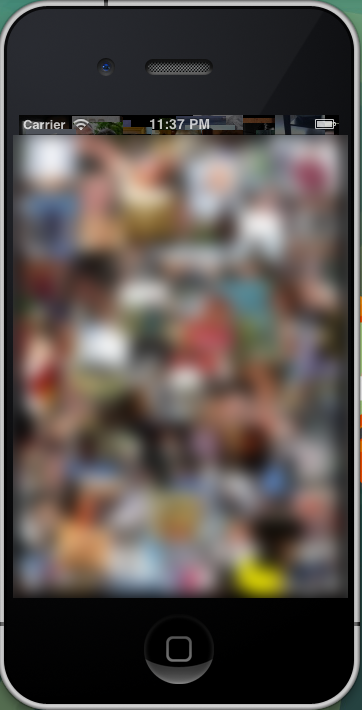
The faces have been blurred to protect the innocent. :)
As a final note, the Facebook API library for Corona will not run properly in the Corona Simulator. The easiest way to test the application we built in the tutorial will be to run it in the actual iOS simulator that comes with Xcode.
Comments