Welcome to part 2 of our 3 part series on user authentication with Titanium Mobile. Titanium is an open source cross compiler that allows you to write iPhone and Android (soon to be blackberry too!) applications using Javascript. No Objective-C required! We will be using PHP as the server side code and my database will be MySQL. For this example, I am using MAMP to develop locally. I strongly recommend that you go through the first part of this series before continuing if you haven't already. However, you can alternatively download the source from part 1, create the database table, and setup the PHP database connections on your own before skipping to this tutorial if you would like.
Synopsis
In part 1, we setup the database for our app and added a user. We then made our login interface by creating a tab group, a tab, and a window. We then gave our login button some actions. Our PHP file queried our database and, upon successful login, it returned our name and email. If login authentication failed, we returned a string simply stating invalid username and/or password. In part 2, we will create a new tab on the main screen that allows a user to create a new account and then login.
Step 1: Creating the Account Window and Tab
Open up app.js and create the account window and tab underneath our login tab script. Also note that I removed the tabBarHidden property on the login window that we did in part 1. Removing that property allows us to see the tabs on the bottom of the phone. We have also added the accountTab to the tabGroup.
Titanium.UI.setBackgroundColor('#fff'); var tabGroup = Titanium.UI.createTabGroup(); var login = Titanium.UI.createWindow({ title:'User Authentication Demo', url:'main_windows/login.js' }); var loginTab = Titanium.UI.createTab({ title:"Login", window:login }); var account = Titanium.UI.createWindow({ title:'New Account', url:'main_windows/account.js' }); var accountTab = Titanium.UI.createTab({ title:'New Account', window:account }); tabGroup.addTab(loginTab); tabGroup.addTab(accountTab); tabGroup.open();
The URL property on the account var tells the compiler to use account.js as our window. If you skip this part, Titanium will throw an ugly red error in the emulator. Upon a successful compile, your screen should look like this:
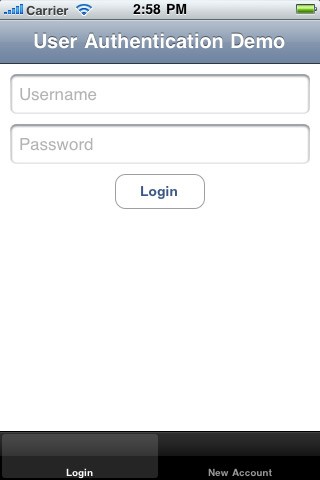
Traditionally, you would see the tab bar on the bottom have icons. Well, with Titanium, that is super easy too! Simply use the icon property in each tab. For example:
var accountTab = Titanium.UI.createTab({ title:'New Account', icon:'images/account_icon.png', window:account });
Step 2: Create account.js
Create a new file and name it account.js and save it in your Resources/main_windows folder. This is the same place we saved our login.js file in part 1.
var win = Titanium.UI.currentWindow; /* * Interface */ var scrollView = Titanium.UI.createScrollView({ contentWidth:'auto', contentHeight:'auto', top:0, showVerticalScrollIndicator:true, showHorizontalScrollIndicator:false }); win.add(scrollView); var username = Titanium.UI.createTextField({ color:'#336699', top:10, left:10, width:300, height:40, hintText:'Username', keyboardType:Titanium.UI.KEYBOARD_DEFAULT, returnKeyType:Titanium.UI.RETURNKEY_DEFAULT, borderStyle:Titanium.UI.INPUT_BORDERSTYLE_ROUNDED }); scrollView.add(username); var password1 = Titanium.UI.createTextField({ color:'#336699', top:60, left:10, width:300, height:40, hintText:'Password', passwordMask:true, keyboardType:Titanium.UI.KEYBOARD_DEFAULT, returnKeyType:Titanium.UI.RETURNKEY_DEFAULT, borderStyle:Titanium.UI.INPUT_BORDERSTYLE_ROUNDED }); scrollView.add(password1); var password2 = Titanium.UI.createTextField({ color:'#336699', top:110, left:10, width:300, height:40, hintText:'Password Again', passwordMask:true, keyboardType:Titanium.UI.KEYBOARD_DEFAULT, returnKeyType:Titanium.UI.RETURNKEY_DEFAULT, borderStyle:Titanium.UI.INPUT_BORDERSTYLE_ROUNDED }); scrollView.add(password2); var names = Titanium.UI.createTextField({ color:'#336699', top:160, left:10, width:300, height:40, hintText:'Name', keyboardType:Titanium.UI.KEYBOARD_DEFAULT, returnKeyType:Titanium.UI.RETURNKEY_DEFAULT, borderStyle:Titanium.UI.INPUT_BORDERSTYLE_ROUNDED }); scrollView.add(names); var email = Titanium.UI.createTextField({ color:'#336699', top:210, left:10, width:300, height:40, hintText:'email', keyboardType:Titanium.UI.KEYBOARD_DEFAULT, returnKeyType:Titanium.UI.RETURNKEY_DEFAULT, borderStyle:Titanium.UI.INPUT_BORDERSTYLE_ROUNDED }); scrollView.add(email); var createBtn = Titanium.UI.createButton({ title:'Create Account', top:260, width:130, height:35, borderRadius:1, font:{fontFamily:'Arial',fontWeight:'bold',fontSize:14} }); scrollView.add(createBtn);
Okay, this mean looking block of code is really super easy to understand, yet it does so much for us. Just by looking at our variable names, it is pretty easy to decipher what is going on here. We created 5 fields:
- Username
- Password1
- Password2
- Name
- Email Address
We also made our 'create account' button.
You will also notice the var at the top called scrollView. Adding the objects to a scroll view allows the view to be scrollable so when the keyboard slides up, it isn't overlapping our text fields.
Go ahead and compile. Upon a successful compile, your screen should look like this after switching to the account tab. The create account button doesn't do anything yet, but play around with selecting text fields and see how the scroll view works.
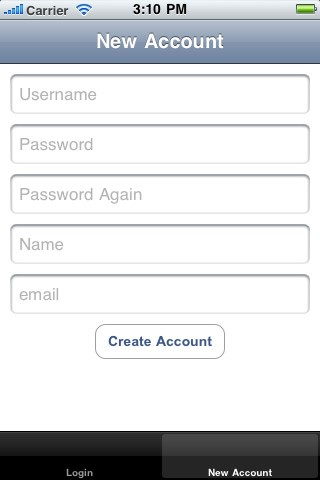
Step 3: Adding the Click Event to Our Button
We now need to create an event listener on our button so when they click 'create account', it sends the data off as well as some validation.
var testresults; function checkemail(emailAddress) { var str = emailAddress; var filter = /^([A-Za-z0-9_\-\.])+\@([A-Za-z0-9_\-\.])+\.([A-Za-z]{2,4})$/; if (filter.test(str)) { testresults = true; } else { testresults = false; } return (testresults); }; var createReq = Titanium.Network.createHTTPClient(); createReq.onload = function() { if (this.responseText == "Insert failed" || this.responseText == "That username or email already exists") { createBtn.enabled = true; createBtn.opacity = 1; alert(this.responseText); } else { var alertDialog = Titanium.UI.createAlertDialog({ title: 'Alert', message: this.responseText, buttonNames: ['OK'] }); alertDialog.show(); alertDialog.addEventListener('click',function(e) { win.tabGroup.setActiveTab(0); }); } }; createBtn.addEventListener('click',function(e) { if (username.value != '' && password1.value != '' && password2.value != '' && names.value != '' && email.value != '') { if (password1.value != password2.value) { alert("Your passwords do not match"); } else { if (!checkemail(email.value)) { alert("Please enter a valid email"); } else { alert("Everything looks good so send the data"); } } } else { alert("All fields are required"); } });
Starting from the top, the checkEmail() method is a simple function that uses Regular Expression to check if the email the user inputs is the correct format. We created a new HTTPClient that will be used to send our data to our PHP file.
In the click event, we first check if any fields are empty. If they are, alert them saying "All fields are required." Our next check is to see if the two password fields are the same. If they aren't, alert them saying "Your passwords do not match." Our final check is to check if the email address is valid. If it isn't, alert them saying "Please enter a valid email."
Once the form is validated, it will alert "Everything looks good so send the data." Go ahead and compile and test submitting the form with no values, non-matching passwords and an invalid email. Upon submitting a valid form, you will see the alert below:
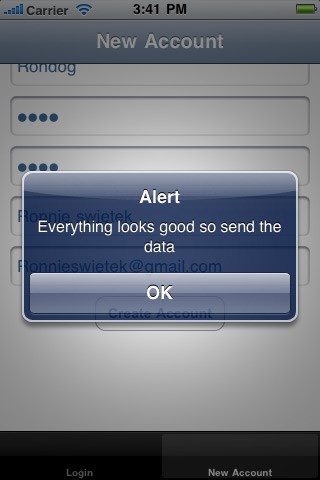
Step 4: Actually Send Some Data
Go ahead and delete the "Everything looks good so send the data" line. We need to replace that with the open() and send() methods.
createBtn.addEventListener('click',function(e) { if (username.value != '' && password1.value != '' && password2.value != '' && names.value != '' && email.value != '') { if (password1.value != password2.value) { alert("Your passwords do not match"); } else { if (!checkemail(email.value)) { alert("Please enter a valid email"); } else { createBtn.enabled = false; createBtn.opacity = 0.3; createReq.open("POST","http://localhost:8888/post_register.php"); var params = { username: username.value, password: Ti.Utils.md5HexDigest(password1.value), names: names.value, email: email.value }; createReq.send(params); } } } else { alert("All fields are required"); } });
So, in replacing that line, we disable our 'create account' button and set the opacity to 30%. We then take the HTTPClient we made and call the open() method on it. It is pointing at a PHP file that we will make in the next step. We then create a params object to contain all the form data. Notice I am running an MD5 encryption on the password field. Final step is to call the send() method and pass it our params object.
Step 5: Creating our Register PHP File
This file will be the PHP file our app talks to when hitting the 'create account' button. The name must reflect the name in our createReq.open() method in the previous step. I've named mine post_register.php. Replace my mysql_connect and mysql_select_db settings with your connection settings.
<?php $con = mysql_connect('localhost','root','root'); if (!$con) { echo "Failed to make connection."; exit; } $db = mysql_select_db('test'); if (!$db) { echo "Failed to select db."; exit; } $username = $_POST['username']; $password = $_POST['password']; $names = $_POST['names']; $email = $_POST['email']; $sql = "SELECT username,email FROM users WHERE username = '" . $username . "' OR email = '" . $email . "'"; $query = mysql_query($sql); if (mysql_num_rows($query) > 0) { echo "That username or email already exists"; } else { $insert = "INSERT INTO users (username,password,name,email) VALUES ('" . $username . "','" . $password . "','" . $names . "','" . $email . "')"; $query = mysql_query($insert); if ($query) { echo "Thanks for registering. You may now login."; } else { echo "Insert failed"; } } ?>
So here we connect to our database and select the database named 'test' (that name will change depending on the name of your database obviously). You can see our $_POST variables reflect the names we set in the params object in our last step. That part is crucial. We then see if the username or email address they entered already exists. If it doesn't, insert the the data into the database. Okay, don't compile just yet! We will next step, I promise.
Step 6: Receiving Data in account.js
Okay back to account.js. Let do some data handling for when our PHP returns something. Place this code under var createReq and above our click event:
createReq.onload = function() { if (this.responseText == "Insert failed" || this.responseText == "That username or email already exists") { win.remove(overlay); alert(this.responseText); } else { var alertDialog = Titanium.UI.createAlertDialog({ title: 'Alert', message: this.responseText, buttonNames: ['OK'] }); alertDialog.show(); alertDialog.addEventListener('click',function(e) { win.tabGroup.setActiveTab(0); }); } };
So when PHP returns something, if 'this.responseText' is equal to "Insert failed" OR "That username or email already exists," remove the overlay window (so they can re-enter information and submit) and alert them with 'this.responseText'.
Upon successful registration, alert them with "Thanks for registering. You may now login" (defined in our post_register.php file). We also add an event listener to the OK button so when clicking it, it automatically takes us to the login screen.
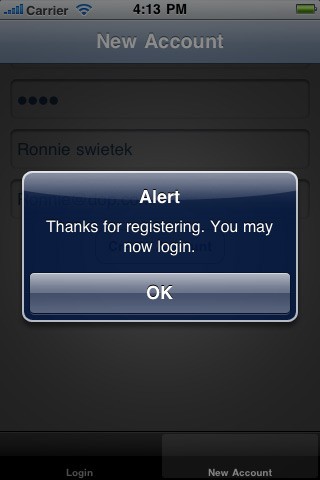
If the alert coming back is some garbled message about mysql_connect and/or access denied, then you need to check your mysql connection settings in the PHP.
Conclusion
In part 2 of this series, we added in tabbed windows that you can switch between. We then made a new form where a user can input data into and submit it. Upon submitting we did some form validation and then had our PHP return a message based on if the data was in use and, if not, we successfully inserted it. I hope you enjoyed reading this mini series tutorial as much as I enjoyed writing it!
Comments