When a user first opens up a Flash game, their initial impression is based upon the menu that they see. Unfortunately, many games' menus are dull, plain and static. This is terrible news! A lot of players will just close the game before even playing it, and move on to another. Don't let this happen to you!
Final Result Preview
Take a look at the menu we'll be building:
Obviously, in a full game, the different menu screens would contain different buttons, text, and submenu items!
Introduction: What is a Dynamic Menu?
Before we learn what a dynamic menu is we must first learn what a static menu is.
A static menu can be described by the definitions of the two words it is made up of: "static", meaning lacking in change, and "menu", meaning a list of available options or choices. Therefore a static menu has an interface of options or choices that is lacking in change.
Conversely, the word "dynamic" means to continuously cause change within a system or process. So in the simplest terms a dynamic menu continuously updates the interface of given options or choices.
Step 1: Setting Up
The first thing that we are going to need to create is a new Flash File(ActionScript 3.0), set its width to 650px and height to 350px, and set the frames per second to 60. Instead of changing the background color leave it as white; later on in the tutorial we will create a background.
Now that we have the basic Flash File set up we can save it; you can name yours whatever you please. I just named mine MenuAccordion.fla.
In the next section we will create the eight MovieClips required for the menu; here is a list of all the colors and their hexidecimal codes that will be used throughout the tutorial:
- White -
#FFFFFF
- Gold -
#E8A317
- Light Gold -
#FBB917
- Blue -
#1569C7
- Light Blue -
#1389FF
- Green -
#347235
- Light Green -
#3E8F1B
- Red -
#990000
- Light Red -
#C10202
- Matte Grey -
#222222
Step 2: Creating the MovieClips
To start off we need to create one main background color, four "bar" MovieClips (these are the ones that will be clicked on) and four background MovieClips (these will slide out after a bar is clicked).
First, right-click the stage and choose Grid, then select Show Grid - by default it will create a 10px by 10px square grid accross the stage. To make your life easier right-click again and select Snapping > Snap to Grid. Now we are ready to start drawing!
Select the Rectangle tool and draw a Matte Grey background over the whole stage. Name the layer Background and lock it. Next, create a new layer and name it Menu. Your timeline and stage should look something similar to this now:
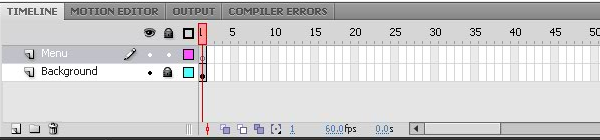
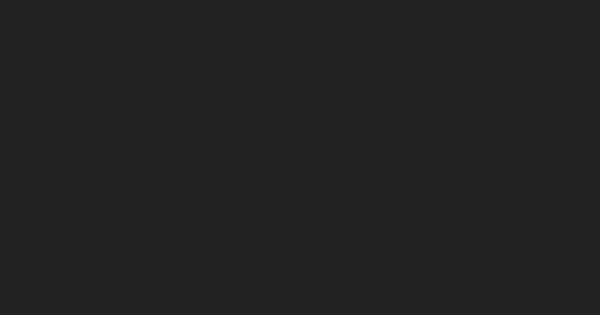
Next we will create one bar MovieClip; to make things easier we will just duplicate it to form four MovieClips and change the color of each.
Select the rectangle tool once again, and draw a 30px by 350px rectangle with no stroke. Right-click the fill and select Convert to Symbol.... Name it goldBar, make sure the type is MovieClip and the registration point is in the top-left corner. Double-click the MovieClip on the stage to edit it.
Right-click the first keyframe in the timeline and go to actions, type in a simple stop();
command to prevent the MovieClip from cycling through frames. Next, right-click the second frame and select Insert Keyframe. It is up to you what you would like to write in each fill; only thing to remember is that if your are writing on a 90 degree angle the text may appear invisible if you have the text set to dynamic. Personally I just broke apart the text until it became a fill, and I drew an arrow that was pointing opposite directions on each frame:
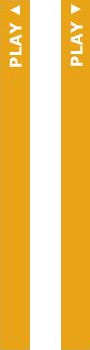
Now that we have one bar completed we can right-click it in the library and select Duplicate Symbol.... Make three copies of it and name them blueBar, greenBar and redBar, respectively. Then just edit their fill colors and change the text. Voila! We have four bar MovieClips.
Now we can begin on the background MovieClips which we will create and duplicate in the same way. Select the rectangle tool again and draw a rectangle of 560x350px. Convert it to a symbol, name it goldBackground, and make sure that the type is a MovieClip and the registration is in the top-left corner. Then just duplicate it three times and name the new MovieClips blueBackground, greenBackground and redBackground respectively.
Your rectangles should look very simple, like this:
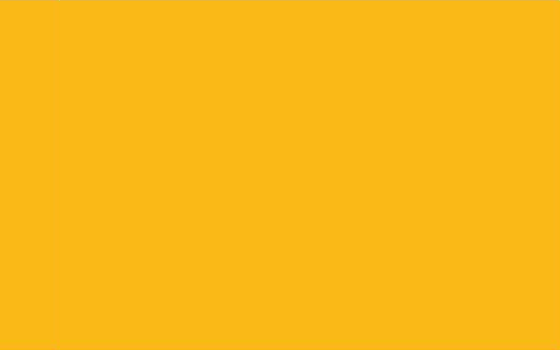
There is only one more MovieClip to create, I promise! Go to the Insert menu and select New Symbol; name it menuAccordionMC, set the type to MovieClip and put the registration point in the top-left corner (because our last MovieClips had this too). Next, check the box that says Export for ActionScript: the class name should be MenuAccordionMC with the capital at the beginning.
Step 3: Positioning the MovieClips
In the previous section we created all the MovieClips required, and now we need to position them!
Delete any MovieClips on the stage if they are on still there. Open your menuAccordionMC from the Library, and create seven more layers in its timeline. Now name them in the following order from top to bottom:
- goldBar
- blueBar
- greenBar
- redBar
- goldBackground
- blueBackground
- greenBackground
- redBackground
Now drag and drop each MovieClip from the library to its corresponding layer. The easiest way to position all of these will be to lock all of the layers besides the one with the MovieClip you are currently positioning.
Start with the goldBar and set its x and y coordinates to (0,0). Next the blueBar with the coordinates (30,0), then the greenBar with (60,0), and finally the redBar with (90,0).
Time for the backgrounds: the goldBackground's coordinates are (-530,0), the blueBackground's coordinates are (-500,0), the greenBackground's coordinates are (-470,0) and the redBackground's coordinates are (-440,0).
Your MovieClip should look like this now, and the (0,0) coordinate should be in the top-left corner of the goldBar MovieClip where the white plus is in the image:
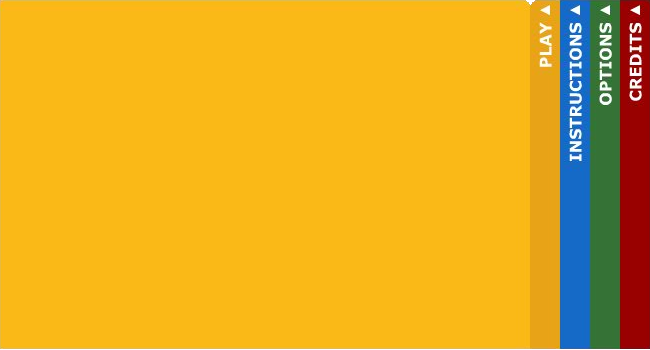
Step 4: Identifying the MovieClips
Before we can begin coding there is one more thing we have to do: give the eight inner MovieClips instance names.
Open up the the menuAccordionMC for the final time then select each MovieClip and make its instance name the same as its MovieClip name. So goldBar's instance name would be goldBar, greenBackground's instance name would be greenBackground, and so on. After this is done we can begin the coding, which is fairly easy to understand!
Step 5: Setting Up the Classes
Go to your Flash file's properties and name the document class MenuAccordion. Now create a new ActionScript 3.0 file and put the following code in it and save the file as MenuAccordion:
package { import flash.display.MovieClip; import flash.events.Event; public class MenuAccordion extends MovieClip { public var menuAccordionMC:MenuAccordionMC = new MenuAccordionMC(); public function MenuAccordion() { addChild(menuAccordionMC); } } }
Here, we first import what we will need (in this case just the MovieClip and Event classes). Next we add the variable for our menuAccordionMC, then in the constructor we attach the MovieClip to the stage at (0,0).
That is all the code we will need for the document class; now let's create a new ActionScript 3.0 file for the MovieClip and save it as MenuAccordionMC.as.
To set up the MenuAccordionMC class, input the following code - I will explain the variables and event listeners shortly:
package{ import flash.display.MovieClip; import flash.events.Event; import flash.events.MouseEvent; public class MenuAccordionMC extends MovieClip{ public var activeColor:String = new String(); public var speed:Number = new Number(); public function MenuAccordionMC(){ activeColor = "none"; speed = 10; addEventListener(MouseEvent.CLICK, accordionClick); addEventListener(Event.ENTER_FRAME, accordionMove); } } }
First thing to notice is that we have imported the MouseEvent: this is very important because without it our mouse click will have no effect. As for the two new variables: activeColor is just a simple string to tell us which color is currently in use, and speed is how many pixels the backgrounds shift every frame.
The first event listener is for a MouseEvent.CLICK and will call the function accordionClick when our menu is clicked anywhere. The second event listener adds the function accordionMove and will be called every frame.
Now we will create the two functions for the event listeners. Place the following code right after the closing curly brace for the constructor.
public function accordionClick(event:MouseEvent):void { } public function accordionMove(event:Event):void { }
The two classes are now set up, so it's time to start putting code in these functions!
Step 6: The accordionClick Function
This function is relatively easy and only has an if, else statement in it. Begin by writing the following in the function:
if(event.target.currentFrame==1){ }else{ }
Many people are unfamiliar with event.target, so I will briefly explain it. In a nutshell, it just directs the code to the target of the the event - so if the blueBar is clicked, event.target is equal to blueBar.
This means that the if statement checks to see whether the bar's currentFrame is equal to 1, and if so it executes that code; if not, it will execute the code in the second curly brackets. We can continue now and change the if, else statement to the following:
if(event.target.currentFrame==1){ event.target.gotoAndStop(2); activeColor = event.target.name; }else{ event.target.gotoAndStop(1); activeColor = "none"; }
The new lines are very basic: the target's currentFrame is changed to the second or first frame and the activeColor variable is set to the target's name or it is set to "none". That is all the code needed for the accordionClick function.
Step 7: The accordionMove Function
Alright, this is where all the magic happens! This is the endgame and its a long stretch to the finish, but if you follow the final instructions correctly you will have a working accordion menu!
First thing we need to add to the function is a switch case, which will eventually include all of the if statements required for this to run smoothly:
switch (activeColor){ case "none": break; case "goldBar": break; case "blueBar": break; case "greenBar": break; case "redBar": break; }
This switch case corresponds to the variable activeColor that is set during the accordionClick function. Now let's start with the easiest part of this case statement, case: none:
if(this.goldBackground.x>=-530){ this.goldBackground.x-=speed; } if(this.blueBackground.x>=-500){ this.blueBackground.x-=speed; } if(this.greenBackground.x>=-470){ this.greenBackground.x-=speed; } if(this.redBackground.x>=-440){ this.redBackground.x-=speed; } if(this.blueBar.x>30){ this.blueBar.x-=speed; } if(this.greenBar.x>60){ this.greenBar.x-=speed; } if(this.redBar.x>90){ this.redBar.x-=speed; }
(Editor's Note: You may argue that this code is not neat enough for practical use. See the comments of the other tutorial in this series for a discussion about this!)
All that the none case does is check whether any of the MovieClips' x-positions are greater than their original ones, if they are it moves them left until they no longer are greater than their original x positions.
The next case, goldBar, does something similar. It moves all the other bars that are on the right side of it to the right side of the stage and the backgrounds to the bars' previous x-positions. Then it just switches all the other bars' currentFrame properties to 1 (so that the arrows point in the opposite direction):
if(this.goldBackground.x<0){ //Moves The Bars & Backgrounds this.goldBackground.x+=speed; if(this.blueBar.x<560){ this.blueBar.x+=speed; } if(this.blueBackground.x<30){ this.blueBackground.x+=speed; } if(this.greenBar.x<590){ this.greenBar.x+=speed; } if(this.greenBackground.x<60){ this.greenBackground.x+=speed; } if(this.redBar.x<620){ this.redBar.x+=speed; } if(this.redBackground.x<90){ this.redBackground.x+=speed; } //Checks if any other bar's currentFrame is 2, if it is it switches it to 1 if(this.blueBar.currentFrame==2){ this.blueBar.gotoAndStop(1); }else if(this.greenBar.currentFrame==2){ this.greenBar.gotoAndStop(1); }else if(this.redBar.currentFrame==2){ this.redBar.gotoAndStop(1); } }
All right, we have two out of the five cases complete. The next three cases work the same as the goldBar - with one exception. They move any backgrounds that are open to the left of them back to their original position and shift themselves back to their original position.
Here is the blueBar case:
if(this.blueBackground.x<30){ this.blueBackground.x+=speed; if(this.greenBar.x<590){ this.greenBar.x+=speed; } if(this.greenBackground.x<60){ this.greenBackground.x+=speed; } if(this.redBar.x<620){ this.redBar.x+=speed; } if(this.redBackground.x<90){ this.redBackground.x+=speed; } if(this.goldBar.currentFrame==2){ this.goldBar.gotoAndStop(1); }else if(this.greenBar.currentFrame==2){ this.greenBar.gotoAndStop(1); }else if(this.redBar.currentFrame==2){ this.redBar.gotoAndStop(1); } } if(this.blueBar.x>30){ this.blueBar.x-=speed; if(this.goldBackground.x>-530){ this.goldBackground.x-=speed; } if(this.greenBar.x<590){ this.greenBar.x+=speed; } if(this.greenBackground.x<60){ this.greenBackground.x+=speed; } if(this.redBar.x<620){ this.redBar.x+=speed; } if(this.redBackground.x<90){ this.redBackground.x+=speed; } if(this.goldBar.currentFrame==2){ this.goldBar.gotoAndStop(1); }else if(this.greenBar.currentFrame==2){ this.greenBar.gotoAndStop(1); }else if(this.redBar.currentFrame==2){ this.redBar.gotoAndStop(1); } }
The only thing new about this case is the second main if statement; this is for when another background was already open, it performs a check to see which one and then it moves it back just like the "none" case, then it changes the frames on the other bar MovieClips.
The final two cases work in exactly the same way; the only difference between the three of these is the positions where each bar is being moved to and where its background is being moved to.
Here are the final two cases:
if(this.greenBackground.x<60){ this.greenBackground.x+=speed; if(this.redBar.x<620){ this.redBar.x+=speed; } if(this.redBackground.x<90){ this.redBackground.x+=speed; } if(this.goldBar.currentFrame==2){ this.goldBar.gotoAndStop(1); }else if(this.blueBar.currentFrame==2){ this.blueBar.gotoAndStop(1); }else if(this.redBar.currentFrame==2){ this.redBar.gotoAndStop(1); } } if(this.greenBar.x>60){ this.greenBar.x-=speed; if(this.goldBackground.x>-530){ this.goldBackground.x-=speed; } if(this.blueBar.x>30){ this.blueBar.x-=speed; } if(this.blueBackground.x>=-500){ this.blueBackground.x-=speed; } if(this.redBar.x<620){ this.redBar.x+=speed; } if(this.redBackground.x<90){ this.redBackground.x+=speed; } if(this.goldBar.currentFrame==2){ this.goldBar.gotoAndStop(1); }else if(this.blueBar.currentFrame==2){ this.blueBar.gotoAndStop(1); }else if(this.redBar.currentFrame==2){ this.redBar.gotoAndStop(1); } }
if(this.redBackground.x<90){ this.redBackground.x+=speed; if(this.goldBar.currentFrame==2){ this.goldBar.gotoAndStop(1); }else if(this.blueBar.currentFrame==2){ this.blueBar.gotoAndStop(1); }else if(this.greenBar.currentFrame==2){ this.greenBar.gotoAndStop(1); } } if(this.redBar.x>90){ this.redBar.x-=speed; if(this.goldBackground.x>-530){ this.goldBackground.x-=speed; } if(this.blueBar.x>30){ this.blueBar.x-=speed; } if(this.blueBackground.x>=-500){ this.blueBackground.x-=speed; } if(this.greenBar.x>60){ this.greenBar.x-=speed; } if(this.greenBackground.x>=-470){ this.greenBackground.x-=speed; } if(this.goldBar.currentFrame==2){ this.goldBar.gotoAndStop(1); }else if(this.blueBar.currentFrame==2){ this.blueBar.gotoAndStop(1); }else if(this.greenBar.currentFrame==2){ this.greenBar.gotoAndStop(1); } }
And with that all of the coding is complete for the accordion!
Conclusion
Right now you should be admiring your hard work and its magnificent result, but if you are wondering how to take the accordion to the next level and add more bars, then just remember the following:
- The backgrounds are the size of the stage minus the total width of all the bars - which in turn is the number of bars that there are multiplied by the width of one bar.
- Each clause of the switch case either moves all the bars and their backgrounds that are on the selected bar's right to the right, or moves the selected bar left with the bars to its left and their backgrounds, revealing its own background
Thank you for taking the time to read this article, I hoped you enjoyed it!
Comments