I hope you are doing well with the Python smooth refreshers series. Let me quickly remind you that the aim of this series is to teach you in a smooth manner the main concepts you need to grasp in order to move forward in your Python learning journey.
Do you remember that day when you had to water 10 flowers? Or when you had to fill 20 cups of water? You were doing the same task on each flower or cup of water. Such repetition is called looping in programming.
To give a feeling of the importance of looping, say that you were writing a program that should print the same statement 100,000 times. Would you dare repeat this statement 100,000 times, manually?! I won't, and I think you won't too. I'm sure you have much more important stuff to do. Here thus comes the beauty of looping.
Without further ado, let's see the Python way of looping.
While Loop
In this type of iteration, as long as the test is evaluating to true
, the statement or block of statements keep executing. Thus, control keeps looping back to the beginning of the statement (i.e. test), and will handle control to the next statement(s) if the test evaluates to false
. If the test always evaluates to true, in this case what we have is an infinite loop.
The general syntax for the while-statement is very simple, and looks as follows:
while test: # loop test statements # loop body
Remember the infinite loop term I mentioned a while ago? That is, the loop which never stops since the test is always true? Let's see an example of such a loop:
while 1: print 'I\'m an infinite loop. Ctrl-C to stop me!'
The value 1
is another form of the boolean value true
. Thus, if you write while true
, this will be equivalent to the while-statement in the example. As you will notice, you will keep getting print statements displayed infinitely.
To terminate the loop, simply click Control-C on your keyboard. The figure below shows how the program was running infinitely and being interrupted by the keyboard (i.e. Control-C).
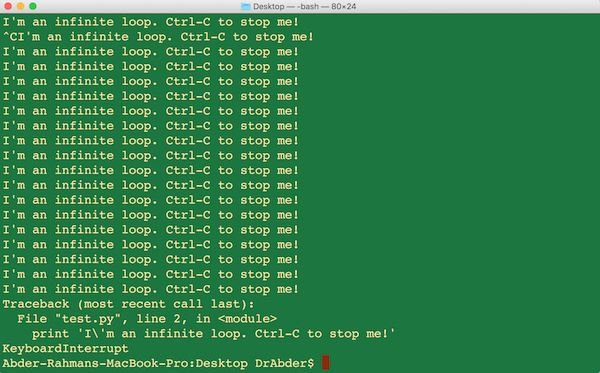
Let's now water our 10 beautiful flowers with Python. This can be done as follows:
flowers = 1 while flowers <= 10: print 'Water the flower # ' + str(flowers) flowers = flowers + 1
The result of the program can be shown in the following figure:
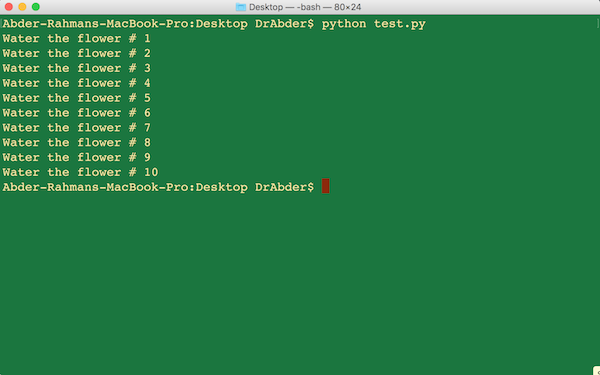
Before moving forward, let me clarify some points in the above Python script. You may be wondering what str()
is and why we have used it here. Based on the documentation:
Return a string containing a nicely printable representation of an object. For strings, this returns the string itself. The difference withrepr(object)
is thatstr(object)
does not always attempt to return a string that is acceptable toeval()
; its goal is to return a printable string. If no argument is given, returns the empty string,''
.
In other words, str()
will return a printable string representation of the object. But, why? Let's see what Python would complain if we didn't use str()
:
Traceback (most recent call last): File "test.py", line 3, in <module> print 'Water the flower # ' + flowers TypeError: cannot concatenate 'str' and 'int' objects
So, the issue is that a str
cannot be concatenated with int
.
The other point I want to mention is the use of flowers = flowers + 1
. If we didn't use such a statement, we would have an infinite loop where the value of flowers would remain 1
, and thus always less than 10
(always true).
For Loop
The for-loop
is an iteration that steps through the items of an ordered sequence such as lists, dictionary keys, tuples, strings, etc.
The Python for-loop
syntax looks as follows:
for var in sequence: statement(s)
Where var
will hold the items of the sequence (i.e. list, tuple) that the for-loop
will be iterating through, such that the for-loop
body will be executed for each item in the sequence.
Time for some examples on the for-loop
!
languages = ['Arabic', 'English', 'French', 'Spanish'] counter = 0 for lang in languages: print 'This language is in the list: ' + languages[counter] counter = counter + 1
What happens if we initialize counter = 1
? In this case, you will get the error shown below since at one point in the loop the index will be out of the list range:
Traceback (most recent call last): File "test.py", line 4, in <module> print 'This language is in the list: ' + languages[counter] IndexError: list index out of range
A small quiz. What would be the output of the following loop? (hint: remember that Python starts counting from 0
):
for x in range(1,5): print x
Statements Used in While and For Loops
The subsections below will show some statements that are frequently used within loops.
break
The statement break
causes the loop to terminate, and program execution is continued on the next statement.
An example of using break
is shown below. It shows how the program quits (break
) the loop when the if-statement
evaluates to true
.
numbers = [1,2,3,4,5,6,7,8,9,10] value = 1 while value in numbers: if value == 5: break print 'I\'m # ' + str(value) value = value + 1 print 'Sorry, I had to quit the loop when the value became 5'
continue
This statement returns control back to the beginning of the loop, ignoring any statements in the loop coming afterward. Let's see the following example:
numbers = [1,2,3,4,5,6,7,8,9,10] value = 1 while value in numbers: if value < 5: print 'I\'m # ' + str(value) value = value + 1 continue print 'I\'m in the if-condition, why are you ignoring me?!' elif value == 5: break print ('I have reached the last statement in the program and need to exit')
Did you figure out how continue
works? What do you think the output of this Python script would be? Go ahead, give it a try.
pass
This statement is a bit tricky. The pass
statement is a null
statement, that is it doesn't do anything. But, why do we use it? Suppose you were writing a program, and at some point you weren't sure what should go in the for-statement
for instance, as follows:
numbers = [1,2,3,4,5,6,7,8,9,10] value = 1 for value in numbers: # not sure what to do here yet print ('I have reached the last statement in the program and need to exit')
If you try to run the program, you will get the following error:
File "test.py", line 6 print ('I have reached the last statement in the program and need to exit') ^ IndentationError: expected an indented block
So Python complains that there should be some statement going inside the for-loop
. If you type pass
in the for-loop
, the output of the program will be:
I have reached the last statement in the program and need to exit
From this, we can conclude that pass
acts as a placeholder to allow the program to run even if you didn't decide yet what required statement(s) has to go in some place of the code.
else
The else
statement is self-explanatory, and will simply contain a block of statements to run when the loop exits in a normal way, and not by a break
. An example of its use is as follows:
numbers = [1,2,3] value = 1 while value in numbers: print 'I\'m # ' + str(value) value = value + 1 else: print 'I\'m part of the else statement block' print 'I\'m also part of the else statement block'
Conclusion
To conclude this article and to imagine the importance of looping, I remember when I had to perform those image analysis operations on each pixel of an image.
Doing this on an image with size 256x256
only means that I had to repeat the operations 65,536 times (which is the number of pixels)! Looping to the rescue!
Comments