People format date information in a variety of ways and this makes collecting raw date information from users a bit tricky. The best way to guarantee valid data is to provide a control that enforces certain rules about how a date is formatted. One way to do this is by using a special date dialog to collect input.
Date dialogs, such as the one shown below, are generated from a special AlertDialog class called DatePickerDialog (android.app.DatePickerDialog). In addition to having all the features of the AlertDialog class, you can use the DatePickerDialog to collect standardized date input from the user. In this quick tutorial, we provide the steps required to implement a date picker dialog to collect a date (such as a birthday) from the user.
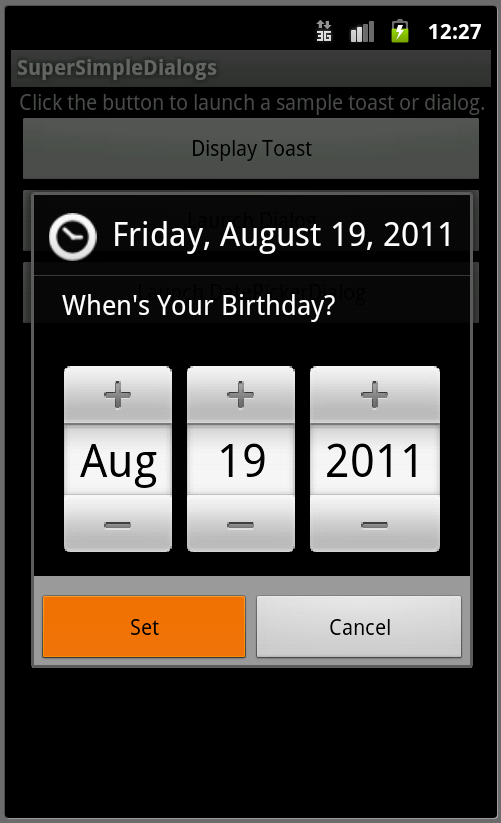
Prerequisites: Dialog Basics
We’ve already discussed how to add basic dialog controls to your Activity classes. This quick tutorial builds upon your knowledge from the tutorial Android User Interface Design: Working With Dialogs tutorial. You can either add this dialog to the dialog sample application provided in the above-mentioned tutorial, or create your own application.
Step 1: Defining a New Date Picker Dialog
Now let's add another new Dialog to your basic Activity class.
Edit your Activity Java class and add the following member variables:
private static final int MY_DATE_DIALOG_ID = 3;
This code defines a unique Dialog identifier for our Activity class. The value is arbitrary, but needs to be unique within the Activity.
Step 2: Creating the DatePickerDialog
To create Dialog instances, you must provide a case for your DatePickerDialog in the onCreateDialog() method of your Activity class. When the showDialog() method is called, it triggers a call to this method, which must return the appropriate Dialog instance. In the example code we have three different Dialogs in our class and thus we will need to check the incoming Dialog identifier to return the Dialog of the appropriate kind.
The specific case statement for your new DatePickerDialog within the onCreateDialog() method is shown here:
case MY_DATE_DIALOG_ID: DatePickerDialog dateDlg = new DatePickerDialog(this, new DatePickerDialog.OnDateSetListener() { public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) { Time chosenDate = new Time(); chosenDate.set(dayOfMonth, monthOfYear, year); long dtDob = chosenDate.toMillis(true); CharSequence strDate = DateFormat.format("MMMM dd, yyyy", dtDob); Toast.makeText(SuperSimpleDialogsActivity.this, "Date picked: " + strDate, Toast.LENGTH_SHORT).show(); }}, 2011,0, 1); dateDlg.setMessage("When's Your Birthday?"); return dateDlg;
Here we create a new instance of a DatePickerDialog, configure it, and return it. The configuration is simple. We include an implementation of the onDateSet() method, which is called when the user selects a specific date and presses the positive dialog button. We also set the dialog’s message to prompt the user for a birth date.
Note: We use the Time class (android.text.format.Time) and DateFormat class (android.text.format.DateFormat) to convert between machine and human readable date formats.
Step 3: Initializing the DatePickerDialog
Recall that an Activity keeps Dialogs around and reuses them whenever they are shown. Because we want to initialize the DatePickerDialog to today’s date, we should initialize it in the onPrepareDialog() method of the Activity. Otherwise, the second time you launch the DatePickerDialog, it will be set to the date chosen the previous time. Let’s assume we want to reuse this dialog, so we want to reset it to the current date each time the dialog is shown. To do this, we must update the onPrepareDialog() method of the Activity. Here is the case statement for the specific DatePickerDialog within this method:
case MY_DATE_DIALOG_ID: DatePickerDialog dateDlg = (DatePickerDialog) dialog; int iDay,iMonth,iYear; Calendar cal = Calendar.getInstance(); iDay = cal.get(Calendar.DAY_OF_MONTH); iMonth = cal.get(Calendar.MONTH); iYear = cal.get(Calendar.YEAR); dateDlg.updateDate(iYear, iMonth, iDay); break;
Here we use the Calendar class (java.util.Calendar) to set the DatePickerDialog control to today’s date each time the dialog is shown with the updateDate() method. Note that the month value is a 0-based month number (i.e. October would be month 9, not month 10).
Step 4: Triggering the DatePickerDialog
Finally, you are ready to trigger your DatePickerDialog to display as required. For example, you might add another Button control to your Activity’s screen to trigger your DatePickerDialog to display. Its click handler might look something like this:
public void onDateDialogButtonClick(View v) { showDialog(MY_DATE_DIALOG_ID); }
Conclusion
Dialogs are a powerful user interface tool that can help you keep your application user interface flexible and uncluttered. DatePickerDialogs are especially helpful for gathering date input from the user in a reproducible and standardized fashion. A DatePickerDialog is a special type of AlertDialog with all its features.
About the Authors
Mobile developers Lauren Darcey and Shane Conder have coauthored several books on Android development: an in-depth programming book entitled Android Wireless Application Development, Second Edition and Sams Teach Yourself Android Application Development in 24 Hours, Second Edition. When not writing, they spend their time developing mobile software at their company and providing consulting services. They can be reached at via email to [email protected], via their blog at androidbook.blogspot.com, and on Twitter @androidwireless.
Comments