Introduction
Kotlin, the open source programming language designed by JetBrains, is becoming increasingly popular among Java developers. It is often touted as Java's successor. Compared to Java, it offers a richer development experience, because it is more modern, expressive, and concise.
If you are looking for an alternative programming language for Android development, you should give Kotlin a try. It can easily be used instead of or alongside Java in your Android projects.
In this tutorial, I will show you how to use Kotlin and Kotlin plugins in your Android Studio projects.
Prerequisites
To follow along with me, you need to have:
- the latest version of Android Studio
- a basic understanding of Kotlin syntax
If you're unfamiliar with the Kotlin programming language, then I recommend reading the Getting Started section of the Kotlin reference to get up to speed with the language.
1. Installing Kotlin Plugins
In Android Studio's quick start menu, select Configure > Plugins.
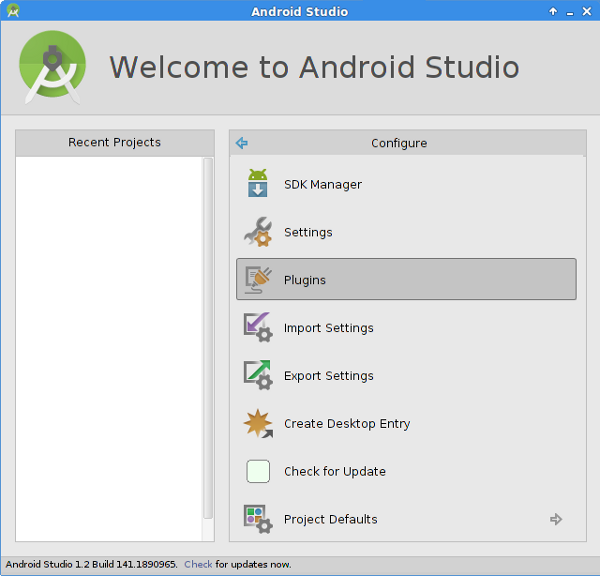
In the next screen, click Install JetBrains plugin... at the bottom.
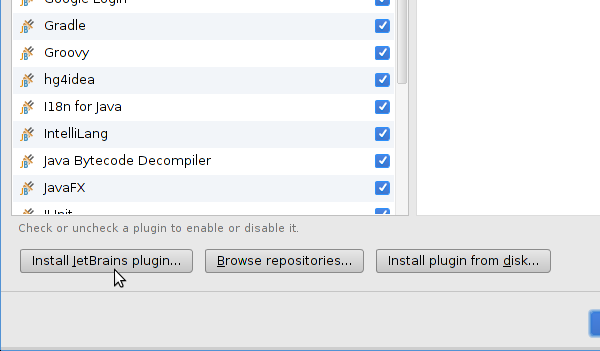
Select Kotlin Extensions For Android from the list and click Install Plugin on the right.
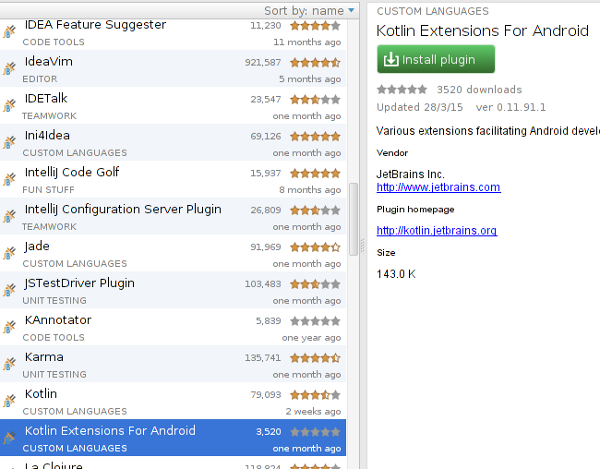
Because the plugin depends on the Kotlin plugin, you will be asked to install both. Click Yes to begin the installation.
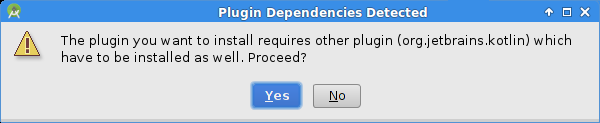
When the installation completes, restart Android Studio to activate the plugins.
2. Creating a Kotlin Activity
In Android Studio, right-click the name of your package and select New > Kotlin File.
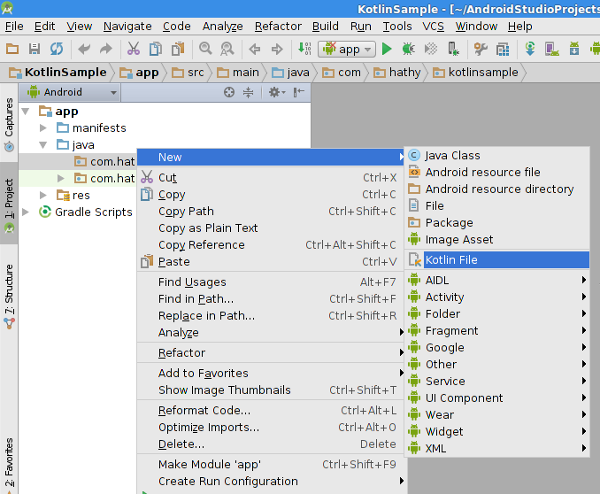
In the dialog that pops up, enter the name of the new Activity
and select Class from the drop-down list. I've named my class MainActivity.
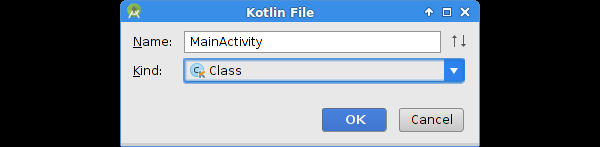
Once the class has been created, you will see an alert asking you to configure your app module to support Kotlin.
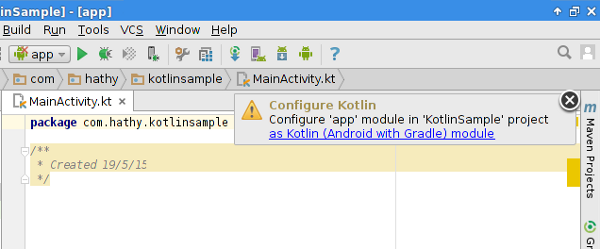
Click the link in the alert and, in the dialog that pops up, click OK to choose the default values.
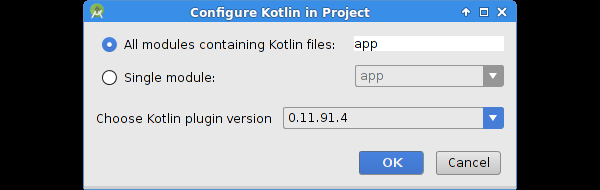
To configure your project to support Kotlin, the Kotlin plugin makes several changes in the build.gradle file. Apply these changes by clicking the Sync Now button as shown below.
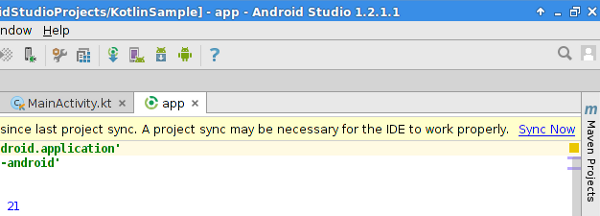
At this point, your project's configuration is complete. Go back to the Kotlin class you created a moment ago to start coding in Kotlin.
3. Using Kotlin
To keep the example simple, I will be showing you how to create an Activity
with a single TextView
that displays a String
.
Make sure your class is a subclass of Activity
and override its onCreate
method. Of course, you have to do that in Kotlin. If you are new to Kotlin, I suggest you use Android Studio's code generation functionality by pressing Control+O to get the method signatures right.
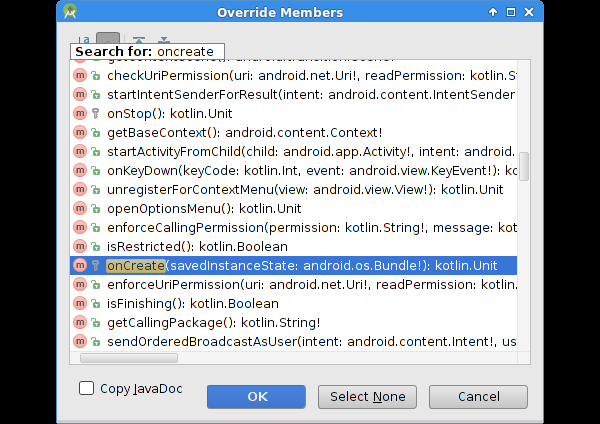
Your class should now look like this:
package com.hathy.kotlinsample import android.app.Activity import android.os.Bundle public class MainActivity: Activity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) } }
Create an instance of TextView
as an assign-once local variable by using the val
keyword.
val myMessage = TextView(this)
Call its setText
method to set the String
you want to display and then call setContentView
to display the text view.
myMessage.setText("Hello") setContentView(myMessage)
Like you would for a Java Activity
, you need to declare your Kotlin Activity
in your app's AndroidManifest.xml for it to be recognized by the Android system. If this is the only Activity
in your project, your manifest file should look like this:
<activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN"/> <category android:name="android.intent.category.LAUNCHER"/> </intent-filter> </activity>
You can now compile and run your app on your Android device or emulator. While the Kotlin compiler is slightly slower than that of Java, you are unlikely to see any significant change in your project's build time.
4. Using Kotlin Android Extensions
The Kotlin Android Extensions plugin lets you treat the widgets you define in the layout XML of an Activity
as if they were properties of that Activity
. In other words, if you use this plugin, you will never have to call findViewById
. These properties are aptly called synthetic properties.
To use this feature in your app, you need to add org.jetbrains.kotlin:kotlin-android-extensions
as a build script dependency in your app module's build.gradle as shown below. Don't forget to click the Sync Now button to sync your project.
buildscript { dependencies { classpath "org.jetbrains.kotlin:kotlin-android-extensions:$kotlin_version" } }
Let's now create an Activity
similar to the one we created in the previous step, but use a layout XML to define the TextView
. Create a new layout XML file named another_activity.xml. In the layout XML file, define a TextView
with an id
of myMessage.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:text="Large Text" android:id="@+id/myMessage" /> </LinearLayout>
Create another Kotlin class, AnotherActivity, that extends Activity
and override its onCreate
method. This is what the implementation should look like:
package com.hathy.kotlinsample import android.app.Activity import android.os.Bundle public class AnotherActivity: Activity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) } }
Call setContentView
in the onCreate
method to use the layout XML you just created as the layout of this Activity
.
setContentView(R.layout.another_activity)
Now, instead of calling findViewById
to get a reference to the TextView
, you can import it using the following code snippet:
import kotlinx.android.synthetic.another_activity.myMessage
If you had more widgets in your layout, you can import all of them using the following code snippet:
import kotlinx.android.synthetic.another_activity.*
You can now access your TextView
using its id
as if it were a property of the Activity
class. For example, to change the text of the TextView
, you can write:
myMessage.setText("Hello")
5. Converting Java Classes to Kotlin
You can use the Kotlin plugin to convert existing Java classes to Kotlin classes. To try this feature, create a new Java class with the following implementation. It's a simple Activity
that logs the sum of two integers.
public class YetAnotherActivity extends Activity { private int a,b; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); a=10; b=20; Log.d("MESSAGE", "The sum is "+(a+b)); } }
Convert the Java class to a Kotlin class by pressing Control+Alt+Shift+J, or, from the menu, selecting Code > Convert Java File to Kotlin File.
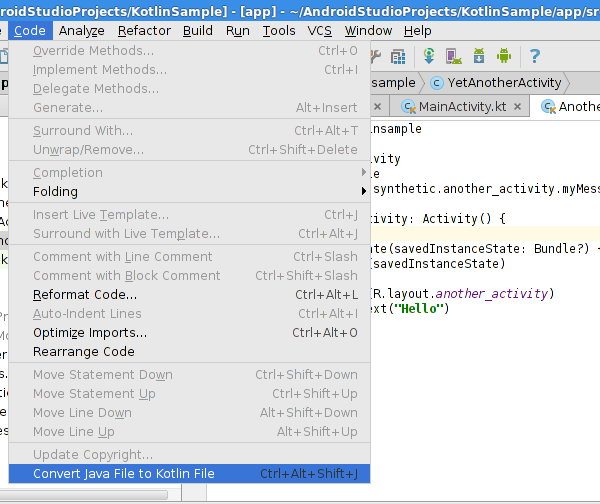
After the conversion, your class will look like this:
public class YetAnotherActivity : Activity() { private var a: Int = 0 private var b: Int = 0 override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) a = 10 b = 20 Log.d("MESSAGE", "The sum is " + (a + b)) } }
You will also notice that the file's extension has changed from .java to .kt.
Conclusion
In this tutorial, you have learned how to use Kotlin in your Android projects after installing the Kotlin plugin and the Kotlin Android Extensions plugin for Android Studio. As Kotlin and Java classes are largely interoperable, if you are still learning Kotlin, it is best to introduce it in your Android projects gradually.
To learn more about Kotlin, I recommend browsing the Kotlin reference. The Getting Started section will help you get up to speed with this new language.
Comments