The Android platform provides libraries you can use to stream media files, such as remote videos, presenting them for playback in your apps. In this tutorial, we will stream a video file, displaying it using the VideoView
component together with a MediaController
object to let the user control playback.
We will also briefly run through the process of presenting the video using the MediaPlayer
class. If you've completed the series on creating a music player for Android, you could use what you learn in this tutorial to further enhance it. You should be able to complete this tutorial if you have developed at least a few Android apps already.
Premium Option
If you want a ready-made solution, check out YoVideo, an Android app template for creating a beautiful mobile video player for Android smartphone.
Users can view videos, follow and share with their friends on Facebook. Using this application template will save you money and time in creating a video-sharing application.
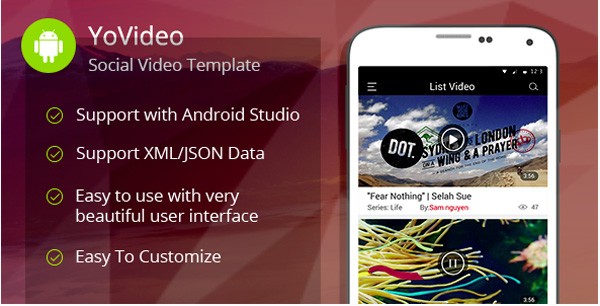
Or you could hire an Android developer to create a custom solution for you. Otherwise, read on for the instructions on how to do it yourself.
1. Create a New App
Step 1
You can use the code in this tutorial to enhance an existing app you are working on or you can create a new app now in Eclipse or Android Studio. Create a new Android project, give it a name of your choice, configure the details, and give it an initial main Activity
class and layout.
Step 2
Let's first configure the project's manifest for streaming media. Open the manifest file of your project and switch to XML editing in your IDE. For streaming media, you need internet access, so add the following permission inside the manifest
element:
<uses-permission android:name="android.permission.INTERNET" />
2. Add VideoView
Step 1
The Android platform provides the VideoView
class in which you can play video files. Let's add one to the main layout file:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:background="#000000" tools:context=".MainActivity" > <VideoView android:id="@+id/myVideo" android:layout_width="fill_parent" android:layout_height="fill_parent" android:layout_centerInParent="true" /> </RelativeLayout>
Alter the parent layout to suit your own app if necessary. We give the VideoView
instance an id
attribute so that we can refer to it later. You may need to adjust the other layout properties for your own design.
Step 2
Now let's retrieve a reference to the VideoView
instance in code. Open your app's main Activity
class and add the following additional imports:
import android.net.Uri; import android.widget.MediaController; import android.widget.VideoView;
Your Activity
class should already contain the onCreate
method in which the content view is set:
@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); }
After the setContentView
line, let's get a reference to the VideoView
instance as follows, using the id
we set in the XML layout:
VideoView vidView = (VideoView)findViewById(R.id.myVideo);
3. Stream a Video File
Step 1
Now we can stream a video file to the app. Prepare the URI for the endpoint as follows:
String vidAddress = "https://archive.org/download/ksnn_compilation_master_the_internet/ksnn_compilation_master_the_internet_512kb.mp4"; Uri vidUri = Uri.parse(vidAddress);
You will of course need to use the remote address for the video file you want to stream. The example here is a public domain video file hosted on the Internet Archive. We parse the address string as a URI so that we can pass it to the VideoView
object:
vidView.setVideoURI(vidUri);
Now you can simply start playback:
vidView.start();
The Android operating system supports a range of video and media formats, with each device often supporting additional formats on top of this.
As you can see in the Developer Guide, video file formats supported include 3GP, MP4, WEBM, and MKV, depending on the format used and on which platform level the user has installed.
Audio file formats you can expect built-in support for include MP3, MID, OGG, and WAV. You can stream media on Android over RTSP, HTTP, and HTTPS (from Android 3.1).
4. Add Playback Controls
Step 1
We've implemented video playback, but the user will expect and be accustomed to having control over it. Again, the Android platform provides resources for handling this using familiar interaction via the MediaController
class.
In your Activity
class's onCreate
method, before the line in which you call start
on the VideoView
, create an instance of the class:
MediaController vidControl = new MediaController(this);
Next, set it to use the VideoView
instance as its anchor:
vidControl.setAnchorView(vidView);
And finally, set it as the media controller for the VideoView
object:
vidView.setMediaController(vidControl);
When you run the app now, the user should be able to control playback of the streaming video, including fast forward and rewind buttons, a play/pause button, and a seek bar control.
The seek bar control is accompanied by the length of the media file on the right and the current playback position on the left. As well as being able to tap along the seek bar to jump to a position in the file, the streaming status is indicated using the same type of display the user will be accustomed to from sites and apps like YouTube.
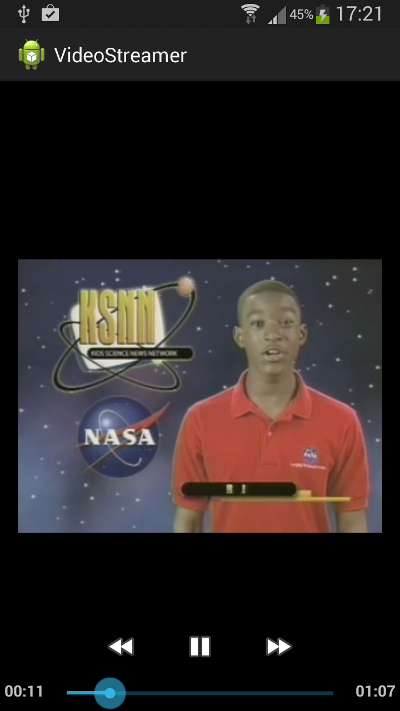
As you will see when you run the app, the default behavior is for the controls to disappear after a few moments, reappearing when the user touches the screen. You can configure the behavior of the MediaController
object in various ways. See the series on creating a music player app for Android for an example of how to do this. You can also enhance media playback by implementing various listeners to configure your app's behavior.
5. Using MediaPlayer
Step 1
Before we finish, let's run through an alternative approach for streaming video using the MediaPlayer
class, since we used it in the series on creating a music player. You can stream media, including video, to a MediaPlayer
object using a surface view. For example, you could use the following layout:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#000000" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity" > <SurfaceView android:id="@+id/surfView" android:layout_width="fill_parent" android:layout_height="fill_parent" /> </RelativeLayout>
We will refer to the SurfaceView
in the implementation of the Activity
class.
Step 2
In your Activity
class, add the following interfaces:
public class MainActivity extends Activity implements SurfaceHolder.Callback, OnPreparedListener
Your IDE should prompt you to add these unimplemented methods:
@Override public void surfaceChanged(SurfaceHolder arg0, int arg1, int arg2, int arg3) { // TODO Auto-generated method stub } @Override public void surfaceCreated(SurfaceHolder arg0) { //setup } @Override public void surfaceDestroyed(SurfaceHolder arg0) { // TODO Auto-generated method stub } @Override public void onPrepared(MediaPlayer mp) { //start playback }
We will add to the surfaceCreated
and onPrepared
methods.
Step 3
To implement playback, add the following instance variables to the class:
private MediaPlayer mediaPlayer; private SurfaceHolder vidHolder; private SurfaceView vidSurface; String vidAddress = "https://archive.org/download/ksnn_compilation_master_the_internet/ksnn_compilation_master_the_internet_512kb.mp4";
In the Activity
's onCreate
method, you can then start to instantiate these variables using the SurfaceView
object you added to the layout:
vidSurface = (SurfaceView) findViewById(R.id.surfView); vidHolder = vidSurface.getHolder(); vidHolder.addCallback(this);
Step 4
In the surfaceCreated
method, set up your media playback resources:
try { mediaPlayer = new MediaPlayer(); mediaPlayer.setDisplay(vidHolder); mediaPlayer.setDataSource(vidAddress); mediaPlayer.prepare(); mediaPlayer.setOnPreparedListener(this); mediaPlayer.setAudioStreamType(AudioManager.STREAM_MUSIC); } catch(Exception e){ e.printStackTrace(); }
Finally, in the onPrepared
method, start playback:
mediaPlayer.start();
Your video should now play in the MediaPlayer
instance when you run the app.
Conclusion
In this tutorial, we have outlined the basics of streaming video on Android using the VideoView
and MediaPlayer
classes. You could add lots of enhancements to the code we implemented here, for example, by building video or streaming media support into the music player app we created. You may also wish to check out associated resources for Android such as the YouTube Android Player API.
Comments