In this short tutorial I will introduce you to ASAudio, and AS3 library that greatly reduces the amount of code needed to create and manipulate sound object in your ActionScript projects.
Step 1: Examining ASAudio
ASAudio is an ActionScript 3 library that greatly reduces the amount of code needed to create and manipulate (volume changes, pan) audio files' within your ActionScript Projects. Traditionally you would need to do the following to load in, play, and change the volume of a Sound within ActionScript:
var urlRequest:URLRequest = new URLRequest("path/to/track.mp3"); var sound:Sound = new Sound(urlRequest); var soundChannel:SoundChannel = sound.play(); var soundTrans:SoundTransform = soundChannel.soundTransform; soundTrans.volume = .5; soundChannel.soundTransform = soundTrans;
With ASAudio all you need to do is the following
var track:Track = new Track("path/to/track.mp3"); track.start(); track.volume = .5;
Step 2: Getting the Library
Head over to the project's Google Code page and download the latest version of ASAudio.
Once you have downloaded it, extract it and copy the the "com" folder that is inside the "src" folder to the folder where you will be creating your ActionScript project.
Step 3: New ActionScript Project
Start a new ActionScript project, and save it in your project folder as "asAudio.fla". Under the "PROPERTIES" panel, set the stage color to white and give it a width and height of 500x150px.
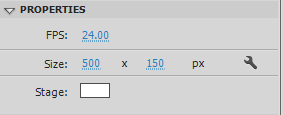
Now create a new ActionScript File and save this as "Main.as". Set this as your Document Class.
Step 5: Imports and Constructor Function
Add the following to the "Main.as" you created in the step above.
package { import flash.display.Sprite; import com.neriksworkshop.lib.ASaudio.*; import flash.media.Sound; import flash.events.MouseEvent; import flash.events.Event; public class Main extends Sprite { public function Main() { trace("Working"); } } }
Here we import the classes we will need throughout this tutorial, and setup our Main()
constructor.
Step 6: Track
The Track
is the fundamental class of ASAudio. Everything you do with the library depends on the Track
. In this step we will create a new Track
, and play it. Enter the following code above the Main()
within "Main.as".
var track:Track = new Track("BoozeandBlues.mp3");
And the following within the Main()
constructor.
public function Main() { track.start(); }
To create a Track
you pass in a path to the mp3. We then call the start()
method of the Track
within Main()
.
If you test now you should hear the mp3 play.
Step 7: Setting up the Interface
In this step we will setup the interface for the project so we can control our Track
's.
From the Component Window drag 4 buttons and a slider onto the Stage. You can get to the Components Window by choosing Window > Components or by pressing CTRL+F7.
Give the buttons the following properties, one by one.
- X: 21.00 , Y:61.00, Label:"Play TracK", Instance Name:"playTrackBtn"
- X: 175.00, Y:61.00, Label:"Play Group", Instance Name"playGroupBtn"
- X: 333.00, Y:61.00, Label:"Play Playlist", Instance Name"playListBtn"
- X: 21.00, Y:100.00, Label:"Stop Track", Instance Name"stopTrackBtn"
Here is a screenshot of the first Button's setup.
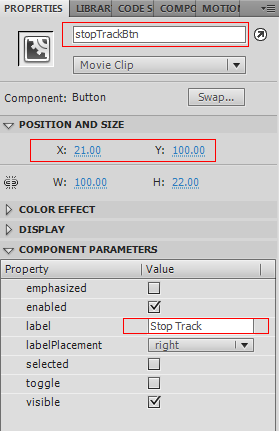
Give the slider the following properties.
- X: 21.00, Y:29.00, Instance Name:"trackSlider"
- maximum: 1
- miniumum: 0.1
- snapInterval: 0.1
- tickInterval: 0.1
- value: 1
- liveDragging: "Make sure it is checked"
Here we set some initial values for the slider. I won't go over them as you can refer to my Quick Tip that covers the sliders functionality.
Step 8: Controlling the Track
In this step we will code the start, stop, and volume functionality for the track we created in the previous step. Add the following to "Main.as".
public function Main() { addListeners(); } private function addListeners():void{ trackSlider.enabled = false; playTrackBtn.addEventListener(MouseEvent.CLICK,playTrack); stopTrackBtn.addEventListener(MouseEvent.CLICK,stopTrack); trackSlider.addEventListener(Event.CHANGE,adjustTrackVolume); }
Here we call the addListeners()
function within our Main()
constructor. Inside addListeners
we set the disable the trackSlider
and add Listeners to 3 of our buttons.
Step 9: playTrack()
The playTrack()
function will tell the track to start playing. Add the following beneath the addListeners()
function you created in the step above.
private function playTrack(e:MouseEvent):void{ trackSlider.enabled = true; track.start(); track.volume = 1.0; }
Here we enable the trackSlider
play the Track
using the start()
method and set the volume of the track using the volume property.
Step 10: stopTrack()
The stopTrack()
function will be used to stop the Track
. Add the following beneath the playTrack()
function you added in the step above.
private function stopTrack(e:MouseEvent):void{ track.stop(); }
Here we simply call the stop()
method on the Track
.
Step 11: adjustTrackVolume()
The adjustTrackVolume()
function will be used to adjust the volume of the Track
. Add the following beneath the stopTrack()
function you created in the step above.
private function adjustTrackVolume(e:Event):void{ track.volume = e.target.value; }
Here we set the volume of the track equal the the sliders value
property. Because we set the maximum to 1 and the minimum to 0.1 it will always be a number between 0.1 and 1.0 incremented by 10ths. i.e 0.1, 0.4, and so on.
If you test the movie now you should be able to play, stop, and adjust the volume of the Track
.
Step 12: Groups
You may have been wondering what the "Play Group" button was for? Well, along with offering basic the basic Track
ASAudio has a notion of "Groups" which allows you to stack sounds together and play them together all at once. Add the following within the addListeners()
function you created in the step above.
private function addListeners():void{ trackSlider.enabled = false; playTrackBtn.addEventListener(MouseEvent.CLICK,playTrack); stopTrackBtn.addEventListener(MouseEvent.CLICK,stopTrack); trackSlider.addEventListener(Event.CHANGE,adjustTrackVolume); playGroupBtn.addEventListener(MouseEvent.CLICK,playGroup); }
Here we add a Listener to our playGroupBtn
that will call the playGroup()
function. We will code this next.
Step 13: playGroup()
Add the following beneath the adjustTrackVolume()
function you creafted in the step above.
private function playGroup(e:Event):void{ var group:Group = new Group( [new Track("piano.mp3"), new Track("drumbeat.mp3")] ); group.start(); }
Here we create a new Group
by passing in an Array of tracks. We then call the start()
method which tells the Group
to start playing.
If you test now you should hear the "piano.mp3" and "drumbeat.mp3" playing simutaneously.
Step 14: Playlist
Along with offering the Group
, ASAudio has a notion of a PlayList
. The PlayList
is like a playlist on an MP3 player. You "queue" up songs and when one finishes it continues to the next. Add the following within the addListeners
function.
private function playPlayList(e:Event):void{ var playList:Playlist = new Playlist( [new Track("piano.mp3"), new Track("drumbeat.mp3")] ); playList.loop = false; playList.start(); }
Like the Group
we create a new PlayList
by passing in an Array of Tracks. We set the PlayList
to not loop and call the start()
method.
You can now test and see the PlayList
in action.
Conclusion
You have learned about ASAudio and seen how it can greatly reduce the amount of code needed to create Audio within your ActionScript Projects. This library has more to offer including fade, pause, and mute methods... I suggest you take a look through the documentation to see what all is available. I hope you found this useful and thanks for reading!
Comments