Avid readers of Envato Tuts+ come from a wide variety of backgrounds in terms of experience, culture, and what they are looking to learn. When it comes to technology, it's easy to take for granted those who are just starting out, especially if you've done any type of development for an extended amount of time.
That said, one of the nice things about becoming a developer is that once you've gotten a handle on a particular language and set of skills, it's easy to translate them into other areas of development.
In an attempt to make sure we're reaching the widest audience possible, we're aiming to publish content aimed directly at beginners who are curious about a given language, platform, or application.
And in this article, we're going to be focused exclusively on jQuery. Specifically, we're going to take a high-level look at everything that jQuery offers and how it can help us, and we're going to review some of the projects that have also come to fruition from the initial library.
We've also built a comprehensive guide to help you learn JavaScript, whether you're just getting started as a web developer or you want to explore more advanced topics:
All About jQuery
First released in 2006 by John Resig, jQuery set out to be a cross-platform JavaScript library that made it easier to write client-side solutions.
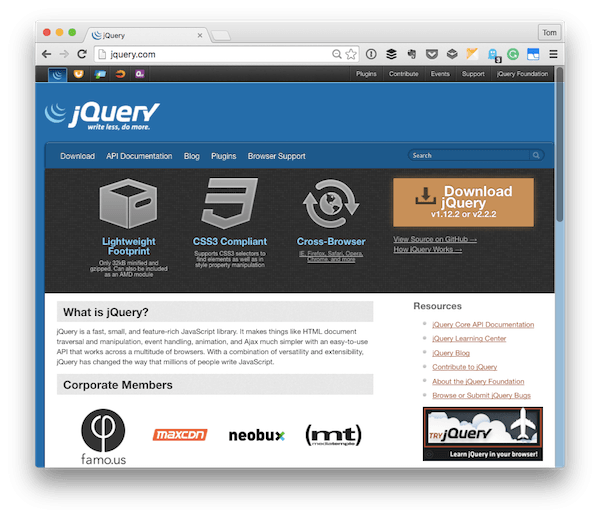
At the time this was released, it was especially useful because of the inconsistencies that existed among JavaScript implementations in Internet Explorer, Firefox, and eventually Google Chrome (which wasn't released until 2008).
jQuery is a cross-platform JavaScript library designed to simplify the client-side scripting of HTML. jQuery is the most popular JavaScript library in use today, with installation on 65% of the top 10 million highest-trafficked sites on the Web. jQuery is free, open-source software licensed under the MIT License.
Furthermore, the jQuery website itself says:
jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers. With a combination of versatility and extensibility, jQuery has changed the way that millions of people write JavaScript.
But what does this mean for us as developers? Perhaps the best way for us to understand what all the library offers is to examine what it claims to offer.
1. HTML Document Traversal
When a browser renders a web page, it's a visual representation of what's known as the DOM (or the document object model). This model can be conceptually modeled as a tree data structure where there are certain nodes each with roots and leaves.
For example, see this image as provided by the Web Step Book:
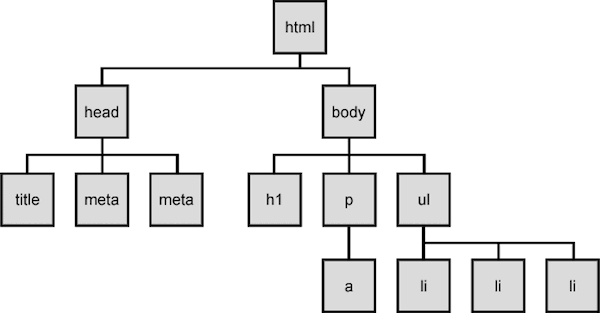
When you're working with jQuery, you can easily traverse the contents of the DOM in order to reach or to find the nodes, elements, or values you're aiming to retrieve.
This means if you're looking for the text of a div
element that has a unique ID, it's easy to do.
/** * This code looks for a div with the ID of "my unique element" * and then removes it from view. */ $( 'div#my-unique-element' ).hide();
If you're trying to loop through all of the span
elements, you can do that as well:
/** * This is the basic way to setup a loop in jQuery. It will * take all of the span elements on the page and then * allow you to iterate through them. */ $( 'span' ).each(function() { // Process the span element here });
We'll review this particular functionality a little bit more in the next section as it goes to show some of the additional work you can do to manipulate the page.
Granted, these examples are simple, and things can get more complicated, especially as we introduce method chaining. For example:
$excerpt.on( 'keydown', function( evt ) { if ( ( 17 === evt.keyCode || 91 === evt.keyCode ) || 86 === evt.keyCode ) { if ( -1 === $.inArray( evt.keyCode, keymap ) ) { keymap.push( evt.keyCode ); } } }).on( 'keyup', function() { if ( user_has_pasted_content( keymap ) ) { resize_textarea( this ); keymap = []; } });
At this point, you're not supposed to know what's going on with the code, but it's meant to show you how useful jQuery can be in certain situations through the use of helper functions and method chaining.
Suffice it to say, the power of jQuery lies in its ability to query the DOM (hence the name jQuery) and then make adjustments to it through the use of a well-documented API (that's replete with examples of how to use each function).
One could argue that everything else stems from that feature alone. So with that said, let's continue to look at some of what that looks like.
2. HTML Document Manipulation
When it comes to actually manipulating the DOM, jQuery has a lot of functions that allow us to change what our visitors see.
Some of these functions are simple, such as allowing us to show
or hide
elements that aren't visible whenever a page loads. Other functions allow us to create new elements and append
them to an existing element, or prepend
them in front of an entire list.
If you're trying to loop through all of the span
elements in order to add a class attribute to them, you can do that, as well:
/** * This is the basic way to setup a loop in jQuery. It will * take all of the span elements on the page and then * add a custom class attribute to them. */ $( 'span' ).each(function() { $( this ).addClass( 'my-custom-class' ); });
This is barely scratching the surface of what DOM manipulation functionality is available within jQuery. By looking at the API, under the Manipulation section, you can see just how many options are available to us (along with good examples).
To give further examples, we can also:
- determine height or width of the document, the window, or any given element
- grab the values from any given element (assuming it offers this ability)
- toggle class names
- and much more
Remember that one of the things that make jQuery an attractive solution for so many developers is that all of the functions and examples we're looking at in this article are cross-browser compatible.
3. Event-Handling
If you're brand new to JavaScript, then one thing that's key to understanding how it works with the page that's being displayed in the web browser is that it responds to various events.
That is, when a user clicks on an element, makes a keystroke, or clicks the mouse, then the browser raises a signal corresponding to the event that occurred. This is what allows us to take advantage of the user's interaction with the browser.
Specifically, every time a user does something to the page, we can respond using a custom event. The problem is that not every browser implements events the same way (this is why there's a need for a specification, but that's content for another post).
Luckily, jQuery makes this much easier by defining a consistent name for all of the events such that we can use the same name for an event to which we're trying to respond and it will work across all of the major browsers.
4. Animation
When jQuery first came out, Flash was still relatively popular, and general animations across the web weren't completely dead.
When we talk about animation in the context of jQuery, though, we're not talking about the same type of effects or behaviors that we're used to seeing with older technology. Instead, we're talking about effects that give users feedback that something has happened on the screen. Furthermore, it's less invasive and can add a nice sense of style to a page or application when used correctly (anything can be abused, though).
You can view the entire effects API on this page, but it's worth noting that jQuery's effects can range anywhere from handling simple fading in and fading out of elements or sliding elements into view to something more complex such as manipulating the queue of effects that are registered to fire against an element.
Granted, the latter case assumes you have a bit of experience with the effects API, but it's something that comes naturally given enough time with the library and the documentation.
5. Ajax
If you're not familiar with Ajax, it's essentially a way that a web page can make a call to the server, handle the response, and update a portion of a page without having to refresh the entire page. Though the technology has been around for quite some time now, it's still something that's really cool and can provide some really neat functionality within the context of a page or web application when used appropriately and effectively.
Though support for Ajax isn't as bad as it was five or ten years ago, the implementation for the API across browsers can vary ever so slightly. This means that we're tasked with writing Ajax code specifically for a browser Microsoft provides, that Google provides, that Apple provides, that Chrome provides, and so on.
At least, that's the case without jQuery. Thanks to its support for Ajax, we can leverage Ajax in a number of different ways without having to get into the cross-browser inconsistencies. In fact, it's trivially easy to handle GET
and POST
requests while also having the ability to make far more advanced calls using the $.ajax
method.
Once you get used to having the API available in the core of your application or at your disposal, it's difficult to imagine not working with it (or with something like it).
A Word About Extensibility
One feature that a lot of server-side frameworks and libraries offer is the ability to create extensions to the core codebase. Modern client-side libraries and frameworks allow this, and jQuery is no different.
Say, for example, you work in a particular niche in which you find yourself creating the same functionality for each project. Or what if you have a product that you're selling and you have a bit of custom code that needs to integrate with jQuery, but it might require different parameters depending on the project.
What do you do then?
Fortunately, jQuery supports plugins. This means that we, as developers, not only have the ability to tap into plugins that others have written (some of which are available on the jQuery website, others being available on GitHub), but we're also able to develop our own plugins.
We can then reuse this code in our own projects, or make them available on sites such as GitHub for others to offer contributions, fixes, features, and so on.
Additional jQuery Projects
Since its inception, jQuery has grown into more than just a JavaScript library that offers us the ability to perform both simple and powerful operations in a cross-platform compatible way.
In addition to the core library, jQuery has also resulted in two other notable projects that are worth mentioning before we wrap this article. Although we're not going to look at the details of what each project affords, we will take a high-level view of each project, if for no other reason than being aware of what's available to us should we need this for future work.
jQuery UI
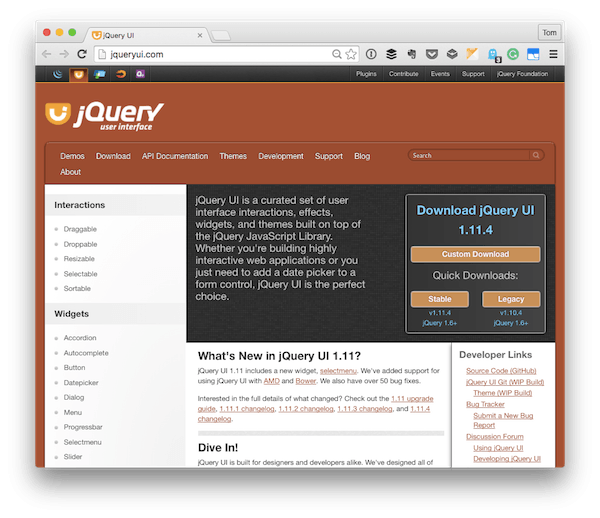
From the jQuery UI homepage:
jQuery UI is a curated set of user interface interactions, effects, widgets, and themes built on top of the jQuery JavaScript Library. Whether you're building highly interactive web applications or you just need to add a date picker to a form control, jQuery UI is the perfect choice.
This library was first published in 2007, about a year after jQuery itself. It works as a complementary library to jQuery in that it leverages the cross-platform compatibility of the library to help create widgets that can be used throughout a website.
Many of the widgets include commonly used pieces of functionality. For example:
- Datepicker
- Dialogs
- Progress Bars
- Tooltips
- Autocomplete
- and more
There are also advanced features such as effects, utilities, and interactions. Everything that we've covered so far (as well as the things we haven't) include a wide variety of callbacks, attributes, and functions that allow us to interact with them to the fullest extent.
All of the aforementioned features also offer various themes to make sure they fit the look and feel of your website. Finally, all of the features outlined here and included on the site are well documented.
jQuery Mobile
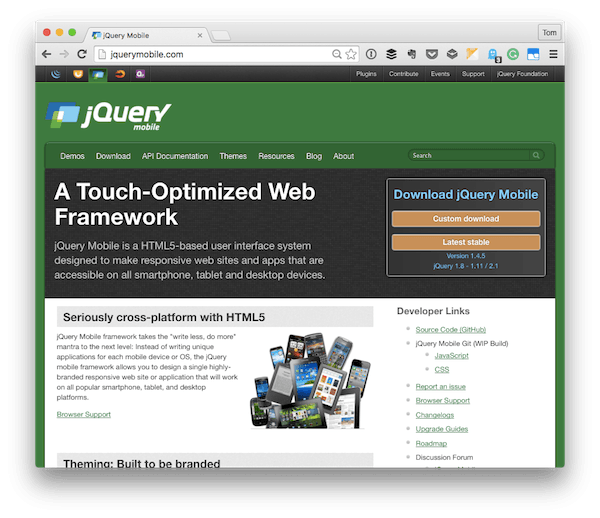
From the jQuery Mobile homepage:
jQuery Mobile is a HTML5-based user interface system designed to make responsive web sites and apps that are accessible on all smartphone, tablet and desktop devices.
This library is the most recent introduction into the family of libraries being released in 2010 (with the last stable release being in 2014).
Much like its UI counterpart, it offers a well-documented API and custom themes that are ideal for the various devices your project may target.
Whereas the previous two libraries offer a set of cross-platform features that allow us to write jQuery and accompanying widgets in a relatively easy manner, jQuery Mobile includes a CSS framework that allows us to also design user interfaces that are ideal for the nature of our respective project.
The framework includes:
From there, the library offers what you'd expect from a project geared towards making web development much easier for various mobile devices. These include things as:
- a consistent icon set
- events that work on a plethora of devices
- properties for the active page
- a number of widgets that are ideal for mobile interfaces
Finally, the number of browsers that are still available and used in the wild is high. Though we've seen a decrease in the usage of older versions of Internet Explorer and wider adoption of Chrome, we still have certain users sticking with older browsers for a number of reasons.
Sometimes, these users are on older browsers because of the nature of their company intranet. Sometimes it has to do with the mobile devices and/or phones they've been assigned for their job. And sometimes it simply has to do with the inability to upgrade to something better.
No matter, though. jQuery Mobile offers support for most of the browsers and operating systems that are currently available. If you're not sure if the platform that you're targeting is supported by the library, you can always check the browser support page.
Additional Resources
- The jQuery Learning Center
- Learning jQuery, Fourth Edition
- jQuery in Action, Third Edition
- jQuery Succinctly
- Why Is jQuery Undefined?
- 20 Useful jQuery Sliders
- Uncommon jQuery Selectors
- Create a Find and Replace Plugin in jQuery
Conclusion
Understanding what jQuery is (and what it isn't) and how it's related to JavaScript is important so that you know what's being done for you and what you can do when needing to work with the library.
As previously mentioned, some may argue that you need to learn JavaScript first and then learn jQuery; others may argue that learning jQuery is a great way to work your way backwards to JavaScript.
Whatever the case, jQuery is a longstanding library in the JavaScript economy and is one that's used in a number of very popular projects (such as WordPress) so learning it will give you a leg up in a number of different ways.
JavaScript has become one of the de-facto languages of working on the web. It’s not without its learning curves, and there are plenty of frameworks and libraries to keep you busy, as well. If you’re looking for additional resources to study or to use in your work, check out what we have available in the Envato marketplace.
If that's not enough, there's plenty of documentation and open-source code available for you to review and read, as well. There are also widely available plugins and an active blog to keep you in the loop with all of the news happening with the library's development.
For those who are interested in JavaScript (particularly in the context of WordPress), feel free to follow me on my blog and/or Twitter at @tommcfarlin. You can catch all of my courses and tutorials on my profile page, as well.
Don't hesitate to leave any questions or comments in the feed below, and I'll aim to respond to each of them.
Comments