In the first article of Swift From Scratch, you learned about Xcode playgrounds and wrote your first lines of Swift. In this article, we'll start learning the fundamentals of the Swift programming language by exploring variables and typing. We'll also take a close look at constants and why you're encouraged to use them as much as possible.
In the next installments of this series, we're going to make use of Xcode playgrounds to learn the fundamentals of the Swift programming language. As we saw in the previous article, playgrounds are ideal for teaching and learning Swift.
Let's start by creating a new playground for this tutorial. I encourage you to follow along! Using a language is a great way to learn its syntax and understand its concepts.
Launch Xcode 8 and create a new playground by selecting New > Playground... from Xcode's File menu. Enter a name for the playground, set Platform to iOS, and click Next. Tell Xcode where you'd like to save the playground and hit Create.
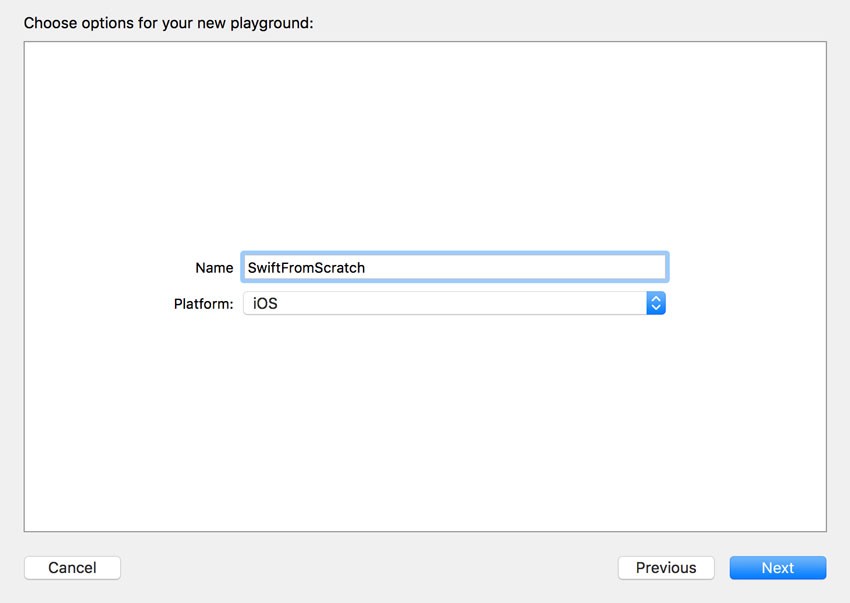
Clear the contents of the playground so we can start with a clean slate. We've already made use of variables in the previous tutorial, but let's now take a closer look at the nitty-gritty details to better understand what Swift is doing behind the scenes.
1. Variables
Declaring Variables
In Swift, we use the var
keyword to declare a variable. While this is similar to how variables are declared in other programming languages, I strongly advise you not to think about other programming languages when using the var
keyword in Swift. There are a few important differences.
The var
keyword is the only way to declare a variable in Swift. The most common and concise use of the var
keyword is to declare a variable and assign a value to it.
var street = "5th Avenue"
Remember that we don't end this line of code with a semicolon. While a semicolon is optional in Swift, the best practice is not to use a semicolon if it isn't required.
You also may have noticed that we didn't specify a type when declaring the variable street
. This brings us to one of Swift's key features, type inference.
Type Inference
The above statement declares a variable street
and assigns the value 5th Avenue
to it. If you're new to Swift or you're used to JavaScript or PHP, then you may be thinking that Swift is a typeless or loosely typed language, but nothing could be further from the truth. Let me reiterate that Swift is a strongly typed language. Type safety is one of the cornerstones of the language.
We're just getting started, and Swift already shows us a bit of its magic. Even though the above statement doesn't explicitly specify a type, the variable street
is of type String
. This is Swift's type inference in action. The value we assign to street
is a string. Swift is smart enough to see that and implicitly sets the type of street
to String
.
The following statement gives us the same result. The difference is that we explicitly set the type of the variable. This statement literally says that street
is of type String
.
var street: String = "5th Avenue"
Swift requires you to explicitly or implicitly set the type of variables and constants. If you don't, Swift complains by throwing an error. Add the following line to your playground to see what I mean.
var number
This statement would be perfectly valid in JavaScript or PHP. In Swift, however, it is invalid. The reason is simple. Even though we declare a variable using the var
keyword, we don't specify the variable's type. Swift is unable to infer the type since we don't assign a value to the variable. If you click the error, Xcode tells you what is wrong with this statement.
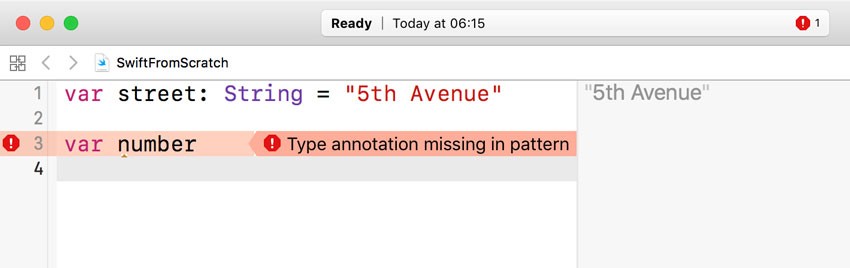
We can easily fix this issue by doing one of two things. We can assign a value to the variable as we did earlier, or we can explicitly specify a type for the variable number
. When we explicitly set the type of number
, the error disappears. The below line of code reads that number
is of type String
.
var number: String
Changing Type
As you can see below, assigning new values to street
and number
is simple and comes with no surprises.
var street: String = "5th Avenue" var number: String street = "Main Street" number = "10"
Wouldn't it be easier to assign the number 10
to the number
variable? There's no need to store the street number as a string. Let's see what happens if we do.
var street: String = "5th Avenue" var number: String street = "Main Street" number = 10
If we assign an integer to number
, Xcode throws another error at us. The error message is clear. We cannot assign a value of type Int
to a variable of type String
. This isn't a problem in loosely typed languages, but it is in Swift.
Swift is a strongly typed language in which every variable has a specific type, and that type cannot be changed. Read this sentence again to let it sink in because this is an important concept in Swift.
To get rid of the error, we need to declare the number
variable as an Int
. Take a look at the updated example below.
var street: String = "5th Avenue" var number: Int street = "Main Street" number = 10
Summary
It's important that you keep the following in mind. You can declare a variable with the var
keyword, and you don't need to explicitly declare the variable's type. However, remember that every variable—and constant—has a type in Swift. If Swift can't infer the type, then it complains. Every variable has a type, and that type cannot be changed.
2. Constants
Constants are similar to variables in terms of typing. The only difference is that the value of a constant cannot be changed once it has a value. The value of a constant is, well... constant.
Declaring Constants
To declare a constant, you use the let
keyword. Take a look at the following example in which we declare street
as a constant instead of a variable.
let street: String = "5th Avenue" var number: Int street = "Main Street" number = 10
If we only update the first line, replacing var
with let
, Xcode throws an error for obvious reasons. Trying to change the value of a constant is not allowed in Swift. Remove or comment out the line in which we try to assign a new value to street
to get rid of the error.
let street: String = "5th Avenue" var number: Int // street = "Main Street" number = 10
Using Constants
I hope you agree that declaring constants is very easy in Swift. There's no need for exotic keywords or a complex syntax. Declaring constants is just as easy as declaring variables, and that's no coincidence.
The use of constants is encouraged in Swift. If a value isn't going to change or you don't expect it to change, then it should be a constant. This has a number of benefits. One of the benefits is performance, but a more important benefit is safety. By using constants whenever possible, you add constraints to your code, which results in safer code.
3. Data Types
Most programming languages have a wide range of types for storing strings, integers, floats, etc. The list of available types in Swift is concise. Take a look at the following list:
Int
Float
Double
String
Character
Bool
It's important to understand that the above types are not basic or primitive types. They are named types, which are implemented by Swift using structures. We explore structures in more detail later in this series, but it's good to know that the types we encountered so far are not the same as the primitive types you may have used in, for example, Objective-C.
There are many more data types in Swift, such as tuples, arrays, and dictionaries. We explore those later in this series.
4. Type Conversion
There is one more topic that we need to discuss, type conversion. Take a look at the following Objective-C snippet. This code block outputs the value 314.000000
to the console.
int a = 100; float b = 3.14; NSLog(@"%f", (a * b));
The Objective-C runtime implicitly converts a
to a floating point value and multiplies it with b
. Let's rewrite the above code snippet using Swift.
var a = 100 var b = 3.14 print(a * b)
Ignore the print(_:separator:terminator:)
function for now. I first want to focus on the multiplication of a
and b
. Swift infers the type of a
, an Int
, and b
, a Double
. However, when the compiler attempts to multiply a
and b
, it notices that they are not of the same type. This may not seem like a problem to you, but it is for Swift. Swift doesn't know what type the result of this multiplication should be. Should it be an integer or a double?
To fix this issue, we need to make sure both operands of the multiplication are of the same type. Swift doesn't implicitly convert the operands for us, but it is easy to do so. In the updated example below, we create a Double
using the value stored in a
. This resolves the error.
var a = 100 var b = 3.14 print(Double(a) * b)
Note that the type of a
hasn't changed. Even though the above code snippet may look like type casting, it is not the same thing. We use the value stored in a
to create a Double
, and that result is used in the multiplication with b
. The result of the multiplication is of type Double
.
What you need to remember is that Swift is different from C and Objective-C. It doesn't implicitly convert values of variables and constants. This is another important concept to grasp.
5. Print
In the previous code snippet, you invoked your first function, print(_:separator:terminator:)
. This function is similar to Objective-C's NSLog
; it prints something and appends a new line. To print something to the console or the results panel on the right, you invoke print(_:separator:terminator:)
and pass it a parameter. That can be a variable, a constant, an expression, or a literal. Take a look at the following examples.
var string = "this is a string" let constant = 3.14 print(string) print(constant) print(10 * 50) print("string literal")
It's also possible to use string interpolation to combine variables, constants, expressions, and literals. String interpolation is very easy in Swift. Wrap the variables, constants, expressions, or literals in \()
. Easy as pie.
let street = "5th Avenue" let number = 10 print("She lives at \(street) \(number).")
Conclusion
It is key that you understand how Swift handles variables and constants. While it may take some time to get used to the concept of constants, once you've embraced this best practice, your code will be much safer and easier to understand. In the next tutorial of this series, we'll continue our exploration of Swift by looking at collections.
If you want to learn how to use Swift 3 to code real-world apps, check out our course Create iOS Apps With Swift 3. Whether you're new to iOS app development or are looking to make the switch from Objective-C, this course will get you started with Swift for app development.
Comments