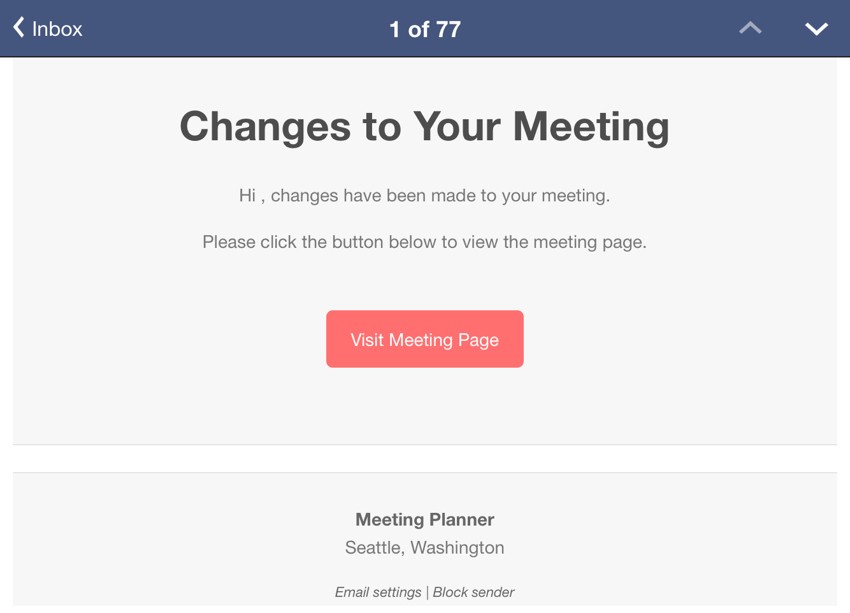
This tutorial is part of the Building Your Startup With PHP series on Envato Tuts+. In this series, I'm guiding you through launching a startup from concept to reality using my Meeting Planner app as a real-life example. Every step along the way, I'll release the Meeting Planner code as open-source examples you can learn from. I'll also address startup-related business issues as they arise.
In this two-part series, I'll describe how we built the notifications infrastructure and their delivery. Today, I'm going to focus on the MeetingLog to track changes that help us determine when to send updates.
If you haven't tried out Meeting Planner yet, go ahead and schedule your first meeting. I do participate in the comment threads below, so tell me what you think! I'm especially interested if you want to suggest new features or topics for future tutorials.
As a reminder, all of the code for Meeting Planner is written in the Yii2 Framework for PHP. If you'd like to learn more about Yii2, check out our parallel series Programming With Yii2.
My Vision for Notifications
Here's what a meeting participant typically sees when they receive an invitation. They'll begin sharing their availability for places and times and sometimes choosing the final selections:
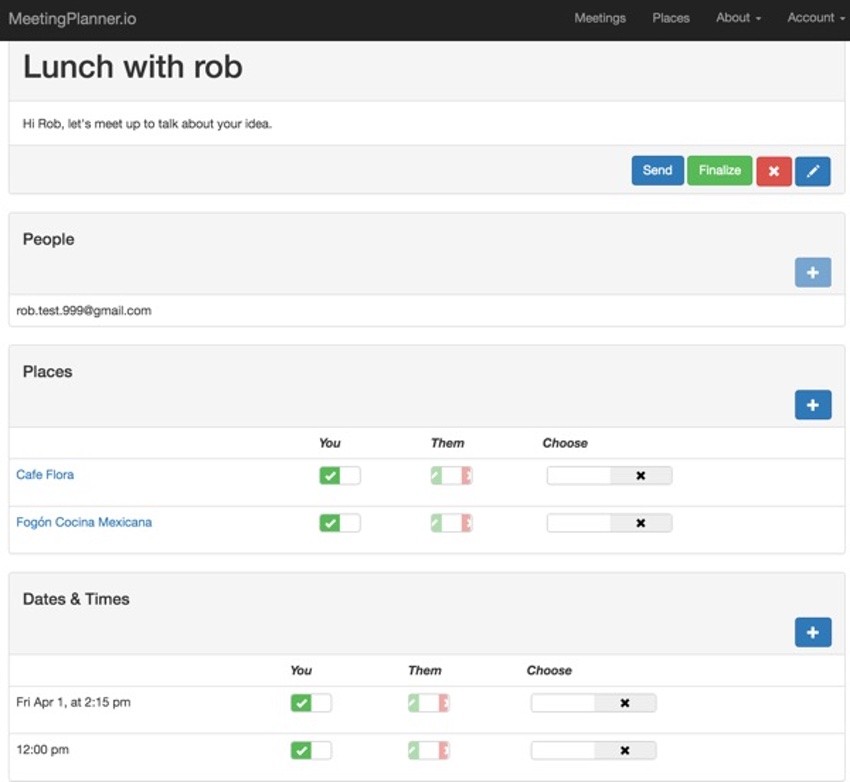
When a meeting participant responds to an invitation like the one above, they may make a handful of changes. For example:
- This date and time works, these two don't.
- This place works, this one doesn't, let's also consider this additional one.
- I choose this place and this time.
- Add a note: "I'm glad we're finally getting together."
After the changes are made, we need to inform the organizer.
Our goal is to simplify meeting planning and reduce the number of emails involved. If we wait a few minutes after the person makes changes and consolidate the changes into a single update, then that's likely a good time to deliver the notification. But how do we build code that does that? How do we keep track of what changes we're made and spell this out for the organizer?
Another idea I had is to eliminate the need to click submit when you're done making changes. Firstly, this would require some user education to assure them we'll send their changes when they're done. But we also needed a way to know when it was okay to deliver the changes to the organizer.
How Notifications Will Work
Restating and summarizing the requirements, here's how notifications will work:
All actions related to meetings are kept in our MeetingLog model. But we'll need a way to create a textual summary of the log to share with participants. We may want to just show the changes that occurred since the last update. So we'll have to track that.
As the person makes changes to the meeting, we'll visually indicate that there's no need to click a non-existent submit or save changes button.
We'll want to monitor for when changes are a few minutes old and consolidate them into a single update to the other participant or organizer.
And finally, we'll need to deliver the email update just to the other participant(s), not the one that made the changes. Or, if both participants have made changes during this time, we'll have to notify each of only the other's actions.
Building the Meeting Log
From the beginning, I wanted a complete log of actions performed when planning meetings for a number of reasons. Perhaps people will want to see the planning history, or revert to a certain moment—but also, it's helpful for debugging. It also turned out to be required for knowing when to deliver updates about meeting changes.
Let's review the structure behind the MeetingLog model. Here's the migration creating the table:
class m141025_220133_create_meeting_log_table extends Migration { public function up() { $tableOptions = null; if ($this->db->driverName === 'mysql') { $tableOptions = 'CHARACTER SET utf8 COLLATE utf8_unicode_ci ENGINE=InnoDB'; } $this->createTable('{{%meeting_log}}', [ 'id' => Schema::TYPE_PK, 'meeting_id' => Schema::TYPE_INTEGER.' NOT NULL', 'action' => Schema::TYPE_INTEGER.' NOT NULL', 'actor_id' => Schema::TYPE_BIGINT.' NOT NULL', 'item_id' => Schema::TYPE_INTEGER.' NOT NULL', 'extra_id' => Schema::TYPE_INTEGER.' NOT NULL', 'created_at' => Schema::TYPE_INTEGER . ' NOT NULL', 'updated_at' => Schema::TYPE_INTEGER . ' NOT NULL', ], $tableOptions); $this->addForeignKey('fk_meeting_log_meeting', '{{%meeting_log}}', 'meeting_id', '{{%meeting}}', 'id', 'CASCADE', 'CASCADE'); $this->addForeignKey('fk_meeting_log_actor', '{{%meeting_log}}', 'actor_id', '{{%user}}', 'id', 'CASCADE', 'CASCADE'); }
The essential elements we're recording are:
- The
meeting_id
tells us which meeting we're tracking. - The
action
tells us what was done. - The
actor_id
tells us who performed the action (e.g.user_id
). - The overloaded
item_id
may represent a time, a place a note. - The
extra_id
is for recording other information depending on the action. -
created_at
tells us when the action was performed.
Then, I defined constants for all of the actions such as ACTION_SUGGEST_PLACE
or ACTION_ADD_NOTE
, etc.
class MeetingLog extends \yii\db\ActiveRecord { const ACTION_CREATE_MEETING = 0; const ACTION_EDIT_MEETING = 3; const ACTION_CANCEL_MEETING = 7; const ACTION_DELETE_MEETING = 8; const ACTION_DECLINE_MEETING = 9; const ACTION_SUGGEST_PLACE = 10; const ACTION_ACCEPT_ALL_PLACES = 11; const ACTION_ACCEPT_PLACE = 12; const ACTION_REJECT_PLACE = 15; const ACTION_SUGGEST_TIME = 20; const ACTION_ACCEPT_ALL_TIMES = 21; const ACTION_ACCEPT_TIME = 22; const ACTION_REJECT_TIME = 25; const ACTION_INVITE_PARTICIPANT = 30; const ACTION_ADD_NOTE = 40; const ACTION_SEND_INVITE = 50; const ACTION_FINALIZE_INVITE = 60; const ACTION_COMPLETE_MEETING = 100; const ACTION_CHOOSE_PLACE = 110; const ACTION_CHOOSE_TIME = 120; const ACTION_SENT_CONTACT_REQUEST = 150; const TIMELAPSE = 300; // five minutes
The add method makes it easy for functionality everywhere within the application to record activity to MeetingLog:
// add to log public static function add($meeting_id,$action,$actor_id=0,$item_id=0,$extra_id=0) { $log = new MeetingLog; $log->meeting_id=$meeting_id; $log->action =$action; $log->actor_id =$actor_id; $log->item_id =$item_id; $log->extra_id =$extra_id; $log->save(); // sets the touched_at field for the Meeting Meeting::touchLog($meeting_id); }
I also added two new fields to the Meeting table: logged_at
and cleared_at
. Whenever log entries are added, the Meeting updates the logged_at
timestamp, showing the moment the meeting was last modified:
public static function touchLog($id) { $mtg = Meeting::findOne($id); $mtg->logged_at = time(); $mtg->update(); }
For example, whenever someone adds a new MeetingPlace option, an afterSave
event adds to the log, and then of course Meeting->logged_at
is also updated:
public function afterSave($insert,$changedAttributes) { parent::afterSave($insert,$changedAttributes); if ($insert) { // if MeetingPlace is added // add MeetingPlaceChoice for owner and participants $mpc = new MeetingPlaceChoice; $mpc->addForNewMeetingPlace($this->meeting_id,$this->suggested_by,$this->id); MeetingLog::add($this->meeting_id,MeetingLog::ACTION_SUGGEST_PLACE,$this->suggested_by,$this->place_id); } }
The logged_at
time tells us when the last change occurred. The Meeting->cleared_at
time will tell us the time that we last shared updates with participants. So, if logged_at
> cleared_at
, we know participants aren't fully updated.
Creating an English Language Summary
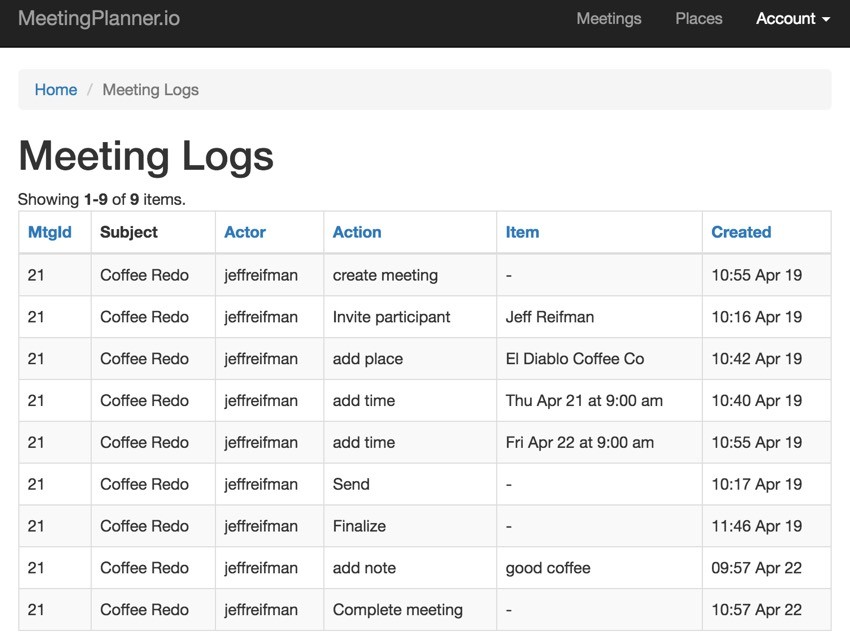
Next, I built methods to help translate the log into an English language history of changes to the meeting.
First, I created getMeetingLogCommand() to get a textual description of the action:
public function getMeetingLogCommand() { switch ($this->action) { case MeetingLog::ACTION_CREATE_MEETING: $label = Yii::t('frontend','create meeting'); break; case MeetingLog::ACTION_EDIT_MEETING: $label = Yii::t('frontend','edit meeting'); break; case MeetingLog::ACTION_CANCEL_MEETING: $label = Yii::t('frontend','cancel meeting'); break; case MeetingLog::ACTION_DELETE_MEETING: $label = Yii::t('frontend','cancel meeting'); break; case MeetingLog::ACTION_DELETE_MEETING: $label = Yii::t('frontend','deleted meeting'); break; case MeetingLog::ACTION_SUGGEST_PLACE: $label = Yii::t('frontend','add place'); break; case MeetingLog::ACTION_SUGGEST_TIME: $label = Yii::t('frontend','add time'); break; case MeetingLog::ACTION_ADD_NOTE: $label = Yii::t('frontend','add note'); break; case MeetingLog::ACTION_INVITE_PARTICIPANT: $label = Yii::t('frontend','Invite participant'); break; case MeetingLog::ACTION_ACCEPT_ALL_PLACES: $label = Yii::t('frontend','accept all places'); break; case MeetingLog::ACTION_ACCEPT_PLACE: $label = Yii::t('frontend','accept place'); break; case MeetingLog::ACTION_REJECT_PLACE: $label = Yii::t('frontend','reject place'); break; case MeetingLog::ACTION_ACCEPT_ALL_TIMES: $label = Yii::t('frontend','accept all times'); break; case MeetingLog::ACTION_ACCEPT_TIME: $label = Yii::t('frontend','accept time'); break; case MeetingLog::ACTION_REJECT_TIME: $label = Yii::t('frontend','reject time'); break; case MeetingLog::ACTION_CHOOSE_PLACE: $label = Yii::t('frontend','choose place'); break; case MeetingLog::ACTION_CHOOSE_TIME: $label = Yii::t('frontend','choose time'); break; case MeetingLog::ACTION_SEND_INVITE: $label = Yii::t('frontend','Send'); break; case MeetingLog::ACTION_FINALIZE_INVITE: $label = Yii::t('frontend','Finalize'); break; case MeetingLog::ACTION_COMPLETE_MEETING: $label = Yii::t('frontend','Complete meeting'); break; case MeetingLog::ACTION_SENT_CONTACT_REQUEST: $label = Yii::t('frontend','Send request for contact information'); default: $label = Yii::t('frontend','Unknown'); break; } return $label; }
Then, I created getMeetingLogItem(), which contextually finds the appropriate object label based on which action was performed. There are still some debug notes to myself in here:
public function getMeetingLogItem() { $label=''; switch ($this->action) { case MeetingLog::ACTION_CREATE_MEETING: case MeetingLog::ACTION_EDIT_MEETING: case MeetingLog::ACTION_CANCEL_MEETING: case MeetingLog::ACTION_DECLINE_MEETING: $label = Yii::t('frontend','-'); break; case MeetingLog::ACTION_INVITE_PARTICIPANT: $label = MiscHelpers::getDisplayName($this->item_id); if (is_null($label)) { $label = 'Error - unknown user'; } break; case MeetingLog::ACTION_SUGGEST_PLACE: $label = Place::find()->where(['id'=>$this->item_id])->one(); if (is_null($label)) { $label = 'Error - suggested unknown place'; } else { $label = $label->name; if (is_null($label)) { $label = 'Error - suggested place has unknown name'; } } break; case MeetingLog::ACTION_ACCEPT_PLACE: case MeetingLog::ACTION_REJECT_PLACE: $label = MeetingPlace::find()->where(['id'=>$this->item_id])->one(); if (is_null($label)) { $label = 'Error - Accept or reject unknown place x1'; } else { if (is_null($label->place)) $label = 'Error Accept or reject unknown place x2'; else { $label = $label->place->name; if (is_null($label)) { $label = 'Error accept or reject unknown place name x3'; } } } break; case MeetingLog::ACTION_CHOOSE_PLACE: $label = MeetingPlace::find()->where(['id'=>$this->item_id])->one(); if (is_null($label)) { $label = 'Error - chose unknown place x1'; } else { if (is_null($label->place)) $label = 'Error chose unknown place x2'; else { $label = $label->place->name; if (is_null($label)) { $label = 'Error - choose unknown place name x3'; } } } break; case MeetingLog::ACTION_CHOOSE_TIME: case MeetingLog::ACTION_SUGGEST_TIME: case MeetingLog::ACTION_ACCEPT_TIME: case MeetingLog::ACTION_REJECT_TIME: // get the start time $mt = MeetingTime::find()->where(['id'=>$this->item_id])->one(); if (is_null($mt)) { $label = 'Error meeting time unknown'; } else { $label = Meeting::friendlyDateFromTimestamp($mt->start); } break; case MeetingLog::ACTION_ADD_NOTE: if ($this->item_id ==0) { $label = 'note not logged'; } else { $label = MeetingNote::find()->where(['id'=>$this->item_id])->one()->note; } break; case MeetingLog::ACTION_ACCEPT_ALL_PLACES: case MeetingLog::ACTION_ACCEPT_ALL_TIMES: case MeetingLog::ACTION_SEND_INVITE: case MeetingLog::ACTION_FINALIZE_INVITE: case MeetingLog::ACTION_COMPLETE_MEETING: case MeetingLog::ACTION_SENT_CONTACT_REQUEST: $label = Yii::t('frontend','-'); break; default: $label = Yii::t('frontend','n/a'); break; } return $label; }
For example, create, edit, cancel, delete meeting does not need item information, whereas accept time requires a specific item_id
corresponding to a MeetingTimeChoice
date and time.
Providing Visual Cues
When a participant changes the sliders for places and times to indicate their preferences, or if allowed, makes a final choice, I wanted to indicate for them that their meeting organizer will be notified when they are done. I also wanted this to be unobtrusive, e.g. a popup alert.
Since many of these changes are AJAX related, the message needed to appear near the mouse actions. On the occasion when a change is submitted (such as a new note), the notification can appear at the top of the page.
Yii Flash notices are great, but they only appear at the top of pages. For example, currently, adding a note requires a page refresh. People will see the notification hint at the top of the page after posting their note:
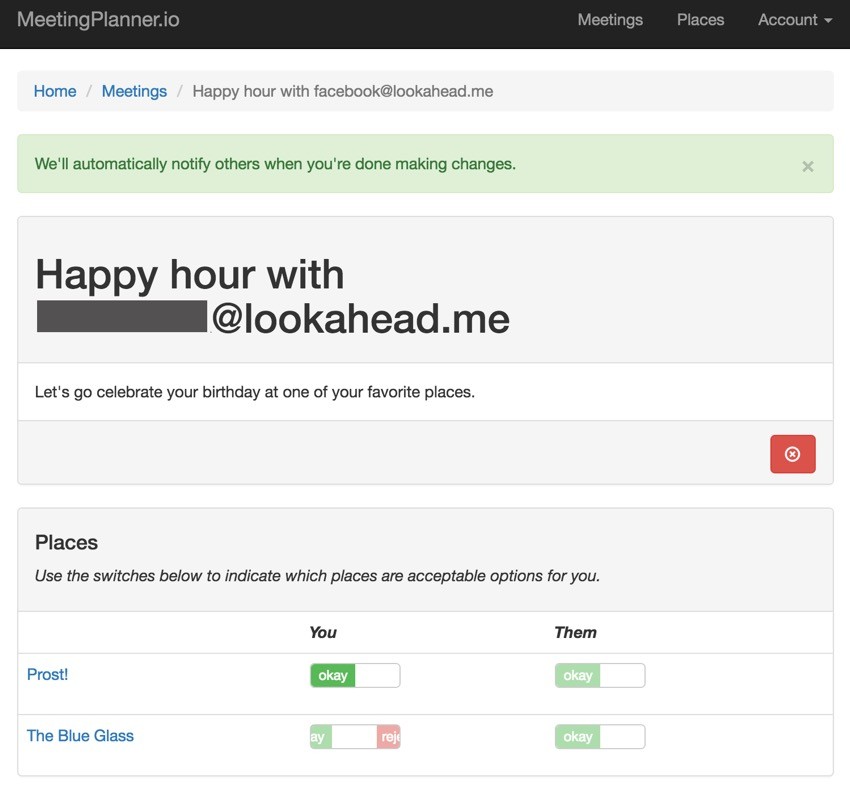
So, for now, while our design remains in flux, I just created hidden notification flash elements above the areas showing places and above the area showing times. Whenever people make changes, they'll see the notification near their mouse action.
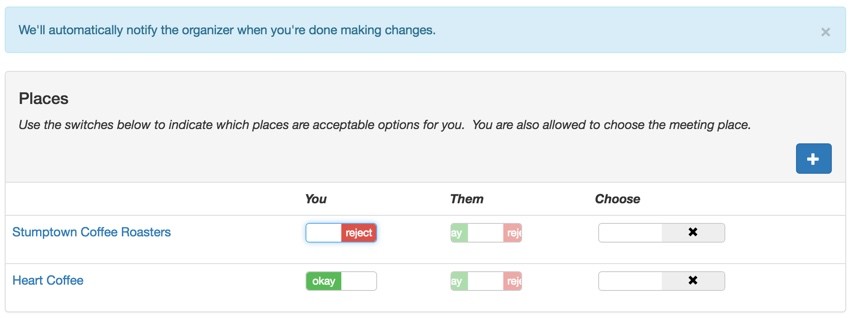
In the future, we won't show these notifications to experienced users as they'll already know.
So, if a person makes a change to a time or place, we call displayNotifier():
// users can say if a time is an option for them $('input[name="meeting-time-choice"]').on('switchChange.bootstrapSwitch', function(e, s) { // set intval to pass via AJAX from boolean state if (s) state = 1; else state =0; $.ajax({ url: '$urlPrefix/meeting-time-choice/set', data: {id: e.target.id, 'state': state}, success: function(data) { displayNotifier('time'); refreshSend(); refreshFinalize(); return true; } }); }); JS;
This code in /frontend/views/meeting/view.php ensures that they don't see the alerts repetitively through a session. It uses session variables to track whether a hint has already been shown:
$session = Yii::$app->session; if ($session['displayHint']=='on' || $model->status == $model::STATUS_PLANNING ) { $notifierOkay='off'; $session->remove('displayHint'); } else { $notifierOkay='on'; } ?> <input id="notifierOkay" value="<?= $notifierOkay ?>" type="hidden"> <?php $script = <<< JS var notifierOkay; // meeting sent already and no page change session flash if ($('#notifierOkay').val() == 'on') { notifierOkay = true; } else { notifierOkay = false; } function displayNotifier(mode) { if (notifierOkay) { if (mode == 'time') { $('#notifierTime').show(); } else if (mode == 'place') { $('#notifierPlace').show(); } else { alert("We\'ll automatically notify the organizer when you're done making changes."); } notifierOkay=false; } }
What's Next?
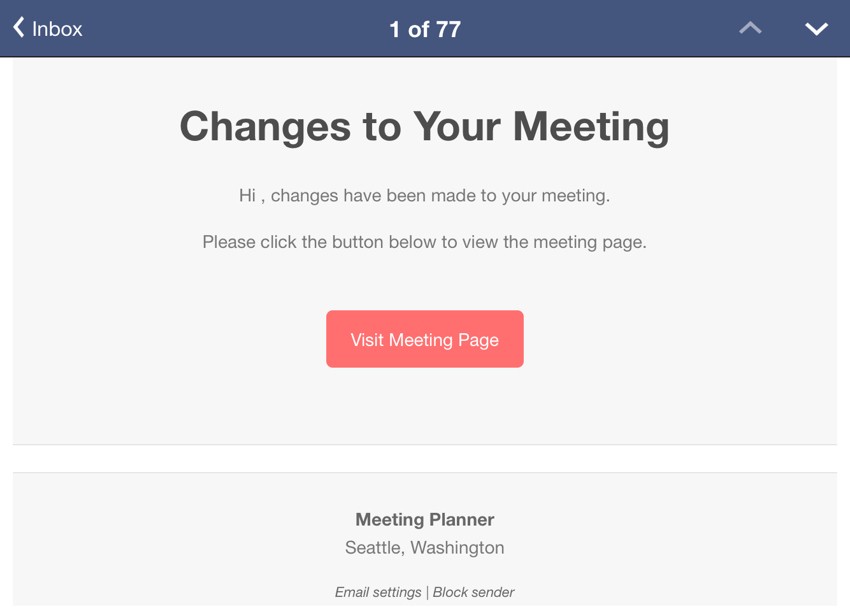
I've described the vision I'm trying to create with planning actions between participants. In the next tutorial, I'll show you how we monitor time to know when and how to deliver updates. And I'll show you how we deliver the updates via email, such as the one above. I'm also going to show you how we create a textual summary of the recent changes that were made.
While you're waiting, try out the notifications feature and schedule your first meeting. Also, I'd appreciate it if you share your experience below in the comments, and I'm always interested in your suggestions. You can also reach me on Twitter @reifman directly.
Watch for upcoming tutorials in the Building Your Startup With PHP series. There are a few more big features coming up.
Comments