Curious about 3D game development? Now is the time to learn! This five-part tutorial series will demonstrate how to build a simple game with ShiVa3D Suite, a cross-platform 3D game engine and development tool. This series was originally intended for Tuts+ Premium members only, but will instead be given away to the community for free with each portion published back-to-back over the next 5 days. Read on to begin your journey into 3D programming!
Series Introduction
In this tutorial series, we will introduce 3D game development for Android platform using ShiVa3D Suite. Developing an Android application with 3D graphics can be challenging for several reasons. Developers should be familiar with the OpenGL API and the specific Android API that supports it. Also, 3D graphics development may include native C/C++ code for performance reasons in addition to Java/Objective-C code. This increases the level of knowledge and effort required for developing a 3D Android application. Furthermore, creating 3D graphics involves unique expertise independent of Java or C/C++ programming competency. In summary, 3D application development requires advanced skills from different disciplines.
ShiVa3D Suite is a set of tools designed to develop multi-platform 3D applications. These tools make it easier to design and compose your application based on specific tasks and concepts. For example, you can separate a 3D visual model from its behavior and work on those aspects independently. In addition, game development in the ShiVa3D Suite allows for abstraction of the game from the particular target platform. Games developed with this suite can be deployed in multiple target platforms, including Windows, iOS and Android OS among others.
This tutorial gives an example of how to develop a 3D game for Android platform using two main elements of the ShiVa3D Suite, the ShiVa Editor and the ShiVa Authoring Tool. The target operating system of this tutorial application is Android 3.2 (Honeycomb). However, we'll also provide a discussion of how to port the game to iOS devices, such as the iPhone/iPod Touch and the iPad/iPad2.
Organization of the Series
This tutorial has been organized as a 5-part series. In part 1, we will introduce the tutorial application, discuss various ShiVa3D concepts and the main tools in the ShiVa3D Suite. In part 1, we will also explain the files in the download archive accompanying this tutorial. In part 2, we will start describing how to develop the game using the ShiVa Editor. We will introduce the ShiVa Editor modules used in developing the tutorial application. Then, we will talk about the Collada model files representing the main characters in the application. Finally, we will discuss some initial steps to create the application such as creating the game and the scene as well as importing the Collada models.
In part 3, we will show how to edit the scene of our application. We will also start entering the code for the AIModels of the game. In part 4, we will finish coding for the remaining AIModels and perform unit testing by animating the game. We will then export the game from the ShiVa Editor for importing into the Shiva Authoring Tool. Finally, we will discuss two different authoring options in the Shiva Authoring Tool, one for generating an Android executable and another one for generating an Eclipse project.
In part 5, we will begin by customizing the game in Eclipse. This will include the Eclipse project setup, code changes and building the Java code and native libraries. At that point we will have completed the tutorial in terms of development and deployment. In the remainder of part 5 we will review the code, discuss how to port the game to iOS devices and give some concluding remarks.
Credits
The Collada model and the related image files for the yellow bathtub duck as well as the Collada model for the egg are the courtesy of Sony Computer Entertainment Inc. Those models have been downloaded from the Basic Samples section of the collada.org Model Bank, and are licensed under the terms of the SCEA Shared Source License.
The 'marble' texture used in the background has been downloaded from http://www.texturewarehouse.com and is licensed under the Creative Commons License, Attribution-NonCommercial-ShareAlike 2.0.
The author has greatly benefited from a book on ShiVa3D, entitled Introduction to 3D Programming with ShiVaÆ. In addition, the multitouch event handling code explained in 'Code Review' borrowed a technique introduced in a tutorial at the ShiVa3D developer site, named Multitouch Management.
Description Of The Game
The game starts with a splash screen that displays the main characters of the game: a yellow bathtub duck in the foreground and an egg behind it, as shown below.
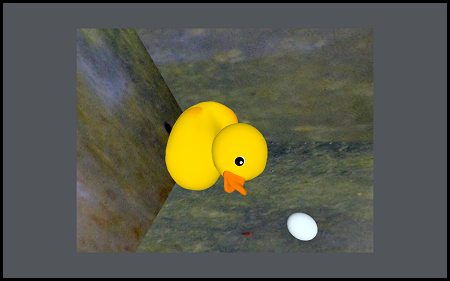
Then, the main screen of the game appears with two objects, the duck and the egg. The duck makes an angular motion in a 3D space on a fixed plane defined by y=3. The egg can only move along a straight line defined by x=0, y=3. The motion of duck and egg in the global x, y, z axes is shown below.
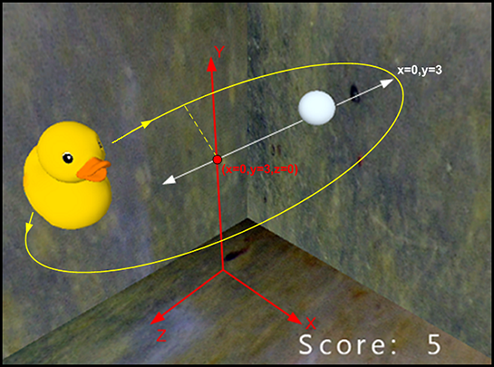
At the same time, the duck rotates around its local x, y, z axes, independent of its global rotation, as shown below.
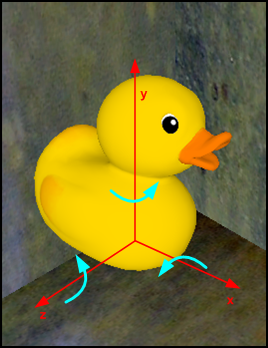
Similarly, the Egg turns around its local x-axis independent of its global motion (see below).
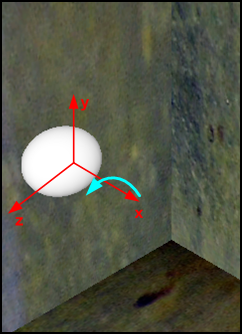
The (global) motion of the egg along the linear path can be controlled by the user's single touch action on the screen. If user's finger is moving from left to right then the egg moves in the negative z direction. Conversely, if user's finger is moving from right to left then the egg moves in the positive z direction. (See below.)
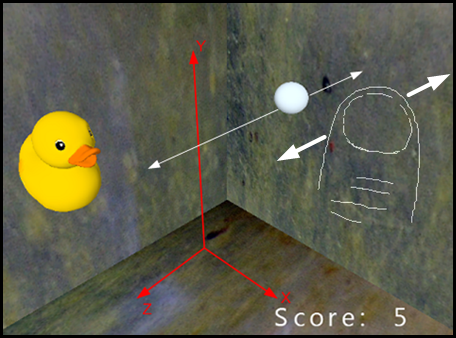
From the geometry in Figure 2, observe that the linear path describing the egg's global motion is on the fixed plane on which the duck makes its global motion. Therefore, the duck and the egg may collide at the two intersection points defined by the egg's linear path and the duck's angular path. The collision points are shown in the diagram below.
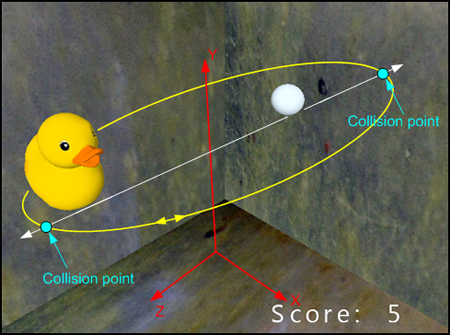
If the duck and the egg collide, the rotation of the duck will change direction, from clockwise to counter clockwise, or vice versa.
The goal of the game is to collide the egg and the duck as many times as possible. Every time a collision occurs, user's score, displayed on lower right corner of the screen, will be incremented. Also, the Android device will vibrate for a duration of 100 milliseconds.
The user can restart the game by applying a double touch action (e.g., moving two fingers on the screen) as shown below.
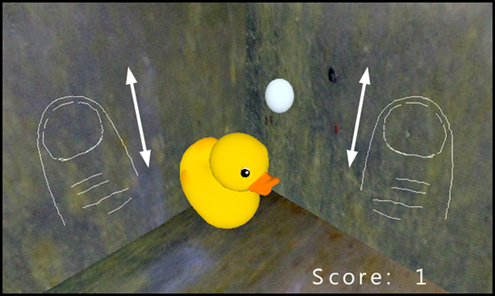
Before the application restarts, an informational message is displayed for about one second as shown below.
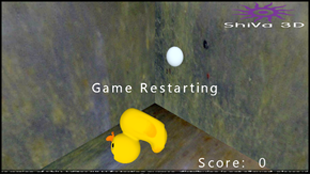
When the application restarts, the positions of the duck and the egg and the initial score are all reset.
ShiVa3D Development Environment
ShiVa3D Concepts
In this section, we will discuss basic concepts of 3D game development with ShiVa3D. Most of the discussion here is borrowed from original ShiVa3D documentation. For more information, please see http://www.stonetrip.com/developer/doc/ and the user manual that comes with ShiVa editor.
Game represents a game, the main entity of the application. It encapsulates everything else in the application such as cameras, scenes, models etc. Game is a stand alone deployment unit.
Scene represents a place or view associated with the game. There is a set of objects or models associated with a scene. A game can have more than one scene. For simplicity, the game in the tutorial application has a single scene.
Camera represents a viewpoint in the game. The user will see the game through the camera. A camera can move from one position to another, or its direction can be changed. In our tutorial, the camera will stay fixed.
Model is an object or a set of objects with various attributes such as shape, light, and sensor. In our game there are two models: a duck and an egg. Those are three dimensional objects with a particular 'role' in our game. Each role of the duck and the egg has its own AIModel (see below) to describe that role via code.
AIModel implies 'artificial intelligence' and represents behavior. An AIModel could be associated with a user who plays the game or an object in the game. In this tutorial, we will use only the object AIModels. An AIModel can have functions, handlers, states and variables to describe the specific behaviors.
Script contains the code in an AIModel, for example, code for a function or handler. The scripting language in ShiVa3D is Lua. (http://www.lua.org)
Sensor can detect certain physical events. In our game, the duck and the egg have collision sensors to detect a collision. When a sensor detects an event, it can be handled by the appropriate event handler in the AIModel associated with the object containing the sensor.
HUD stands for "head up display" and is a term used to represent various user interface widgets such as buttons, labels, lists, and sliders, allowing the user to interact with the game. The only HUD components we are going to use in our game are the text labels.
ShiVa3D Tools
To develop our tutorial application we will use the free version of the ShiVa3D Suite (http://www.stonetrip.com/download.html), which includes the ShiVa Editor PLE (personal learning edition) and the ShiVa Authoring Tool. The diagram below gives an overview of the development process we used with those tools.
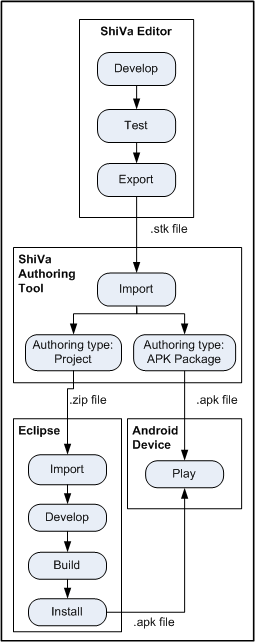
Let us discuss individual steps of that process.
ShiVa Editor
The ShiVa Editor has various components for developing and testing a 3D game from scratch. An important feature of the ShiVa Editor is that a game developed with that tool can be deployed (after being authored in the ShiVa Authoring Tool) in different devices with different operating systems (e.g. a PC running Windows OS, or a tablet with Android OS or iPhone).
Some of the basic actions you can perform with the ShiVa Editor are as follows.
- Develop:
- Create a new game.
- Create a new scene and associate it with the game.
- Import 3D models, edit their attributes and position them in a scene.
- Create AIModels, associate them with the models and write scripts for the AIModels.
- Attach sensors to models and write scripts to handle events associated with those sensors.
- Compile the scripts in the game.
- Test:
You can perform an initial test of your game in the development environment before deploying it in a target device. Testing is performed via the Animate feature of ShiVa Editor. For animation, you can change the settings for the screen size to see how the game will be displayed in the actual target device. You can input mouse click and key events from the mouse and keyboard of your development machine. To emulate touch events you may need additional provisions. For example, to test a handler for touch events, one option is to write a handler for key events that encapsulates functionality in the touch event handler. (See http://www.stonetrip.com/developer/1720-mix-mouse-and-multitouch.) Another option is to install the Device Development Tools (http://www.stonetrip.com/download.html). Then integrate the actual target device, running the ShiVa 3D Device Input Simulator, with the ShiVa Editor in the development machine over a Wi-Fi network. With that option, you can simulate the inputs directly using the target device. - Export:
After your testing is complete, export the game. This will generate a file with an .stk extension. The exported game will be used by the Shiva Authoring Tool, which is discussed next.
ShiVa Authoring Tool
The main purpose of the Shiva Authoring Tool is to convert a game, created via the ShiVa Editor, into an application that can be deployed in a particular device (e.g. an iPhone, iPad or Android tablet). Certain restrictions apply with regards to the operating system of the machine running the Shiva Authoring Tool and the target device for conversion. For example, the Shiva Authoring Tool running on a Windows machine cannot generate an iPad or iPhone application. In this tutorial, we used a Windows PC as the development machine and our target platform is Android. The information below applies to this particular environment.
Some of the basic actions one may perform with the Shiva Authoring Tool are the following.
- Import: Import the game which was exported via the ShiVa Editor.
-
Authoring: There are two authoring types: APK package and Project.
- If the authoring type is selected as an APK package, this will create an apk file ready for deployment into the target device. Optionally you can choose the install option while building. This will automatically install the APK file in the device as part of the build process. If you do not choose the install option while building, the resulting APK package can be installed in the target device another time using the Android SDK ADB tool.
- If the authoring type is selected as Project, this will create a zip archive that can be imported into Eclipse as an Android project. The project will contain Java and C files created by the Shiva Authoring Tool. You can edit those files to further customize the application for the target device. Please note that there is no need to choose the authoring type as Project unless you want to further customize your application in Eclipse.
Note: For each authoring type, there are two build options: Distribution and Development. In this tutorial, we will only discuss the Development build type.
Eclipse
One can customize the game in Eclipse as follows.
- Import: Import the Android application into Eclipse.
- Develop: Customize the code. You can change both the Java and C code that were automatically generated by the Shiva Authoring Tool.
- Build: Compile the Java code via Eclipse. Compile the C code and build the native libraries via the Cygwin make utility.
- Install: Install the application into the target device via Eclipse.
Software Pre-requisites
To use the ShiVa Authoring Tool in the Windows environment for Android development, we used the following pre-requisite software. Note that Eclipse and ADT for Eclipse are necessary only if you want to generate an Eclipse project for customization of the code. For details, see http://www.stonetrip.com/developer/doc/authoringtool/installation.
Software | Version Used in Tutorial Application |
Android SDK | revision 13 (Android 3.2) |
Android NDK | revision 7 |
Cygwin, GNU make package | version 3.82.90 |
Apache Ant | version 1.8.0 |
Java SDK | version 1.6 |
Eclipse | version 3.7 |
ADT for Eclipse | version 16.0.1 |
Note that our main target in this tutorial is Android 3.2 (Honeycomb), for which the application has been tested.
Files In Download Archive
In this section, we will give a description of the files in the archive file accompanying this tutorial.
The archive file has three sub-folders: set1, set2 and set3.
- The folder set1 consists of duck.dae, duckCM.tga, marble.jpg and sphere.dae used to create the game in ShiVa Editor. Please see the section 'Developing The Game In ShiVa Editor' for details on how to use those files.
- The folder set2 consists of app_icon.png, app_icon_72x72.png, app_splash.png and app_splash_800x1280.png. See the section named 'Shiva Authoring Tool' for details of how to use app_icon.png and app_splash.png. See'Customizing The Game In Eclipse' for details on how to use app_icon_72x72.png and app_splash_800x1280.png.
- In set3, there are three files: Duck.ste, Duck.stk and Duck_Android_final.zip.
- Duck.ste is an export of the tutorial game Duck from the ShiVa Editor. It provides you with a comprehensive version of the game with all of its resources, including the code as well as duck.dae, duckCM.tga, marble.jpg and sphere.dae. It represents the final state of the game in the section 'Developing The Game In ShiVa Editor'. If you experience an issue while following the instructions in that section, Duck.ste should be particularly useful. You can open two separate instances of the ShiVa Editor, import Duck.ste in one instance as a reference and work on the other instance to carry out the instructions. (In order to import Duck.ste in the ShiVa Editor, go to Main -> Projects to bring up the Settings dialog and click Add to add a new project. Then, in that project open up Data Explorer and select Import -> Archive. In the Import Archive text field specify the full path to Duck.ste.)
- Duck.stk is an export of the tutorial game Duck from ShiVa Editor as an Android runtime package. This is input to the ShiVa Authoring Tool. If you want, you can skip all the steps in 'Developing The Game In ShiVa Editor', go to the section named 'Shiva Authoring Tool' and start authoring by importing Duck.stk.
- Finally, Duck_Android_final.zip is an Android project archive in which the code customization has already been made. This project archive can be beneficial in several ways:
- You can use it as a reference while following the instructions in 'Customizing The Game In Eclipse'.
- If you want to skip the the instructions in 'Customizing The Game In Eclipse', simply import it into Eclipse. Make no code changes, then deploy the game into your device. (Note: Your Eclipse environment must have the Android SDK location set up in Eclipse Preferences, as described in 'Customizing The Game In Eclipse'.)
- This archive contains the Android application file Duck.apk in Duck\bin\classes folder that can be readily installed in an Android 3.2 compatible device via the Android SDK ADB tool without Eclipse.
Closing Remarks For Part 1
In part 1 of this tutorial, we introduced the tutorial application, discussed various ShiVa3D concepts as well as the main tools in the ShiVa3D suite. We also explained the files in the download archive accompanying this tutorial. In part 2, we will start describing how to develop the game using the ShiVa Editor. We will introduce the ShiVa Editor modules used in developing the tutorial application. Then, we will talk about the Collada model files representing the main characters in the application. We will then discuss some initial steps to create the application, such as creating the game and the scene as well as importing the Collada models.
Comments