This is the third part in the iOS Multitasking Series. Compared to Local Notifications, Background Audio, Background Location, and VOIP (Not covered in this series), Background Tasks and Fast App Switching are the easiest to implement. For this tutorial, you will need to understand blocks.
Step 1: Creating the Project
For the simplicity of this tutorial, create a window based application so we do not have to deal with View Controllers and extras (same implementation is used no matter what template you use). Name it Background Task and uncheck "Use Core Data" and "Include Unit Tests".
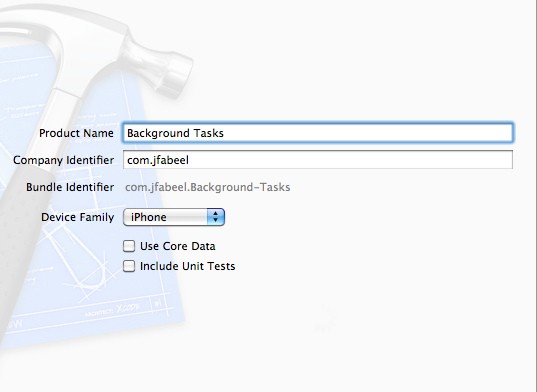
Then open up the Background_TasksAppDelegate.m.
Step 2: Implementing the code
Now scroll down to the applicationDidEnterBackground: method and add the following code:
if ([[UIDevice currentDevice] respondsToSelector:@selector(isMultitaskingSupported)]) { //Check if our iOS version supports multitasking I.E iOS 4 if ([[UIDevice currentDevice] isMultitaskingSupported]) { //Check if device supports mulitasking UIApplication *application = [UIApplication sharedApplication]; //Get the shared application instance __block UIBackgroundTaskIdentifier background_task; //Create a task object background_task = [application beginBackgroundTaskWithExpirationHandler: ^ { [application endBackgroundTask: background_task]; //Tell the system that we are done with the tasks background_task = UIBackgroundTaskInvalid; //Set the task to be invalid //System will be shutting down the app at any point in time now }]; //Background tasks require you to use asyncrous tasks dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_DEFAULT, 0), ^{ //Perform your tasks that your application requires NSLog(@"\n\nRunning in the background!\n\n"); [application endBackgroundTask: background_task]; //End the task so the system knows that you are done with what you need to perform background_task = UIBackgroundTaskInvalid; //Invalidate the background_task }); } }
Wrap Up
As you can see, Background Tasks are extremely short and easy to implement. If you have any need for help or just want to post a suggesion, then comment below!
Comments