In this series, we're in the process of talking about how we can build web applications using WordPress. And though this isn't a technical series in which we'll be looking at code, we are covering topics such as frameworks, foundations, design patterns, architectures, and so on.
If you've not read the first post in the series, I recommend it; however, for the purposes of this post, we can summarize the previous post as follows:
In short, software can be built on frameworks, software can extend foundations.
Simply put, we differentiated between frameworks and foundations - two terms that are often used interchangeably in software despite the fact that they are not the same thing. WordPress is a foundation because it's an application unto itself. It's not a framework.
To that end, when it comes to building web applications on WordPress, we need to rethink architecture or re-consider our conceptual models for how applications are built.
The Structure of a Web Application
At the high-lest level possible, web applications are normally structured with the following three components:
- The Database Layer
- The Application Layer
- The Presentation Layer
Generally speaking, the Presentation Layer is what the user sees and what the user interacts with. It includes all of the styles, client-side code, and markup necessary to put something in front of the user.
When the user clicks on something, or the page renders information retrieved from the database, it's interacting with the Application Layer.
The Application Layer is responsible for coordinating information from the browser and/or from the user's action to the database. Sometimes, this consists of writing information to the database - such as information from a form field - to reading information from the database, like retrieving a user's account information.
Much like the Presentation Layer consists of different components - such as styles, JavaScript, markup, and so on - the Application Layer can consist of a variety of different components such as systems that are necessary for reading and writing data to the database, sanitizing information, validating information, and enforcing certain rules that are unique to the problem at hand.
Finally, the Database Layer is where the data is stored. That may consist of the file system, it may consist of a MySQL database, or it may consist of a third-party solution such as a datastore that's "in the cloud" such as Amazon S3 or something like that.
It's All Abstract
The main point to take away from this is that in software, we're always dealing with some level of abstraction. For example, we talk about the data store or the database layer, but we're not really being specific. Same goes with the Application Layer and the Presentation Layer.
- Are we talking about a relational database with multiple tables, or are we talking about cloud storage?
- What kind of data access layer are we going to hook up to the application layer to talk to the database?
- What frameworks and languages are we using on the front-end? Vanilla JavaScript, jQuery, Knockout.js? What about CSS preprocessors - LESS or Sass?
Obviously, we aren't looking to provide answers to these questions right now, but the point is that all web applications consist of similar components, but the details of each component vary from project to project.
The Components of WordPress
As a web application itself, WordPress is a perfect example of how various technologies come together to form a web application:
- The Database Layer is a MySQL database.
- The Application Layer - which some would consider WordPress itself - is written in PHP and handles a lot of the core operations for reading and writing to the data store all the while providing APIs for developers to take further advantage of it.
- The Presentation Layer uses basic CSS (at least for now), HTML (with some themes now using HTML5), jQuery, and with parts of the dashboard using Backbone.js.
So that's the WordPress architecture, but what about the projects that we want to build on top of the application? How do they follow the same architecture?
Well, remember that WordPress is a foundation - not a framework - so we're subjected to WordPress' architecture by default. This does not mean that we can't bring in our own libraries, in some cases, but it does influence the way in which our applications and projects are built.
We'll talk more about the libraries, extensibility and more in a bit, but first, it's important to note that in a day-and-age where MVC (and MVVM and other variants of the Model, View, Whatever) paradigm are all the rage, WordPress does not follow that convention.
There are arguments for and against why that may be a good thing or a bad thing, but that's not the purpose of this post. Instead, it's simply worth noting that WordPress uses an event-driven pattern, rather than the model-view-control panel.
And to that end, it's worth understanding how the event-driven model works so that you have a clear understanding of how WordPress hooks work, and how you can shift you're thinking from MVC or whatever other paradigm you're used to using, to how WordPress manages its information.
What Does It Mean to Be Event-Driven?
Before we take a look at an example of an event-driven application, let's revisit what it means to follow the MVC paradigm.
- First, the view serves as the presentation. The user sees information and interacts with the user interface.
- Next, the controllers coordinate information to and from the model and the view. They respond to user actions, and retrieve information from the model to pipe into the view.
- After that, the model represents the data in the database. This can be done any number of ways, but one of the most popular ways is to map the data in the database into an object-relational model so that the data is represented in the format of objects.
The whole MVC model looks like this:
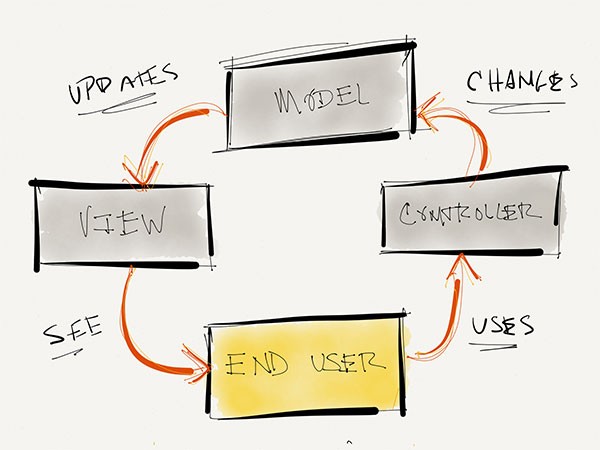
Now, event-driven applications can have some of the same components - that is, they can have views and models or views and data objects - but they don't necessarily have a controller that's coordinating information from the front end to the back end.
Instead, event-driven programming works off of the premise that "something as happened." Hence the name for actions in the WordPress lingo (of course, we also have filters, but I'll cover those momentarily).
WordPress provides hooks which are literally points in execution in which we can introduce our own functionality such that WordPress recognizes that "when this event happens, I need to fire these functions" where these functions are defined as whatever we've provided.
The truth is, filters work the same way, but their purpose is different. In short, filters are actions that are meant for manipulating data (such as appending, prepending, removing, or updating the content) in some way before returning back to the application's execution.
So what does this look like?
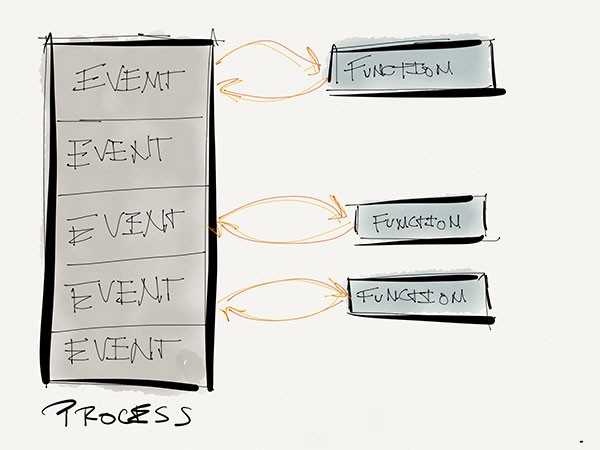
Nothing terribly complicated, right?
So What's Our New Architecture?
The point of this article is primarily getting us to think in terms of event-driven programming and how to coordinate our efforts of building web applications specifically on WordPress.
That is to say that it's important for us to think in terms of events or the fact that "something has happened" so that we know when to appropriately insert our own actions. We'll talk about this a little bit more in detail in the next article, but the main point that I want you guys to take away from this particular article is that just because something isn't MVC (or whatever the next popular paradigm is) doesn't mean that it's not well-suited for application development.
Each pattern and architecture provides us with advantages and disadvantages all of which can contribute to the success of building a web application.
Up Next...
Next up in the series, we'll take a more detailed look at how hooks play a vital role in building web applications on WordPress, and then we'll start looking at some of the facilities that WordPress offers out-of-the-box that make it such a solid option for certain types (read: not all types) of web applications.
Comments