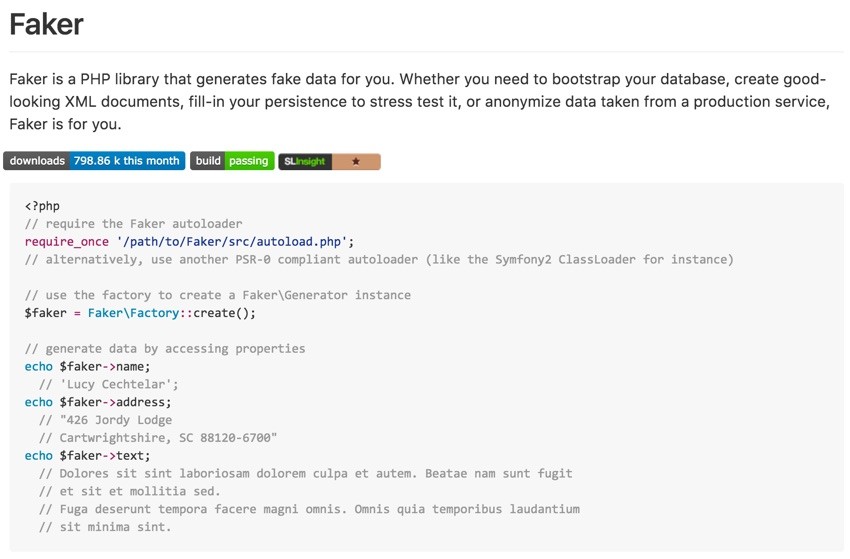
Faker is an open-source library created by Francois Zaninotto that generates artificial filler data for your application and its testing needs.
Faker can be used in a vanilla PHP application, a framework such as Yii or Laravel, or within a testing library such as we alluded to with Codeception in this earlier Envato Tuts+ tutorial.
In today's tutorial, I'll review the basic installation and usage of Faker and its capabilities. As Zaninotto says:
"Whether you need to bootstrap your database, create good-looking XML documents, fill-in your persistence to stress test it, or anonymize data taken from a production service, Faker is for you."
And, overall, it delivers a wide range of simple capabilities useful for any testing regimen.
A little reminder before we get started, I do participate in the comment threads below. I'm especially interested if you have additional thoughts or want to suggest topics for future tutorials. If you have a question or topic suggestion, please post below. You can also reach me on Twitter @reifman directly.
Getting Started
Installing Faker
I began by creating a new code tree and adding fzaninotto/faker
to composer.json below:
{ "name": "Faker Demonstration", "description": "for Envato Tuts+", "keywords": ["faker","php","jeff reifman"], "homepage": "http://www.lookahead.io/", "type": "project", "license": "BSD-3-Clause", "minimum-stability": "stable", "require": { "php": ">=5.6.0", "fzaninotto/faker" : "*" } }
Then, I updated composer:
$ composer update Loading composer repositories with package information Updating dependencies (including require-dev) - Installing fzaninotto/faker (v1.6.0) Loading from cache Writing lock file Generating autoload files
Faker is installed into the vendor directory. So, then I loaded at the top of an index.php file:
<?php // require the Faker autoloader require __DIR__ . '/vendor/autoload.php';
Next, I wanted to try a few simple example scenarios of generating data.
Simple Examples
I extended the Faker examples as follows and ran them from http://localhost:8888/faker:
<?php // require the Faker autoloader require __DIR__ . '/vendor/autoload.php'; // use the factory to create a Faker\Generator instance $faker = Faker\Factory::create(); // generate data by accessing properties echo $faker->name; // 'Lucy Cechtelar'; spacer(); echo $faker->address; // "426 Jordy Lodge // Cartwrightshire, SC 88120-6700" spacer(); echo $faker->text; // Dolores sit sint laboriosam dolorem culpa et autem. Beatae nam sunt fugit // et sit et mollitia sed. // Fuga deserunt tempora facere magni omnis. Omnis quia temporibus laudantium // sit minima sint. spacer(); function spacer() { echo '<br />'; } ?>
With quick refreshes, I was presented with varying results such as:
Ruthie Beier 37851 Gusikowski Flat Suite 594 Port Keithmouth, NM 06110 Reprehenderit eos suscipit sit modi architecto necessitatibus. Error maiores qui vero non omnis. Quaerat mollitia dolore deserunt quia quidem beatae.
And:
Darron Hessel 657 Elijah Lock Suite 202 Nitzschemouth, AZ 11166 Culpa dolorum quidem distinctio eius necessitatibus. Mollitia ut nostrum et ut quas veniam et. Unde iure molestiae aperiam fuga voluptatibus quo.
And:
Fredy Fadel MD 53328 Eldora Isle Apt. 634 West Eleanore, CA 95798-3025 Eligendi eos laudantium eveniet ad. Qui a voluptatibus est quia voluptatem. Dolorum pariatur quaerat nulla.
Faker delivers on its promise.
Faker's Default Providers
All of the data generation methods in Faker are created from the implementation of providers. Here's the code that registers Faker's default providers—it's done for you:
<?php $faker = new Faker\Generator(); $faker->addProvider(new Faker\Provider\en_US\Person($faker)); $faker->addProvider(new Faker\Provider\en_US\Address($faker)); $faker->addProvider(new Faker\Provider\en_US\PhoneNumber($faker)); $faker->addProvider(new Faker\Provider\en_US\Company($faker)); $faker->addProvider(new Faker\Provider\Lorem($faker)); $faker->addProvider(new Faker\Provider\Internet($faker));
So above, when I requested an address
from Faker, it searched all the providers for methods which matched, ultimately using the Address()
provider.
You can also write your own providers or browse a number of extensions to Faker that are available on the web.
Modifiers
Faker also offers special modifiers to aid your testing, such as unique(), optional(), or valid(). For example, you can generate unique numbers:
// unique() forces providers to return unique values $values = array(); for ($i=0; $i < 10; $i++) { // get a random digit, but always a new one, to avoid duplicates $values []= $faker->unique()->randomDigit; } print_r($values);
Here's the output of unique values:
Array ( [0] => 7 [1] => 6 [2] => 0 [3] => 2 [4] => 5 [5] => 1 [6] => 8 [7] => 4 [8] => 9 [9] => 3 )
If you use the optional()
method, some numbers will be returned as NULL
as if the user didn't enter a field on your form. Note: I couldn't get this method to work properly.
With valid()
, you can register functions which determine whether the filler data meets specific requirements or would return an error or generate an error message on a user form.
Exploring the Providers
Faker offers a broad set of methods for generating random data for your application:
- Base: simple methods for random letters, numbers, processed strings and regex
- Lorem Ipsum Text: random Latin text
- Person: names of people
- Address: mailing addresses
- Phone Number: phone numbers
- Company: names of companies
- Real Text: actual text written by human beings vs. meaningless Latin strings
- Date and Time: random dates and times
- Internet: emails, domains, etc.
- User Agent: browser strings
- Payment: credit card and SWIFT strings and numbers
- Color: random colors
- File: file extensions, file types, and file names
- Image: URLs of filler images of different kinds
- Uuid: unique IDs
- Barcode: various barcode types, e.g. ISBN13
- Miscellaneous: encryption codes, country codes, etc.
- Biased: random numbers with bias
Let's experiment with a few more of these methods.
Payment Information
The code below generates ten people, their credit card information and security codes:
$faker = Faker\Factory::create(); for ($i=0;$i<10;$i++) { $cc = $faker->creditCardDetails; $cc['security']=$faker->numberBetween(199,499); var_dump ($cc);spacer(2); }
Here's some output from the above code:
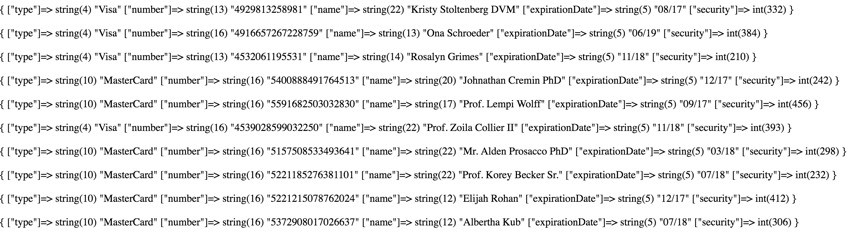
Images
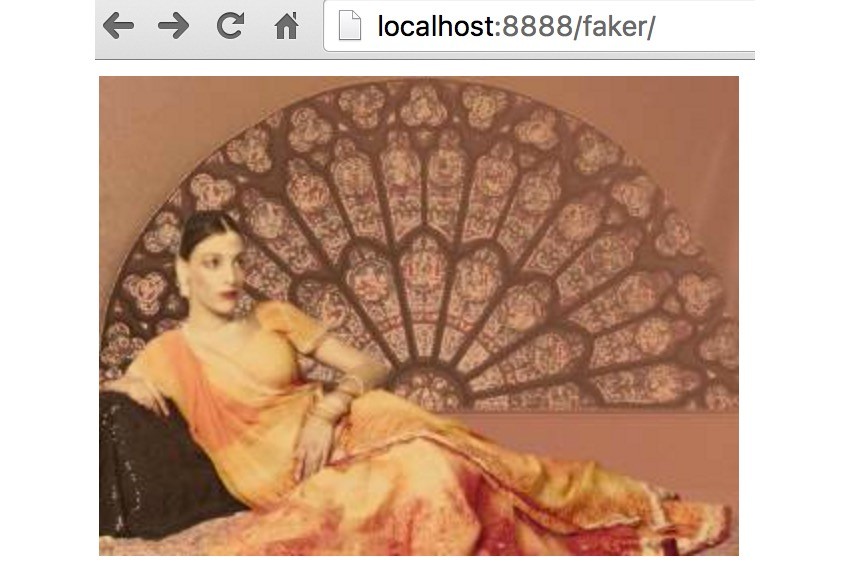
Here's a simple example of image generation:
$faker = Faker\Factory::create(); $width=320; $height=240; echo '<img src="'.$faker->imageUrl($width, $height).'"/>';
But you can also generate cats:
echo '<img src="'.$faker->imageUrl($width, $height, 'cats', true, 'Faker', true) .'"/>';
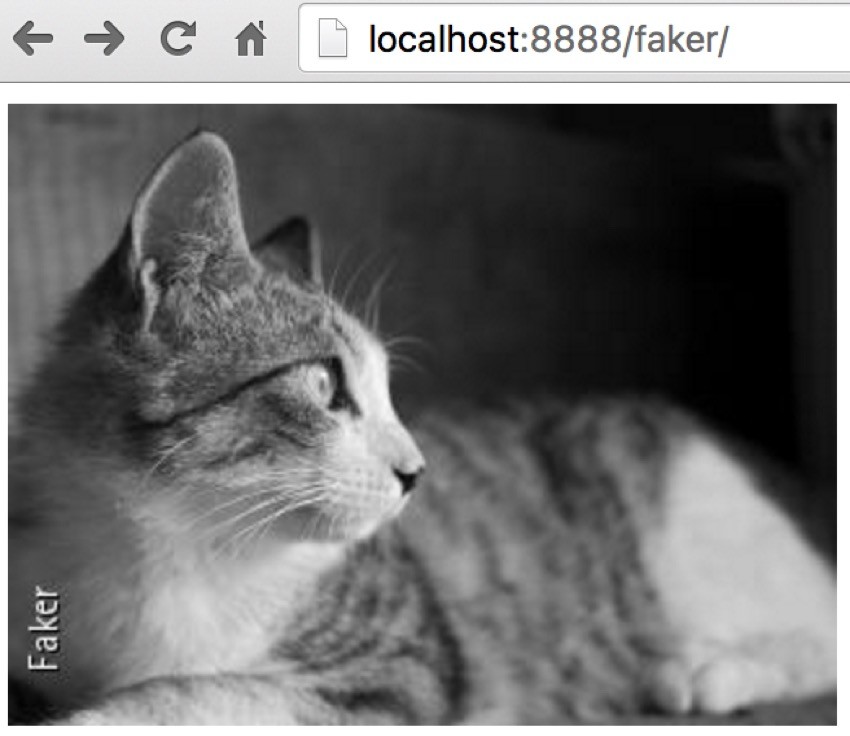
It may be the cat generation capability that won me over. I can't wait for three-dimensional printing and soul activation to work with stuff like this.
Internationalized Data
With the code below, I created a table with four columns of names from France, Russia, America, and China:
echo '<table> <thead> <th>French</th> <th>Russian</th> <th>English</th> <th>Chinese</th> </thead> <tr><td>'; $faker = Faker\Factory::create('fr_FR'); // create a French faker for ($i=0; $i < 10; $i++) { echo $faker->name; spacer(); } spacer(2); echo '</td><td>'; $faker = Faker\Factory::create('Ru_RU'); // create Russian for ($i=0; $i < 10; $i++) { echo $faker->name; spacer(); } spacer(2); echo '</td><td>'; $faker = Faker\Factory::create('En_US'); // create English for ($i=0; $i < 10; $i++) { echo $faker->name; spacer(); } spacer(2); echo '</td><td>'; $faker = Faker\Factory::create('zh_CN'); // create Chinese for ($i=0; $i < 10; $i++) { echo $faker->name; spacer(); } echo '</td></tr></table>';
Here's the output:
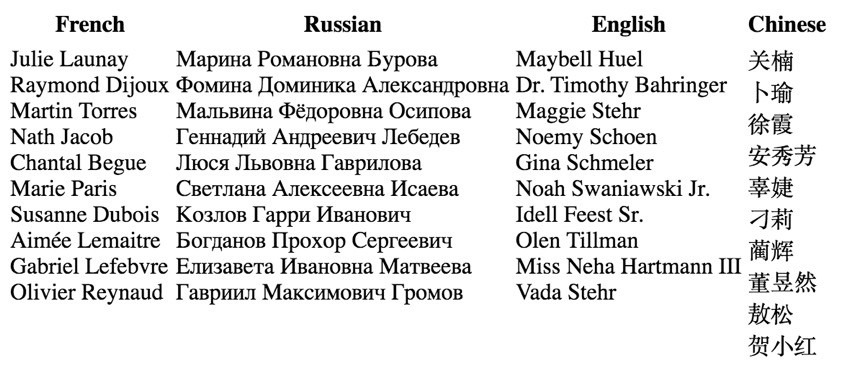
Creating Fake Email Addresses
Here's sample code to generate 25 fake email addresses from free providers such as Gmail and Yahoo:
$values = array(); for ($i=0; $i < 25; $i++) { // get a random digit, but also null sometimes $values []= $faker->freeEmail(); } print_r($values); exit;
Here's the resulting output:
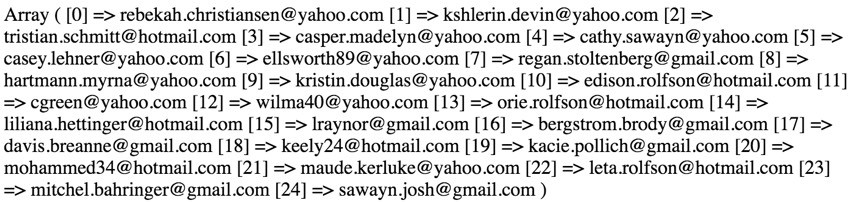
Generating XML Documents
Faker offers a helpful example of generating XML; however, it requires that you're working with a framework and have a view architecture:
<?php require_once '/path/to/Faker/src/autoload.php'; $faker = Faker\Factory::create(); ?> <?xml version="1.0" encoding="UTF-8"?> <contacts> <?php for ($i=0; $i < 10; $i++): ?> <contact firstName="<?php echo $faker->firstName ?>" lastName="<?php echo $faker->lastName ?>" email="<?php echo $faker->email ?>"/> <phone number="<?php echo $faker->phoneNumber ?>"/> <?php if ($faker->boolean(25)): ?> <birth date="<?php echo $faker->dateTimeThisCentury->format('Y-m-d') ?>" place="<?php echo $faker->city ?>"/> <?php endif; ?> <address> <street><?php echo $faker->streetAddress ?></street> <city><?php echo $faker->city ?></city> <postcode><?php echo $faker->postcode ?></postcode> <state><?php echo $faker->state ?></state> </address> <company name="<?php echo $faker->company ?>" catchPhrase="<?php echo $faker->catchPhrase ?>"> <?php if ($faker->boolean(33)): ?> <offer><?php echo $faker->bs ?></offer> <?php endif; ?> <?php if ($faker->boolean(33)): ?> <director name="<?php echo $faker->name ?>" /> <?php endif; ?> </company> <?php if ($faker->boolean(15)): ?> <details> <![CDATA[ <?php echo $faker->text(400) ?> ]]> </details> <?php endif; ?> </contact> <?php endfor; ?> </contacts>
In Conclusion
I hope that this has served as a basic introduction for you to Faker, an incredibly useful free, open-source PHP library.
If you want to read further, I recommend Jim Nielsen's Filler Content: Tools, Tips and a Dynamic Example (Envato Tuts+), which provides an application designer's take on generating data. He suggests that you can be more effective when you apply fake data to create a more realistic experience during your design process.
If you'd like to know when my next Envato Tuts+ tutorial arrives, follow me @reifman on Twitter or check my instructor page. Currently, I'm working on two series you may appreciate:
- Programming With Yii2 series
- Building Your Startup with PHP about Meeting Planner; go schedule your first meeting there today.
Comments