The release of the iPhone 5 and iOS 6 has generated a lot of excitement, but the many new hardware and SDK changes can leave iOS developers feeling overwhelmed. This article will help you keep your skills up-to-date by providing a quick, high-level overview of the most important changes for iOS developers!
A Brand New Display
The first thing you're likely to notice about the iPhone 5 is the increased screen size of the retina display. This increase can be measured in both physical screen space and the screen resolution. The iPhone 4S has a 3.5" diagonal screen with 640x960px resolution, whereas the iPhone 5 sports a 4" diagonal screen with 640x1136px resolution.
As a user, you're likely to love the new form-factor. However, as a programmer, you may have cringed a bit when you first learned that you would need to support an additional display size. While the new resolution will undoubtedly create additional work for many of us at some point, the good news is that the iOS 6 SDK comes with a new Auto Layout System to make developing for multiple screen sizes much easier (more on this later).
The following chart will show you how the new iPhone 5 display stacks up against other iOS devices:
Device | Display Size | Resolution | PPI | Aspect Ratio |
iPhone | 3.5" Diagonal | 320x480px | 163 PPI | 3:2 |
iPhone 3G | 3.5" Diagonal | 320x480px | 163 PPI | 3:2 |
iPhone 3GS | 3.5" Diagonal | 320x480px | 163 PPI | 3:2 |
iPhone 4 | 3.5" Diagonal | 640x960px | 326 PPI | 3:2 |
iPhone 4S | 3.5" Diagonal | 640x960px | 326 PPI | 3:2 |
iPhone 5 | 4" Diagonal | 640x1136px | 326 PPI | 16:9 |
iPad | 9.7" Diagonal | 768x1024px | 132 PPI | 4:3 |
iPad (2nd Gen.) | 9.7" Diagonal | 768x1024px | 132 PPI | 4:3 |
iPad (3rd Gen.) | 9.7" Diagonal | 1536x2048px | 264 PPI | 4:3 |
Fortunately, you don't have to manage this complexity completely on your own. In 2011, Apple introduced the Auto Layout System to Cocoa, and with iOS 6 it has now come to Cocoa-Touch as well. While it hasn't received the same fanfare as other iOS 6 features, Auto Layout is a powerful new enhancement that will allow you to quickly create intelligent, fluid layouts using a system of constraints. An Auto Layout is the opposite of a hard-coded layout, where interface objects are placed at specific X,Y coordinates. Instead, Auto Layout allows programmers to describe the relationships between objects in almost mathematical terms, and then iOS simply takes care of the rest and adjusts object positioning as needed. Refer to the WWDC 2012 Introduction to Auto Layout video for more detail.
If you already have an iPhone application in the store, one step you need to urgently take is to adjust your app to use the full iPhone 5 screen. If you haven't already done so, by default your app will appear on iPhone 5 devices with additional black space at the top and bottom of the screen. The following screenshot demonstrates how this will look with screenshots taken from an iPhone 5:
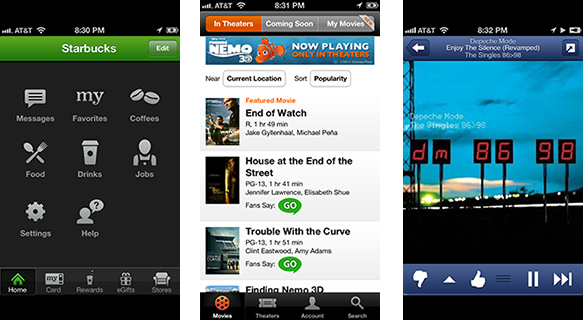
The Fandango app above makes full use of the iPhone 5 display, while the Starbucks and Pandora apps currently do not.
You must update your existing apps to fill the full iPhone 5 screen.
For the majority of iOS applications built with UIKit components and using proper autoresizing masks, the process of making your app fill the entire iPhone 5 screen is as simple as adding a 4-inch launch screen image to your project. For more details, refer to this post on StackOverflow.
Better, Faster, Stronger: The A6 Chip
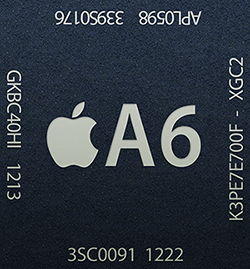
Many people are quick to overlook this point, but the Apple A6 chip built-in to the iPhone 5 is up to twice as fast as the previous generation. As programmers, our applications are ultimately limited by the hardware on which they run. This significant boost in performance will allow developers to create more complex applications, including more advanced Augmented Reality apps and more realistic 3D games. The best part is that despite the performance jump, the battery life of the device has improved as well!
The following table shows how the last three generations of iPhone systems-on-a-chip (SoCs) compare:
Release | SoC | Max Clock Rate | CPU Cores | GPU Cores | L1 Cache | L2 Cache |
iPhone 4 | Apple A4 | 1 GHz | 1 | 1 | 32/32 kB | 512 kB |
iPhone 4S | Apple A5 | 800 MHz to 1 GHz | 2 | 2 | 32/32 kB | 1024 kB |
iPhone 5 | Apple A6 | 1.25 GHz | 2 | 3 | 32/32 kB | 1024 kB |
New Cocoa-Touch Frameworks
Four new frameworks have been added to the iOS 6 SDK.
The Pass Kit Framework
The Passbook app has been one of the most discussed features of iOS 6. Passbook allows users to purchase and store the following four types of documents on their device:
- Boarding Passes
- Event Tickets
- Retail Coupons
- Store Cards
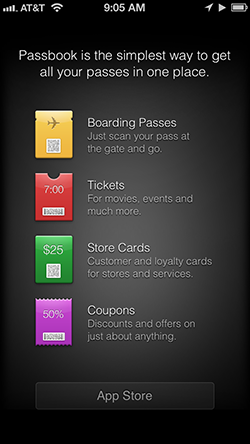
As described in the official Apple docs, the Passbook application can be deconstructed into the following three parts:
- A package format for creating passes.
- A web service API for updating passes, implemented on your server.
- An Objective-C API used by your apps to interact with the user’s pass library.
-The Passbook Programming Guide
As you can see, implementing passbook in your own applications involves integrating technologies across multiple layers. Pass Kit is responsible for the third item in the list above, providing an Objective-C API that can interact with the user's pass library.
While it may take some time to get past the initial Passbook learning curve, at the time of writing (9/23/12), the App Store only has about a dozen apps with Passbook support. This means that developers who work quickly may be able to help their applications stand out in the store by becoming an early Passbook adopter.
For more information on Passbook, be sure to refer to the getting started guide from Apple and, of course, the official documentation.
The Social Framework
When iOS 5 was released, Apple made it much easier for developers to add Twitter support into their applications via the Twitter Framework. iOS 6 takes a new approach to social network integration with the Social Framework. The idea is very similar to the ShareKit open-source project, in that user's are presented with a menu containing multiple sharing options and are free to select the one they want to use. However, what really makes the Social Framework stand out is that it integrates with the Account Framework in an effort to implement Single Sign-On (SSO) and eliminate the repetitiveness of logging into social networks multiple times across many different apps.
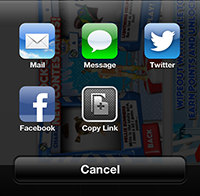
While I'm excited to make the switch to the Social Framework, for now I'll likely be sticking with ShareKit 2.0 as a matter of pragmatism. However, within another year or two, I suspect the Social Framework will supplant the need for many of us to resort to third party libraries for this functionality.
The Ad Support & Media Toolbox Frameworks
Two new frameworks that have gone unnoticed by many are the Ad Support Framework and the Media Toolbox Framework. The Ad Support framework will likely become standard among many third-party advertising partners as it allows the developer to send a unique, user identifier for ad placement. It also has a flag for opting-out of ad tracking, and developers are required to check this flag at run-time to honor the user's ad settings.
The Media Toolbox Framework is a low-level addition that adds new audio support to the AVFoundation framework, and a complete explanation can be found in the WWDC 2012 session 517 video.
Cocoa-Touch Enhancements & Revisions
As with all new OS releases, many new changes, additions, and deprecations have been introduced with iOS 6. Some of the changes that stood out the most to me are highlighted in this section.
UITableView Enhancements
Many new changes have been introduced to the way UITableView
works. To begin with, the UITableViewCell
method dequeueReusableCellWithIdentifier
has now been replaced with two new UITableView
methods, dequeueReusableCellWithIdentifier:forIndexPath:
and registerClass:forCellReuseIdentifier:
. This was done in an effort to streamline the way UITableViewCell
objects are created and supplied to the table view.
In addition, a new class called UITableViewHeaderFooterView
has been introduced for easily creating custom UITableView
headers and footers. The contentView
and backgroundView
properties of this class allow complete customization, while the textLabel
and detailTextLabel
are useful shortcuts for creating minimally customized header or footer views.
Another notable enhancement to table views is the UIRefreshControl
. This control allows you to easily add pull-to-refresh functionality to your table views.
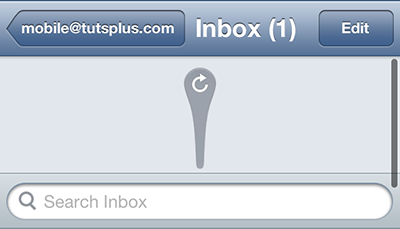
New UIKit Theming Options
iOS 5 introduced a host of new customizations for the most popular UIKit controls, and iOS 6 takes this trend a step further by providing even more options for interface customization. For example, UIPopoverBackgroundView
, UIStepper
, UISwitch
, UINavigationBar
, UITabBar
, UIToolBar
, UIBarButtonItem
, and UIPageControl
have all received additional methods or properties related to interface control.
As mentioned above, UITableView
objects have also become easier to theme via a custom header/footer view class. Table views also received two new interface properties specific to theming: sectionIndexColor
and sectionIndexTrackingBackgroundColor
. These properties allow greater control of table view index appearance.
Grid Based Layouts with UICollectionView
The UICollectionView
class brings the ability to natively create grid-based layouts to the iOS SDK. This is an incredibly useful class, especially on the iPad where displaying rows and columns of views is common.
The iPad version of the Kindle and Reeder apps are great examples of grid based layouts that would make good candidates for implementation with UICollectionView
:
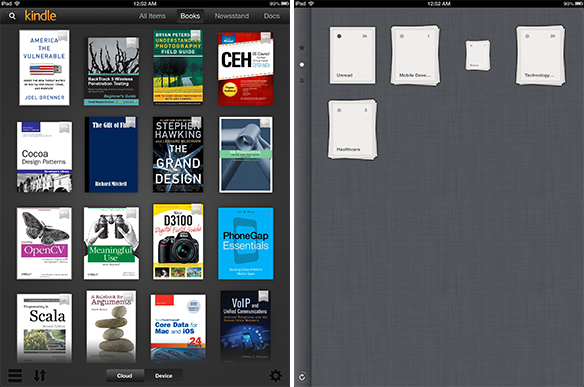
While the above apps were not made with UICollectionView, they represent the general type of layouts this control will allow developers to more easily create.
Stylized Text with NSAttributedString
Anyone who has worked extensively with the SDK has probably had to deal with the aesthetic limitations of UILabel
and NSString
. Displaying an otherwise simple string with a few aesthetic tweaks such as bold text, a hyperlink underline, and substring italics was notoriously cumbersome, and many resorted to using either multiple UILabel
instances or HTML and CSS within a UIWebView
. iOS 6 makes this much easier with the introduction of NSAttributedString
and NSMutableAttributedString
. These new classes are just like their NSString
counterparts, with the notable exception that any given NSAttributedString
may have a number of advanced attributes assigned such as underline, background color, strikethrough, etc. After creating a new attributed string, you can then assign the object to any class offering attributed string support, including UILabel
, UIButton
, or UITableViewCell
.
Auto-Rotation Issues
The iOS 6 SDK takes a new approach to handling view auto-rotation. The shouldAutorotateToInterfaceOrientation
method that we've all grown accustomed to has been deprecated in favor of a new paradigm that uses supportedInterfaceOrientationsForWindow:
and a number of shouldAutorotate
methods.
Further, applications that are setup as landscape orientation only but call a portrait-only view controller (i.e. Game Center login screen, UIPickerViewController
) will actually cause the app to crash.
Calling a portrait-only view controller from within a landscape-only orientation will crash your application.
For more information on this topic, be sure to read the release notes for iOS 6 under the UIKit heading and consider watching the WWDC 2012 session 240 video entitled "Polishing Your Interface Rotations".
New Mobile Safari Features
As one might expect, Mobile Safari took another step forward in HTML5 and associated feature support with the latest release. While a comprehensive review of the changes is beyond the scope of this article, a few of the highlights include:
- Camera access with the Media Capture API and the File API
- A local-cache increase from 5MB to 25MB
- A New Web Audio API
- Additional CSS3 Support (cross-fade, filters, etc.)
- Built-in support for images of different resolutions
- A remote web inspector for simplified debugging
- Performance enhancements to the WebKit JavaScript engine
Apart from the above, there is one major issue worth noting. If you currently have any apps that incorporate a UIWebView
with embedded YouTube content, that code will no longer work. Apple removed the YouTube app from iOS 6, and with it went the default playback support web views were previously able to offer. I suspect this will be a major issue for many developers with apps that depended on this functionality, and I'm certainly disappointed to see this feature removed.
YouTube videos embedded within a UIWebView will no longer play.
Want to learn more? Max Firtman released a great breakdown of all of the above and more. We'll be incorporating many of these new features and changes into future Mobiletuts+ tutorials as well.
A New UI for the App Store
This point hasn't received much publicity yet, but app developers should be aware of sweeping changes in the App Store UI introduced with iOS 6. Searching for new games? Take a look at the difference between the search results for the term "RPG" on iOS 5/iPhone 4S and iOS 6/iPhone 5:
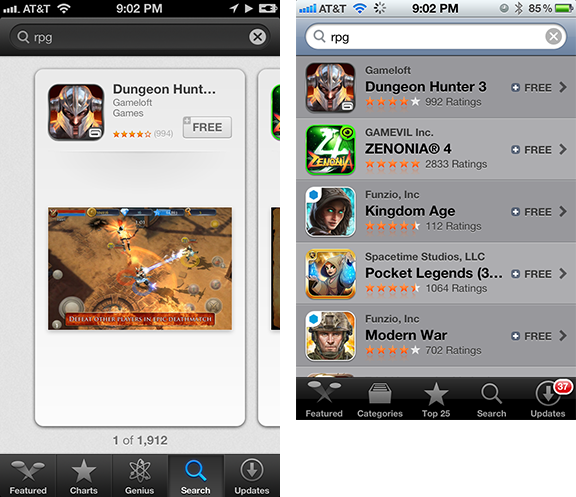
With the old interface, you could easily see 5 different apps at any time and swipe through 25 different apps within just a few seconds. The new interface only displays one result per screen, and forces the user to perform a unique screen swipe to flip through each app.
While ultimately this design decision is a matter of preference, I think it's fair to assume that these App Store changes will mean that users are exposed to far fewer applications overall. It simply takes too long to flip through them one-by-one. The take away for developers is that you're going to need to try harder than ever to get noticed, and you absolutely must make that first screenshot count!
The new App Store UI emphasizes the first screenshot. Make it count!
As much as I'm personally disappointed by the new App Store interface experience as a whole, there is one outstanding feature that has the potential to assist developers. Thanks to the Social Framework, users are now apple to "Share" any of the apps they find within the store:
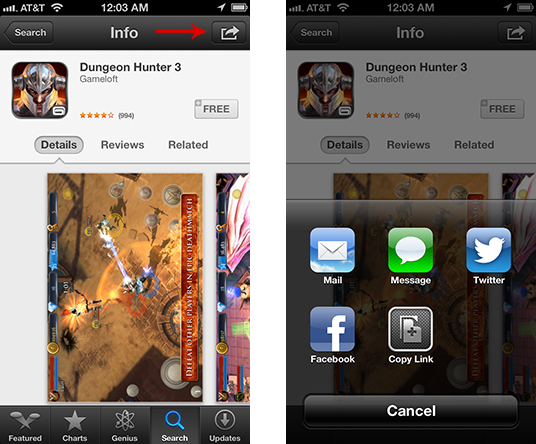
I'm sure most developers will still choose to include the share button within their own applications as well, but the additional exposure here can only help indie developers get the word out about their releases.
Where to Learn More
iOS 6 introduced far more changes than I can cover in any one article or tutorial. This article simply highlights the issues that stood out to me the most as an iOS developer. All future iOS tutorials published on Mobiletuts+ will be compatible with both the iPhone 5 and iOS 6. We'll also show you how to make your own applications continue to run on devices with iOS 5 installed as well. We've got some great content lined up, so stick around!
In the meantime, the best source of information on iOS 6 remains the WWDC 2012 session videos and the official Apple documentation. You should also read the official What's New in iOS 6 post and the official iOS 6 release notes. Be sure to also look over the iOS 6 Checklist and the API Diffs as well.
And one more thing. . .if you haven't already watched the Apple introduction to the iPhone 5, you should probably do that right now:
Wrap Up
Are you planning on building apps that leverage the power of the iPhone 5? Let me know in the comments section below. Feel free to leave any other feedback you might have on this post as well. As always, you can also send questions or comments my way via @markhammonds on Twitter or my newly minted Google+ profile.
Comments