I'm a big fan of the WP_Query
class: I use it in many of my client sites to query and output content in custom ways.
If you want to run multiple loops on a page, the easiest way to do it is to run WP_Query
each time you need to run a loop. But there is a downside: every time WordPress runs a query, it's sending requests to the database, which takes time and can slow your site down. And if you're using WP_Query
instead of the main query to output content, then it makes the main query redundant, which is a waste of resources.
So here I'll show you how to use one query to run more than one loop. You can either do this with the main query (which is what I'll do as it's more efficient) or you can use the same technique with WP_Query
.
There are three parts to this:
- Create a child theme and a template file.
- Create a template part for the loop contents.
- Create our loops.
So let's get started!
What You'll Need
To follow along, you'll need:
- A development installation of WordPress.
- A code editor.
- Posts in your site with multiple categories assigned—I'm using the WordPress theme unit test data.
- The twenty sixteen theme installed on your site.
- A child theme of twenty sixteen installed and activated—I'll briefly cover setting up the child theme here.
You don't have to be using twenty sixteen with a child theme—you can adapt this technique for your own theme. But I'll be using a child of twenty sixteen.
Creating the Child Theme
First let's create the child theme of twenty sixteen. I'm doing this because I don't want to edit the twenty sixteen theme itself.
In your wp-content/themes
directory, create a new empty folder. I'm calling mine tutsplus-one-query-two-loops
.
In that folder, create a file called style.css
and add this to it:
/* Theme Name: Tutsplus One Query Multiple Loops Theme URI: http://.tutsplus.com/tutorials/how-to-code-multiple-loops-while-only-querying-the-database-once--cms-25703 Description: Theme to support Tutorial on running multiple loops while querying the database just once. Child theme for the Twenty Sixteen theme. Author: Rachel McCollin Author URI: http://rachelmccollin.co.uk/ Template: twentysixteen Version: 1.0 */ @import url("../twentysixteen/style.css");
Now save that file and activate your new theme.
The next step is to create a template file for categories, which is what we'll be working with.
Make a copy of the archive.php
file from twenty sixteen in your new theme. Don't move it, but copy it. Rename it category.php
. This is now the template file for categories on your site.
Creating a New Template Part File
The first step is to set up a new template part file in our theme which will contain an edited version of the loop from twenty sixteen.
In your theme folder, create a subfolder called includes
. Inside that, create a new file called loop-category.php
.
Now open the template-parts/content.php
file from the twenty sixteen file and find this code (which is most of the file):
<article id="post-<?php the_ID(); ?>" <?php post_class(); ?>> <header class="entry-header"> <?php if ( is_sticky() && is_home() && ! is_paged() ) : ?> <span class="sticky-post"><?php _e( 'Featured', 'twentysixteen' ); ?></span> <?php endif; ?> <?php the_title( sprintf( '<h2 class="entry-title"><a href="%s" rel="bookmark">', esc_url( get_permalink() ) ), '</a></h2>' ); ?> </header><!-- .entry-header --> <?php twentysixteen_excerpt(); ?> <?php twentysixteen_post_thumbnail(); ?> <div class="entry-content"> <?php /* translators: %s: Name of current post */ the_content( sprintf( __( 'Continue reading<span class="screen-reader-text"> "%s"</span>', 'twentysixteen' ), get_the_title() ) ); wp_link_pages( array( 'before' => '<div class="page-links"><span class="page-links-title">' . __( 'Pages:', 'twentysixteen' ) . '</span>', 'after' => '</div>', 'link_before' => '<span>', 'link_after' => '</span>', 'pagelink' => '<span class="screen-reader-text">' . __( 'Page', 'twentysixteen' ) . ' </span>%', 'separator' => '<span class="screen-reader-text">, </span>', ) ); ?> </div><!-- .entry-content --> <footer class="entry-footer"> <?php twentysixteen_entry_meta(); ?> <?php edit_post_link( sprintf( /* translators: %s: Name of current post */ __( 'Edit<span class="screen-reader-text"> "%s"</span>', 'twentysixteen' ), get_the_title() ), '<span class="edit-link">', '</span>' ); ?> </footer><!-- .entry-footer --> </article><!-- #post-## -->
Copy that to your new file.
Editing the Template Part
The loop from twenty sixteen displays more than I need it to for this archive, so I'm going to edit it. I just want to display the excerpt and not the content, so we'll remove that.
In your new loop-category.php
file, find this code and delete it:
<div class="entry-content"> <?php /* translators: %s: Name of current post */ the_content( sprintf( __( 'Continue reading<span class="screen-reader-text"> "%s"</span>', 'twentysixteen' ), get_the_title() ) ); wp_link_pages( array( 'before' => '<div class="page-links"><span class="page-links-title">' . __( 'Pages:', 'twentysixteen' ) . '</span>', 'after' => '</div>', 'link_before' => '<span>', 'link_after' => '</span>', 'pagelink' => '<span class="screen-reader-text">' . __( 'Page', 'twentysixteen' ) . ' </span>%', 'separator' => '<span class="screen-reader-text">, </span>', ) ); ?> </div><!-- .entry-content -->
The other step is to replace the twenty_sixteen_excerpt()
function with the standard the_excerpt()
function, as the twenty sixteen version doesn't include a link to the full post.
Find this line:
<?php twenty_sixteen_excerpt(); ?>
Replace it with this:
<?php the_excerpt(); ?>
We also need to make a few tweaks to the heading tags.
In the template part, edit the line:
<?php the_title( sprintf( '<h2 class="entry-title"><a href="%s" rel="bookmark">', esc_url( get_permalink() ) ), '</a></h2>' ); ?>
Change the h2
tags to h3
:
<?php the_title( sprintf( '<h3 class="entry-title"><a href="%s" rel="bookmark">', esc_url( get_permalink() ) ), '</a></h3>' ); ?>
Save your template part file. Now go back to your category.php
file and continue working in that.
Creating Our Loops
First let's remove the call to the twenty sixteen template part from our category.php file, as we'll need to use the new file instead.
In your category.php
file find this code:
get_template_part( 'template-parts/content', get_post_format() );
Delete that.
Now we'll create the loops.
In this example I'm going to list all posts with the 'content' tag first, using the has_tag()
conditional tag.
This means I'll have to run three loops:
- The first checks if the query has returned any posts with this tag.
- If so, the second outputs posts with this tag.
- A third outputs posts without this tag.
Between each of these, I'll use rewind_posts()
to rewind the posts without resetting the query: we're still working with the main query each time.
The First Loop: Checking for Posts
In your category.php
file, find the start of the loop:
while ( have_posts() ) : the_post();
Above that line, define a new variable called $count
:
$count = 0;
Now inside that loop, add this code:
// check if there are any posts with the '' tag $tag = 'content'; if ( has_tag( $tag ) ) { $count +=1; }
This checks if posts have the 'content' tag and then adds 1
to the count if so.
Your loop will now look like this:
// Check for posts in the first loop. $count = 0; while ( have_posts() ) : the_post(); // check if there are any posts with the '' tag $tag = 'content'; if ( has_tag( $tag ) ) { $count +=1; } endwhile;
The Second Loop: Outputting Posts With the Tag
The next step is to run a loop to output posts with that tag, but only if there are any, i.e. if the value of $count
is greater than 0
.
Add this below your first loop:
if ( $count > 0 ) { rewind_posts(); echo '<h2>Posts tagged with ' . $tag . '</h2>'; while ( have_posts() ) : the_post(); if ( has_tag( $tag ) ) { get_template_part( 'includes/loop', 'category'); } // End the loop. endwhile; }
This checks that $count
is greater than zero and if so, rewinds posts and runs the loop again. For each post it checks if the post has our tag and, if so, it calls the template part we just created.
The Third Loop: Outputting the Rest of Our Posts
The final loop will output the remaining posts. If this category didn't have any posts with the 'content' tag, then it will output all posts in the category.
Below your second loop, add this:
rewind_posts(); // Second Loop - posts not with the 'content' tag while ( have_posts() ) : the_post(); if ( !has_tag( $tag ) ) { get_template_part( 'includes/loop', 'category'); } // End the loop. endwhile; ?>
This rewinds posts (which you'll be doing for the first time if there weren't any posts with the tag or the second time if there were), and then runs the loop again. This time it checks if a post doesn't have the 'content' tag and then outputs it using our template part.
The Finished Archive
Now test the category archive page on your site.
If you're using the WordPress test unit data like me, you'll find that the 'Markup' category has posts with the 'content' tag. Here's the category archive page for the 'Markup' category on my site:
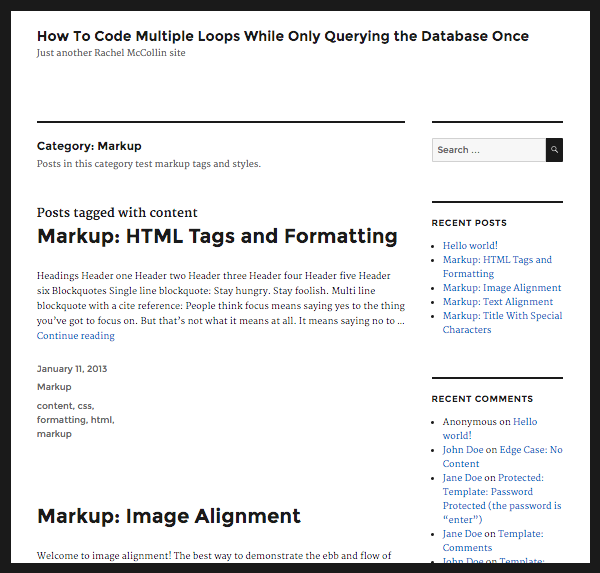
Summary
Running multiple loops from one query isn't complicated. Instead of resetting the query and creating a new one, you use the rewind_posts()
function to rewind the query and run it again. And instead of defining new query parameters, you use conditional tags to specify which posts are output.
Important note: Don't be tempted to use query_posts()
to alter the main query when you're doing this. This will slow your site down more than if you'd used multiple queries.
In this example we've run two loops based on the main query, which save using WP_Query
to run two additional queries and will reduce server load. You could apply this technique with a query you define using WP_Query
or with the main query on other archive pages, by editing the appropriate template file.
Comments