In this tutorial, you'll see how to get started with setting up and using a fake REST API server using json-server, which you can use while developing mobile or web applications. This tutorial assumes that you have a basic knowledge of JSON and HTTP requests.
What Is REST?
REST stands for Representational State Transfer. It is an architecture style for designing connected applications. It uses simple HTTP to make communication between machines possible. So, instead of using a URL for manipulating some user information, REST sends an HTTP request like GET, POST, DELETE, etc. to a URL to manipulate data.
For example, instead of making a GET request to a URL like /deleteuser?id=10
, the request would be like DELETE /user/10
.
Why Do We Need a Fake REST API?
REST APIs form the back end for mobile and web applications. When developing applications, sometimes you won't have the REST APIs ready to be used for development purposes. To see the mobile or web app in action, we require a server which throws in some dummy JSON data.
That's when the fake REST API comes into the picture. json-server
provides the functionality to set up a fake REST API server with minimum effort.
Getting Started
To get started with using json-server
, install the package using Node Package Manager (npm).
npm install -g json-server
Create a dummy JSON file with some data as per your requirement. For example, I need some JSON data with user information like id, name, location, etc. So I'll create a file called info.json
with the following JSON information:
{ "users": [{ "id": 1, "name": "roy", "location": "india" }, { "id": 2, "name": "sam", "location": "wales" }] }
From the terminal, run the json server with info.json
as data source and you should have the REST API running at http://localhost:3000.
json-server info.json
Testing the REST API Endpoints
Since our fake REST API server is up and running, let's see how to access the REST API using a client. I'm using Postman REST client to make the API calls.
GET Request
Let's start by making a GET
request to the REST URL. Inside the json file, we have defined an endpoint users
which contains information related to users. On making a GET
request to the URL http://localhost:3000/users, it should display the existing data.
[ { "id": 1, "name": "roy", "location": "india" }, { "id": 2, "name": "sam", "location": "wales" } ]
POST Request
In order to add new data to the existing data, we'll make a POST
request to the URL http://localhost:3000/users. Here's what the POST
request would look like:
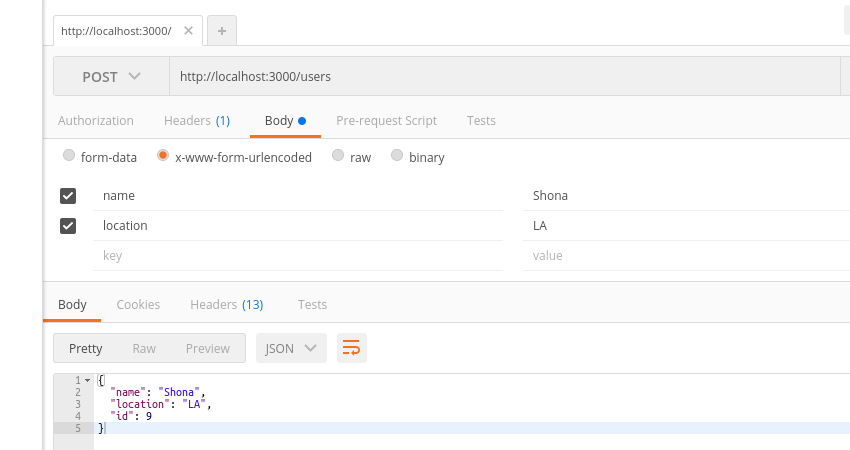
Try doing a GET
request and you should have the newly added data in the info.json
file.
[ { "id": 1, "name": "roy", "location": "india" }, { "id": 2, "name": "sam", "location": "wales" }, { "name": "ii", "location": "la", "id": 7 }, { "name": "Shona", "location": "LA", "id": 8 }, { "name": "Shona", "location": "LA", "id": 9 } ]
DELETE Request
To delete an entry from the json-server
data, you need to send a DELETE
request to the API endpoint with the user Id. For example, to delete the user with Id 1, you need to send a DELETE
request to the endpoint http://localhost:3000/users/1. Once it's deleted, try doing a GET
request, and the user with Id 1 should not be in the returned JSON.
PATCH Request
To update an existing entry, you need to send a PATCH
request with the details that need to be updated for that particular entry. For example, in order to update the details for user with Id 2, we'll send a PATCH
request to the URL http://localhost:3000/users/2 as shown:
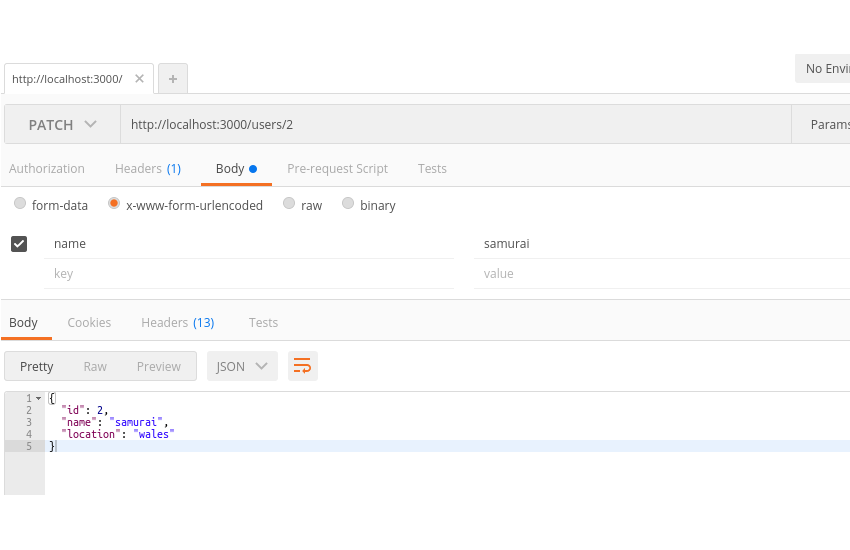
Searching json-server REST APIs
Using json-server
REST APIs, you can search through the data to find data based on certain criteria. For example, in order to find users with a particular name, you need to send a GET request to the REST API URL as shown:
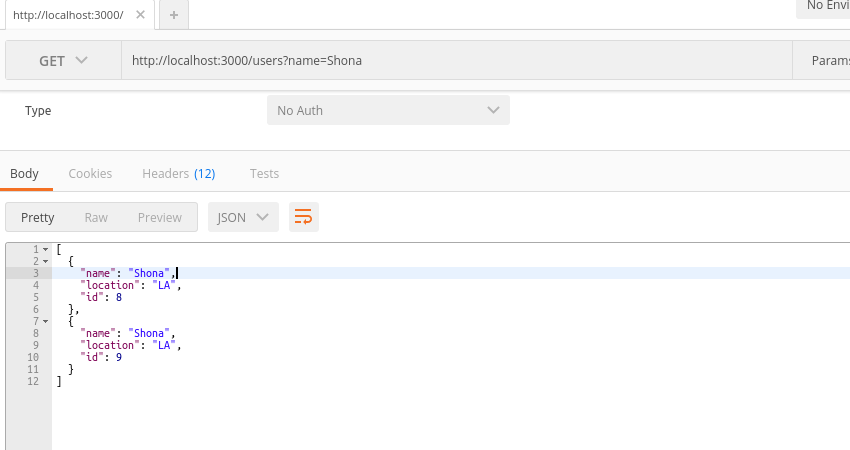
As seen in the above image, sending a GET request to the URL http://localhost:3000/users?name=Shona would return the users with name Shona
. Similarly, to search for users with any other fields, you need to add that field to the query string.
In order to execute a full text search across the REST API endpoint, you need to add the search string along with the parameter q
to the endpoint. For example, in order to search for users with info containing search string s
, the request would look like:
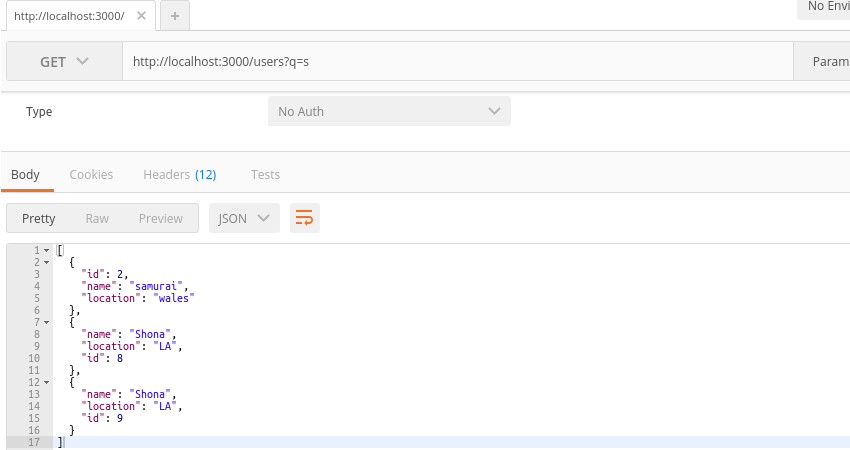
Handling Pagination
While displaying a paginated data grid, it would be required to fetch some data based on pagination. In such scenarios, json-server
provides the functionality to paginate the JSON data. By default, the count of data returned from json-server
is 10. We can explicitly define this limit using the _limit
parameter.
http://localhost:3000/users?_limit=5
A GET request to the above URL would return five records. Now, to paginate the data, we need to add another parameter _page
to the URL. _page
defines the page which needs to be fetched on returning the data.
http://localhost:3000/users?_limit=5&_page=2
A GET request to the above URL would return the second page of the data set with five records per page. By changing the _page
variable, we can fetch the required page records.
Handling Sorting
json-server
provides the functionality to sort the retrieved data. We can sort the data by providing the column name which needs to be sorted and the order in which the data needs to be sorted. By default, the data is sorted in ascending order. We can provide the column name in the endpoint URL using the keyword _sort
and define the order using the keyword _order
. Here is an example URL:
http://localhost:3000/users?_sort=id&_order=DESC
The above URL would sort the data based on the column Id
, and it would be sorted in descending order.
Handling Operators
json-server
also provides the functionality to support operators like finding an entry with Id in range between two values or an entry matching a particular regular expression.
For finding an entry within a particular range, we can make use of the _gte
and _lte
operators. For example, to find users with Id greater than 1 and less than 2, make a GET request to the URL http://localhost:3000/users?id_gte=1&id_lte=2 as shown:
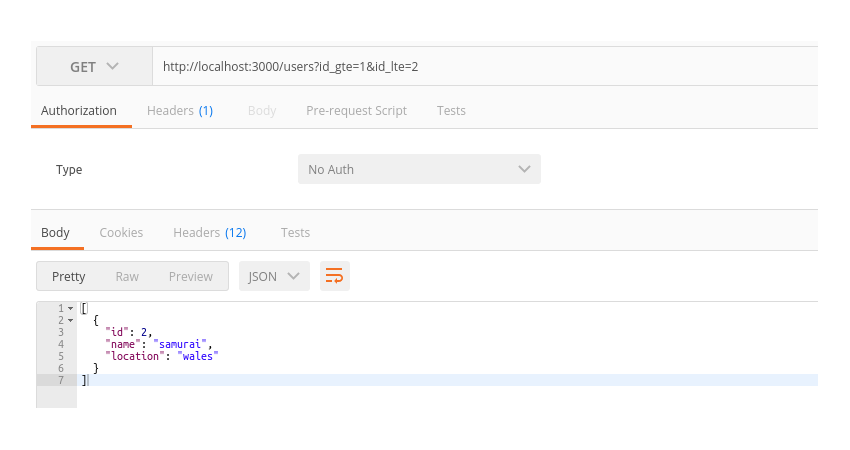
If we want to search for names starting with a certain letter, we can use regular expressions. For example, to search for names starting with letters sa
, we'll make use of the _like
operator and make a GET
request to the URL http://localhost:3000/users?name_like=^sa.
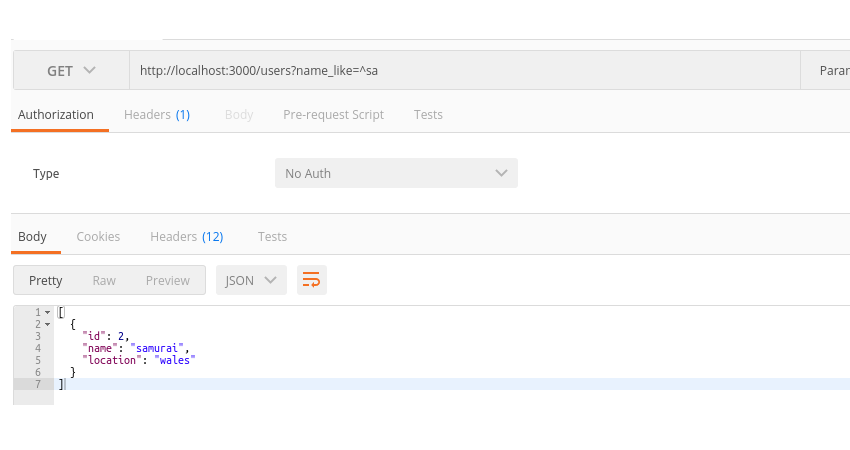
Conclusion
In this tutorial, you saw how to use json-server
REST APIs to create a dummy database for quick use. You learnt how to get started with using json-server
and query the URL to add, update, modify and delete data. You saw how to paginate, sort and search the dummy data. You also saw how to use operators to search for entries using regular expressions.
Have you used json-server
or any other fake REST API server for dummy data creation? How was your experience? Do let us know your thoughts in the comments below.
Comments