Text messaging has become extremely widespread throughout the world -- to the point where an increasing number of web applications have integrated SMS to notify users of events, sales or coupons directly through their mobile devices.
In this tutorial, we will cover the fundamentals of sending text messages with PHP.
Premium Option
Before we get into the step-by-step process, you might want to look at a ready-made solution: SMS Sender, available on Envato Market. This PHP script lets you:
- create and import contacts and groups
- send a single SMS, or send bulk SMS to a group or multiple groups
- connect to any SMS gateway with minimal configuration
- customize your SMS or email
- add a link to your SMS and email
- and much more
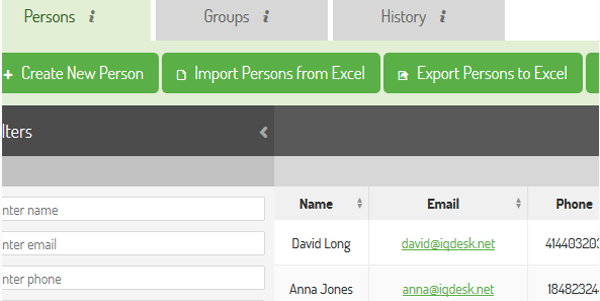
If you'd prefer to build your text-message sender from scratch, here's how to do it.
Overview
Sending a text message (SMS) message is actually pretty easy.
Below is a simplified diagram of how a message can be sent from a web application to a wireless device.
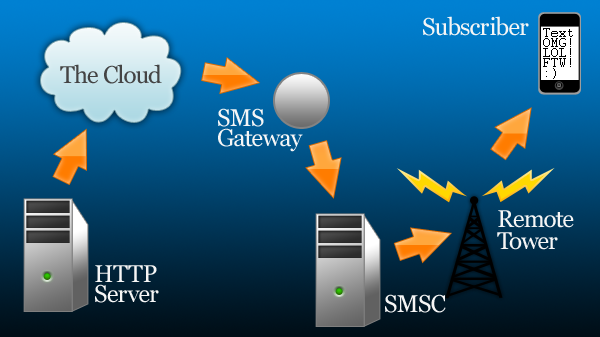
We'll break this down -- one piece at a time:
- The message is composed using a web application that is stored and executed on a HTTP server and then sent through the internet ("the cloud") as an email message.
- The email is received by a Short Message Service Gateway (SMS Gateway), which converts the message from an email message to a SMS message.
- The SMS message is then handed to a Short Message Service Center (SMSC), which is a server that routes data to specific mobile devices.
- The message is finally transmitted over the wireless network to the recipient.
Most wireless networks have a SMS gateway through which email messages can be sent as text messages to a mobile device. This is nice, because, from a developer's standpoint, it is generally free—however, it is of course not a free service for the end user. Fees still apply to the recipient of the message and messages sent via email will be billed as a non-network text message.
Email to SMS
To send a SMS via email, you'll generally require only two things:
- The phone number or unique identifier of the mobile device you want to reach.
- And the wireless network's domain name (many can be found in this list of email to SMS addresses)
The following convention can be followed for most carriers:
[email protected]
phoneNumber
is the phone number of the mobile device to send the message to, and domainName.com
is the address for the network's SMS Gateway.
To send a text to Mr. Example, you could simply add [email protected] to any email client, type a message and hit send. This will send a text message to phone number +1 (385) 555-0168 on the Verizon Wireless Network.
For example, I'll send a text message to myself using Gmail.
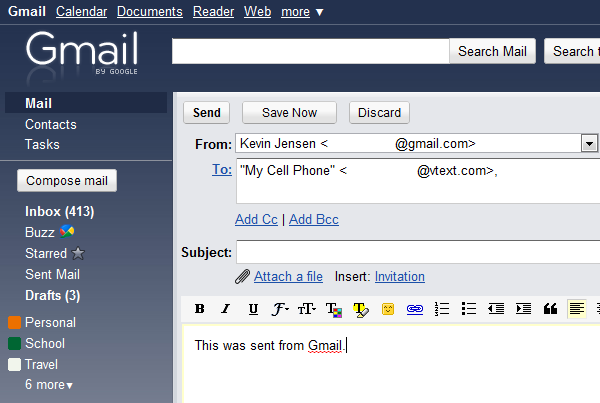
When my phone receives the message, it should look like so:
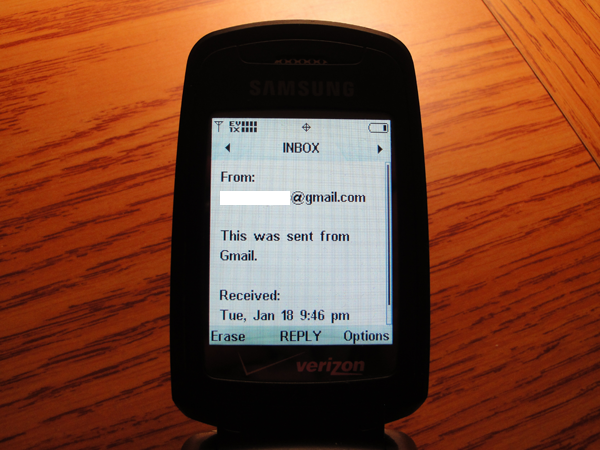
Pretty awesome!
PHP's mail
Function
Let's take things a step further. Using the SMS Gateway, we can send a text message via email using PHP's mail
function. The mail
function has the following signature:
bool mail ( string $to , string $subject , string $message [, string $additional_headers [, string $additional_parameters ]] )
You can read more about it here.
-
$to
defines the receiver or receivers of the message. Valid examples include:- [email protected]
- [email protected], [email protected]
- User <[email protected]>
- User1 <[email protected]>, User2 <[email protected]>
-
$subject
is rather self explanatory; it should be a string containing the desired subject. However, SMS do not require a subject. -
$message
is the message to be delivered. As mentioned in the PHP manual, "each line should be separated with a LF (\n). Lines should not be larger than 70 characters."
To replicate the earlier functionality, we could write the following PHP code:
mail( '[email protected]', '', 'Testing' );
A Test Drive
Let's run a test with PHP to make that sure everything is setup correctly and that the mail
function will, in fact, send a text message. Using the following code, we can run:
<?php var_dump( mail( '##########@vtext.com', '', 'This was sent with PHP.' ) ); // bool(true) ?>
When my phone receives the message, it looks like so:
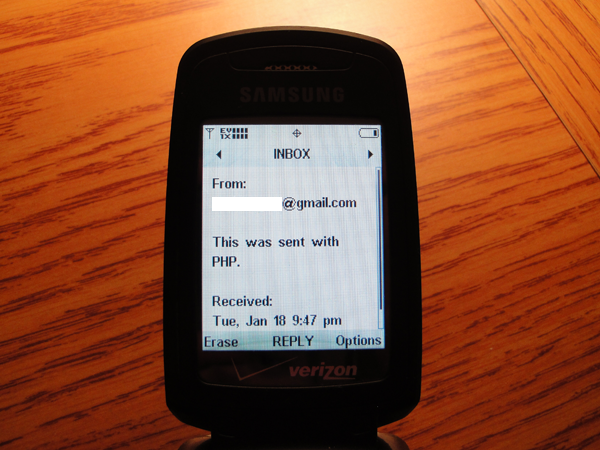
If you are getting an error, see the troubleshooting section.
As you can see in the image above, the message shows that it is from Gmail. This is because I route all my outgoing messages from my local server through that service. Unfortunately, as of this writing, I have been unsuccessful at altering the From
header to reflect an alternate address. It seems that the email headers are stripped and replaced with headers prepared by the SMS gateway. If anyone knows of a workaround, please leave a comment and let the rest of us know!
Adding Usability
The Markup
With the basics out of the way, let's take this idea and wrap a user interface around it. First we'll set up a simple form:
<!DOCTYPE html> <head> <meta charset="utf-8" /> </head> <body> <div id="container"> <h1>Sending SMS with PHP</h1> <form action="" method="post"> <ul> <li> <label for="phoneNumber">Phone Number</label> <input type="text" name="phoneNumber" id="phoneNumber" placeholder="3855550168" /></li> <li> <label for="carrier">Carrier</label> <input type="text" name="carrier" id="carrier" /> </li> <li> <label for="smsMessage">Message</label> <textarea name="smsMessage" id="smsMessage" cols="45" rows="15"></textarea> </li> <li><input type="submit" name="sendMessage" id="sendMessage" value="Send Message" /></li> </ul> </form> </div> </body> </html>
The Style
Next we'll sprinkle in some CSS:
body { margin: 0; padding: 3em 0; color: #fff; background: #0080d2; font-family: Georgia, Times New Roman, serif; } #container { width: 600px; background: #fff; color: #555; border: 3px solid #ccc; -webkit-border-radius: 10px; -moz-border-radius: 10px; -ms-border-radius: 10px; border-radius: 10px; border-top: 3px solid #ddd; padding: 1em 2em; margin: 0 auto; -webkit-box-shadow: 3px 7px 5px #000; -moz-box-shadow: 3px 7px 5px #000; -ms-box-shadow: 3px 7px 5px #000; box-shadow: 3px 7px 5px #000; } ul { list-style: none; padding: 0; } ul > li { padding: 0.12em 1em } label { display: block; float: left; width: 130px; } input, textarea { font-family: Georgia, Serif; }
This gives us the following simple form:
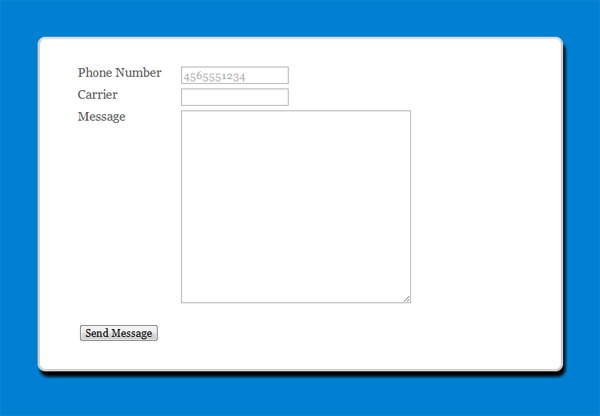
The Script
The most important part to this is the PHP script. We'll write that bit of code now:
<?php if ( isset( $_REQUEST ) && !empty( $_REQUEST ) ) { if ( isset( $_REQUEST['phoneNumber'], $_REQUEST['carrier'], $_REQUEST['smsMessage'] ) && !empty( $_REQUEST['phoneNumber'] ) && !empty( $_REQUEST['carrier'] ) ) { $message = wordwrap( $_REQUEST['smsMessage'], 70 ); $to = $_REQUEST['phoneNumber'] . '@' . $_REQUEST['carrier']; $result = @mail( $to, '', $message ); print 'Message was sent to ' . $to; } else { print 'Not all information was submitted.'; } } ?> <!DOCTYPE html>
- The script first checks to see if the form has been submitted.
- If yes, it checks to see if the
phoneNumber
,carrier
andsmsMessage
variables were sent. This is useful in the case where there may be more than one form on the page. - If
phoneNumber
,carrier
andsmsMessage
are available andphoneNumber
andcarrier
are not empty, it is okay to attempt to send the message. - The message argument in the
mail
function should be 70 characters in length per line. We can chop the message into 70 character chunks using thewordwrap
function. -
phoneNumber
andcarrier
are concatenated and then the message is sent using themail
function. - If data is missing or it cannot be validated, the script simply returns Not all information was submitted.
- Finally,
mail
returns a boolean indicating whether it was successful or not. The value is stored in$result
in case I needed to verify that the message was in fact sent.
Note: The mail
method only notifies whether the message was sent or not. It does not provide a way to check to see if the message was successfully received by the recipient server or mailbox.
The Final Code
<?php if ( isset( $_REQUEST ) && !empty( $_REQUEST ) ) { if ( isset( $_REQUEST['phoneNumber'], $_REQUEST['carrier'], $_REQUEST['smsMessage'] ) && !empty( $_REQUEST['phoneNumber'] ) && !empty( $_REQUEST['carrier'] ) ) { $message = wordwrap( $_REQUEST['smsMessage'], 70 ); $to = $_REQUEST['phoneNumber'] . '@' . $_REQUEST['carrier']; $result = @mail( $to, '', $message ); print 'Message was sent to ' . $to; } else { print 'Not all information was submitted.'; } }
?> <!DOCTYPE html> <head> <meta charset="utf-8" /> <style> body { margin: 0; padding: 3em 0; color: #fff; background: #0080d2; font-family: Georgia, Times New Roman, serif; } #container { width: 600px; background: #fff; color: #555; border: 3px solid #ccc; -webkit-border-radius: 10px; -moz-border-radius: 10px; -ms-border-radius: 10px; border-radius: 10px; border-top: 3px solid #ddd; padding: 1em 2em; margin: 0 auto; -webkit-box-shadow: 3px 7px 5px #000; -moz-box-shadow: 3px 7px 5px #000; -ms-box-shadow: 3px 7px 5px #000; box-shadow: 3px 7px 5px #000; } ul { list-style: none; padding: 0; } ul > li { padding: 0.12em 1em } label { display: block; float: left; width: 130px; } input, textarea { font-family: Georgia, Serif; } </style> </head> <body> <div id="container"> <h1>Sending SMS with PHP</h1> <form action="" method="post"> <ul> <li> <label for="phoneNumber">Phone Number</label> <input type="text" name="phoneNumber" id="phoneNumber" placeholder="3855550168" /></li> <li> <label for="carrier">Carrier</label> <input type="text" name="carrier" id="carrier" /> </li> <li> <label for="smsMessage">Message</label> <textarea name="smsMessage" id="smsMessage" cols="45" rows="15"></textarea> </li> <li><input type="submit" name="sendMessage" id="sendMessage" value="Send Message" /></li> </ul> </form> </div> </body> </html>
Troubleshooting
Localhost Error
In order to use the mail
function, you must have a mail server running. If you're running this on a web host, you're probably okay. But if you are unsure, I recommend talking to an administrator. This also holds true for personal machines. So if you get errors like..
Warning: mail() [function.mail]: Failed to connect to mailserver at "localhost" port 25, verify your "SMTP" and "smtp_port" setting in php.ini or use ini_set() in C:\wamp\www\sms\mail-test.php
...you will have to install and configure a mail server. This is out of the scope of this tutorial. However, if you are working on your local machine, switching to something like XAMPP might solve this problem. Alternatively, try installing Mercury Mail alongside WAMP, MAMP or on a LAMP (or SAMP or OAMP, etc.) system (that's a lot of 'AMPs').
PHPMailer
Another option (which is the method I prefer) is to use PHPMailer. Below is an example of how to use PHPMailer to connect to Gmail's SMTP server and send the message.
Using it is as simple as including a class in your script.
require 'class.phpmailer.php'; // Instantiate Class $mail = new PHPMailer(); // Set up SMTP $mail->IsSMTP(); // Sets up a SMTP connection $mail->SMTPDebug = 2; // This will print debugging info $mail->SMTPAuth = true; // Connection with the SMTP does require authorization $mail->SMTPSecure = "tls"; // Connect using a TLS connection $mail->Host = "smtp.gmail.com"; $mail->Port = 587; $mail->Encoding = '7bit'; // SMS uses 7-bit encoding // Authentication $mail->Username = "[email protected]"; // Login $mail->Password = "password"; // Password // Compose $mail->Subject = "Testing"; // Subject (which isn't required) $mail->Body = "Testing"; // Body of our message // Send To $mail->AddAddress( "##########@vtext.com" ); // Where to send it var_dump( $mail->send() ); // Send!
This should print out something along the lines of:
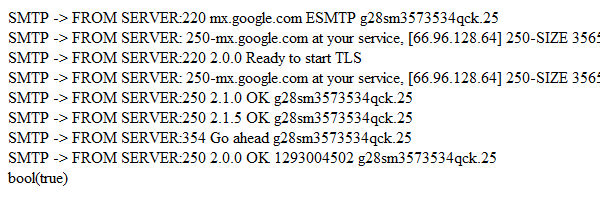
It may take a little more to set up the connection depending on your situation. If you're planning on using Gmail, Google has provided information on connecting.
Conclusion
There are a myriad of methods to accomplish the task of sending a SMS through a web application. This method is really meant for low volume messaging (most likely less than 1,000 text messages per month) and developers looking to get their feet wet without forking out cash. Other options include:
-
Using a SMS Gateway Provider
- Doing a Google search will return plenty of options.
- Most SMS gateway providers include an API for sending messages through a web application.
- You can usually sign up for service for a reasonable price, assuming you are planning on sending at least 1,000 SMS message per month.
- You can rent a short code number.
-
Using a GSM modem
- This can be a costly and slow way to do it, since you have to buy a modem and have a contract with a wireless network
- You will also have to use the AT (Hayes) command set.
- Use a direct connection to a wireless network, which will require some strong negotiating and a whole lot of money.
This tutorial is in no way a comprehensive review of sending tex messages with PHP; but it should get you started! I hope this tutorial has been of interest to you. Thank you so much for reading!
If you still need help with this or any other PHP issue, try contacting one of the experienced PHP developers on Envato Studio.
Comments