This tutorial will teach you how to use the Cocos2D iOS framework in order to create simple yet advanced 2D games aimed at all iOS devices. It's designed for both novice and advanced users. Along the way, you'll learn about the Cocos2D core concepts, touch interaction, menus and transitions, actions, particles, and collisions. Read on!
The Cocos2D tutorial will be divided into three parts in order to completely cover each section. After the three part tutorial, you'll be able to create an interesting 2D game using Cocos2D!
Each part will produce a practical result, and the sum of all parts will produce the final game. Despite the fact that each part can be read independently, for better understanding and guidance we suggest that the tutorial be followed step by step. We've also included the source code for each part separately, providing a way to start the tutorial at any point of the tutorial without having to read the prior portions.
Organization of the Series:
Before we start the tutorial, we'd like to thank Rodrigo Bellão for providing us with the images of the monsters.
1. Installation and Basics
If this is your first time working with Cocos2D, you'll need to download and install the SDK from the Cocos2D for iPhone Webpage or from the GitHub Repository. Once you've downloaded it, you'll need to install the Xcode project templates. Unzip the package and open up a terminal window on the directory you downloaded Cocos2D to.
Enter the following command.
./install-templates.sh -f -u
This command will only work if Xcode is installed within the default directory.
2. Monster Smashing!
Now we have Cocos2D installed. To start our game, we need to launch Xcode and go to File Menu > New > Project. We'll see something like the following image.
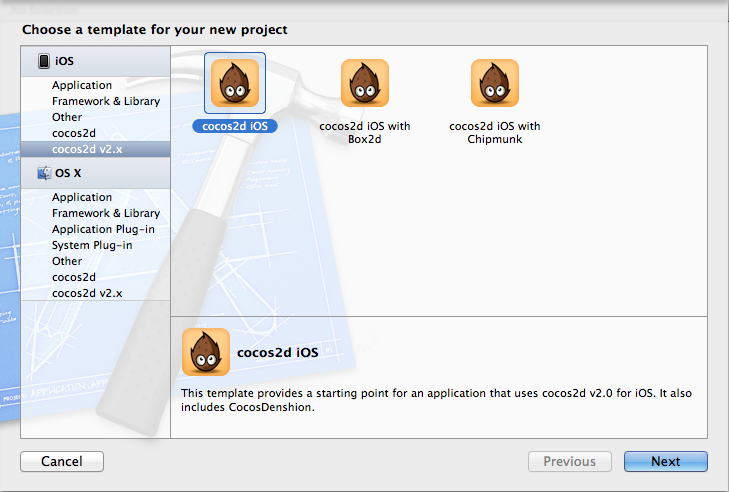
We need to choose the "Cocos2D iOS" template (from the Cocos2D v2.x lateral list). This template creates what we need to start our project, and includes the libraries required to run Cocos2D. The project is created with three classes (AppDelegate, HelloWorldLayer, and IntroLayer). Today, you will be working with HelloWorldLayer.
3. Pixel and Point Notation
A pixel is the smallest controllable element of a picture represented on the screen. There are several display resolutions in the iDevice family. Thus, the Cocos2D framework introduces a notation to help developers positioning objects in the screen. This is called point notation.
The device coordinate space is normally measured in pixels. However, the logical coordinate space is measured in points. The next table presents the direct relation between devices, points, and pixels.
Device | Points | Pixel |
iPhone 3G | 480x320 | 480x320 |
iPhone Retina | 480x320 | 960x640 |
iPad 3 | 1024x768 | 2048x1536 |
From Cocos2D version 0.99.5-rc0 and above, the user can place objects in the screen using only point notation. This will offer a great advantage since the user knows that the object placement will be the same despite the end device where the application will run.
Nevertheless, if the user wants to, it's possible to place objects using pixel notation simply by modifying the type of message that we pass to the object.
// Director CCDirector *director [CCDirector sharedDirector]; CGSize objectA = [director winSize]; // points notation CGSize objectB = [director winSizeInPixels]; // pixel notation // Node CCNode *node = [...]; CGPoint posA = [node position]; // points notation CGPoint posB = [node positionInPixels]; // pixel notation // Sprite CCSprite *sprite = [...]; CGRect rectA = [sprite rect]; // points notation CGRect rectB = [sprite rectInPixels]; // pixel notation
Note that the object setters can also be used in both notations.
[sprite setPosition:ccp(x,y)]; // points notation [sprite setPositionInPixels:(x,y)] // pixel notation
We recommend using the point notation since we know that the positions will be the same in all devices.
Now that we know how the pixel and point notation work, the next step is to understand where the objects are positioned when we pass a specific coordinate. In Codos2D, the point (0,0) is in the bottom left corner of the screen. Thus, the top-right corner has the (480,320) point.
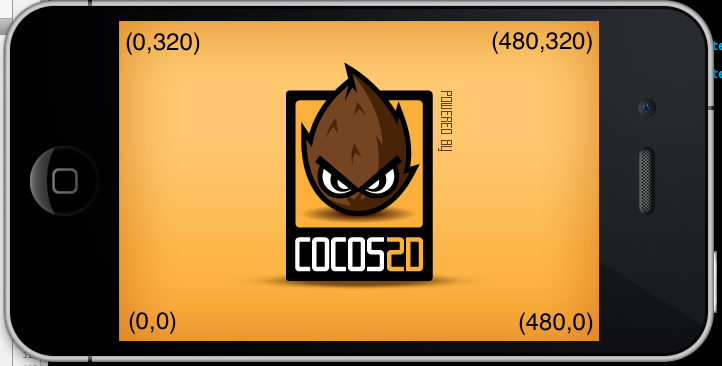
For example, to position a sprite you had to do:
sprite.position = ccp(480,320); // at the top-right corner sprite.position = ccp(0,320); // at the top-left corner sprite.position = ccp(480,0); // at the bottom-right corner sprite.position = ccp(240,160); // at the center of the screen
4. Background and Logo
Cocos2D introduced the concept of scenes. Each scene can be seen as a different screen, which is used to incorporate menus, levels, credits, and everything else that you see during the game. Note that only one scene can be active at a given time.
Inside the scenes, you can have several layers (like Photoshop). The layers can have several properties, and we can have a large number of them on the screen simultaneously. They can have several properties such as background color, animation, a menu, or even a shadow.
Sprites are simply 2D images that can be moved, rotated, scaled, animated, and so on. In the next step, you will change the project source code and add a sprite.
Before you add a sprite, some artwork, which is available in the resources folder, is required.
All resources used in this tutorial are designed for the original iPad resolution.
Once you have downloaded the resources, you will find six images. Copy them to the resources folder of your project and make sure that the option "Copy items into destination goups's folder (if needed)" is checked.
Now, in the HelloWorldLayer.m you can delete everything inside the condition if( (self=[super init]))
. The sprite creation can be achieved in code using the following snippet.
CGSize winSize = [CCDirector sharedDirector].winSize; CCSprite *backgroundImage = [CCSprite spriteWithFile:@"WoodRetroApple_iPad_HomeScreen.jpg"]; backgroundImage.position = ccp(winSize.width/2, winSize.height/2); [self addChild:backgroundImage z:-2];
With the above-mentioned snippet you can change the default background image of that class. The code is simple to understand, and to summarize.
- Ask for the window size
- Initialize a CCSprite with the picture we want to load
- Center the sprite on screen
- Add the CCSprite to the scene
Note that we put the z = -2
because the background needs to stay behind all objects.
Let's add a logo. Looking at the above-mentioned code, we already know how to achieve it. The process is simple and straightforward. If you need some help, you can use the following code.
CCSprite *logo = [CCSprite spriteWithFile:@"MonsterSmashing.png"]; logo.scale = 1.2; logo.position = ccp(winSize.width /2 , 800 ); [self addChild: logo];
It's now time to run the project and see if everything is working as expected. You should see similar to the next screen.
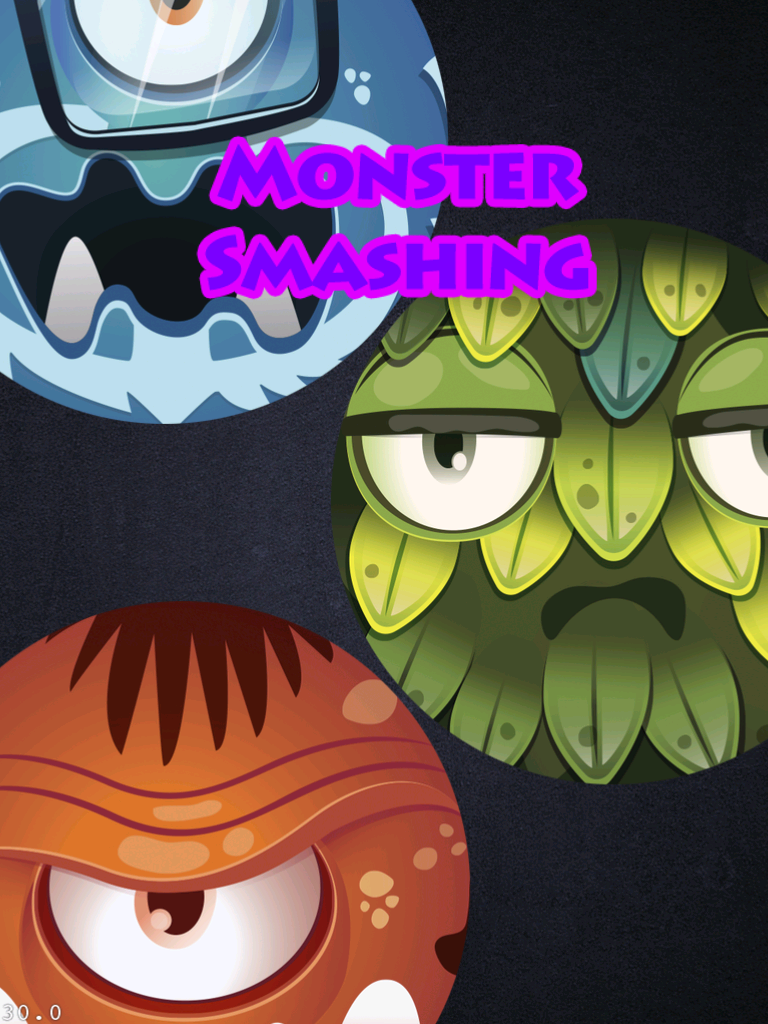
5. Creating the Menu
The main objective for this step is to add a default menu. The menu will have two options:
- Play
- Sound
The Play option will start a new game, while the Sound option is a toggle button that will provide us a way to stop or start the sound engine (in this case the background music).
To implement the Play button (using the CCMenuItem
class), we just need to add a line (for now). This line represents an Item of the Menu. The option is represented using an image (added previously to the resources)
CCMenuItem *startGameButtonImage = [CCMenuItemImage itemFromNormalImage:@"playButton.png" selectedImage:@"playButtonSelected.png" target:self selector:@selector(onStartGamePressed)];
To create and describe the Play Button we need to pass it through several messages. The itemFromNormalImage
is the image location information, and the selectedImage
is the image that appears when our finger is hovering over the item. The selector
is the method that will be called when the user lifts their finger from the menu item, which in this case is the method onStartgamePressed
. We'll talk about that later.
The toggle button is more complex than the play button since it uses two states, on and off. We need to define the two states and then add them to the final toggle button, CCMenuItemToogle
. The on
and off
button is defined as the play button.
After we create the two options, we need to join the options. Use the snippet below for this.
CCMenuItem *startGameButtonImage = [CCMenuItemImage itemFromNormalImage:@"playButton.png" selectedImage:@"playButtonSelected.png" target:self selector:@selector(onStartGamePressed)]; CCMenuItem *_soundOn = [[CCMenuItemImage itemFromNormalImage:@"soundOn.png" selectedImage:@"soundOnSelected.png" target:nil selector:nil] retain]; CCMenuItem *_soundOff = [[CCMenuItemImage itemFromNormalImage:@"soundOff.png" selectedImage:@"soundOffSelected.png" target:nil selector:nil] retain]; CCMenuItemToggle *toggleItem = [CCMenuItemToggle itemWithTarget:self selector:@selector(soundButtonTapped:) items:_soundOn, _soundOff, nil];
After adding the above snippet, we need to create the real menu with the inherent options. We need to use a CCMenu
and send several CCMenuItems
through the method menuWithItems
. As with any other object, we must define its position and its padding, which in this case is vertical. Here's the code.
CCMenu *menu = [CCMenu menuWithItems:startGameButtonImage, toggleItem, nil]; menu.position = ccp(winSize.width * 0.5f, winSize.height * 0.4f); [menu alignItemsVerticallyWithPadding:15]; //Add the menu as a child to this layer [self addChild:menu];
Previously, we said that the selector parameter called a method. We call two methods, onStartGamePressed
and soundButtonTapped
. The first one will replace the current scene, while the second will activate or deactivate the game sound.
Before we go to the method implementations, we need to create a new scene. So, go to File > New > File... and select a CCNode class and name it "MonsterRun". Two new files will be added to your project, MonsterRun.h and MonsterRun.m). Make sure that the class uses the following code.
+(CCScene *) scene { CCScene *scene = [CCScene node]; MonsterRun *layer = [MonsterRun node]; [scene addChild: layer]; return scene; }
Now, we can go back to the HelloWorldLayer.m
and reference the MonsterRun. We use the following line to create it.
#import "MonsterRun.h"
For now, we'll leave this class and its code alone. It'll be covered in the next tutorial. Let's rewind a bit for the menus and the two methods that we talked about before, onStartGamePressed
and soundButtonTapped
.
- (void)soundButtonTapped:(id)sender { // Covered in Part 3 } - (void)onStartGamePressed { CCScene *MonsterRunScene = [MonsterRun scene]; CCTransitionFlipAngular *transition = [CCTransitionFlipAngular transitionWithDuration:0.5f scene:helloWorldScene]; [[CCDirector sharedDirector] replaceScene:MonsterRunScene]; }
Now run the project in order to see if everything is working correctly. You will see something similar to the next image.
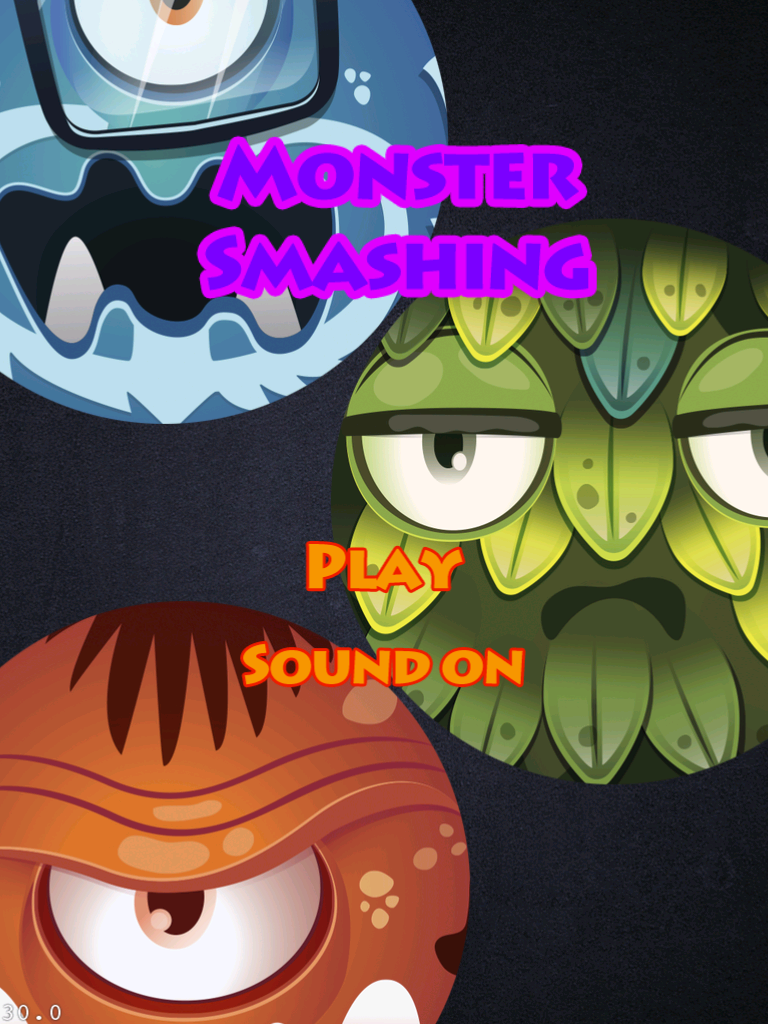
6. Results
At this point, you have to be able to understand and perform the following tasks:
- Install the Cocos2D framework.
- Configure a Cocos2D project.
- Implement scenes, layers, sprites and use the director.
- Pixel and point differences.
- Screen positioning and x-, y- axis orientation.
- Define and use menus.
Comments