Internationalization - something you constantly hear developers talking about but rarely actually see people using in practice - is getting a kick in the pants with the new ECMAScript Internationalization API. Currently supported in Chrome 24, Chrome for Android, Firefox 29, IE 11, and Opera 15 (sadly no Safari support), the new Intl namespace provides a set of functionality to add internationalization to your numbers, dates, and sorting. In this article I'll demonstrate the major features of Intl and get you on the path to adopting support for the billions of people on the Internet who live outside your own country!
Core Features
The Intl namespace covers three main areas of functionality:
- Formatting numbers
- Formatting dates
- Sorting strings
Within each of these are various options for controlling both the locales used for formatting as well as formatting options. As an example, the number formatter includes support for handling currency. The date formatter has options for what parts of the date to display.
Lets take a look at a few examples.
Our Application
Our first application is a simple data reporter. It uses AJAX to fetch a set of data containing dates and numbers. First, the HTML:
Listing 1: test1.html
:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1"> <title></title> <meta name="description" content=""> <meta name="viewport" content="width=device-width"> </head> <body> <h2>Current Stats</h2> <table id="stats"> <thead> <tr> <th>Date</th> <th>Visits</th> </tr> </thead> <tbody></tbody> </table> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/2.1.0/jquery.min.js"></script> <script src="app1.js"></script> </body> </html>
Make note of the empty table. That's where we will be dumping our data. Now let's take a look at the JavaScript.
Listing 2: app1.js
:
$(document).ready(function() { //get the table dom $table = $("#stats tbody"); //now, get our data from the api, which is fake btw $.getJSON("stats.json").done(function(s) { //iterate over stats and add to table for(var i=0; i < s.length; i++) { $table.append(""+s[i].date+""+s[i].views+""); } }).fail(function(e) { console.log("we failed"); console.dir(e); }); });
All that the code does here, is make an AJAX call to a file and render the result to the data. The data file, stats.json
, is simply an array of ten hard coded values. Here is a portion of the file:
[{"date":"4/1/2013","views":938213},{"date":"4/2/2013","views":238213},
As you can see, the dates are formatted as month/date/year
and the numbers are passed, as is. This renders acceptably:
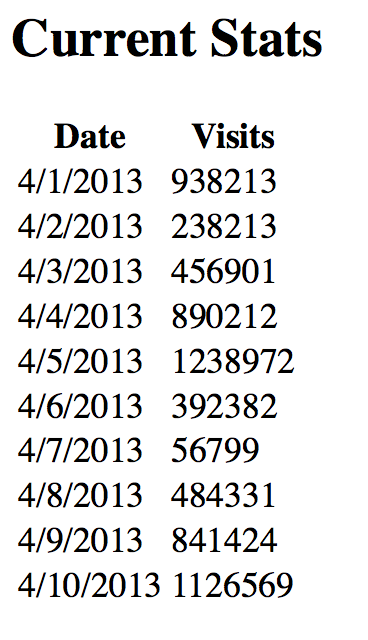
But note the the numbers are a bit hard to read without formatting. Let's begin by adding some formatting to the numbers.
Adding Number Formatting
The following modifications can be seen in app2.js
and tested with test2.html
. First, I'll modify my code to call a new function, numberFormat
:
$table.append(""+s[i].date+""+numberFormat(s[i].views)+"");
And here is that function:
function numberFormat(n) { //cache the formatter once if(window.Intl && !window.numberFormatter) window.numberFormatter = window.Intl.NumberFormat(); if(window.numberFormatter) { return window.numberFormatter.format(n); } else { return n; } }
The function begins by checking if Intl
exists as part of the window
object. If it does, we then check to see if we've made the formatter before. The Intl
object creates a formatting object that you can reuse, and since we're formatting a bunch of numbers, we only want to create it one time. Which is exactly what we do as soon as we see that we need too. We don't bother with any options for now, just to keep it simple. Finally, if there was no support for Intl
at all, we just return the number as is. The result is a significant improvement, with minimal work:
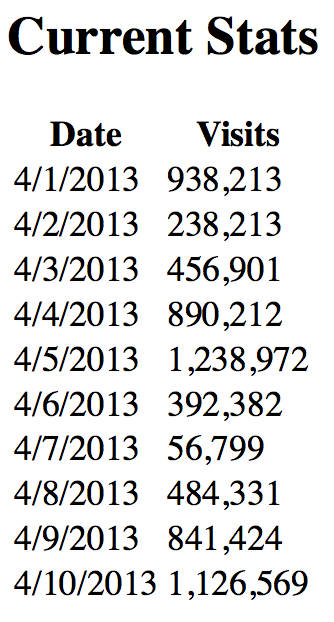
Cool! So, how do we test other languages? You may be tempted to check your browser settings. All browsers have a preference for language, but unfortunately, changing the language in the browser is not enough. Changing it does impact how the browser behaves.
If you open your dev tools and look at network requests, you can see that a header called "Accept-Lanage" will change based on your settings. If you add French, for example (I'm assuming you aren't a native French speaker), you will see "fr" added to this header. But this does not impact Intl
. Instead, you have to change your operating system language and restart the browser. This is not as scary as it sounds. When I tested, I was worried my entire operating system would change immediately. But in my tests, I was able to change the language, restart my browser, and see the change. I quickly changed back. The Intl formatter functions allow you to override the current locale and pass one in instead.
I modified the application to allow the end user to specify a language via a drop down. Here's the modification made to the HTML. (This modification may be found in test3.html
)
<select id="langDropdown"> <option value="">None Specified</option> <option value="en-US">English (US)</option> <option value="fr-FR">French (France)</option> <option value="lt">Lithuanian</option> </select>
The languages I picked were pretty arbitrary. Next - I updated my application code to listen to changes to this drop down as well as checking the desired locale when formatting.
Listing 3: app3.js
:
function numberFormat(n) { if(window.Intl) { var lang = $("#langDropdown").val(); if(lang === "") lang = navigator.language; var formatter = new window.Intl.NumberFormat(lang); return formatter.format(n); } else { return n; } } function getStats() { $.getJSON("stats.json").done(function(s) { //iterate over stats and add to table for(var i=0; i < s.length; i++) { $table.append(""+s[i].date+""+numberFormat(s[i].views)+""); } }).fail(function(e) { console.log("we failed"); console.dir(e); }); } $(document).ready(function() { //get the table dom $table = $("#stats tbody"); //notice changes to drop down $("#langDropdown").on("change", function(e) { $table.empty(); getStats(); }); getStats(); });
Starting at the bottom - note that I've added a simple event handler for changes to the drop down. When a change is detect, the table is emptied out and the function getStats
is run. This new function simply abstracts the AJAX code used previously. The real change now is in numberFormat
. I check the language selected and if one of them was chosen, we pass that as the locale to the NumberFormat
constructor. Note that if something wasn't chosen, we default to navigator.language
. This now gives us a way to quickly test different locales and see how they render numbers.
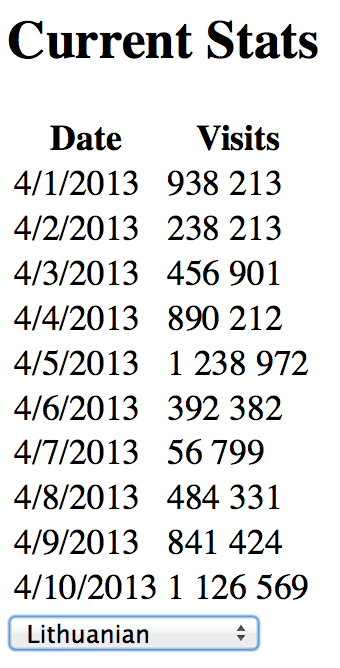
Adding Date Formatting
Now is a perfect time to take care of the other column of data - the numbers. I followed the same style as before and added a call to a new function, dateFormat
.
$table.append(""+dateFormat(s[i].date)+""+numberFormat(s[i].views)+"");
And here is dateFormat
(You can find the code in app4.js
, which is used by test4.html
):
function dateFormat(n) { //Used for date display var opts = {}; opts.day = "numeric"; opts.weekday = "long"; opts.year = "numeric"; opts.month = "long"; if(window.Intl) { var lang = $("#langDropdown").val(); if(lang === "") lang = navigator.language; var formatter = new window.Intl.DateTimeFormat(lang, opts); n = new Date(n); return formatter.format(n); } else { return n; } }
This is very similar to number formatting, except this time we are explicitly passing some options when we create the formatter. The options specify both what fields are visible in the date, as well as how they look. Each part of a date can be shown or not, and each one has different options. The options include:
- weekday
- era
- year
- month
- day
- hour
- minute
- second
- timeZoneName
For a full list of what values you can use, see the MDN documentation for DateTimeFormat
, but as an example, months can be displayed as a number or in different textual forms. So what does this create? Here is the English version:
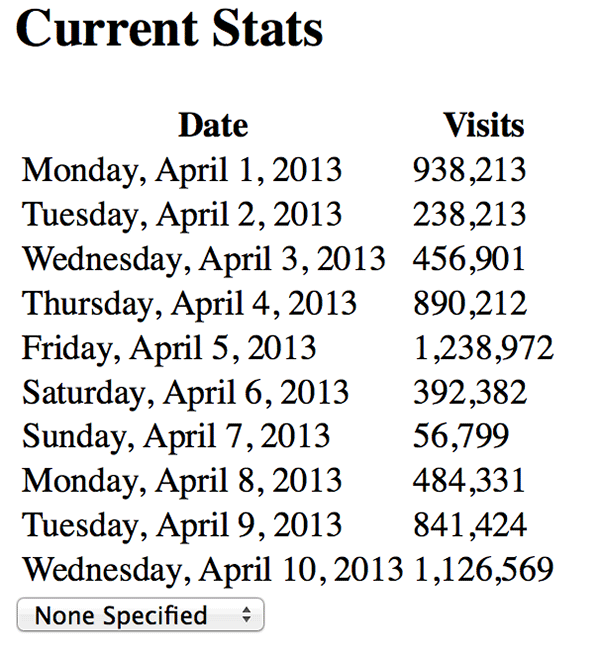
And here it is in French:
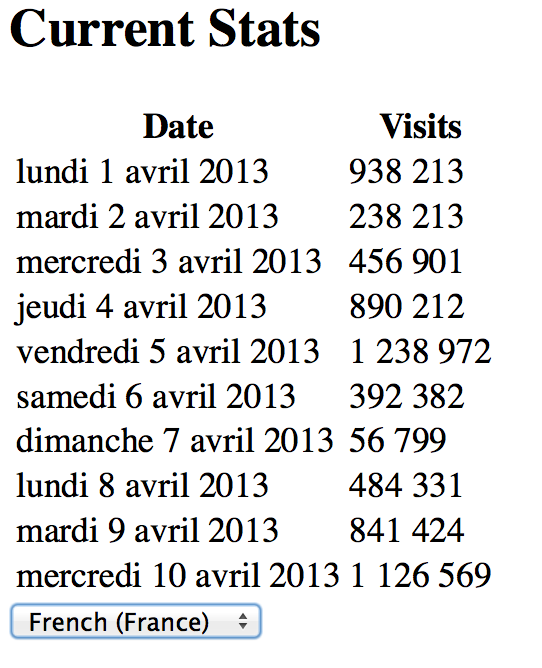
You may be wondering - what covers the location of each field? As far as I can tell, you have no control over this. You could, of course, create multiple formatters and then combine them together, but using one formatter the layout of the fields is driven by internal logic. If you turn off the day of the month, for example, here is what you get: April 2013 Monday. Why? Honestly I have no idea.
Finally - note that you need to pass a date value, not a string, to the formatter. You can see where I use the date constructor in the formatter to parse my string-based date. This is - a bit loose - so keep this in mind when using this functionality.
Show Me the Money
Currency formatting isn't a separate object, but rather an optional use of the number formatter. For the next demo, we've created a new data file, stats2.json
, and added a "sales" column to our data. Here is a sample:
{"date":"4/1/2013","views":938213,"sales":3890.21},{"date":"4/2/2013","views":238213,"sales":9890.10}
The column was added to the HTML (test5.html
), added within the JavaScript iterating over the rows of data (see app5.js
), and passed to a new function called currencyFormat
. Let's look at that.
function currencyFormat(n) { var opts = {}; opts.style = "currency"; opts.currency = "USD"; if(window.Intl) { var lang = $("#langDropdown").val(); if(lang === "") lang = navigator.language; var formatter = new window.Intl.NumberFormat(lang,opts); return formatter.format(n); } else { return n; } }
Displaying numbers as currencies requires two optional values. First, a style of "currency", and then the currency type. Other options (like how to display the name of the currency) exists as well. Here comes the part that may trip you up a bit. You must specify the currency type.
You may think - how in the heck do I figure out the currency type for all the possible values? The possible values are based on a spec (http://www.currency-iso.org/en/home/tables/table-a1.html) and in theory you could parse their XML, but you don't want to do that. The reason why is pretty obvious but I can honestly say I forgot initially as well. You do not want to just redisplay a particular number in a locale specific currency. Why? Because ten dollars American is certainly not the same as ten dollars in pesos. That's pretty obvious and hopefully I'm the only person to forget that.
Using the code above, we can see the following results in the French locale. Note how the numbers are formatted right for the locale and the currency symbol is placed after the number.
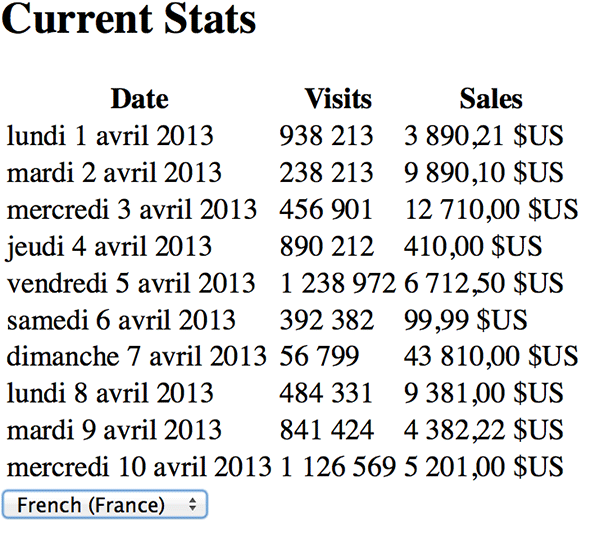
Sorting With Collator
For our final example, we'll look at the Collator
constructor. Collators allow you to handle text sorting. While some languages follow a simple A to Z ordering system other languages have different rules. And of course, things get even more interesting when you start adding accents. Can you say, for certain, if ä comes after a? I know I can't. The collator constructor takes a number of arguments to help it specify exactly how it should sort, but the default will probably work well for you.
For this example, we've built an entirely new demo, but one similar to the previous examples. In test6.html
, you can see a new table, for Students
. Our new code will load up a JSON packet of students and then sort them on the client. The JSON data is simply an array of names so I'll skip showing an excerpt. Let's look at the application logic.
Listing 4: app6.js
:
function sorter(x,y) { if(window.Intl) { var lang = $("#langDropdown").val(); if(lang === "") lang = navigator.language; return window.Intl.Collator(lang).compare(x,y); } else { return x.localeCompare(y); } } function getStudents() { $.getJSON("students.json").done(function(s) { //iterate over stats and add to table s.sort(sorter); for(var i=0; i < s.length; i++) { $table.append(""+s[i]+""); } }).fail(function(e) { console.log("we failed"); console.dir(e); }); } $(document).ready(function() { //get the table dom $table = $("#students tbody"); //notice changes to drop down $("#langDropdown").on("change", function(e) { $table.empty(); getStudents(); }); getStudents(); });
As I said, this code is pretty similar to the previous examples, so focus on getStudents
first. The crucial line here is: s.sort(sorter)
. We're using the built in function for arrays to do sorting via a custom function. That function will be passed two things to compare and must return -1
, 0
, or 1
to represent how the two items should be sorted. Now let's look at sorter.
If we have an Intl
object, we create a new Collator
(and again, we're allowing you to pass in a locale) and then we run the compare
function. That's it. As I said, there are options to modify how things are sorted, but we can use the defaults. The fallback is localeCompare
, which will also attempt to use locale specific formatting, but has (in this form) slightly better support. We could check for that support as well and add one more fallback for really good support, but I'll leave that for you, as an exercise.
We've also modified the front end to use Swedish as a language. I did this because the excellent MDN documentation showed that it was a good way to see the sorting in action. Here's the English sort of our student names:
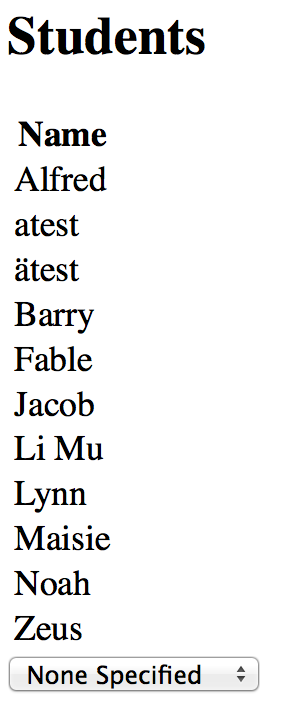
And here it is in Swedish:
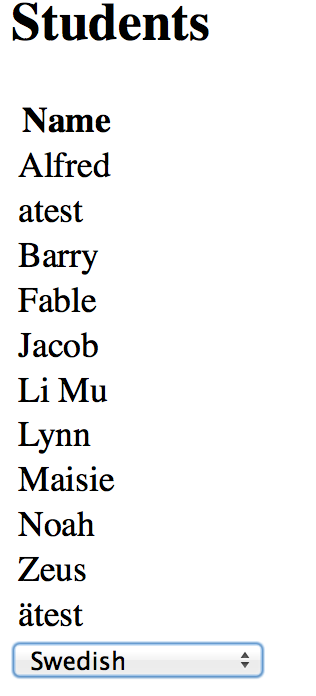
Note how ätest is sorted differently. (Sorry, I couldn't think of a name that began with ä.)
Wrap Up
All in all, the Intl
class provides some very simple ways to add locale specific formatting to your code. This is certainly something you can find now, probably in a few thousand different JavaScript libraries, but it is great to see the browser manufacturers adding in support directly within the language itself. The lack of iOS support is a bummer, but hopefully it will be added soon.
Thanks goes to the excellent Mozilla Developer Network for its great Intl documentation.
Comments