Now we understand that Twig—along with the WordPress plugin Timber—can help developers write modular code while developing WordPress themes. With such a modular approach you can handle the logic and the view layer of your web application separately. Let's jump to slightly more technical stuff: creating Twig templates, converting HTML to Twig files, and how to render the logic or data with twig templates.
Install Timber
First of all, I am going to install Timber, which is a WordPress plugin that helps integrate the Twig templating engine with WP. So, let's do it.
- Log in to your WordPress dashboard.
- Go to Plugins > Add New.
- Search for the Timber plugin.
- Install and activate the plugin.
Once Timber is installed, you can now start splitting your template files into data and view files.
Creating a Twig Template
Before we start creating Twig templates, I am assuming that you have some kind of local WordPress setup. For this particular tutorial, my setup is:
- A localhost WP install (I'm using DesktopServer by ServerPress).
- Timber plugin installed and activated.
- Optional: Any base/starter theme (I'm using my own, i.e. Neat).
UpStatement has also built a Timber Starter Theme.
Let's begin. I want to display a welcome message at the top of my homepage. How would I go about it without Twig? Probably, I'd include HTML code inside the PHP file and echo the welcome message, as I did in the code example below. My index.php
file looks something like this.
<?php /** * Homepage */ get_header(); ?> <div> <?php echo "Welcome to my blog!"; ?> </div> <?php get_footer(); ?>
Now the homepage of my local WordPress install displays a welcome message right at the top. Here's the screenshot.
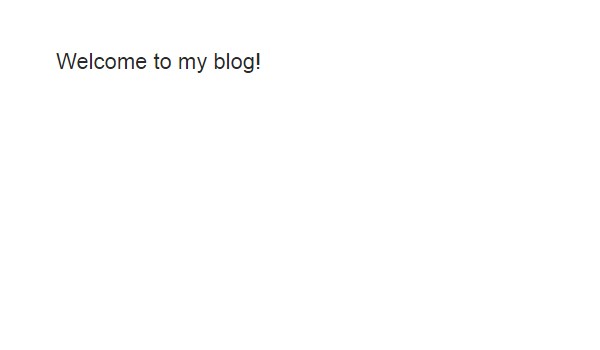
Problem
The problem with this approach is that we are mixing data/logic with view. The more complex this file gets, the harder it is to maintain let alone understand—for example, if you add the WordPress loop after this code with a few arguments and then filter and pagination after that. Add to that, PHP inside HTML doesn't look good when you end up with a bit of the logic.
Modular Approach
To make it more modular, we can think of our content on the homepage in the form of blocks or components. There can be two complete ones by default, i.e. Posts from The_Loop and pagination. Since now we want to add another component at the top, i.e. the welcome message, let's create a Twig file for that component.
Open a new blank file in the editor and copy paste the following lines of code.
<!-- Welcome Template --> <section class="welcome_message"> <h2>Welcome to my website!</h2> </section>
Create a new folder in your theme's root called views and save this file with the .twig
extension. For example, I saved the file as welcome.twig
.
Rendering the Twig Template
Timber provides us with a few useful functions, one of which is the render function. You can call it like this:
Timber::render();
This function can take up to four arguments. Since that's out of the scope of this article, you can read about it in the Timber docs. We can pass the name of any Twig file that's present in the views folder of your theme to this function.
Let's render the welcome message in the index.php
file.
<?php /** * Homepage */ get_header(); // Timber Render. Timber::render( 'welcome.twig' ); get_footer();
Timber renders the welcome.twig
file, loads the HTML and displays the new modified view layer at the front-end like this:
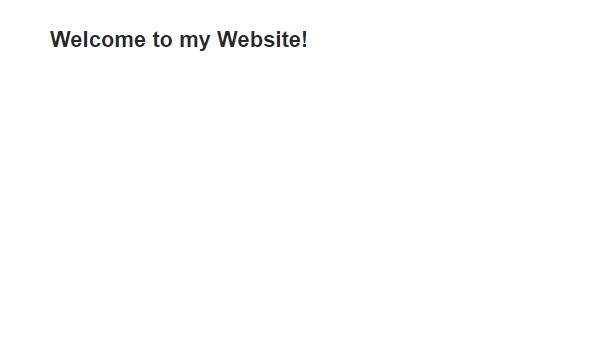
The render()
function takes welcome.twig
as its argument, but it automatically reads this file as long as the twig templates reside inside the folder named views.
If you want to use a custom name/path for the folder then you'll have to provide the path of that folder. For example, I created a twig folder in my theme's root and added it to the render function's argument.
<?php Timber::render('twig/welcome.twig'); ?>
The Official Load Order
Timber will first look in the child theme and then fall back to the parent theme (same as WordPress logic). The official load order is:
- User-defined locations
- Directory of calling PHP script (but not theme)
- Child theme
- Parent theme
- Directory of calling PHP script (including the theme)
Item 2 is inserted above others so that if you're using Timber in a plugin it will use the twig files in the plugin's directory.
So, now the index.php file has no HTML inside it, and it is rendering a Twig template.
Now let's send some dynamic data from index.php
to the welcome.twig
file and render it with Timber.
Sending Data Through Timber to Twig Files
To send data from the PHP file to Twig file, you need to define a context array. The render function takes an array of data to provide Twig templates with some kind of context. Let's call the array $context
, which is an associative array, i.e. it takes up the key-value pairs.
Let's add a key-value pair that would be a dynamic welcome message, which our PHP file would send to the Twig file.
Data File
My index.php
file looks like this now.
<?php /** * Homepage */ get_header(); // Context array. $context = array(); // Dynamic message. $var = 'Dynamic Message'; // Dynamic data. $context['the_message'] = $var; // Render twig file with the give $context array. Timber::render( 'welcome.twig', $context ); get_footer();
So, inside the index.php
file, I defined an empty $context
array at line #8. Then at line #11, I've created a variable $var
with the value 'Dynamic Message'
. At line #14, I have created a key the_message
which is equal to $var
.
At line #17, I called the render function with the welcome.twig
file, but this time, it takes an additional argument, i.e. the $context
array. This new argument actually has the data which Timber will send from the PHP file to the Twig file.
So, we defined the context array and added a dynamic message (You can show a different message to different users by adding some kind of logic to it, like displaying the current user's first name).
Template File
Now we need to use this data, i.e. the_message
, in the Twig file. We can echo a variable inside our Twig template by putting double braces around it. For instance, to echo $var in a twig file, we can write {{ var }}
. That's exactly what I did.
<!-- Message Template --> <section class="message"> <h2>{{ the_message }}</h2> </section>
The value of the_message
will be printed inside the h2 tags. I know, it's just that simple, and the code looks pretty clean.
That's it! Save the code and view the dynamic welcome message at the front-end. Here's the final screenshot.
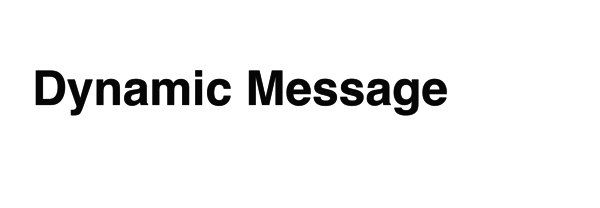
Conclusion
To summarize, now you can use the PHP file for coding the logic and providing data to a Twig template which contains HTML and renders the Twig variables or functions inside the double braces.
This modular approach can lead to a separate template file for every component in your WordPress theme. Think about having a message.twig component which you could use anywhere in the theme to display any message you want, any number of times.
This was a basic implementation of Twig with Timber. However, in the next two articles I will write about the Timber WordPress Cheatsheet, managing images with Timber, and creating menus without using the crazy walker function.
If you have any questions, post them in the comments below or reach out on Twitter. You can also take a look at my theme code at this GitHub repo.
Comments