Creating your own theme for WordPress is a great way to give your blog or other WordPress powered web site an original touch. But even the nicest looking theme is not that nice if you have to get under the hood and edit the theme's HTML or PHP code whenever it's time change some aspects of it. Especially, when it's not you but a paying customer using your theme. Luckily, creating a settings page for your theme in WordPress is not very hard, and after reading this tutorial, you will be able to create one in no time!
Step 1 Deciding What Settings Are Needed
It all starts from the need: to create a clear and useful settings page, you have to figure out the things that will need to be changed and leave out everything else. Every new setting you add to the admin menus adds complexity to the user interface and risks making the theme harder to use. That's why it's better to be careful and handpick the options that are going to be changed often and leave out one time customizations that can easily be done changing one file inside the theme.
Another question to keep in mind is "Who is going to be changing these settings?" If the user is familiar with PHP and WordPress, it might be reasonable to expect that she is OK with embedding her Google Analytics code in the code herself, but you shouldn't require that from a graphic designer, not to mention a writer who doesn't even need to know anything about HTML and CSS.
Common ideas for things to define in theme settings include:
- The site's Google Analytics tracking code
- The number of sidebars and their positioning (left, right, maybe even up and down)
- Page width
- The contents of your footer
- Options for features that are specific to the theme, such as custom teaser formats.
Once you have collected the list of theme features that you'd like to control through a settings page, you are almost ready to start the implementation. Before you move on and create your settings page, you can save time by making sure that there isn't a WordPress feature already available for the customization you have in mind. Widgets, custom menus, custom backgrounds and header images are all useful tools for making your theme customizable with a lot less work than required for creating your own settings. They are, however, topics for a different tutorial.
Settings Created in This Tutorial
For this tutorial, I dreamed up a theme front page that consists of a grid with a varying number of featured posts that can be picked, edited and reordered by the admin using a custom settings page.
In the editor, the front page elements will be presented as a list of elements to which new ones can be added using JavaScript and jQuery.
I like to be able to preview the admin page in the WordPress admin as I design the HTML, so I usually start by linking a settings page to WordPress, and only then move to designing the contents of the page. That's why, our next step is creating a placeholder settings page and hooking it to WordPress.
Step 2 Hooking the Settings Page to WordPress
Creating a settings page starts by creating a function that sets up the menu and hooking it to the WordPress action admin_menu
. This tells WordPress to call your function when its time to create the menus so that everything is done at its proper time. Add this code to your theme's functions.php
file:
function setup_theme_admin_menus() { // We will write the function contents very soon. } // This tells WordPress to call the function named "setup_theme_admin_menus" // when it's time to create the menu pages. add_action("admin_menu", "setup_theme_admin_menus");
We'll now put the code for creating the settings pages inside the function we just created.
When creating your settings page, you have the choice of either adding the page as a submenu to one of the existing settings groups or of creating your own top level menu.
Adding a submenu is done with the function add_submenu_page
:
<?php add_submenu_page($parent_slug, $page_title, $menu_title, $capability, $menu_slug, $function) ?>
-
$parent_slug
is a unique identifier for the top menu page to which this submenu is added as a child. -
$page_title
is the title of the page to be added -
$menu_title
is the title shown in the menu (often a shorter version of$page_title
-
$capability
is the minimum capability required from a user in order to have access to this menu. -
$menu_slug
is a unique identifier for the menu being created -
$function
is the name of a function that is called to handle (and render) this menu page
If you choose to add the menu page as a submenu to one of the WordPress groups, you can use the following values as the $parent_slug
parameter:
- Dashboard:
index.php
- Posts:
edit.php
- Media:
upload.php
- Links:
link-manager.php
- Pages:
edit.php?post_type=page
- Comments:
edit-comments.php
- Appearance:
themes.php
- Plugins:
plugins.php
- Users:
users.php
- Tools:
tools.php
- Settings:
options-general.php
The Appearance group looks like a good candidate for placing our settings page. Let's try that, and create our first settings page. Here's an updated version of our menu setup function:
function setup_theme_admin_menus() { add_submenu_page('themes.php', 'Front Page Elements', 'Front Page', 'manage_options', 'front-page-elements', 'theme_front_page_settings'); }
We still need to create the function theme_front_page_settings
for this to work. Here it is in its simplest form:
function theme_front_page_settings() { echo "Hello, world!"; }
And this is how it looks in action:
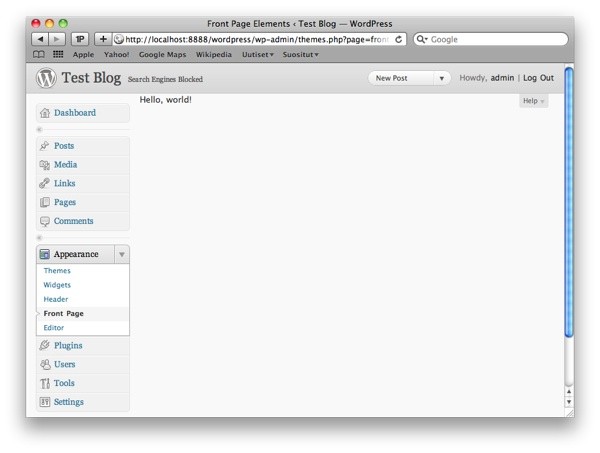
We also need to check that the user has the rights required for editing the settings page. To do that, add the following code at the beginning of the settings page function:
// Check that the user is allowed to update options if (!current_user_can('manage_options')) { wp_die('You do not have sufficient permissions to access this page.'); }
Now, if a user who isn't allowed to manage options comes to the settings page, she will see nothing but the message, "You do not have sufficient permissions to access this page."
If your theme needs multiple settings pages, it can be confusing for the user to look for them scattered all around the menu structure. In that case, creating your own settings group makes it easier for the theme user to find all the menu pages for the theme.
To add your own settings group, you need to create a top level menu page and link the submenu pages to it. Here is a new version of our menu setup function. The add_menu_page
function used to create the top level menu is similar to add_submenu_page
except that it doesn't take the $parent_slug
parameter.
function setup_theme_admin_menus() { add_menu_page('Theme settings', 'Example theme', 'manage_options', 'tut_theme_settings', 'theme_settings_page'); add_submenu_page('tut_theme_settings', 'Front Page Elements', 'Front Page', 'manage_options', 'front-page-elements', 'theme_front_page_settings'); } // We also need to add the handler function for the top level menu function theme_settings_page() { echo "Settings page"; }
If you test the code and refresh the WordPress admin, you'll see your new menu group appear at the bottom of the menu list:
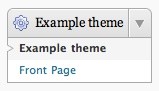
But something doesn't look quite right yet. Clicking the top menu element doesn't lead you to the "Front Page" menu but a menu page called "Example theme." This is not consistent with how the other WordPress menus function, so let's do one more thing: by changing the $menu_slug
attribute in the add_submenu_page
call to the same value as in the top level menu, we can link the two menus so that selecting the top menu selects the front page menu:
function setup_theme_admin_menus() { add_menu_page('Theme settings', 'Example theme', 'manage_options', 'tut_theme_settings', 'theme_settings_page'); add_submenu_page('tut_theme_settings', 'Front Page Elements', 'Front Page', 'manage_options', 'tut_theme_settings', 'theme_front_page_settings'); } function theme_settings_page() { }
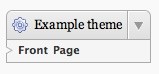
Looks better. If you want to still improve the looks of your menu group, there are two optional fields in the add_menu_page
function that you will find useful. Just add the values after the function name in the method call:
-
$icon_url
specifies the URL of an icon for the top level menu. -
$position
specifies the position of your menu group in the menu list. The higher the value, the lower the position in the menu.
Step 3 Creating the HTML Form For the Settings Pages
Now that we have created the settings page, and it shows up nicely in the side menu, it's time to start adding some content. So, let's go back to the list of settings we had in mind, and draft a page for editing them.
In this tutorial, we need a field for defining how many elements should be listed on one row, and a list for defining the actual elements. To start from the easier, let's create a text field for the number of elements on one row. Edit your settings page function:
function theme_front_page_settings() { ?> <label for="num_elements"> Number of elements on a row: </label> <input type="text" name="num_elements" /> <?php }
When you reload your settings page, you'll see the first settings field appear:
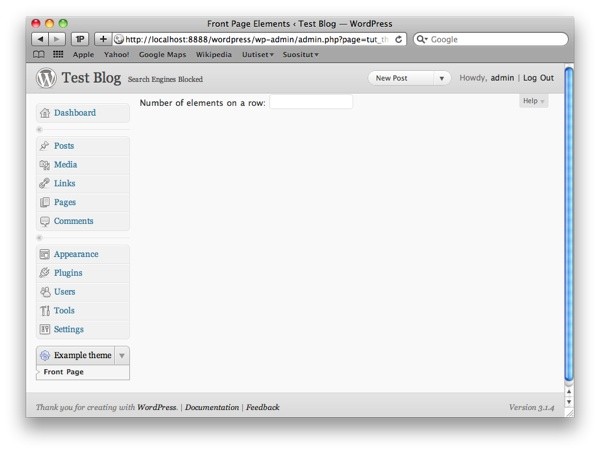
To make the settings page fit seamlessly in the WordPress experience and to give your plugin a professional touch, it's a best practice to use the CSS classes and styles that WordPress uses in its own settings pages. A good way to learn the tricks is to just go ahead and analyze the WordPress source code.
The most important thing is to wrap your setting page with a div
with the class "wrap"
. Within that div
element, you can use many predefined styles such as headings, buttons, and form fields. Let's start by styling the title of our settings page:
- We will create a
h2
heading for the page (You can use the heading tags fromh2
toh6
to create headings with different sizes.) - We will show the theme settings page icon before the heading. (You can use the predefined WordPress icons with the
screen_icon
function. The function can take one of the following parameters:index
,edit
,upload
,link-manager
,pages
,comments
,themes
,plugins
,users
,tools
oroptions-general
.) - We will put the
input
element inside a form and a table with the classform-table
.
function theme_front_page_settings() { ?> <div class="wrap"> <?php screen_icon('themes'); ?> <h2>Front page elements</h2> <form method="POST" action=""> <table class="form-table"> <tr valign="top"> <th scope="row"> <label for="num_elements"> Number of elements on a row: </label> </th> <td> <input type="text" name="num_elements" size="25" /> </td> </tr> </table> </form> </div> <?php }
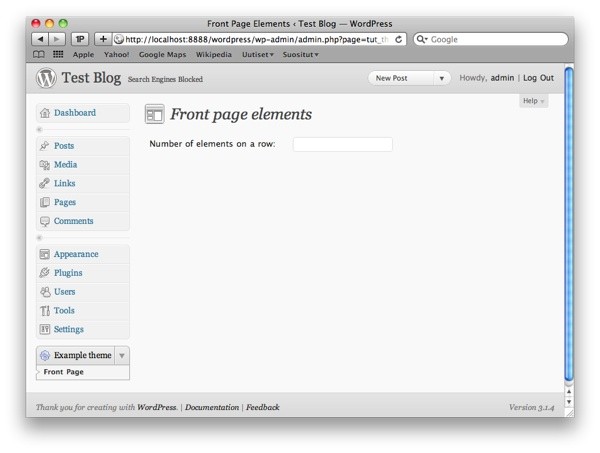
Next, it's time to start adding the elements.
To do this, we'll use jQuery as it makes things much easier than writing JavaScript by from scratch, and comes bundled with WordPress. If you have used jQuery before, there is just one thing to keep in mind: the $
notation that you would normally use with jQuery doesn't work in WordPress -- you have to type the whole word, jQuery
instead.
First, we'll create the element for editing the settings for one main page block to serve as a template for the elements that are added by the user. Add this code right between the closing table tag and the closing form tag right after it.
<?php $posts = get_posts(); ?> <li class="front-page-element" id="front-page-element-placeholder"> <label for="element-page-id">Featured post:</label> <select name="element-page-id"> <?php foreach ($posts as $post) : ?> <option value="<?php echo $post-<ID; ?>"> <?php echo $post-<post_title; ?> </option> <?php endforeach; ?> </select> <a href="#">Remove</a> </li>
Now, it looks like this:
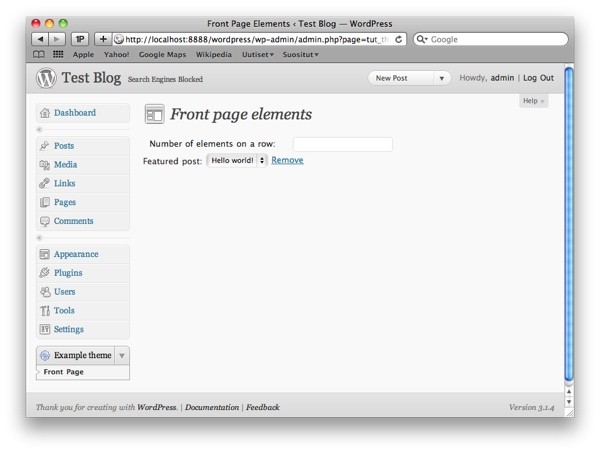
Now that we have our template, it's time to hide it and create the JavaScript for using it to create new featured post rows to the settings page. Set the style for the li
element above to display:none;
<li class="front-page-element" id="front-page-element-placeholder" style="display:none">
Then, we'll create a list for holding the front page elements as they are added, and a link that the user will click to add the new elements. I'm repeating the entire HTML so that you can clearly see where the changes go:
<div class="wrap"> <?php screen_icon('themes'); ?> <h2>Front page elements</h2> <form method="POST" action=""> <table class="form-table"> <tr valign="top"> <th scope="row"> <label for="num_elements"> Number of elements on a row: </label> </th> <td> <input type="text" name="num_elements" size="25" /> </td> </tr> </table> <h3>Featured posts</h3> <ul id="featured-posts-list"> </ul> <input type="hidden" name="element-max-id" /> <a href="#" id="add-featured-post">Add featured post</a> </form> <li class="front-page-element" id="front-page-element-placeholder" style="display:none;"> <label for="element-page-id">Featured post:</label> <select name="element-page-id"> <?php foreach ($posts as $post) : ?> <option value="<?php echo $post->ID; ?>"> <?php echo $post->post_title; ?> </option> <?php endforeach; ?> </select> <a href="#">Remove</a> </li> </div>
In a real-life theme it's a good practice to put your JavaScript code in a separate file, but to make this tutorial a bit easier to follow, I am now adding the JavaScript in the same function with the HTML above, right before the wrap
div:
<script type="text/javascript"> var elementCounter = 0; jQuery(document).ready(function() { jQuery("#add-featured-post").click(function() { var elementRow = jQuery("#front-page-element-placeholder").clone(); var newId = "front-page-element-" + elementCounter; elementRow.attr("id", newId); elementRow.show(); var inputField = jQuery("select", elementRow); inputField.attr("name", "element-page-id-" + elementCounter); var labelField = jQuery("label", elementRow); labelField.attr("for", "element-page-id-" + elementCounter); elementCounter++; jQuery("input[name=element-max-id]").val(elementCounter); jQuery("#featured-posts-list").append(elementRow); return false; }); }); </script>
The JavaScript code above creates a function that is called when the user clicks the link with id add-featured-post
. This function clones the template list item we created earlier and updates its fields to have unique ids and names. This way they will all be properly sent with the form when the user clicks submit. The variable elementCounter
contains the next id to add. It is also saved in a hidden field so that when the form is submitted, we know how many front page elements to expect.
If you click the "Add featured post" link a couple of times, you'll see hownew elements are added to the list:
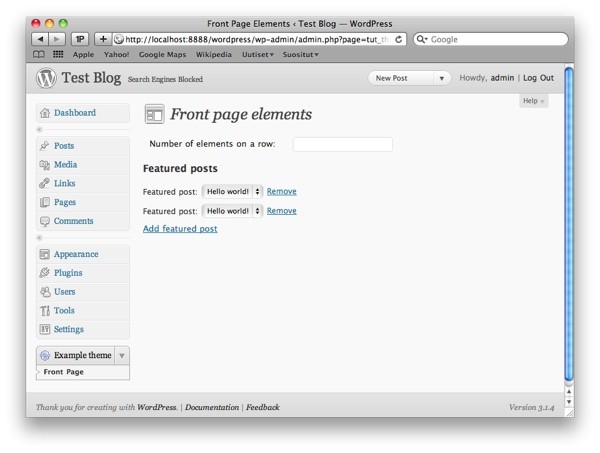
But when you click on the remove link, you'll notice that nothing happens. Let's add a function for removing elements from the list:
function removeElement(element) { jQuery(element).remove(); }
We also need to call to the function. Add the following code right before incrementing elementCounter
.
var removeLink = jQuery("a", elementRow).click(function() { removeElement(elementRow); return false; });
Before moving on to saving the form, there is one more thing to do. We'll use the ui.sortable jQuery plugin to make the front page elements sortable by dragging them on the page. To enable the sorting functionality, we'll need to include the proper JavaScript file (which also comes bundled with WordPress). This can be done by adding the following line of code at the end of functions.php
:
if (is_admin()) { wp_enqueue_script('jquery-ui-sortable'); }
Then, we'll add the following JavaScript right before (or after) the jQuery("#add-featured-post").click
function defined above.
jQuery("#featured-posts-list").sortable( { stop: function(event, ui) { var i = 0; jQuery("li", this).each(function() { setElementId(this, i); i++; }); elementCounter = i; jQuery("input[name=element-max-id]").val(elementCounter); } });
This snippet makes the list sortable and adds an event that is called whenever the user finishes sorting. The event handler updates all the ids in the elements so that the new order is preserved also when saving the form (this will become clearer once we implement the saving). When writing this stop handler, I noticed that the code for setting the id for the contents of the template was duplicated in two places so I refactored it into its own function, which I placed right before theline with jQuery(document).ready()
:
function setElementId(element, id) { var newId = "front-page-element-" + id; jQuery(element).attr("id", newId); var inputField = jQuery("select", element); inputField.attr("name", "element-page-id-" + id); var labelField = jQuery("label", element); labelField.attr("for", "element-page-id-" + id); }
With adding new elements, sorting them, and removing them working, it's time to move on to saving the data. But before that, add a submit button right before the form's closing tag.
<p> <input type="submit" value="Save settings" class="button-primary"/> </p>
Step 4 Saving the Form
The settings page looks good, but there is something missing: it doesn't do anything yet. It's time to save some data. WordPress provides an easy system for saving theme and plugin settings as key value pairs to the database using two functions: get_option
and update_option
. The data stored using the functions can be as simple as a number value or as complex as an array nested multiple times.
The handling of the form is done in the same function that renders the form. To know whether a form was submitted or not, we add a hidden field, update_settings
to the form and then check whether that field was sent or not in the handling function.
if (isset($_POST["update_settings"])) { // Do the saving }
The hidden field that goes inside the form looks like this:
<input type="hidden" name="update_settings" value="Y" />
Let's start by saving the easier setting, num_elements
. We'll escape the attribute to make sure the user isn't sending malicious content in the from of HTML tags and then save it to the WordPress settings storage. When using update_option
, we don't need to worry about whether the setting has already been saved or not.
$num_elements = esc_attr($_POST["num_elements"]); update_option("theme_name_num_elements", $num_elements);
Before we move to saving the list, let's add the current value of num_elements
to the settings form so that the user always sees what value she has entered in before deciding the next value. This will also help us test that the value was actually saved.
<input type="text" name="num_elements" value="<?php echo $num_elements;?>" size="25" />
And for cases where we haven't saved anything yet, we'll need to load the current value from options, so let's add this piece of code to be executed when there is no form submitted.
$num_elements = get_option("theme_name_num_elements");
When a form is saved, it's important to notify the user so that she isn't left wondering whether something happened or not. So, let's render a simple notice saying "Settings saved." right after the update_option
:
?> <div id="message" class="updated">Settings saved</div> <?php
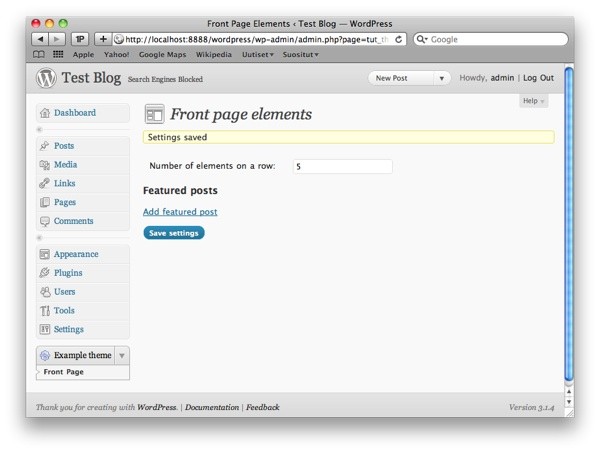
Then, let's save the front page elements. The highest id value in the front page elements is passed in as element-max-id
, so we can take that value and loop through elements up to that id, saving their data into an array in the correct order:
$front_page_elements = array(); $max_id = esc_attr($_POST["element-max-id"]); for ($i = 0; $i < $max_id; $i ++) { $field_name = "element-page-id-" . $i; if (isset($_POST[$field_name])) { $front_page_elements[] = esc_attr($_POST[$field_name]); } } update_option("theme_name_front_page_elements", $front_page_elements);
This saves the data, but we still need to present the values on the settings page. So, let's do the same as with the num_elements
field and load the default options in the beginning of the function:
$front_page_elements = get_option("theme_name_front_page_elements");
And then, render the existing elements when doing the form:
<?php $element_counter = 0; foreach ($front_page_elements as $element) : ?> <li class="front-page-element" id="front-page-element-<?php echo $element_counter; ?>"> <label for="element-page-id-<?php $element_counter; ?>">Featured post:</label> <select name="element-page-id-<?php $element_counter; ?>"> <?php foreach ($posts as $post) : ?> <?php $selected = ($post->ID == $element) ? "selected" : ""; ?> <option value="<?php echo $post->ID; ?>" <?php echo $selected; ?>> <?php echo $post->post_title; ?> </option> <?php endforeach; ?> </select> <a href="#" onclick="removeElement(jQuery(this).closest('.front-page-element'));">Remove</a> </li> <?php $element_counter++; endforeach; ?>
We also need to set the initial value for the elementCounter
variable used in JavaScript by setting the hidden field's initial value in PHP and reading it in when initializing the JavaScript variable:
<input type="hidden" name="element-max-id" value="<?php echo $element_counter; ?>" />
And the JavaScript part:
var elementCounter = jQuery("input[name=element-max-id]").val();
Step 5 Using the Settings Inside the Theme
Saving and showing settings values within the admin area is great, but what really counts is how you use them to customize your theme, so now, we have come to the point where it's time to take our settings and do something cool with them.
From here on, changes go to index.php
instead of functions.php
. First, we'll read the options to variables:
<?php $num_elements = get_option("theme_name_num_elements"); $elements = get_option("theme_name_front_page_elements"); ?>
Let's loop through the $elements
list, grouping them into rows with $num_elements
blocks on each.
<div id="front-page-element-container"> <div class="front-page-element-row"> <?php foreach($elements as $post_id) : ?> <?php if ($num == $num_elements) : ?> </div> <div class="front-page-element-row"> <?php endif; ?> <!-- Render element here --> <?php endforeach; ?> </div> </div>
And then, using the data saved for each element, we'll fill in the element rendering part above:
<?php $element_post = get_post($post_id); ?> <div class="front-page-element"> <div class="thumbnail-image"> <?php if (has_post_thumbnail($post_id)) : ?> <?php echo get_the_post_thumbnail($post_id, 'tutorial-thumb-size'); ?> <?php endif; ?> <a class="title" href="<?php echo get_permalink($post_id); ?>"><?php echo $element_post->post_title;?></a> </div> </div>
With a couple elements, it looks like this:
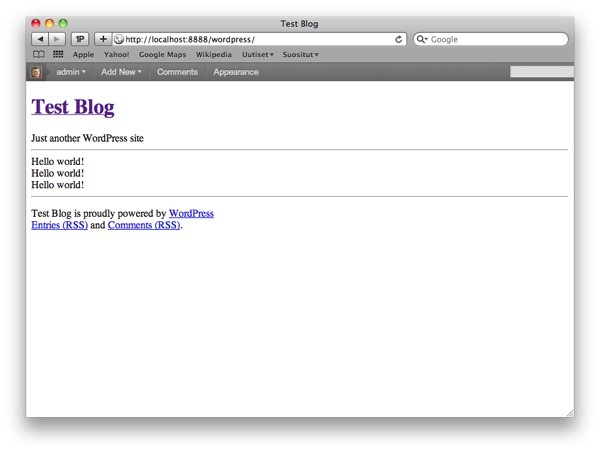
Still quite boring. The posts don't have thumbnail images and there is no styling for them. To make them look better, let's first add support for post thumbnail images. This is done by hooking a new function that sets up theme features to be called right after the theme has been loaded
function setup_theme_features() { if (function_exists('add_theme_support')) { add_theme_support('post-thumbnails'); } if (function_exists("add_image_size")) { add_image_size('tutorial-thumb-size', 200, 200, true); } } add_action('after_setup_theme', 'setup_theme_features');
The function, setup_theme_features
turns on the post thumbnails using WordPress function add_theme_support
so that WordPress adds this functionality to the post saving page. On the post's page, we can now add one image as thumbnail by clicking on "Use as featured image" on the image upload page after uploading the photo.
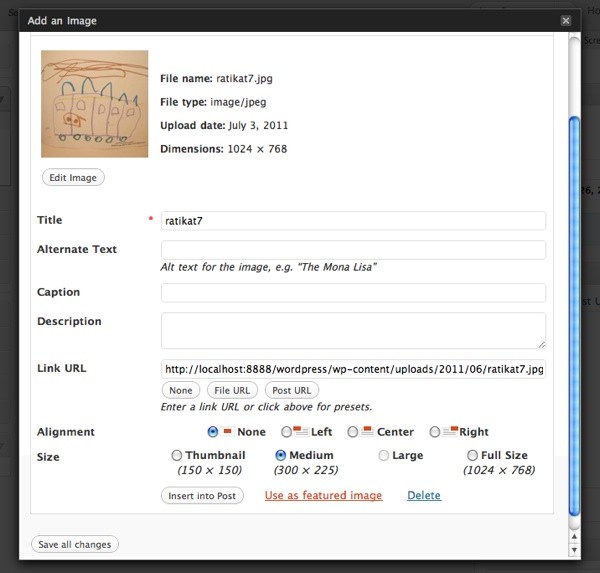
The function also defines a new image size type, tutorial-thumb-size
which is used when getting the post thumbnail in the rendering code.
After selecting the featured image, save the changes and reload the front page. Looks more interesting already:
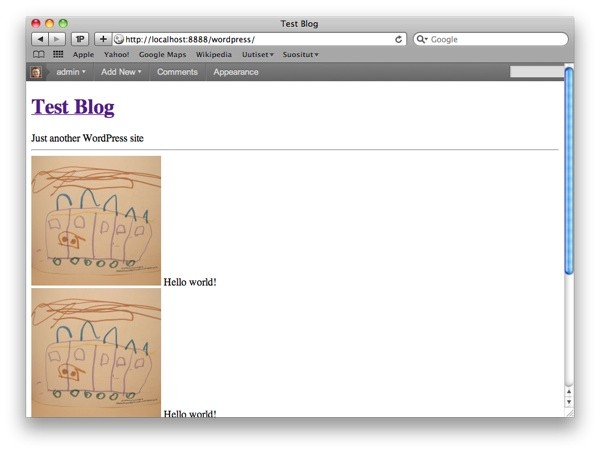
Finally, we'll add a few styles to style.css
, and there we go, the theme has a configurable featured posts display:
.front-page-element-row { overflow: auto; } .front-page-element { float: left; margin: 10px 10px 10px 10px; padding: 0px; width: 200px; height: 200px; } .thumbnail-image { width: 200px; height: 200px; background: #eee; position: relative; } .thumbnail-image .title { position: absolute; bottom: 20px; display: block; background: #000; color: #fff; padding: 10px; font-family: Arial; font-size: 12pt; text-decoration: none; }
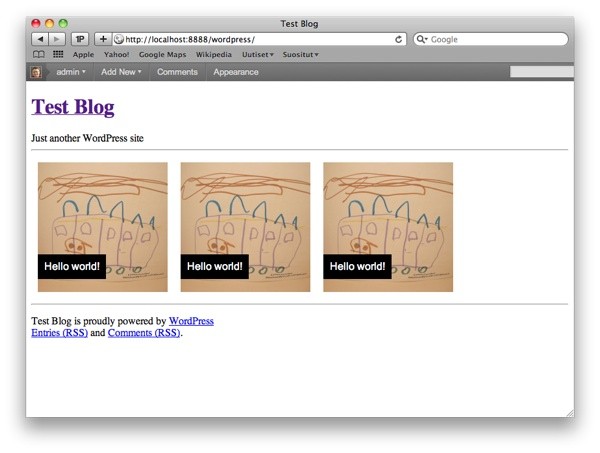
Conclusion
Now, we have created a settings page for a custom theme. The theme is far from complete, but I hope this introduction got you started with adding settings and customizable elements to your next WordPress theme.
Comments