Throughout this series, we've been taking a look at how WordPress can serve as a foundation for web application development.
The thing is, up to this point, we haven't really taken a look at the features of WordPress that really contribute to building web applications. Instead, we've spent time looking at how WordPress serves as a foundation rather than a framework, and we've looked at how WordPress is organized in comparison to many of the modern frameworks that are available.
Specifically, we've taken a look at the event-driven design pattern in contrast to the the popular model-view-controller design pattern (and variants thereof), and how this looks within the context of WordPress.
By that, we've worked to rethink application architecture and how its structure within the context of WordPress to help us get a better conceptual model for how things fit together within WordPress, and how to take advantage of the hook system - that is, actions and filters - and how they impact various points during the WordPress page life cycle.
Though this merits a little more discussion, we need to take a look at the facilities that WordPress offers out-of-the-box in order to fully understand the features and APIs with which we have to work before actually taking a look at how to work with them.
Over the next couple of articles, we're going to do just that.
Web Application Components
When you think of the components of a web application, a number of things likely come to mind. That is to say that aside from the usual architecture of the database, middleware, and presentation layer, there are also the following features:
- User management
- Permissions
- Session management
- Email functionality
- Data serialization and retrieval
- URL routing (sometimes referred to as URL rewriting or rewrite rules or even just routes)
- Caching
- Support for custom queries
Though this list is by no means comprehensive, it hits the high notes of what goes into building a standard web application (of course, if there are points that are missed, don't hesitate to mention them in the comments).
And no - this isn't prescriptive. Sometimes, web applications won't have user management, sometimes users won't have roles, perhaps you won't have a need for caching, and so on.
But the point isn't to make a case for all the things you need. Instead, it's about making a case for the things that are available should you need them.
So with that said, let's take a look at what WordPress offers in the way of user management, permissions, email functionality, and session management.
User Management & Permissions
Anyone who has used WordPress - even if it's just for blogging or basic content management - is familiar with the basic user system. That is, you create a user name, a password, and then fill out your profile as much as you want.
Furthermore, more experienced developers are familiar with the ideas of roles and capabilities. I'd even venture to say that those who use WordPress are familiar with the system even if they've never really taken a look at the Codex or messed around with writing any user-based code.
But the general idea is really simple: Users represent an individual profile for an account in WordPress. Their role is indicated by what they've been assigned during account creation.
These roles include:
- Subscriber
- Contributor
- Author
- Editor
- Administrator
Again, most of us who have worked with WordPress are familiar with these roles, right? But how do capabilities fit into the picture?
Simply put, capabilities are what grant users certain permissions throughout the WordPress application, as well as what restricts them from certain areas of the application. This is relatively well-documented in the Codex.
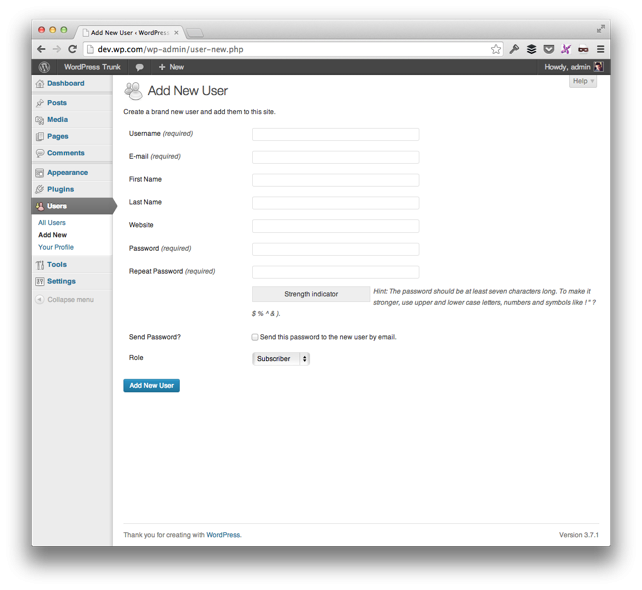
But here's the thing: If you're building an application on WordPress, the available APIs make it possible to lock users out of custom areas that you've created based on their roles and capabilities.
Programmatically Creating a User
First, let's say that at some point while working on your web application, you want to be able to provide the user the ability to register or sign up from a custom sign up form (as opposed to the standard method of creating the user account in the backend as shown above).
This can be done by creating a template with a form and then reading the post data.
In fact, it can really be as simple as capturing the user's email address. I mention this because, although usernames are nice and fun, emails tend to be unique to an individual so it makes error checking easier.
So the steps for doing so would be:
- Capture the user's email address
- Check to see if a user exists with that email
- If not, create the user
- Otherwise, don't create the user and generate an error message
Relatively straight forward, right? Of course, this does imply that you're able to generate a password for the user upon creation of their account. Luckily, there's an API for that.
So here's an example how to programmatically create a user based on a specified email address (while auto-generating a password).
if ( null == username_exists( $email_address ) ) { $password = wp_generate_password( 12, false ); $user_id = wp_create_user( $email_address, $password, $email_address ); wp_update_user( array( 'ID' => $user_id, 'nickname' => $email_address ); ); } else { // The username already exists, so handle this accordingly... }
After that, you need to actually create a new instance of WP_User
.
$user = new WP_User( $user_id );
This is what will allow us to set roles for the given user.
Setting a User's Role and Capability
Finally, it's time to determine what role and set of capabilities you'll be assigning to the user.
On one hand, you can hard code these values based on the options that WordPress offers. The truth is, you can even create custom roles and capabilities. For now, that's outside the scope of the article; however, we may visit it in a future series.
So let's say that we want all users who are currently signed up to be given the subscriber role. You can see what set of capabilities they'll be granted in this Codex article.
Setting a role is trivial:
$user->set_role( 'contributor' );
At this point, you've successfully created a new user within the WordPress application programmatically without having to use any of the default administrative functionality.
Simply wire this up to your own template, validate, sanitize, and check the incoming $_POST
data, and you'll be ready to go.
The full code looks like this:
if ( null == username_exists( $email_address ) ) { // Generate the password and create the user $password = wp_generate_password( 12, false ); $user_id = wp_create_user( $email_address, $password, $email_address ); // Set the nickname wp_update_user( array( 'ID' => $user_id, 'nickname' => $email_address ); ); // Set the role $user = new WP_User( $user_id ); $user->set_role( 'contributor' ); } else { // The user already exists } // end if/else
Not bad, right?
Checking a User's Role and Capability
But creating a user and then actually saving their information in the database in only half of it, right?
Once the user signs in and is authenticated, you're likely going to want to restrict content based on their role. So this raises the question: Once a user is logged in, how do we retrieve a user's role?
Luckily, WordPress' API makes this relatively easy:
- First, we need to be able to get the current user's ID
- After that, we can grab the user's role and proceed with conditional logic from there
We're going to need to take advantage of the wp_get_current_user()
function, and then we'll need to get an instance of the WP_User
object using the current user's ID after which we can take a look at the user's capabilities.
Take a look at the code below:
// Get an instance of the current user $user_id = wp_get_current_user()->ID; $user = new WP_User( $user_id );
Not bad, right?
This makes it really easy to write conditional code such as:
// This will print the user capabilities print_r( $user->wp_capabilities ); // Which in turn will allow you to perform a check like this: if ( '1' === $user->wp_capabilities['subscriber'] ) { // We have a subscriber }
There is an alternative way of doing this, though:
global $current_user; get_currentuserinfo(); if ( 0 === $current_user->user_level ) { // We have a subscriber }
But this raises the question of where did that zero come from? Check out exactly that.
The differences between the two approaches depend on how object-oriented you want your code to be.
I tend to be less of a fan of using global
when there is an object-oriented approach available (such as in the first example); however, the second example provides a relatively simple solution, as well. Your choice.
Anyway, once you're able to conditionally check the role of a user's account, you can then restrict them to specific areas of the application.
But User's Need Emails!
You're right: At the very least, user's need to receive emails for when their accounts have been created, and they need to be able to receive emails whenever something about their account has changed.
Again, WordPress provides an API that makes this really easy, but it can be extended beyond simple actions such as when a user's profile information is changed.
In fact, given the fact that WordPress has a rich event system, you could potentially hook into any number of events and send emails when anything happens. That's obviously overkill, but it goes to show just how powerful the API really is.
So in the next section, we're going to take a look at WordPress' email API and how it can be used to send messages about certain activity, as well as how it can be hooked into other features in the application.
Comments