While reading articles on websites, I've found that when a post is over four years old, a notification stating that the post is old is displayed on certain websites. A similar notification is also found in WordPress plugin directory when a plugin hasn't been updated in over two years.

In this article, we will be building a plugin that displays a similar notification when a post is over X-years old where "X" an integer that denotes the number of years that defines old.
The plugin will provide you the option to specify your own custom notification sentence and the number of years before a post is considered old.
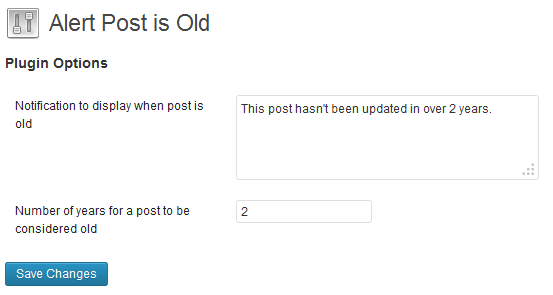
This plugin will be built using object-oriented programming in an attempt to make the code more organized and to adhere to the DRY principle. As such, some experience with object-oriented programming is required if you are to understand this tutorial.
Planning the Plugin
The plugin will consist of two protected
class properties and ten methods . The properties are described below.
-
$_notification
stores the notification message retrieved from the database that was previously set by the plugin user. -
$_years
stores the number of years retrieved from the database.
I will be explaining the role of each method (known as functions in procedural programming) and their respective code as we journey.
Coding the Plugin
First, let's include the plugin header, create the class
and define the properties:
<?php /* Plugin Name: Alert Post is Old Plugin URI: Description: Display a notification when a post is older than X-years where X is any number of years. Author: Agbonghama Collins Version: 1.0 Author URI: http://tech4sky.com/ */ class AlertOldPost { // stores the notification retrieved from DB protected $_notification; // stores the number of years retrieved from DB protected $_years;
When writing WordPress plugins in OOP, all the action and filter hooks can be set in the constructor (which is named __construct
). Our plugin's constructor method will consist of five functions: three action hooks, a filter hook and a register_activation_hook
function.
function __construct() { // Initialize setting options on activation register_activation_hook( __FILE__, array( $this, 'aop_settings_default_values' ) ); // register Menu add_action( 'admin_menu', array( $this, 'aop_settings_menu' ) ); // hook plugin section and field to admin_init add_action( 'admin_init', array( $this, 'pluginOption' ) ); // add the plugin stylesheet to header add_action( 'wp_head', array( $this, 'stylesheet') ); // display notification above post add_filter( 'the_content', array( $this, 'displayNotification' ) ); }
- The
register_activation_hook
calls the method to set the plugin default settings on activation. - The next three
add_action
functions call the hook functions to register the plugin menu, hook the plugin section and field toadmin_init
and add the plugin stylesheet to header respectively. - The
add_filter
call thedisplayNotification
method which displays the notification when a post is old.
Looking at the __construct
method above, the register_activation_hook
function calls the aop_settings_default_values
method to set the default plugin settings.
public function aop_settings_default_values() { $aop_plugin_options = array( 'notification' => 'This post hasn\'t been updated in over 2 years.', 'years' => 2 ); update_option( 'apo_alert_old_post', $aop_plugin_options ); }
The aop_settings_menu
method creates the plugin sub-menu under the existing Settings menu.
public function aop_settings_menu() { add_options_page( 'Alert Post is Old', 'Alert Post is Old', 'manage_options', 'aop-alert-post-old', array( $this, 'alert_post_old_function' ) ); }
The third argument passed to the add_options_page
function above is the alert_post_old_function
method that displays the page content of the plugin's settings.
public function alert_post_old_function() { echo '<div class="wrap">'; screen_icon(); echo '<h2>Alert Post is Old</h2>'; echo '<form action="options.php" method="post">'; do_settings_sections('aop-alert-post-old'); settings_fields('aop_settings_group'); submit_button(); }
To add the plugin settings, we will be using WordPress Settings API to add the settings forms.
Firstly, we define the section, add the settings fields and finally register the settings. All this will be done in the pluginOption
method that was hooked to the admin_init
action earlier in the __construct
method.
public function pluginOption() { add_settings_section( 'aop_settings_section', 'Plugin Options', null, 'aop-alert-post-old' ); add_settings_field( 'notification', '<label for="notification">Notification to display when post is old</label>', array( $this, 'aop_notification' ), 'aop-alert-post-old', 'aop_settings_section' ); add_settings_field( 'years', '<label for="years">Number of years for a post to be considered old</label>', array( $this, 'aop_years' ), 'aop-alert-post-old', 'aop_settings_section' ); register_setting( 'aop_settings_group', 'apo_alert_old_post' ); }
The settings field callback method: aop_notification
and aop_years
that fill fills the field with the desired form inputs are as follows.
public function aop_notification() { $this->databaseValues(); echo '<textarea id="notification" cols="50" rows="3" name="apo_alert_old_post[notification]">'; echo esc_attr( $this->_notification ); echo '</textarea>'; }
public function aop_years() { $this->databaseValues(); echo '<input type="number" id="years" name="apo_alert_old_post[years]" value="' . esc_attr($this -> _years) . '">'; }
We will retrieve the plugin notification and year settings and store them in the two protected properties: $_notification
and $_years
because they will come in handy when we are to determine if a post is over the set age and when displaying the notification message.
public function databaseValues() { $options = get_option( 'apo_alert_old_post' ); $this->_notification = $options['notification']; $this->_years = $options['years']; }
The CSS code use in styling the notification will be in the stylesheet
method.
public function stylesheet() { echo <<<HTML <!-- Alert post is old (author: http://tech4sky.com) --> <style type="text/css"> .oldPost { padding-top: 8px; padding-bottom: 8px; background-color: #FEEFB3; color: #9F6000; border: 1px solid; padding: 4px 12px 8px; margin-bottom: 20px; border-radius: 6px; } span.oldPost { background-color: #9F6000; color: #fff; padding: 1px 10px 0px; border-radius: 20px; font-size: 18px; font-weight: bold; font-family: Verdana; float: left; margin: 0px 8px 0px 0px; } span.oldtext { padding-top: 0px; color: #9F6000; } </style> <!-- /Alert post is old --> HTML; }
Finally, the function that displays the notification above the post content that was deemed old is as follows:
public function displayNotification( $content ) { global $post; $this->databaseValues(); // get settings year $setYear = $this->_years; // get notification text $notification = $this->_notification; // calculate post age $year = date( 'Y' ) - get_post_time( 'Y', true, $post->ID ); // show notification only on post if ( is_single() ) : if ( $year > $setYear ) { echo '<div class="oldPost">'; echo '<span class="oldPost"> ! </span>'; echo "<span class='oldtext'>$notification</span>"; echo '</div>'; } endif; return $content; }
Let's talk through the code above: First, we retrieve the number of years that determine when a post is old, subtracted the year the post was written from the present year. If the result is greater than the year that defines old, the notification that the post is old is displayed.
Finally, we are done coding the plugin class. To put the class to work, we need to instantiate it like so:
new AlertOldPost;
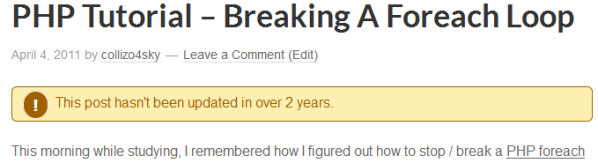
Conclusion
In this article, we learned how to calculate the age of a post, display a notification when a post is considered old and did so using object-oriented programming practices.
I urge you to review the code to gain an in-depth knowledge of how it works. Your questions, comments, and contributions are welcome.
Comments