One of the core feature provided by WordPress in extending its functionality is its Meta Box API. These meta boxes enable you to easily add additional data to your content. For example, the Post Tags meta box enables you set tags for your post.
In this article, we will build a basic SEO plugin that adds a meta description, and an Open Graph title and description tag to the head
element of WordPress pages. In doing this, we'll also learn how to create a custom meta boxes, how to sanitize user-provided data, how to save the data to a post or page, and how to and retrieve the saved data.
Because I will not be explaining each and every bit of what the codes use in this tutorial does, a basic knowledge of meta boxes and what the PHP functions does is assumed. If You're not familiar with the basics, then a great start in How to Create Custom WordPress Write/Meta Boxes.
Creating the Meta Box
First, we need to decide decide where the meta box should appear.
In our plugin, the meta box will be added in the post
and page
screen. To achieve this, a function is created containing a variable that stores an array of where to show the meta box and a foreach
loop that loop through the array and add the meta box to the given screen using the add_meta_box
function.
And finally, the function is hooked to the add_meta_boxes
action.
function tes_mb_create() { /** * @array $screens Write screen on which to show the meta box * @values post, page, dashboard, link, attachment, custom_post_type */ $screens = array( 'post', 'page' ); foreach ( $screens as $screen ) { add_meta_box( 'tes-meta', 'Search Engine Listing', 'tes_mb_function', $screen, 'normal', 'high' ); } } add_action( 'add_meta_boxes', 'tes_mb_create' );
Alternatively, you could add a double add_meta_box
function to include the meta box at both the post and page screen like so:
function tes_mb_create() { add_meta_box( 'tes-meta', 'Search Engine Listing', 'tes_mb_function', 'post', 'normal', 'high' ); add_meta_box( 'tes-meta', 'Search Engine Listing', 'tes_mb_function', 'page', 'normal', 'high' ); } add_action('add_meta_boxes', 'tes_mb_create');
Coding the Meta Box Fields
From the code above, the callback function to print out the HTML for the edit screen section is referenced as tes_mb_function
which is the third argument passed to add_meta_box
function.
In our plugin, we are only coding two HTML form field to handle the Title and Description data.
function tes_mb_function($post) { / /retrieve the metadata values if they exist $tes_meta_title = get_post_meta( $post->ID, '_tes_meta_title', true ); $tes_meta_description = get_post_meta( $post->ID, '_tes_meta_description', true ); // Add an nonce field so we can check for it later when validating wp_nonce_field( 'tes_inner_custom_box', 'tes_inner_custom_box_nonce' ); echo '<div style="margin: 10px 100px; text-align: center"> <table> <tr> <td><strong>Title Tag:</strong></td><td> <input style="padding: 6px 4px; width: 300px" type="text" name="tes_meta_title" value="' . esc_attr($tes_meta_title) . '" /> </td> </tr> <tr> <td><strong>Meta Description:</strong></td><td> <textarea rows="3" cols="50" name="tes_meta_description">' . esc_attr($tes_meta_description) . '</textarea></td> </tr> </table> </div>'; }
The explanation of the tes_mb_function
code above is as follows:
- Retrieve and store the meta-data values in a variable only if it exists. This is done in order to populate the fields with its values when it present in the database.
- A nonce feed is added so we can check for it later during verification before the data inserted into the form fields are save to the database.
- The HTML form consisting of a text field input element and a text-area for capturing the title and description tag data respectively is echoed / printed.
At this point, you should be seeing the meta box in the post and page screen.
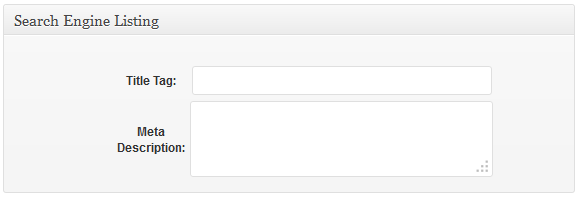
Saving Meta Box Data
A meta box isn't complete until it can save it the data to the database. The name of the function to handle the saving of data will be tes_mb_save_data
. Its code is as follows.
function tes_mb_save_data($post_id) { /* * We need to verify this came from the our screen and with proper authorization, * because save_post can be triggered at other times. */ // Check if our nonce is set. if ( ! isset( $_POST['tes_inner_custom_box_nonce'] ) ) return $post_id; $nonce = $_POST['tes_inner_custom_box_nonce']; // Verify that the nonce is valid. if ( ! wp_verify_nonce( $nonce, 'tes_inner_custom_box' ) ) return $post_id; // If this is an autosave, our form has not been submitted, so we don't want to do anything. if ( defined( 'DOING_AUTOSAVE') && DOING_AUTOSAVE ) return $post_id; // Check the user's permissions. if ( 'page' == $_POST['post_type'] ) { if ( ! current_user_can( 'edit_page', $post_id ) ) return $post_id; } else { if ( ! current_user_can( 'edit_post', $post_id ) ) return $post_id; } /* OK, its safe for us to save the data now. */ // If old entries exist, retrieve them $old_title = get_post_meta( $post_id, '_tes_meta_title', true ); $old_description = get_post_meta( $post_id, '_tes_meta_description', true ); // Sanitize user input. $title = sanitize_text_field( $_POST['tes_meta_title'] ); $description = sanitize_text_field( $_POST['tes_meta_description'] ); // Update the meta field in the database. update_post_meta( $post_id, '_tes_meta_title', $title, $old_title ); update_post_meta( $post_id, '_tes_meta_description', $description, $old_description ); } add_action( 'save_post', 'tes_mb_save_data' );
Let's examine the above code:
- First, we verify that this came from our screen and with proper authorization, because
save_post
can be triggered at other times and also verify that the nonce previously set intes_mb_function
is valid. - Then, if an entry already exists in the database, we'll retrieve it and store in
$old_title
and$old_description
variable. We are doing this because theupdate_post_meta
function that saves the data to the database optionally requires an old value to be check before updating the meta box database row with the new values. - After that, the submitted data gets sanitized using WordPress'
sanitize_text_field
function which convert HTML to its entity, strip all tags, remove line breaks, tabs and extra white space, strip octets. - The meta data is updated to the database via
update_post_meta
. - Finally, the
tes_mb_save_data
is hooked to thesave_post
action to save the meta box data when the post or page is updated.
Making Use of the Saved Data
Don't forget, the saved data is to be used in adding an Open Graph title and description as well as the meta description tag in the head
element of each page.
To do this, we will create a function named tes_mb_display
which will contain the desired tags and afterward, hook it into wp_head
action.
function tes_mb_display() { global $post; // retrieve the metadata values if they exist $tes_meta_title = get_post_meta( $post->ID, '_tes_meta_title', true ); $tes_meta_description = get_post_meta( $post->ID, '_tes_meta_description', true ); echo ' <!-- Tutsplus Easy SEO (author: http://tech4sky.com) --> <meta property="og:title" content="' . $tes_meta_title . '" /> <meta property="og:description" content="' . $tes_meta_description . '" /> <meta name="description" content="' . $tes_meta_description . '" /> <!-- /Tutsplus Easy SEO --> '; } add_action( 'wp_head', 'tes_mb_display' );
- To successfully detect the post ID, we grab a reference to the post using the
$post
object global. - The meta data is then retrieved from the database and saved to the
$tes_meta_title
and$tes_meta_description
variables, respectively. - Next, we defined the meta tag to be inserted into the template
head
element. - Finally, we hook the function to
wp_head
.
If you have added and saved a title and description against a post or page, viewing that page source should reveal the presence of Open Graph title and description tag alongside meta description used by search engines.

Summary
In this article, we created a basic SEO plugin that adds a meta description and Open Graph tags used by search engines social networks to the header section of WordPress.
We learned how to create meta boxes, it form fields, sanitizing the data before saving to the database and retrieving the saved data for use.
An additional assignment to learn more about this process: Extend this plugin and add a meta keyword field to the meta box form and also include it among the tags that are inserted into WordPress header.
Comments