Frame layouts are one of the simplest layout types used to organize controls within the user interface of an Android application.
Understanding layouts is important for good Android application design. In this tutorial, you learn all about frame layouts, which are primarily used to organize individual or overlapping view controls on the screen. When used correctly, frame layouts can be the fundamental layout upon which many interesting Android application user interfaces can be designed.
What Is A Frame Layout?
Frame layouts are one of the simplest and most efficient types of layouts used by Android developers to organize view controls. They are used less often than some other layouts, simply because they are generally used to display only one view, or views which overlap. The frame layout is often used as a container layout, as it generally only has a single child view (often another layout, used to organize more than one view).
TIP: In fact, one place you’ll see frame layouts used is as the parent layout of any layout resource you design. If you pull up your application in the Hierarchy Viewer tool (a useful tool for debugging your application layouts), you’ll see that any layout resources you design are displayed within a parent view-a frame layout.
Frame layouts are very simple, which makes them very efficient. They can be defined within XML layout resources or programmatically in the application's Java code. A child view within a frame layout is always drawn relative to the top left-hand corner of the screen. If multiple child views exist, then they are drawn, in order, one atop the other. This means that the first view added to the frame layout will display on the bottom of the stack, and the last view added will display on top.
Let’s look at a simple example. Let’s say we have a frame layout that is sized to control the entire screen (in other words, layout_width and layout_height attributes are both set to match_parent). We could then add three child controls to this frame layout:
- An ImageView with a picture of a lake.
- A TextView with some text to display towards the top of the screen.
- A TextView with some text to display towards the bottom of the screen (Simply use the layout_gravity attribute to have the TextView “sink” to the bottom of the parent).
The following figure shows what this sort of layout would look like on the screen.
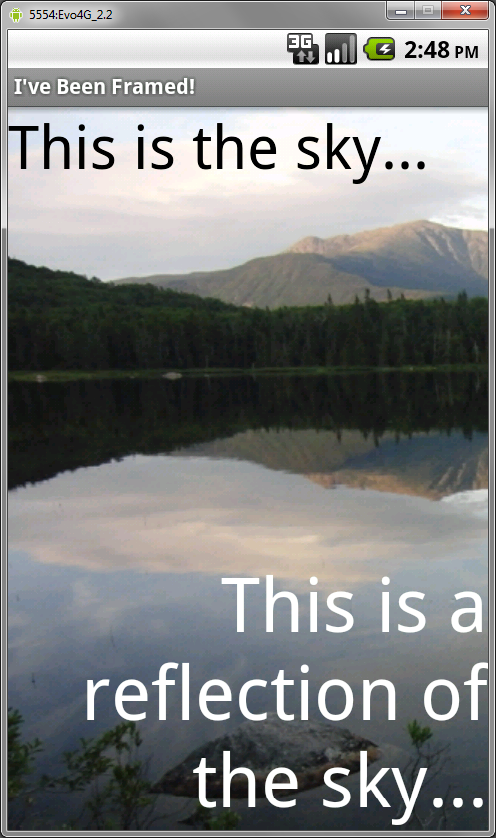
Defining an XML Layout Resource with a Frame Layout
The most convenient and maintainable way to design application user interfaces is by creating XML layout resources. This method greatly simplifies the UI design process, moving much of the static creation and layout of user interface controls and definition of control attributes, to the XML, instead of littering the code.
XML layout resources must be stored in the /res/layout project directory hierarchy. Let’s take a look at the simple frame layout introduced in the previous section. Again, this screen is basically a frame layout with three child views: an image that fills the entire screen, upon which two text controls are drawn, each with the default, transparent background. This layout resource file, named /res/layout/framed.xml, is defined in XML as follows:
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent"> <ImageView android:id="@+id/ImageView01" android:layout_height="fill_parent" android:layout_width="fill_parent" android:src="@drawable/lake" android:scaleType="matrix"></ImageView> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:textColor="#000" android:textSize="40dp" android:text="@string/top_text" /> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/bottom_text" android:layout_gravity="bottom" android:gravity="right" android:textColor="#fff" android:textSize="50dp" /> </FrameLayout>
Recall that, from within the Activity, only a single line of code within the onCreate() method is necessary to load and display a layout resource on the screen. If the layout resource was stored in the /res/layout/framed.xml file, that line of code would be:
setContentView(R.layout.framed);
Defining a Frame Layout Programmatically
You can also programmatically create and configure frame layouts. This is done using the FrameLayout class (android.widget.FrameLayout). You’ll find the frame-specific parameters in the FrameLayout.LayoutParams class. Also, the typical layout parameters (android.view.ViewGroup.LayoutParams), such as layout_height and layout_width, as well as margin parameters (ViewGroup.MarginLayoutParams), still apply to FrameLayout objects.
Instead of loading a layout resource directly using the setContentView() method as shown earlier, you must instead build up the screen contents in Java and then supply a parent layout object which contains all the control contents to display as child views to the setContentView() method. In this case, your parent layout would be the frame layout.
For example, the following code illustrates how to reproduce the same layout described earlier programmatically. Specifically, we have an Activity instantiate a FrameLayout and place one ImageView control followed by two TextView controls within it in its onCreate() method:
public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); TextView tv1 = new TextView(this); tv1.setText(R.string.top_text); tv1.setTextSize(40); tv1.setTextColor(Color.BLACK); TextView tv2 = new TextView(this); tv2.setLayoutParams(new LayoutParams(LayoutParams.FILL_PARENT, LayoutParams.WRAP_CONTENT, Gravity.BOTTOM)); tv2.setTextSize(50); tv2.setGravity(Gravity.RIGHT); tv2.setText(R.string.bottom_text); tv2.setTextColor(Color.WHITE); ImageView iv1 = new ImageView(this); iv1.setImageResource(R.drawable.lake); iv1.setLayoutParams(new LayoutParams(LayoutParams.FILL_PARENT, LayoutParams.FILL_PARENT)); iv1.setScaleType(ScaleType.MATRIX); FrameLayout fl = new FrameLayout(this); fl.setLayoutParams(new LayoutParams(LayoutParams.FILL_PARENT, LayoutParams.FILL_PARENT)); fl.addView(iv1); fl.addView(tv1); fl.addView(tv2); setContentView(fl); }
The resulting screen looks exactly the same as the figure shown previously.
When to Use Frame Layouts
With other powerful layout types like linear layouts, relative layouts, and table layouts at your disposal, it’s easy to forget about frame layout. The efficiency of a frame layout makes it a good choice for screens containing few view controls (home screens, game screens with a single canvas, and the like). Sometimes other inefficient layout designs can be reduced to a frame layout design that is more efficient, while other times a more specialized layout type is appropriate. Frame layouts are the normal layout of choice when you want to overlap views.
Looking at Similar Controls
FrameLayout is relatively simple. Because of this, numerous other layout types and view controls are based upon it. For instance, ScrollView is simply a FrameLayout that has scrollbars when the child content is too large to draw within the bounds of the layout. All Home screen app widgets reside within a FrameLayout.
One notable addition to all FrameLayouts is that they can take a foreground drawable in addition to the normal background. This is done via the android:foreground XML attribute. This could be used for, quite literally, a frame around the underlying views.
Conclusion
Android application user interfaces are defined using layouts, and frame layouts are one of the simplest and most efficient layout types available. The child control(s) of a frame layout are drawn relative to the top left-hand corner of the layout. If multiple child views exist within the frame layout, they are drawn in order, with the last child view on top.
Comments