Isn't it fun that we get to play with the latest CSS techniques, like shadows and transitions? There's only one problem: how do we compensate, or more importantly, detect the browsers that do not support them? Well, there's a few solutions. In this tutorial and screencast, though, we'll create a JavaScript function that will accept a CSS property name as its parameter, and will return a boolean
, indicating whether or not the browser supports the passed property.
Prefer a Video Tutorial?
Subscribe to our YouTube page to watch all of the video tutorials!
Step 1
Let's begin by determining how we want to call our function
. We'll keep things simple here; something like the following should do the trick:
if ( supports('textShadow') ) { document.documentElement.className += ' textShadow'; }
That should be the final function
call. When we pass a CSS property name to the supports()
function, it'll return a boolean
. If true
, we'll attach a className
to the documentElement
, or <html>
. This will then provide us with a new `class` name to hook onto, from our stylesheet.
Step 2
Next, we'll construct the supports() function
.
var supports = (function() { })();
Why aren't we making supports
equal to a standard function? The answer is because we have a bit of prep work to do first, and there's absolutely no reason to repeat those tasks over and over every single time the function is called. In cases like this, it's best to make supports
equal to whatever is returned from the self-executing function.
Step 3
To test whether or not the browser supports specific properties, we need to create a *dummy* element, for testing. This generated element will never actually be inserted into the DOM; think of it as a test dummy!
var div = document.createElement('div');
As you're probably aware of, there are a handful of vendor-prefixes that we can use, when working with CSS3 properties:
- -moz
- -webkit
- -o
- -ms
- -khtml
Our JavaScript will need to filter through those prefixes, and test them. So, let's place them in an array
; we'll call it, vendors
.
var div = document.createElement('div'), vendors = 'Khtml Ms O Moz Webkit'.split(' ');
Using the
split()
function to create anarray
from astring
is admittedly lazy, but it saves a handful of seconds!
As we'll be filtering through this array
, let's be good boys and girls, and cache the length
of the array
as well.
var div = document.createElement('div'), vendors = 'Khtml Ms O Moz Webkit'.split(' '), len = vendors.length;
The prep work, above, is static, in nature, and doesn't need to be repeated every time we call supports()
. This is why we only run it once, when the page loads. Now, let's return
the function
that will actually be assigned to the supports
variable
.
return function(prop) { };
The beauty of
closures
is that, even thoughsupports()
is equal to that returnedfunction
, it still has access to thediv
,vendors
, andlen
variables.
Step 4
The immediate test: if the passed property is available to the div
's style
attribute, we know the browser supports the property; so return true
.
return function(prop) { if ( prop in div.style ) return true; };
Think of, say, the text-shadow
CSS3 property. Most modern browsers support it, without the need for a vendor prefix. With that in mind, why filter through all of the prefixes if we don't need to? That's why we place this check at the top.
Step 5
You're likely used to typing CSS3 property names, like so: -moz-box-shadow
. However, if, in Firebug, you review the style
object
, you'll find that it's spelled, MozBoxShadow
. As such, if we test:
'mozboxShadow' in div.style // false
False
will be returned. This value is case-sensitive.
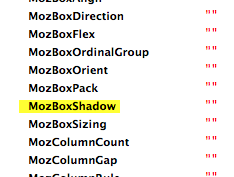
This means that, if the user passes boxShadow
to the supports()
function, it'll fail. Let's think ahead, and check if the first letter of the argument is lowercase. If it is, we'll fix the error for them.
return function(prop) { if ( prop in div.style ) return true; prop = prop.replace(/^[a-z]/, function(val) { return val.toUpperCase(); }); };
Regular expressions to the rescue! Above, we're checking if there is a single lowercase letter at the beginning of the string (^
). Only on the condition that one is found, we use the toUpperCase()
function to capitalize the letter.
Step 6
We next need to filter through the vendors
array, and test if there's a match. For instance, if box-shadow
is passed, we should test if the style
attribute of the div
contains any of the following:
- MozBoxShadow
- WebkitBoxShadow
- MsBoxShadow
- OBoxShadow
- KhtmlBoxShadow
If a match is found, we can return true
, because the browser does, indeed, provide support for box shadows!
return function(prop) { if ( prop in div.style ) return true; prop = prop.replace(/^[a-z]/, function(val) { return val.toUpperCase(); }); while(len--) { if ( vendors[len] + prop in div.style ) { return true; } } };
Though we could use a for
statement to filter through the array
, there's no real need to in this case.
- The order isn't important
-
while
statements are quicker to type, and require fewer characters - There's a tiny performance improvement
Don't be confused by vendors[len] + prop
; simply replace those names with their real-life values: MozBoxShadow
.
Step 7
But, what if none of those values match? In that case, the browser doesn't seem to support the property, in which case we should return false
.
while(len--) { if ( vendors[len] + prop in div.style ) { return true; } } return false;
That should do it for our function! Let's test it out, by applying a className
to the html
element, if the browser supports, say, the text-stroke
property (which only webkit does).
if ( supports('textStroke') ) { document.documentElement.className += ' textStroke'; }
Step 8: Usage
With a class
name that we can now hook onto, let's try it out in our stylesheet.
/* fallback */ h1 { color: black; } /* text-stroke support */ .textStroke h1 { color: white; -webkit-text-stroke: 2px black; }
Final Source Code
var supports = (function() { var div = document.createElement('div'), vendors = 'Khtml Ms O Moz Webkit'.split(' '), len = vendors.length; return function(prop) { if ( prop in div.style ) return true; prop = prop.replace(/^[a-z]/, function(val) { return val.toUpperCase(); }); while(len--) { if ( vendors[len] + prop in div.style ) { // browser supports box-shadow. Do what you need. // Or use a bang (!) to test if the browser doesn't. return true; } } return false; }; })(); if ( supports('textShadow') ) { document.documentElement.className += ' textShadow'; }
For a more comprehensive solution, refer to the Modernizr library.
Comments