Stop using static menus! Most players immediately base their initial impression of a Flash game on the menu that they see when they load it. Stand out from the crowd with an active menu!
This tutorial was first posted in December 2011, but has since been updated with extra steps that explain how to make the code more flexible!
Final Result Preview
Introduction: Static vs Active
The word "static" essentially means lacking in change. The majority of menus we see throughout web games are lacking in change, you simply press Play and the game starts. Menus like that are overused and show little creativity or innovation.
To make a menu "active" we must continuously cause change. So in this tutorial that is exactly what we are going to accomplish: a menu that continuously changes.
Step 1: Setting Up
The first thing we are going to need to create is a new Flash File (ActionScript 3.0). Set its width to 650px, its height to 350px, and the frames per second to 30. The background color can be left as white.
Now save the file; you can name it whatever you please, but I named mine menuSlides.fla
.
In the next section we will create the nine MovieClips used in the menu. For reference, here is a list of all the colors used throughout the tutorial:
- White - #FFFFFF
- Gold - #E8A317
- Light Gold - #FBB917
- Blue - #1569C7
- Light Blue - #1389FF
- Green - #347235
- Light Green - #3E8F1B
- Red - #990000
- Light Red - #C10202
- Matte Grey - #222222
Step 2: Creating the Slide MovieClips
To start with we will create the slides used in the transitions, but before we begin let's turn on some very useful Flash features.
Right-Click the stage and select Grid > Show Grid. By default it will create a 10px by 10px grid across the stage. Next, right-click the stage again and this time select Snapping > Snap to Grid.
Now we can begin drawing! Select the Rectangle Tool and draw a Light Gold rectangle, 650px wide and 350px tall (you can Alt-click on the stage to make this easier). Now change the color to Gold and draw groups of three squares, each 20x20px, to form the shape of an L in each corner,:
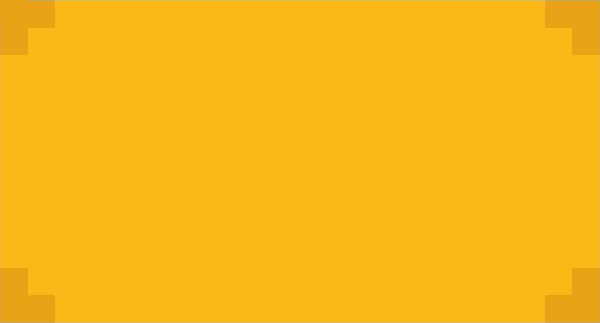
Select the whole stage, right-click and choose Convert to Symbol. Name the MovieClip goldSlide
and make sure that the type is MovieClip and the registration is top-left.
To save time and make things a whole lot easier, right-click the goldSlide
MovieClip in the Library and select Duplicate Symbol three times to make three more copies. Change the colors in the new MovieClips to blue, green and red, then rename the MovieClips to blueSlide
, greenSlide
and redSlide
.
Before we continue we should add some text to each slide. On goldSlide write PLAY, on blueSlide write INSTRUCTIONS, on greenSlide write OPTIONS and on redSlide write CREDITS.
Now that we have the text in place we can break it apart by right-clicking on it and selecting Break Apart twice; this will break the text down to a fill which will transition more smoothly. Plus as a bonus there will be no need to embed a font if you are just using it for the menu!
The Buttons
Now that we have drawn the 4 slides we can focus on the sideButton
MovieClip that is used to move the slides either left or right.
First, draw a rectangle 30x60px with only a stroke (no fill), then draw diagonal lines 45 degrees from the top-right and bottom-right corners until they snap together in the middle of the opposite side. Now apply a Matte Grey fill to the triangle:

Next, delete the lines, then right-click the triangle and select Convert to Symbol. Name it sideButton
, set the type to Button and make sure the registration is in the top-left corner.
Now insert 3 keyframes in the timeline by right-clicking the timeline and selecting Insert Keyframe. On the Up frame, select the fill of the triangle, go to the Windows tab and select Color. Change the Alpha to 50%. On the Over Frame repeat the same process, but this time set the alpha to 75%.
Now we can begin on the four numbered circle buttons, for jumping directly to a particular slide.
To start draw a white 30px circle with no stroke. Convert it to a symbol, name it circleOne
, and set its type to Button and its registration point to the center. Insert three keyframes like we did before and then go to the Up frame.
Draw a black 25px circle with no stroke and center it to the middle through the coordinates or by using the Align menu. Next deselect the black circle, then reselect it and delete it. You should now have a white ring remaining. Now grab the text tool and put a white "1" in the center of the ring. Then break this number apart until it is a fill.

Go to the Over frame and draw a black "1". Center it and break it apart until it becomes a fill. Now deselect and reselect the fill, then delete it. Select everything on the frame and copy it, then go to the Down frame, select everything on it and hit delete. Paste in what we have copied.

Now create three more circle MovieClips, following the same process, for the numbers 2, 3 and 4.
Step 3: Positioning the MovieClips
Okay, we're almost half-way done! First drag all of the slides onto the stage and position them with the following coordinates:
- goldSlide: (0, 0)
- blueSlide: (650, 0)
- greenSlide: (1300, 0)
- redSlide: (1950, 0)
Now drag and drop two copies of the sideButton. The first copy should be positioned at (10,145); before we can position the second copy we must first flip it!
Select the second copy and press Ctrl-T. Change the left-right to -100% and leave the up-down at 100%. Now move the second copy to (640,145).
Finally drag and drop the four circle MovieClips and position them as so:
- circleOne: (30, 320)
- circleTwo: (70, 320)
- circleThree: (110, 320)
- circleFour: (150, 320)
Your stage should now look like this:
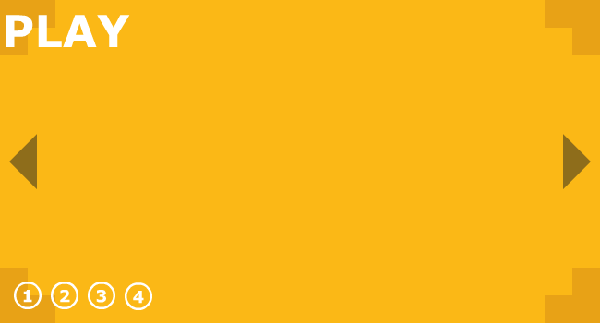
The blue, green and red slides are hidden just off to the right of the stage. Now select everything on the stage and convert to a symbol. Name it menuSlidesMC
, set the type to MovieClip and the registration to the top-left corner, and export it for ActionScript as MenuSlidesMC
.
Before we finish we must give each of the MovieClips inside menuSlidesMC
an instance name. Select each slide in the order they appear from the left and name them slide1
, slide2
, slide3
and slide4
respectively. Name the circle buttons one
, two
, three
and four
, and finally name the side buttons left
and right
.
Step 4: Setting Up the Classes
Now that all of our MovieClips have been created we can start setting up the two classes we are going to use.
First go to your Flash file's Properties and set its class to menuSlides
; then, create a new ActionScript 3.0 file and save it as menuSlides.as
.
Now copy the following code into it; I will explain it after:
package{ import flash.display.MovieClip; import flash.events.Event; import flash.events.MouseEvent; public class menuSlides extends MovieClip{ public var menuSlidesMC:MenuSlidesMC = new MenuSlidesMC(); public function menuSlides(){ addChild(menuSlidesMC); } } }
Pretty basic - it's a document class, into which we imported the MovieClips and Events we will use. Then we created an instance of MenuSlidesMC
, and added it to the stage.
Now create a new ActionScript 3.0 file for the menuSlidesMC
instance. Save it as MenuSlidesMC.as
and copy the following code into it:
package{ import flash.display.MovieClip; import flash.events.Event; import flash.events.MouseEvent; public class MenuSlidesMC extends MovieClip{ public var speed:Number = new Number(); public var activeSlide:Number = new Number(); public function MenuSlidesMC(){ speed = 10; activeSlide = 1; addEventListener(MouseEvent.CLICK, slidesClick); addEventListener(Event.ENTER_FRAME, slidesMove); } } }
Just like last time, we imported what we are going to need, but we created two number variables. The first variable, speed
, is actually how many pixels the slides are moved by each frame. (Note: this number has to evenly divide into your stage's width to give a smooth transition). The second variable, activeSlide
, tells us which slide is currently set to be on screen.
We also added two event listeners for functions we are going to create; one of them is called on a mouse click, and the other is called at the beginning of every frame.
Step 5: Creating the Event Handler Functions
To begin we will get the mouse click function out of the way. Start by creating a public function named slidesClick()
:
public function slidesClick(event:MouseEvent):void { }
Next we will create some if-statements regarding the event.target.name
. Basically, this property stores the name of the object that was targeted by the mouse click. We can use this to check which button is pressed:
if(event.target.name == "left"){ if(activeSlide>1){ activeSlide-=1; } }else if(event.target.name == "right"){ if(activeSlide<4){ activeSlide+=1; } } if(event.target.name == "one"){ activeSlide=1; }else if(event.target.name == "two"){ activeSlide=2; }if(event.target.name == "three"){ activeSlide=3; }else if(event.target.name == "four"){ activeSlide=4; }
The code above goes in the slidesClick()
function. The first set of if-statements are for the left and right side buttons; they increase or decrease the value of activeSlide
, but never allow the value to become less than 1 or greater than 4 (since we only have four slides). The second set of if-statements are for the circle buttons; instead of just incrementing or decrementing the value of activeSlide
they set it to the selected value.
Now let's begin with the ENTER_FRAME
handler function:
public function slidesMove(event:Event):void { }
Add the slidesMove()
function below your slidesClick()
function and we'll start adding some code to it. First, we'll use a switch
to check which slide should be on the screen, based on the value of activeSlide
:
switch (activeSlide){ case 1: break; case 2: break; case 3: break; case 4: break; }
Now in each case we will create an if/else block that will check that slide's current x-position, and move all of the slides either left, right, or not at all, depending on where the desired slide currently sits.
The first case looks like this:
if(slide1.x<0){ slide1.x+=speed; slide2.x+=speed; slide3.x+=speed; slide4.x+=speed; }else if(slide1.x>0){ slide1.x-=speed; slide2.x-=speed; slide3.x-=speed; slide4.x-=speed; }
Now all we have to do is repeat the same process for the other cases! After you are done your swtich should look like this:
switch (activeSlide){ case 1: if(slide1.x<0){ slide1.x+=speed; slide2.x+=speed; slide3.x+=speed; slide4.x+=speed; }else if(slide1.x>0){ slide1.x-=speed; slide2.x-=speed; slide3.x-=speed; slide4.x-=speed; } break; case 2: if(slide2.x<0){ slide1.x+=speed; slide2.x+=speed; slide3.x+=speed; slide4.x+=speed; }else if(slide2.x>0){ slide1.x-=speed; slide2.x-=speed; slide3.x-=speed; slide4.x-=speed; } break; case 3: if(slide3.x<0){ slide1.x+=speed; slide2.x+=speed; slide3.x+=speed; slide4.x+=speed; }else if(slide3.x>0){ slide1.x-=speed; slide2.x-=speed; slide3.x-=speed; slide4.x-=speed; } break; case 4: if(slide4.x<0){ slide1.x+=speed; slide2.x+=speed; slide3.x+=speed; slide4.x+=speed; }else if(slide4.x>0){ slide1.x-=speed; slide2.x-=speed; slide3.x-=speed; slide4.x-=speed; } break; }
And that’s it! We are all finished with the code and the menu should be working great right now.
...But wait, what if we want to add more slides or take some away?
Step 6: Adding Slides to an Array
At the moment our code isn't very flexible due to all of those hard-coded if
statements. So let's do something bold: delete all of the code in the slidesMove() function because we will no longer be needing it, and also delete the if-statements for the circle buttons as we are going to optimize those as well.
Now declare a new variable (underneath speed
and activeSlides
):
public var slidesArray:Array = new Array();
The first variable, slidesArray
, will be an array that contains all of our slides, which will allow us to access them by referencing an item in the array (so we can use slidesArray[2]
instead of slide3
).
One thing to note is that the first item in an array is given an index of 0
, so we will have to make some changes to our instance names.
Select each slide in the order they appear from the left and name them slide0
, slide1
, slide2
and slide3
, respectively. And to help us cut down on the number of lines of code we use, select each circle button in the order they appear from the left and name them circle0
, circle1
, circle2
and circle3
, respectively.
If you are going to add more slides and buttons, now is the time to do so. Just position the extra slides at the end of the row of slides, then give them instance names following the same order. Then do the same for the circle buttons.
Now that we have the instance names correct we must add the slides to the array. Do so by adding the following code to your constructor:
slidesArray = [slide0, slide1, slide2, slide3, slide4, slide5];
Now the slides are in the array and can be accessed by their index in the array. For example, slidesArray[0]
is equivalent to slide0
because that is the first item in the list.
Next, inside the "right" else-if statement, change the condition to:
if(activeSlide < slidesArray.length-1){
The value of slidesArray.length
is equal to the number of elements in the array, so this new condition will now allow us to press the button and shift the slides over as long as the active slide is not the final slide.
Step 7: Handling Circle Button Presses
Now, when a circle button is clicked, we need to figure out which one it is (and which slide it refers to).
Create an array to hold all the circle buttons. First, define it, beneath the slide array:
public var slidesArray:Array = new Array(); public var circlesArray:Array = new Array();
Then, add the circle buttons to the array in the constructor:
circlesArray = [circle0, circle1, circle2, circle3, circle4, circle5];
Now, move to the slidesClick()
function, underneath the whole if-else block. We're going to check whether the button clicked is in the circle buttons array:
if (circlesArray.indexOf(event.target) != -1) { }
The array's indexOf() function checks to see whether an object is in the array; if it's not, it returns -1
. So, we're checking to see whether it's not equal to -1
, which will check to see whether it is in the array.
Assuming it is, then the indexOf()
function will return the index of the button within the circle buttons array - so, if circle3
was clicked, circlesArray.indexOf(event.target)
will be equal to 3
. This means we can just set activeSlide
to 3, and we're done!
if (circlesArray.indexOf(event.target) != -1) { activeSlide = circlesArray.indexOf(event.target); }
Step 8: Moving the Slides
The only thing left to do is move all of the slides. Begin by adding the same loop as we had before, in the slidesMove()
function:
for(var i:int = 0; i < slidesArray.length; i++){ }
An if-else statement needs to be added now; this will use the variable activeSlide
to select a slide out of the array and check where its x-position is, just like before.
if(slidesArray[activeSlide].x<0){ }else if(slidesArray[activeSlide].x>0){ }
Since activeSlide
is a number, slidesArray[activeSlide]
refers to one specific slide, so slidesArray[activeSlide].x
is equal to that slide's x-position.
In the first case we will add a for
loop to move all of the movie clips to the right, and in the second case we will add a for
loop to move all of the movie clips to the left.
Right:
for(var j:int = 0; j < slidesArray.length; j++){ slidesArray[j].x+=speed; }
Left:
for(var k:int = 0; k < slidesArray.length; k++){ slidesArray[k].x-=speed; }
If you test this now, you will notice that our optimised code has lead to a much zippier interface!
Step 9: Taking It Further
If you wanted to take this even further, you could use a for
loop to position the slides and the circles, rather than needing to drag and drop them in the Flash IDE. For example, to position the slides, we'd first position slide0
in the constructor:
slidesArray = [slide0, slide1, slide2, slide3, slide4, slide5]; slidesArray[0].x = 0; slidesArray[0].y = 0;
Then, we'd loop through all the other slides, starting at slide1
:
slidesArray = [slide0, slide1, slide2, slide3, slide4, slide5]; slidesArray[0].x = 0; slidesArray[0].y = 0; for (var i:int = 1; i < slidesArray.length; i++) { }
We can give all the slides an y-position of 0:
slidesArray = [slide0, slide1, slide2, slide3, slide4, slide5]; slidesArray[0].x = 0; slidesArray[0].y = 0; for (var i:int = 1; i < slidesArray.length; i++) { slidesArray[i].y = 0; }
...and then we can set each slide's x-position to be 620px to the right of the slide before it:
slidesArray = [slide0, slide1, slide2, slide3, slide4, slide5]; slidesArray[0].x = 0; slidesArray[0].y = 0; for (var i:int = 1; i < slidesArray.length; i++) { slidesArray[i].x = slidesArray[i-1].x + 620; slidesArray[i].y = 0; }
If your slides aren't 620px wide, you can even detect their width automatically!
slidesArray = [slide0, slide1, slide2, slide3, slide4, slide5]; slidesArray[0].x = 0; slidesArray[0].y = 0; for (var i:int = 1; i < slidesArray.length; i++) { slidesArray[i].x = slidesArray[i-1].x + slidesArray[i-1].width; slidesArray[i].y = 0; }
You can do the same thing with the circle buttons, but I'll leave that up to you to experiment with.
The great thing about this is, you can add as many slides as you want to the menu; all you have to do is add them to the array, and they'll be dealt with by this code.
(You can remove slides from the array, too, but they won't be affected by any of the code, so you'll probably need to reposition them in the Flash IDE.)
Conclusion
Thank you for taking the time to read through the tutorial, I hope it was helpful and that you learned a little something about active game menus.
Comments