In this tutorial you will be introduced to one of the most commonly used Cocoa-Touch classes: UIAlertView. With this class, you can quickly notify users of important or urgent application information. You may also force user feedback by combining the UIAlertView class with the UIAlertViewDelegate protocol. Read on to learn how!
Step 1: Project Setup
Create a new Xcode project using the "Single View Application" template.
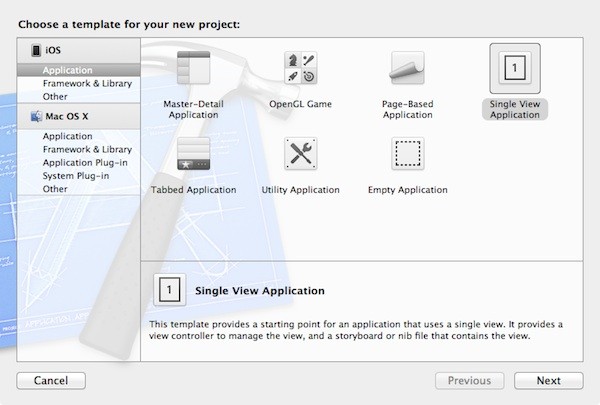
Name the application "AlertDemo" and insert your own company identifier or use "com.mobiletuts.alertdemo" if you don't have one yet. Set "iPhone" for the device family and keep the default checks. When ready, click Next.
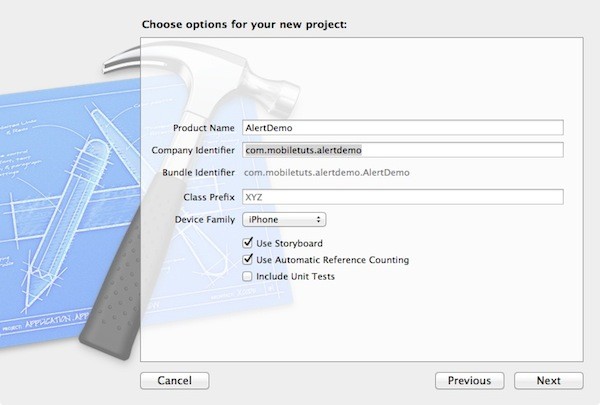
Select a location on your hard drive to store the project and then click "Save".
After creating your project, expand the Supporting Files folder in the Project Navigator pane to the left of the Xcode window. CTRL + Click (or right click) on the Supporting Files folder and select Add > New Group. Name the new group "images" as this is where we will store the graphics for our application.
Download the project resource file attached to this tutorial and open the "images" folder. Drag and drop all of the images into the "images" group you just created. Be sure to check "Copy items into destination group's folder (if needed)" as this will ensure the resources are actually copied into your project and not simply linked as references. Your setup in Xcode should now look similar to this:
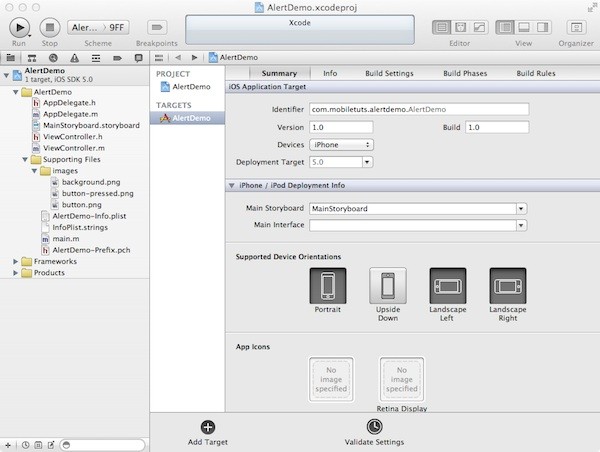
Step 2: Setup the Interface
Find the MainStoryboard.storyboard
file in the Project Navigator and select it. You've just opened the storyboard for the project, which should consist of a single scene, or View Controller, that we can modify to create the interface for the application.
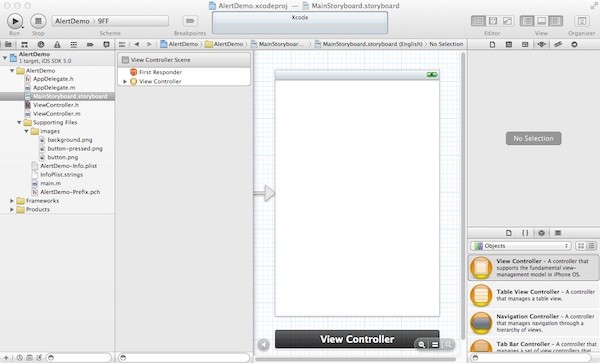
With the Storyboard open, the Xcode interface should now display the object library in the bottom right corner. Use the object library search box to find a UIImageView
object. Place the image object on the center of the screen and manually resize it to fill the view. Next, find the image field in the attributes inspector (the attribute inspector should be in the top right portion of Xcode). Set the value for the image field to "background.png". You should now have a nice, steel texture that fills the view controller.
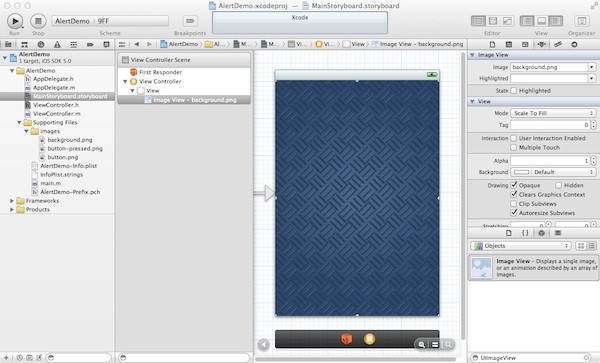
Using the Object Library again, find a UIButton
object and then drag that button onto the Scene. The attribute inspector should now display information for the button object. Set the type drop down field to "custom". Next, set the image field to "button.png". You should now see the button image on the scene view controller, but it probably doesn't look right. With the button object selected, you'll need to select Editor > Size to Fit Content in order to automatically resize the button bounds to the size of the image content. Next, center the button in the screen by dragging it toward the middle.
You should now have the button configured correctly for the default state, but we want to display a different image when the button is actually being pressed. Change the state config drop down value to "highlighted". The changes you make in the attributes inspector now will apply only to the highlighted state. Set the image attribute for the highlighted button state to button-pressed.png. Your Storyboard should now look like this:
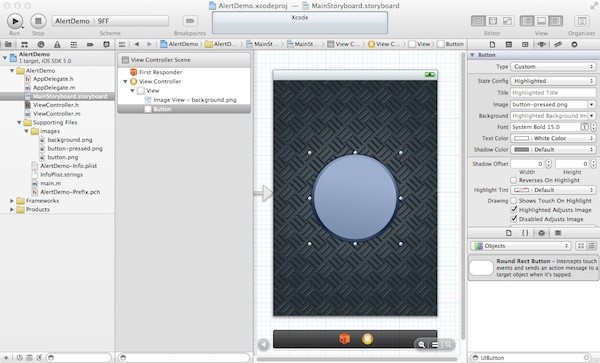
This is a good time to test your progress, so save your work ( File > Save) and then run the project in the iPhone simulator (Product > Run). You should now be able to tap the button and see the image change in the simulator.
Step 3: Create the Alert Method
With the project storyboard still open, click the "Show the Assistant Editor" button near the top right of the Xcode window to display the source code listing for ViewController.h. From this perspective, hold down both the Control (CTRL) button and the mouse button (the left mouse button if your mouse has two) over the button object in the Storyboard scene, and then drag into the source code listing, just above the @end
line.
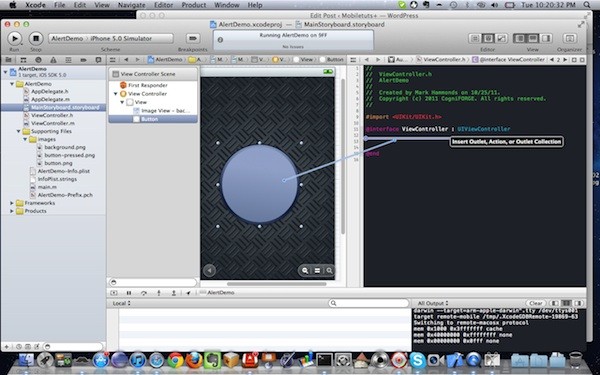
A new dialogue box will open that will automatically create a connection between the interface element and your view controller class. From this popup you can create outlets or actions. Outlets are used to create properties that reference your Interface Builder objects, and actions are used to connect methods to IB object actions.
Select "Action" from the connection drop down, enter "showMessage" as the connection name, and then click Connect. Interface Builder should have just added the following line to your ViewController.h file:
- (IBAction)showMessage:(id)sender;
In addition, ViewController.m should now have this method definition:
- (IBAction)showMessage:(id)sender { }
When the user taps the button, the code in this method will be executed. That means we're finally ready to dig into the code for displaying a UIAlertView
and responding to its delegate methods!
Step 4: Create a Basic Alert Message
Now that you have your project template setup, you are ready to use UIAlertView to trigger your message when the custom button is pressed. Open the ViewController.m
file, and type the following into the showMessage
method you created earlier:
- (IBAction)showMessage:(id)sender { UIAlertView *message = [[UIAlertView alloc] initWithTitle:@"Hello World!" message:@"This is your first UIAlertview message." delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil]; [message show]; }
Above you are creating and initializing your instance of the UIAlertView class. The initWithTitle:
and message:
parameters are self-explanatory and easily understood after seeing an alert displayed. The delegate:
parameter refers to which class should receive delegate notification messages for the UIAlertViewDelegate
(demonstrated later). The cancelButton:
parameter is the default button to be displayed along with the alert, and the otherButtonTitles:
parameter is used to display one or multiple additional options that the user may select.
After creating an alert object, the [message show]
call actually shows our message by popping our new UIAlertView onto the screen.
If you build and debug the application now, you'll see that tapping the button results in our message being displayed!
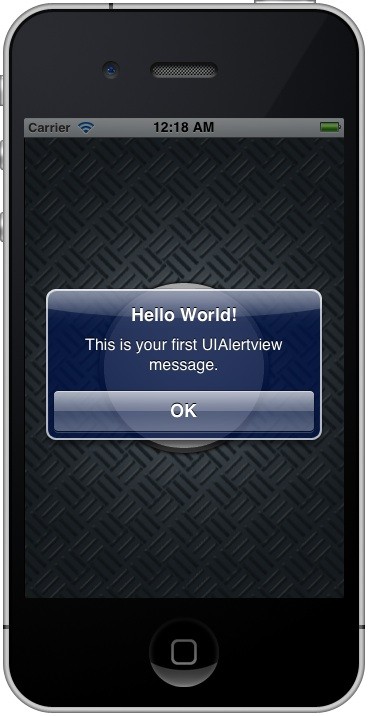
NOTE: If nothing happens when you tap the button in the simulator, be sure that you have actually saved the changes you made in the Storyboard file and that the "Touch up inside" action is connected to the showMessage:
method.
Step 5: Add Buttons to the Alert
Adding additional buttons to a UIAlertView can be done either when you initialize your object or anytime after. To add buttons at initialization time in the code above, you would simply modify the showMessage:
method like so:
UIAlertView *message = [[UIAlertView alloc] initWithTitle:@"Hello World!" message:@"This is your first UIAlertview message." delegate:nil cancelButtonTitle:@"Button 1" otherButtonTitles:@"Button 2", @"Button 3", nil]; [message show];
To add buttons after having initialized your alert, you would use the following lines of code:
UIAlertView *message = [[UIAlertView alloc] initWithTitle:@"Hello World!" message:@"This is your first UIAlertview message." delegate:nil cancelButtonTitle:@"Button 1" otherButtonTitles:nil]; [message addButtonWithTitle:@"Button 2"]; [message addButtonWithTitle:@"Button 3"]; [message show];
Both of these code fragments are equivalent, but using the addButtonWithTitle:
method makes it easy to conditionally add buttons to your alerts, perhaps by wrapping them in if
statements.
Step 6: Respond to Alert Button Selection
Switch back to ViewController.h
and modify your class declaration line to read as follows:
@interface ViewController : UIViewController <UIAlertViewDelegate> {
This allows your class to respond to the UIAlertViewDelegate
protocol methods. In order to respond to button taps in our UIAlertView
, we will use the – alertView:clickedButtonAtIndex:
protocol method. Back in ViewController.m
, add this into your class:
- (void)alertView:(UIAlertView *)alertView clickedButtonAtIndex:(NSInteger)buttonIndex { }
As you can see from the method name, this method will accept a pointer to the UIAlertView
object we created earlier and the button index that was selected by the user. You may be tempted to simply switch conditionally on the buttonIndex variable to determine which button was selected by the user, but what would happen if you were conditionally adding buttons to the alert? In that scenario, the button with index 1 might not always be the one you want. A much better way is to test against the title of the button and then act accordingly, like so:
- (void)alertView:(UIAlertView *)alertView clickedButtonAtIndex:(NSInteger)buttonIndex { NSString *title = [alertView buttonTitleAtIndex:buttonIndex]; if([title isEqualToString:@"Button 1"]) { NSLog(@"Button 1 was selected."); } else if([title isEqualToString:@"Button 2"]) { NSLog(@"Button 2 was selected."); } else if([title isEqualToString:@"Button 3"]) { NSLog(@"Button 3 was selected."); } }
There is one more tweak that we need to make before this will work. Go back to the UIAlertView initialization statement, and update the delegate:
parameter to accept the self
keyword instead of nil
. After doing so, the code block should look like this:
UIAlertView *message = [[UIAlertView alloc] initWithTitle:@"Hello World!" message:@"This is your first UIAlertview message." delegate:self cancelButtonTitle:@"Button 1" otherButtonTitles:@"Button 2", @"Button 3", nil]; [message show];
If you build and debug the application now, you should be able to select multiple buttons and see a statement print to the console when a button is selected (need help finding the console? Select View > Debug Area > Activate Console from the Xcode menu).
This is what the final alert view should look like in the simulator:
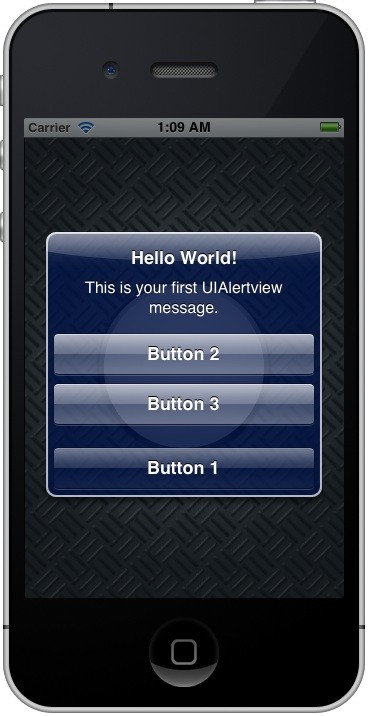
Conclusion
This concludes our introductory tutorial on UIAlertView
. In addition to what we covered today, there are many other options provided by the UIAlertViewDelegate
protocol and, with the release of iOS 5, some additional ways to use UIAlertView
. If you would like to see an additional tutorial on these advanced alert view topics, let me know in the comments section below! Also feel free to send in questions or comments via either my personal twitter @markhammonds or the official Mobiletuts+ twitter account @envatomobile.
Comments