WordPress uses posts and pages to provide the dynamic content for applications. The introduction of Custom Post Types has increased the possibility of developing complex applications with WordPress.
Generally, normal posts go through a well-defined workflow, before they get published on the website or on the application. During this workflow, various statuses are assigned to posts and handled internally by WordPress.
Post statuses can be used as a powerful technique for managing status in a custom web application. In this article, we are going to discuss how to use WordPress custom post statuses and transitions to build applications which go beyond the conventional websites or blogs.
Do you have experience in working with custom post status transitions? All of you are welcome to discuss your experiences.
Understanding WordPress Post Status
WordPress uses the wp_posts
table to store both posts and pages. THe status of a post defines a temporary state until it gets published onto the website. Generally, a post's status starts as a draft and switches between existing statuses until it comes to the published status. Let's take a look at the existing WordPress post status list and their roles.
- publish - A post is considered to be published and will be publicly available on the website.
-
pending - A post is pending review from a higher user role. This status will be mainly available in the site where you have multiple authors or users who can create records on the
wp_post
table. - draft - A post is saved temporarily and the post author may make further changes before publishing.
- auto-draft - A post is saved temporarily without any content and the author may make further changes before publishing.
- future - A post is scheduled to be published at a future date. This is a commonly used technique to keep up consistency of posting.
- private - A post is only visible to logged in users.
- inherit - This is considered a revision of a post. WordPress allows multiple revisions of the same post.
- trash - A post is considered to be deleted.
Usually, each and every post starts with a draft or auto-draft status and continues to progress until it gets to the desired final state. In the next section, we are going to look at WordPress post status transitions and their usage.
Working With Post Status Transitions
Post status transition is the process of switching between one status to another status. Usually, existing post transitions and their respective functionality are handled internally by WordPress. But there are many effective ways of adding features with post transitions. As a result, WordPress now provides hooks for working with all the post status transitions; therefore, we can dynamically add new features on a post's transition.
Let's see how it actually works.
Assume that we want to do something when the post status changes from draft to future. The following code shows you how to implement a post status transition for the preceding requirement.
function callback_function_name( $new_status, $old_status, $post ) { // Code here } add_action( 'draft_to_future', 'callback_function_name', 10, 3 );
WordPress provides an action hook of the format {old-status}_to_{new-status} for each and every post transition. We can use a callback function to provide custom functionality. This custom function takes the old status, new status, and the changed post object as parameters.
In the previous section, we discussed about eight predefined post statuses. Here, we have nine post statuses for transitions, including a status called new. Before the post is saved, it will be considered as new. As soon as the post saves to the database, transition will occur from new_to_{custom status}.
Now, let's see the post transitions for publishing a post in normal circumstances.
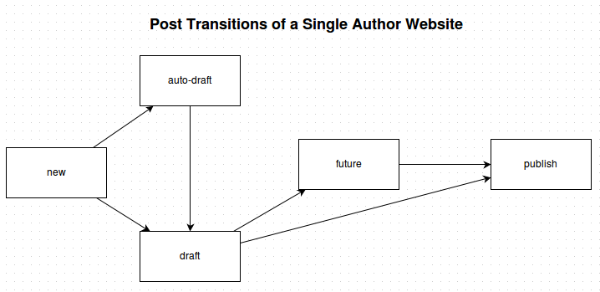
The preceding screen shows the post transitions of a website with a single author. Basically, we can work with post status transitions between the statuses joined with arrows. In a single author website, post transitions are simpler compared to multi author websites.
So let's take a look at the process of multi author website.
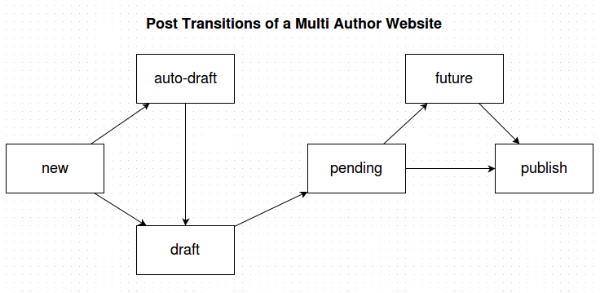
In a multi-author website, the process is slightly changed as all the posts need to be reviewed and approved by an authorized person before publishing; therefore, multi-author websites have an additional step in the post transition process.
So far, we've looked at the default post status transitions in a WordPress website. Now the question is, how are these transitions going to be useful?
There are plenty of ways of using post status transitions in applications. Let's look at some of the commonly used functionality in post status transitions.
- Draft to Pending - Notify the editor to review the post.
- Pending to Future - Notify the post author.
- Pending to Future - Add the post to the post calender on the site.
- Future to Publish - Notify subscribers through email.
These are some of the most basic and common functionalities done during post transitions. Up until now, we've looked at the post status transition process for predefined statues.
The real value of post status transitions comes with the use of custom post statuses. The next section covers the details of working with custom post statuses for custom web applications.
Introduction to Custom Post Status
WordPress is slowly becoming a framework for developing web applications by going beyond the general content management system. Custom post status becomes vital for the development of complex applications. WordPress allows us to create our own post statuses and supports transitions between those statuses. Let's take a look at the following code for creating a custom post status.
function add_custom_post_status() { register_post_status( 'custom_status', $args ); } add_action( 'init', 'add_custom_post_status' );
Custom post statuses can be defined using the register_post_status
function, which takes a post status name as the mandatory parameter. This syntax is similar to the code used for custom post type creation. Also we can pass additional arguments based on our preferences. You can find a complete arguments list at in the WordPress Codex. Once the above code is used, the new custom post status will be added to the existing list.
Unfortunately, WordPress' admin panel doesn't have the built-in support for custom post statuses; therefore, we have to find alternative ways of adding custom post statuses to the admin panel.
Explaining the process of integrating custom post status into the admin panel is beyond the scope of this article, so I am going to use an existing plugin to show you how to work with custom statuses.
Integrating Custom Post Status Into the Admin Panel
Basically, we have to customize the existing admin post submit metabox in order to show custom post statuses in the Status drop down field. At this stage WordPress support for this feature is very limited and hence it's difficult to find quality plugins for working with custom post statuses.
We can use a plugin called Edit Flow for managing custom post statuses. You can grab a copy of this plugin from http://wordpress.org/plugins/edit-flow/. Once activated, navigate to the Custom Statuses section under the Edit Flow menu and you will get a screen similar to the following.
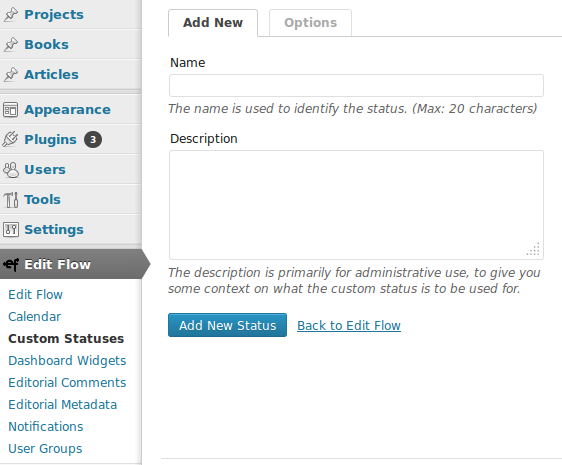
We can use this form to create new custom post statuses. This plugin internally uses the register_post_status
function to define the custom status and stores it in the wp_terms
table. All the status management is done internally by the plugin.
Ideally, we would like to see these features available within WordPress core. Once created, you will find the list of new statuses as shown in the following screen.
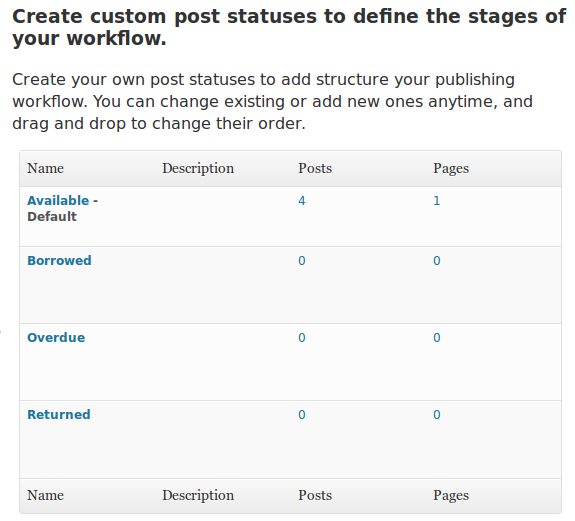
Now the statuses are ready and you can go to the post creation screen and select the necessary status before saving the post. Then you can implement status transitions on the post to add more features or manage existing features.
Using Status Transitions in Custom Web Applications
We need to make use of custom post types in custom web applications. Custom post statuses play a vital role in managing custom post types.
Usually, existing post types have a very limited meaning in working with custom post types; therefore, we need to use custom status transitions to manage the state of custom posts. Let's look at practical scenarios for understanding the need for custom post statuses.
Online Product Selling System
These days most products are being sold online using shopping carts. There are plenty of existing WordPress sites for selling products. In such a system, we need to have a custom post type called Products to store all the information about products.
Now think how we can match the existing post statuses into products. Statuses like draft, future, and pending have no meaning in the context of products. So we need custom statuses to cater to such scenarios. Let's think about the possible statuses for products.
Usually, we can use statuses like In Stock, Ordered, Shipped, Delivered, and Returned for Products. Let's look at the following screen for possible status transitions.
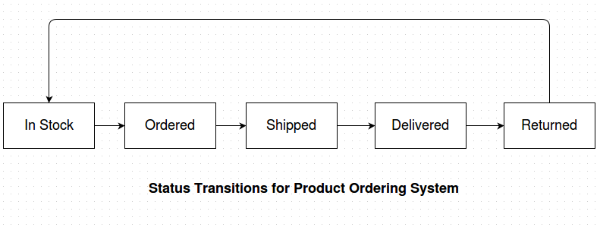
Product starts with a status of In Stock, and finishes with a status of Delivered or Returned. Each status transition can be used to execute various tasks. For example when the Product status changes from In Stock to Ordered, we can update the stock values. So the action to be used for this scenario is {In stock}_to_{ordered}. We can do similar activities on other status transitions to improve the process.
Library Management System
This is a another scenario where custom statuses become very important. In a library system, the status of a book changes according to the activities done by library members. In such a system a book can have statuses like Borrowed, Renewed, Available, and Overdue. Let's consider the following screen for possible status transitions.
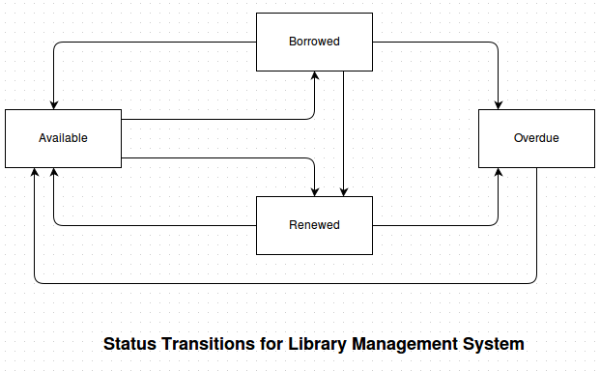
In this scenario, status transitions have become much more complex compared to the previous scenario. A book starts its process from the Available status and switches between other statuses until it returns back to the Available status. Let's consider a scenario for using post status transitions in this system.
Generally, there is a maximum limit for the number of renewals of a single book. So when the book status changes from Renewed to Available, we can check the member account to see if the member has already reached the maximum limit and block the member from renewing further.
Here, we discussed two scenarios for the need of custom status transitions. Real applications are much more complex and hence you will find many occasions for the need of custom status transitions.
Wrap Up
Post status transitions are a very powerful way of adding new features or managing the work flow in applications, but there are some drawbacks with this technique. Consider a situation where you need to send a large number of notifications in a single post status transition.
In such cases you won't be able to complete the status transition until all the notifications are sent, so it will become a difficult task to publish posts. Generally, post status transitions should not be used for extensive processes which take considerable amounts of time. It's up to you to choose wisely based on the requirements.
Now I have few questions for you, and I hope you all can share your knowledge by answering these questions:
- Do you want WordPress to support custom statuses by default? and Why?
- What are the other real world practical scenarios for using post status transitions?
- What are the types of features that you would like to provide with post status transitions?
- How would you plan to implement an extensive process with post status transitions?
Looking forward to hearing from you.
Comments