Sails is a Javascript framework designed to resemble the MVC architecture from frameworks like Ruby on Rails. It makes the process of building Node.js apps easier, especially APIs, single page apps and realtime features, like chat.
Installation
To install Sails, it is quite simple. The prerequisites are to have Node.js installed and also npm, which comes with Node. Then one must issue the following command in the terminal:
sudo npm install sails -g
Create a New Project
In order to create a new Sails project, the following command is used:
sails new myNewProject
Sails will generate a new folder named myNewProject
and add all the necessary files to have a basic application built. To see what was generated, just get into the myNewProject
folder and run the Sails server by issuing the following command in the terminal:
sails lift
Sails's default port is 1337, so if you visit http://localhost:1337
you should get the Sails default index.html
page.
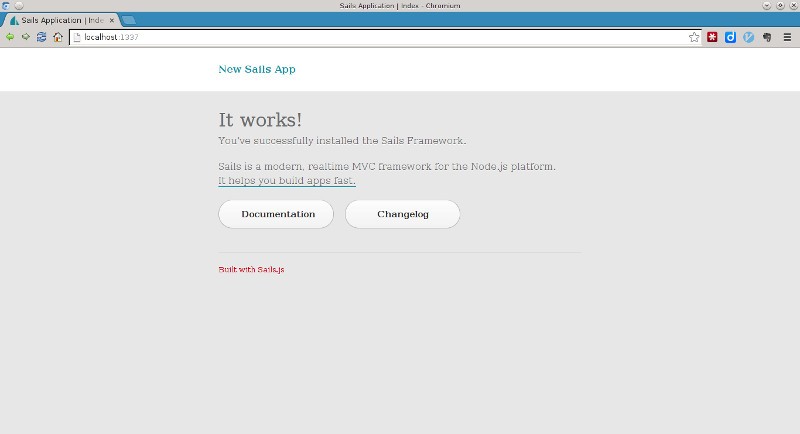
Now, let's have a look at what Sails generated for us. In our myNewProject
folder the following files and sub-folders were created:
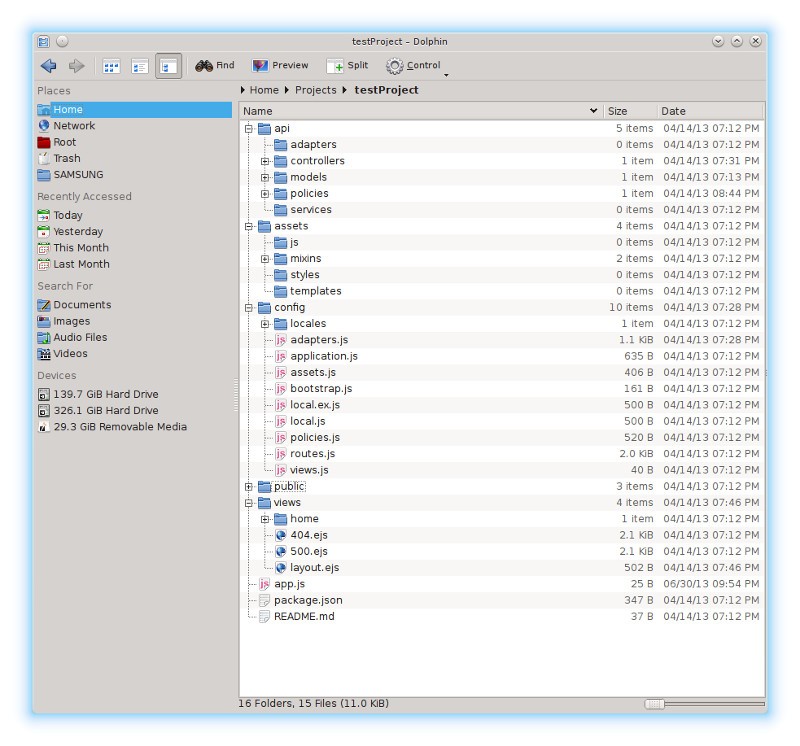
The assets
Folder
The assets
folder contains subdirectories for the Javascript and CSS files that should be loaded during runtime. This is the best place to store auxiliary libraries used by your application.
The public
Folder
Contains the files that are publicly available, such as pictures your site uses, the favicon, etc.
The config
Folder
This is one of the important folders. Sails is designed to be flexible. It assumes some standard conventions, but it also allows the developer to change the way Sails configures the created app to fit the project's needs. The following is a list of configuration files present in the config
folder:
-
adapters.js
- used to configure the database adapters -
application.js
- general settings for the application -
assets.js
- asset settings for CSS and JS -
bootstrap.js
- code that will be ran before the app launches -
locales
- folder containing translations -
policies.js
- user rights management configuration -
routes.js
- the routes for the system -
views.js
- view related settings
The sails.js
documentation contains detailed information on each of these folders.
The views
Folder
The application's views are stored in this folder. Looking at its contents, we notice that the views are generated by default as EJS (embedded JavaScript). Also, the views
folder contains views for error handling (404 and 500) and also the layout file (layout.ejs
) and the views for the home controller, which were generated by Sails.
The api
Folder
This folder is composed from a buch of sub-folders:
- the
adapters
folder contains the adapters used by the application to
handle database connections - the
controllers
folder contains the application controllers - the application's models are stored in the
models
folder - in the
policies
folder are stored rules for application user access - the api services implemented by the app are stored in the
services
folder
Configure the Application
So far we have created our application and took a look at what was generated by default, now it's time to configure the application to make it fit our needs.
General Settings
General settings are stored in the config/application.js
file. The configurable options for the application are:
- application name (
appName
) - the port on which the app will listen (
port
) - the application environment; can be either development or production (
environment
) - the level for the logger, usable to control the size of the log file (
log
)
Note that by setting the app environment
to production, makes Sails bundle and minify the CSS and JS, which can make it harder to debug.
Routes
Application routes are defined in the config/routes.js
file. As you'd expect, this file will be the one that you will most often work with as you add new controllers to the application.
The routes are exported as follows, in the configuration file:
module.exports.routes = { // route to index page of the home controller '/': { controller: 'home' }, // route to the auth controller, login action '/login': { controller: 'auth', action: 'login' }, // route to blog controller, add action to add a post to a blog // note that we use also the HTTP method/verb before the path 'post /blog/add': { controller: 'blog', action: 'add_post' }, // route to get the first blog post. The find action will return // the database row containing the desired information '/blog/:item': { controller: blog, action: find } }
Views
Regarding views, the configurable options are the template engine to be used and if a layout should or not be used, for views.
Models
Models are a representation of the application data stored in a database. Models are defined by using attributes and associations. For instance, the definition of a Person
model might look like this:
// Person.js var Person = { name: 'STRING', age: 'INTEGER', birthDate: 'DATE', phoneNumber: 'STRING', emailAddress: 'STRING' }; exports = Person;
The communication with the underlying database is done through adapters. Adapters are defined in api/adapters
and are configured in the adapters.js
file. At the moment of writing this article, Sails comes with three adapters: memory, disk and mysql but you can write your own adapter (see the documentation for details).
Once you have a model defined you can operate on it by creating records, finding records, updating and destroying records.
Controllers
Controllers are placed in api/controllers
. A controller is created using the following command:
sails generate controller comment
This command will generate a CommentController
object. Actions are defined inside this object. Actions can also be generated when you issue the generate controller
command:
sails generate controller comment create destroy tag like
This will create a Comment
controller with actions for create
, destroy
, tag
and like
.
Actions receive as parameters the request and the response objects, which can be used for getting parameters of the URI (the request object) or output in the view (using the response object).
To communicate with the model, the callback of the appopriate action is used. For instance, in the case of querying a database with find
, the following pattern is used to manipulate the model:
Blog.find(id).done(err, blog) { // blog is the database record with the specified id console.log(blog.content); }
Views
Views are used to handle the UI of the application. By default, views are handled using EJS, but any other templating library can be used. How to configure views was discussed previously in the Configuration chapter.
Views are defined in the /views
directory and the templates are defined in the /assests/templates
folder.
There are mainly four types of views:
- server-side views
- view partials
- layout views
- client-side views
Server-Side Views
Their job is to display data when a view is requested by the client. Usually the method res.view
corresponds to a client with the appropriate view. But if no controller or action exists for a request, Sails will serve the view in the following fashion: /views/:controller/:action.ejs
.
The Layout View
The Layout can be found in /views/layout.ejs
. It is used to load the application assets such as stylesheets or JavaScript libraries.
Have a look at the specified file:
<!DOCTYPE html> <html> <head> <title><%- title %></title> <!-- Viewport mobile tag for sensible mobile support --> <meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1"> <!-- JavaScript and stylesheets from your public folder are included here --> <%- assets.css() %> <%- assets.js() %> </head> <body> <%- body %> <!-- Templates from your view path are included here --> <%- assets.templateLibrary() %> </body> </html>
The lines assets.css()
and assets.js()
load the CSS and JS assets of our application and the assets.templateLibrary
loads the client templates.
Client-Side Templates
These are defined in the /assets/templates
and are loaded as we saw above.
Routes
We discussed how to configure routes in the Configuration chapter.
There are several conventions that Sails follows when routes are handled:
- if the URL is not specified in the
config/routes.js
the default route for a URL is/:controller/:action/:id
with the obvious meanings for controller and action andid
being the request parameter derived from the URL. - if
:action
is not specified, Sails will redirect to the appropriate action. Out of the box, the same RESTful route conventions are used as in Backbone. - if the requested controller/action do not exist, Sails will behave as so:
- if a view exists, Sails will render that view
- if a view does not exist, but a model exists, Sails will return the JSON form of that model
- if none of the above exist, Sails will respond with a 404
Conclusion
Now I've barely scratched the surface with what Sails can do, but stay tuned, as I will follow this up with an in-depth presentation showing you how to build an application, using Sails.
Also keep in mind that Sails is currently under development and constantly changing. So make sure to check out the documentation to see what's new.
Comments