Angular 2 is a powerful and feature-complete framework that you can use to build the best web apps. Built with TypeScript in mind, Angular 2 takes advantage of futuristic language features such as decorators and interfaces, which make coding faster and easier.
In this video from my course, Modern Web Apps With Angular 2, I'll show you how to use Angular 2 to build a service which will interact with the server. Note that in this video, we're building on code from earlier in the course, and you can find the full source code for the course on GitHub.
How to Build an Angular 2 Service
How to Create a Project Service
In the course so far, we've been building a project management application. Right now, the projects that we are displaying to the user are just hard coded right into our projects component. However, that's not a long-term solution. We want to have some way of getting a list of projects from our server. So in this video, we are going to create a project service.
In Angular, a service is basically any set of functionality that we want to be available to multiple components. It's just an easy way to wrap up some functionality. So inside of our app directory, let's create a projects service. And we'll call this projects.service.ts
.
Now of course a service is not a component, so there's no need to import the component decorator. But there is another decorator that we need, and that is Injectable
. So let's import Injectable
from angular/core
. Now as I said, Injectable
is a decorator, and it doesn't take any properties. So we'll just call Injectable
, and then export our class. We'll call the class ProjectsService
.

Injectable
makes this class something that Angular can use as dependency injection. As we'll see a bit later on, we use dependency injection to get an instance of this project service within a component that uses the project's service. An Angular queue uses dependency injection in this way so that it can easily inject mock services and things like that if you want to test your components.
Add Methods to the Service
So let's go ahead and add some methods to our ProjectsService
here. First of all we're going to need the Http
module that Angular has. This will allow us to make requests directly to the server. So let's import Http
, and we will also import the response class which we will need for some type checking. And both of these come from @angular/http
.
Now, we should also import the Http
module into our app modules file. So, let's go ahead and do that before we forget. In our native modules at the top, I will import the HttpModule
, and then down in our imports, let's include the HttpModule
.
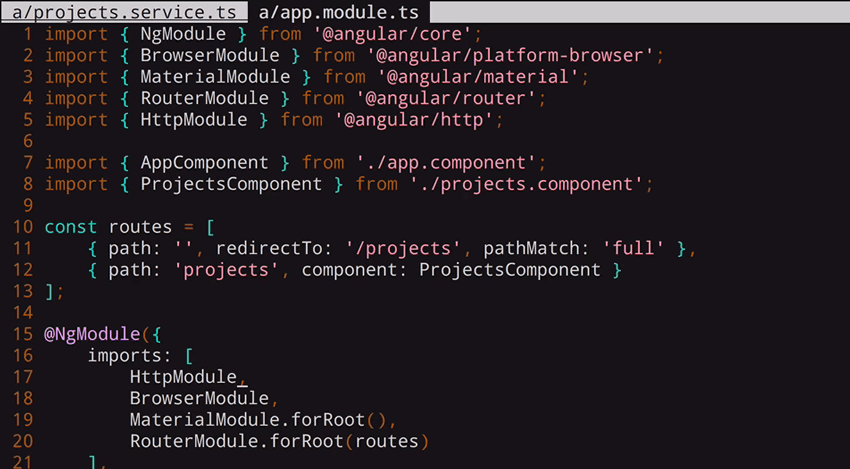
Now that we've imported that in both the necessary places, we can use dependency injection to inject this Http
class into our ProjectsService
. So instead of doing something like new Http()
in here, what we'll do is create a constructor function. And this constructor will take a property of type Http
.
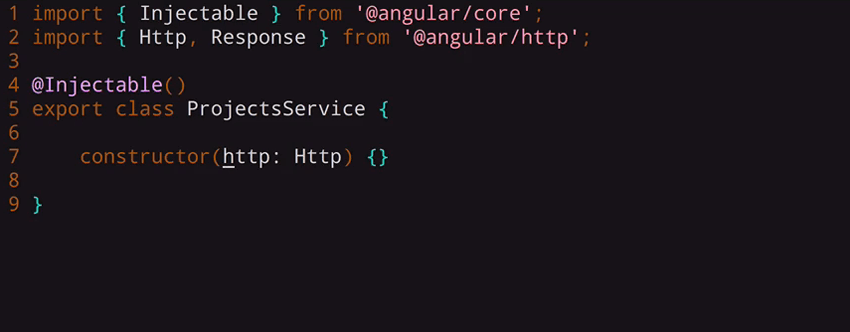
Angular will see this parameter when it's creating our ProjectsService
instance, and it will match this Http
class to the HttpModule
that we imported into our app module, and it will inject an instance of that into the constructor.
Now, we could write this.http = http;
to assign this parameter to a property of our class. But TypeScript actually has some shortcut syntax for that, so we can just apply the keyword private
directly inside the constructor, and TypeScript will automatically make it a class property. And now from within the other methods of the class, we can use this.http
.
So let's create a function called getProjects()
. This is going to be the method that we call to get our list of projects.
Now with functions in TypeScript, we can still use the : Type
syntax to specify the type of the return value of the function. And for getProjects()
we are going to return an Observable
that wraps Project
.
So before we talk about what that is, let's import those two classes. So I'm going to import Observable
from rxjs
, and let's also import our Project
model.
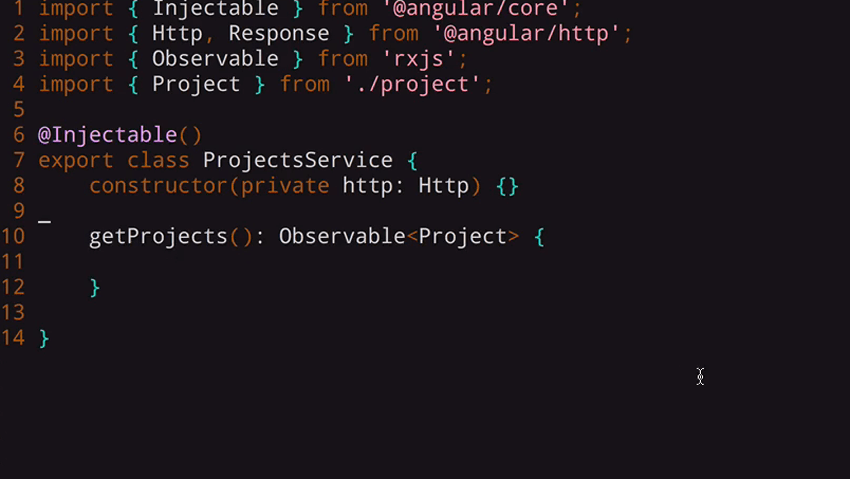
Working With Observables
So what is an observable? Unfortunately there's no way I could give you a complete introduction to observables here, but Angular 2 does depend quite a bit on observables, and I will try and make them as simple as possible as we go through this.
Basically, an observable is a wrapper similar to a promise or an array. Both promises, arrays, and observables have other items inside of them. In the case of an array, we have multiple items. In the case of a promise, we basically have some single value that we will get at some time in the future.
With observables, it could be one value or it could be many values. One definition that's sometimes used is an asynchronous array. Basically, an observable is a stream of data that we may get more of at any time. And I think you'll see over the course of some lessons here how we can use observables to make getting and setting some of our data quite a bit easier. For now, if you haven't seen observables before, you can just think of them as a type of promise.
So what will we return from this function? Well we can do this.http.get()
, so let's get /api/projects
which will return our list of projects. And then what we can do is map the response to a function that we're going to write called this.extractData
.
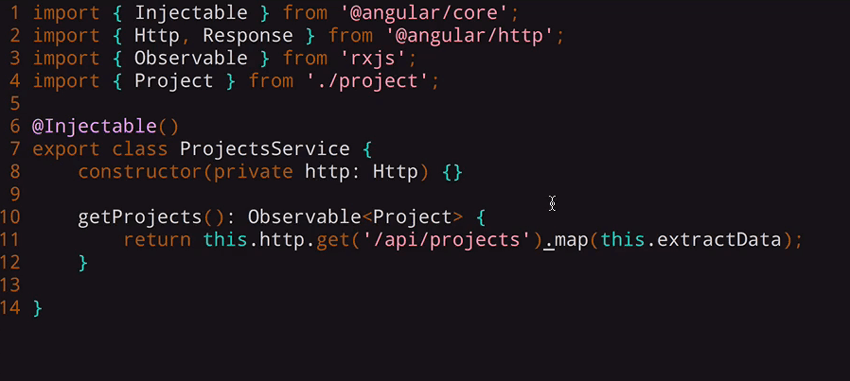
You can think of the map()
function here as the then()
method on a promise. It's works just like on an array where map()
will perform some operation on each one of the values inside that array and then return a new array with those new values.
So basically, map()
allows you to perform some kind of action on the values inside a container. And the same thing is true with the then()
method in a promise. You can call then()
on a promise to call some function on the value inside of a promise. And then that returns a new promise with whatever new value you created..
It's the same thing with map()
here. We are going to call extractData()
on the response that's inside this observable, and what we will return from this is an observable that wraps a project.
So let's create an extractData()
function, and this is going to take an Angular HTTP library Response
class.
So we'll return res.json()
, and this will convert the HTTP response into the actual JSON body. Now the value from extractData()
will be returned inside of our getProjects()
call, and Angular will see that this matches our return type here because it will be an observable array of projects.
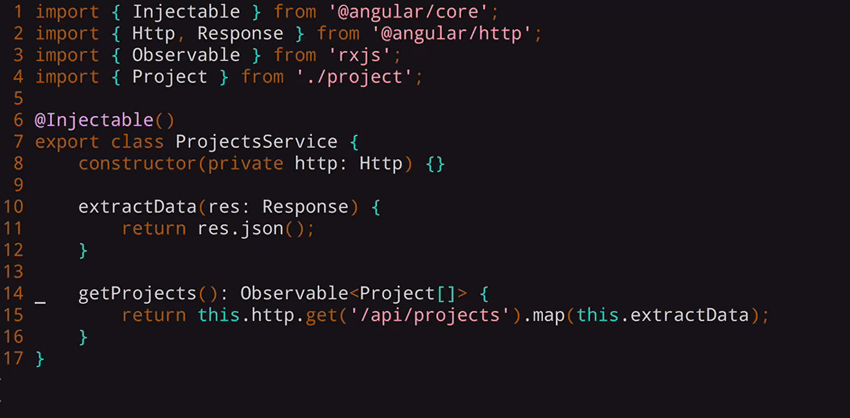
Import the Function in the Projects Component
Now that we have this getProjects()
function, let's head over to our projects component and import it. First of all, let's import the ProjectsService
.
Now because we want to inject a ProjectsService
instance into this component, we need to tell Angular that it should provide an instance for this component. So let's add a providers
property to our component decorator, and we'll tell it that it's going to need that ProjectsService
inside this component. So let's add a constructor, and we can use dependency injection in the same way that we did in our service.
We will create a parameter called service
, and this is going to be a ProjectsService
object, and so Angular will know to inject one of our ProjectsService
instances into this class. We'll give this parameter it the private
keyword here so that it sets that immediately as a property.
With this in place, we can go ahead and use it inside ngOnInit
. So in here we can call this.service.getProjects()
—remember this returns an observable—and the method that we want to call here subscribe()
.
You can think of the subscribe()
method as if we were calling then()
on a promise that was returned, or if you think about this as an array, subscribe()
is like the forEach()
method on an array. It's kind of like map()
in that it receives whatever is inside of the array, or in this case the observable.
However, forEach()
does not return a new array, and subscribe()
does not return a new observable. So it's kind of like the end of the line. So subscribe()
is going to get our projects list as its parameter, and we can just say this.projects
, which refers to our array of projects, will equal projects
. That way we can unwrap its value from the observable and the value will now be available from within the class.
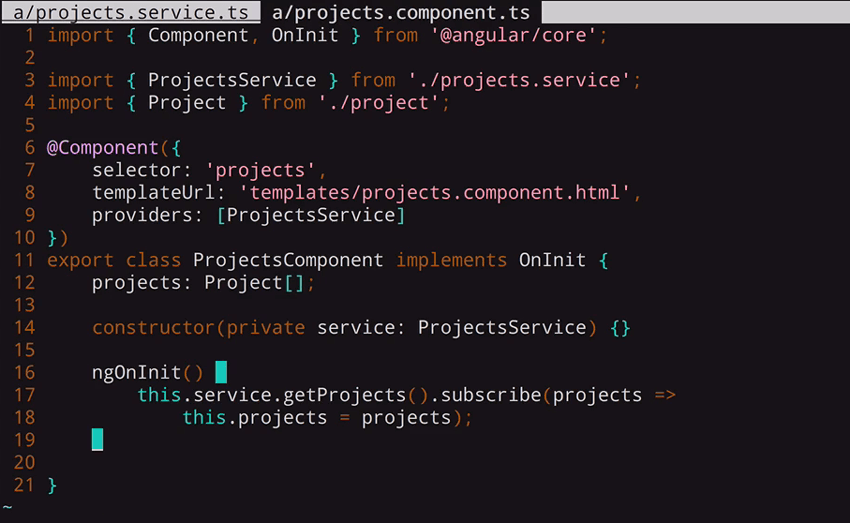
And if we go back to the browser to see our list of projects, we will see the three projects that I put in the server.
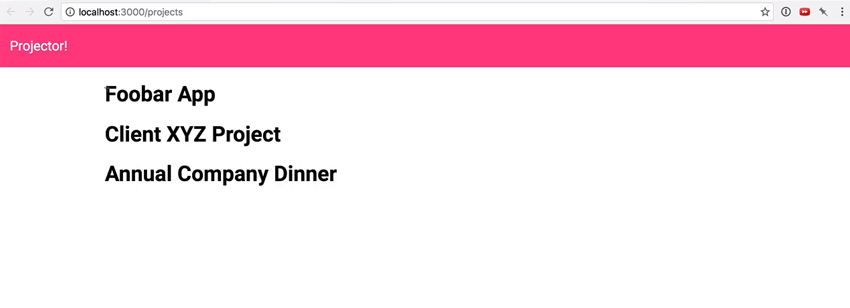
Watch the Full Course
In the full course, Modern Web Apps With Angular 2, I'll show you how to code a complete web app with Angular 2, using the most current features and architectural patterns.
You can follow along with me and build a full-featured project management app, with user login and validation and real-time chat. You'll get lessons on the Angular 2 template language, structuring your app, routing, form validation, services, observables, and more.
If you've never used Angular 2, learn everything you need to know in our course Get Started With Angular 2. If you want to build on your Angular 2 experience, why not check out:
Comments