In the last tutorial, we reviewed the original state of our autoloader and then went through a process of object-oriented analysis and design. The purpose of doing this is so that we can tie together everything that we've covered in this series and the introductory series.
Secondly, the purpose of doing this in its own tutorial is so we can spend the rest of this time walking through our class, seeing how each part fits together, implementing it in our plugin, and then seeing how applying object-oriented programming and the single responsibility principle can lead to a more focused, maintainable solution.
Before We Get Started
At this point, I'm assuming that you've been following along with this series. If not, then please review part one and part two. This one assumes you've been following along thus far.
If you're a beginner, I recommend reading the initial series in its entirety, as well. Once you're caught up, you should be in a good position to finish out the series as we conclude with the remainder of the source code covered in this tutorial.
What We've Done Thus Far
To provide a quick summary and to make sure we're all on the same page, we've covered the following topics in this series:
- reviewed the definition of a class interface
- seen how a class implements an interface
- reviewed the single responsibility principle
- analyzed our existing autoloader
- created a roadmap for our object-oriented version of the autoloader
- and designed a basic implementation for an object-oriented version of the autoloader
At this point, we're ready to swap out our existing autoloader with the object-oriented based code. Note, however, that this will not be a simple matter of changing out files.
What We Need to Do
Instead, we're going to need to create the files, make sure they are following the WordPress Coding Standards, implement them, test their implementation to make sure the plugin still works, and then remove the existing autoloader.
It sounds like a lot of work, but if our analysis and design from the previous tutorial was done correctly and proves to be accurate, then we should have little problem doing everything listed above.
Your Development Environment
Before we jump into the implementation, I want to provide a quick rundown of the development environment you should have on your system. Technically, this is something you should already have running as per directions in previous tutorials, but I want to be as complete as possible.
- a local development environment suitable for your operating system
- a directory out of which WordPress 4.6.1 is being hosted
- a text editor or IDE
- knowledge of the WordPress Plugin API
With that said, let's get started.
Object-Oriented Implementation
In this section, we're going to revisit all of the code that we reviewed in the previous tutorial; however, we're going to be looking at each individual file along with complete documentation.
Additionally, we're going to be including it in our project so that by the end of the tutorial, we'll be able to use this code in place of the single, procedural-based code we used earlier.
Note that each of the following files should be named as listed and should be included in the inc
directory. Furthermore, all of this is available for download by using the blue button in the sidebar of this post.
class-autoloader.php
<?php /** * Dynamically loads the class attempting to be instantiated elsewhere in the * plugin. * * @package Tutsplus_Namespace_Demo\Inc */ /** * The primary point of entry for the autoloading functionality. Uses a number of other classes * to work through the process of autoloading classes and interfaces in the project. * * @package Tutsplus_Namespace_Demo\Inc */ class Autoloader { /** * Verifies the file being passed into the autoloader is of the same namespace as the * plugin. * * @var NamespaceValidator */ private $namespace_validator; /** * Uses the fully-qualified file path ultimately returned from the other classes. * * @var FileRegistry */ private $file_registry; /** * Creates an instance of this class by instantiating the NamespaceValidator and the * FileRegistry. */ public function __construct() { $this->namespace_validator = new NamespaceValidator(); $this->file_registry = new FileRegistry(); } /** * Attempts to load the specified filename. * * @param string $filename The path to the file that we're attempting to load. */ public function load( $filename ) { if ( $this->namespace_validator->is_valid( $filename ) ) { $this->file_registry->load( $filename ); } } }
class-namespace-validator.php
<?php /** * Looks at the incoming class or interface determines if it's valid. * * @package Tutsplus_Namespace_Demo\Inc */ /** * Looks at the incoming class or interface determines if it's valid. * * @package Tutsplus_Namespace_Demo\Inc */ class NamespaceValidator { /** * Yields the deciding factor if we can proceed with the rest of our code our not. * * @param string $filename The path to the file that we're attempting to load. * @return bool Whether or not the specified file is in the correct namespace. */ public function is_valid( $filename ) { return ( 0 === strpos( $filename, 'Tutsplus_Namespace_Demo' ) ); } }
class-file-investigator.php
<?php /** * This class looks at the type of file that's being passed into the autoloader. * * @package Tutsplus_Namespace_Demo\Inc */ /** * This class looks at the type of file that's being passed into the autoloader. * * It will determine if it's a class, an interface, or a namespace and return the fully-qualified * path name to the file so that it may be included. * * @package Tutsplus_Namespace_Demo\Inc */ class FileInvestigator { /** * Returns the fully-qualified path to the file based on the incoming filename. * * @param string $filename The incoming filename based on the class or interface name. * @return string The path to the file. */ public function get_filetype( $filename ) { $file_parts = explode( '\\', $filename ); $filepath = ''; $length = count( $file_parts ); for ( $i = 1; $i < $length; $i++ ) { $current = strtolower( $file_parts[ $i ] ); $current = str_ireplace( '_', '-', $current ); $filepath .= $this->get_file_name( $file_parts, $current, $i ); if ( count( $file_parts ) - 1 !== $i ) { $filepath = trailingslashit( $filepath ); } } return $filepath; } /** * Retrieves the location of part of the filename on disk based on the current index of the * array being examined. * * @access private * @param array $file_parts The array of all parts of the file name. * @param string $current The current part of the file to examine. * @param int $i The current index of the array of $file_parts to examine. * @return string The name of the file on disk. */ private function get_file_name( $file_parts, $current, $i ) { $filename = ''; if ( count( $file_parts ) - 1 === $i ) { if ( $this->is_interface( $file_parts ) ) { $filename = $this->get_interface_name( $file_parts ); } else { $filename = $this->get_class_name( $current ); } } else { $filename = $this->get_namespace_name( $current ); } return $filename; } /** * Determines if the specified file being examined is an interface. * * @access private * @param array $file_parts The parts of the filepath to examine. * @return bool True if interface is contained in the filename; otherwise, false. */ private function is_interface( $file_parts ) { return strpos( strtolower( $file_parts[ count( $file_parts ) - 1 ] ), 'interface' ); } /** * Retrieves the filename of the interface based on the specified parts of the file passed * into the autoloader. * * @access private * @param array $file_parts The array of parts of the file to examine. * @return string The filename of the interface. */ private function get_interface_name( $file_parts ) { $interface_name = explode( '_', $file_parts[ count( $file_parts ) - 1 ] ); $interface_name = $interface_name[0]; return "interface-$interface_name.php"; } /** * Generates the name of the class filename on disk. * * @access private * @param string $current The current piece of the file name to examine. * @return string The class filename on disk. */ private function get_class_name( $current ) { return "class-$current.php"; } /** * Creates a mapping of the namespace to the directory structure. * * @access private * @param string $current The current part of the file to examine. * @return string The path of the namespace mapping to the directory structure. */ private function get_namespace_name( $current ) { return '/' . $current; } }
class-file-registry.php
<?php /** * Includes the file from the plugin. * * @package Tutsplus_Namespace_Demo\Inc */ /** * This will use the fully qualified file path ultimately returned from the other classes * and will include it in the plugin. * * @package Tutsplus_Namespace_Demo\Inc */ class FileRegistry { /** * This class looks at the type of file that's being passed into the autoloader. * * @var FileInvestigator */ private $investigator; /** * Creates an instance of this class by creating an instance of the FileInvestigator. */ public function __construct() { $this->investigator = new FileInvestigator(); } /** * Uses the file investigator to retrieve the location of the file on disk. If found, then * it will include it in the project; otherwise, it will throw a WordPress error message. * * @param string $filepath The path to the file on disk to include in the plugin. */ public function load( $filepath ) { $filepath = $this->investigator->get_filetype( $filepath ); $filepath = rtrim( plugin_dir_path( dirname( __FILE__ ) ), '/' ) . $filepath; if ( file_exists( $filepath ) ) { include_once( $filepath ); } else { wp_die( esc_html( 'The specified file does not exist.' ) ); } } }
Including the Files, Starting the Autoloader
Now that we have our files created, we need to make two more small changes:
- We need to include all of the classes in the inc directory.
- We need to get rid of the old autoloader code.
- And we need to use our new autoloader with the
spl_autoload_register
function.
Ultimately, the final version of autoload.php should look like this:
<?php /** * Loads all of the classes in the directory, instantiates the Autoloader, * and registers it with the standard PHP library. * * @package Tutsplus_Namespace_Demo\Inc */ foreach ( glob( dirname( __FILE__ ) . '/class-*.php' ) as $filename ) { include_once( $filename ); } $autoloader = new Autoloader(); spl_autoload_register( array( $autoloader, 'load' ) );
And it will accomplish exactly what we've outlined above.
But Wait, I'm Getting an Error!
At this point, you've done a lot of work. You've refactored your entire autoloader to use object-oriented programming. You've documented your classes and functions. You've created new files, removed code from old files, and you're ready to make sure everything is working as expected.
So, as any developer would do, you launch the browser window to refresh the page, only to be shown an error message:
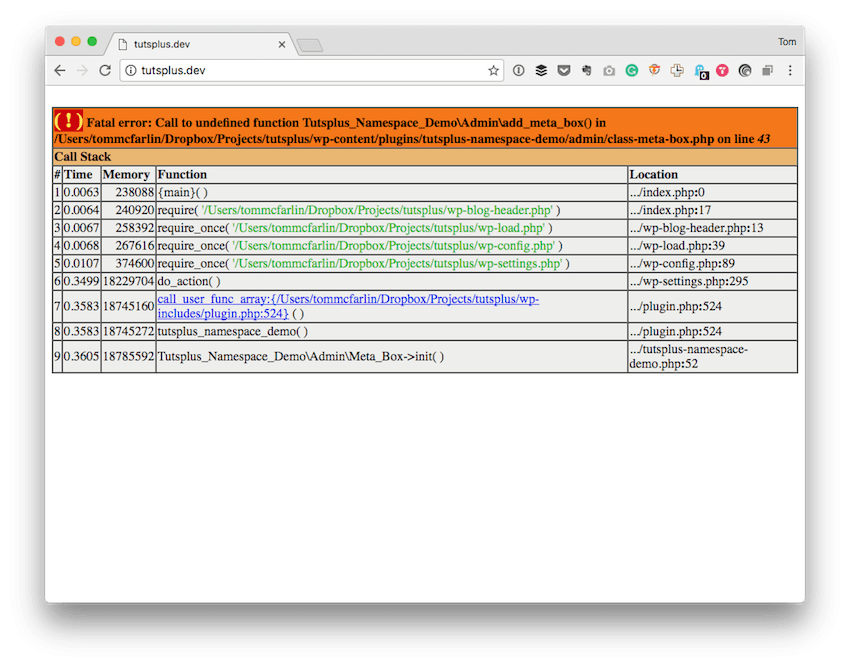
Luckily, this is an easy fix. The problem is that we're attempting to add our meta box too early. To fix this, we'll update the init
method in our Meta_Box
class to include this:
<?php /** * Registers the add_meta_box function with the proper hook in the WordPress page * lifecycle. */ public function init() { add_action( 'add_meta_boxes', array( $this, 'add_meta_box' ) ); }
And then we'll introduce a function that will be hooked to the work we just did:
<?php /** * Registers this meta box with WordPress. * * Defines a meta box that will render inspirational questions at the top * of the sidebar of the 'Add New Post' page in order to help prompt * bloggers with something to write about when they begin drafting a post. */ public function add_meta_box() { add_meta_box( 'tutsplus-post-questions', 'Inspiration Questions', array( $this->display, 'render' ), 'post', 'side', 'high' ); }
At this point, you should be able to execute the new code with no problems, no warnings, no notices, and no errors.
Conclusion
Working through all of this might have seemed like a lot—and it was! But the nice thing is that it covered a lot of ground in three tutorials, and it built on the work of a previous series. In that regard, a lot of new topics were covered and new techniques were learned.
Note that I write regularly for Envato Tuts+, and you can find all of my previous tutorials on my profile page. Additionally, I often discuss software development in the context of WordPress on my blog and on Twitter so feel free to follow me on either place.
With that said, study the code we've covered throughout this series (and perhaps the one prior to it), and see if you can't employ some of these techniques in your existing or future work.
Comments