When working with Flash and Actionscript 3.0 you may often find yourself repeating code from one project to the next. Creating your own library of reusable code snippets (on snipplr.com for example) is a great way to speed up development. Check out these 15 snippets which you may find useful for integrating into your own code library.
Some of the following snippets are presented here as functions which can simply be used as-is. While you should be able to drop these into your own code as needed, I highly recommend you take the time to really look at the code to get a deeper understanding of what is happening. Looking at code snippets is a great way to learn new techniques and improve your programming skills overall.
Introducing Snipplr.com
Snipplr is one of Envato's most recent acquisitions. A code snippet repository, a social hub and a darn useful addition to the Envato toolbelt! With Snipplr you can keep all of your frequently used code snippets in one place, accessible from any computer. You can share your code with other visitors and make use of what they post.
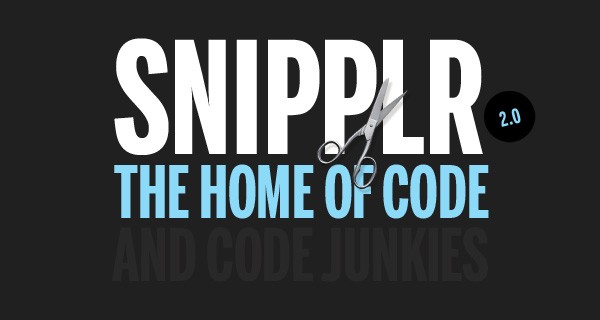
You can follow @snipplr on Twitter and keep up to date via the new Snipplr Facebook page. If you have any of your own AS3 snippets you'd like to share, add them to Snipplr and leave the url in the comments!
1. Randomize an Array
To randomize an array we loop over the length of the array, removing an object chosen at random and then adding it back onto the Array's end position. Think of this as having a deck of cards where you pick a card at random from the deck and move it to the top of the stack repeated for the total number of cards in the deck.
It's important to note that the splice method returns an Array containing the removed object and not the object itself hence adding the [0]
after the splice call to reference the contained object.
2. Position Display Objects in a Grid Layout
This is a short way of positioning display objects in a grid layout. It makes use of the modulo operator (%
) to position each display object along the x axis and the floor
method of the Math class for the y position.
This example creates 20 instances of a custom display object called MyDisplayObject
positioning each instance in a grid 5 columns wide. As you may have guessed, the two 5's in the snippet represent the number of columns in the grid.
3. Remove All Children From a DisplayObjectContainer
DisplayObjectContainer
, a parent class of the more common container classes such as MovieClip and Sprite, does not have a built in method to immediately remove all children. To remove all children we simply use a while loop to remove the child which occupies index 0 in the stack until no children remain.
4. Get URL of the Page Where SWF is Embedded
To get the complete URL as it appears in your web browser's address bar we use AS3's ExternalInterface
class. Without going into too much detail, know that in this example we use ExternalInterface to access the DOM (Document Object Model) of the HTML page which contains our Flash.
A downside of ExternalInterface is that the SWF must be embedded in the HTML page with the "allowscriptaccess" parameter set to either "sameDomain" or "always". This is OK when you control the embedding of a SWF but unfortunately cannot be relied upon if the SWF may be run on third party websites. Don’t forget to add import flash.external.ExternalInterface
at the top of your document.
Alternatively, to access the URL where the SWF resides we can access the url property of the LoaderInfo
object belonging to our SWF's root. Note this is the location of the SWF file and NOT the HTML page it's embedded in. It's important to make this distinction as both the SWF and HTML could reside on different domains.
Knowing where a SWF file is running is useful for showing domain specific content. In the context of a Flash game this might be having 'bonus' levels only available when the SWF is running on the developer's site or whether ads are displayed or not.
5. Round the Positional Values of a DisplayObjectContainer and its Children
This is a recursive function which takes a DisplayObjectContainer
(such as a Sprite or MovieClip) and rounds the x and y values of it and its children. It's recursive as the function calls itself when it encounters a child which is also a DisplayObjectContainer
so its children also get rounded. This recurses the entire display list beneath the passed DisplayObjectContainer
, its children, its children's children etc.
The point of this function is to make sure the passed DisplayObjectContainer
and all its children sit on whole pixels. This can be important when dealing with graphics which aren't sitting on whole pixels as it will cause them to appear blurry. This is probably most noticeable when dealing with pixel fonts.
You'll notice the first if statement checks to see if the passed DisplayObjectContainer
is NOT the stage. This is needed as Stage inherits from DisplayObjectContainer
but does not implement the x and y properties (along with several other properties). This allows you to safely pass the stage as a parameter of this function which will in-turn round the position of all objects on the display list.
6. Random Number Between Two Values
Generating a random number is often useful when developing applications in AS3 (more so when developing games). AS3 has a built in random number method as a part of its Math class which generates a number less than 1 and greater than or equal to 0. We can use this to create a useful function for generating a random number between two other numbers.
7. Random Boolean
Similarly to needing a random number, you may also require a random Boolean for use in an expression. Again we use the random
method of the Math class to simply return true if random()
generates a number greater than or equal to 0.5 or false if less.
8. Find the Angle Between Two Points
This is another common snippet used by game developers for finding the angle between two points on a plane. This function has four parameters; the first pair being the x and y values of your first point and the second pair being the x and y values of your second point.
It's important to note this function returns the angle in radians NOT degrees.
9. Converting Between Radians and Degrees
When dealing with equations involving angles you will often be dealing in radians and not degrees. Display objects in Flash use degrees when dealing with their rotation property so we need a way to convert between the two. These helper function are useful for accomplishing just that; one for converting radians to degrees and the other degrees to radians.
10. Email Validation
When accepting input from a user through a form it is often necessary to validate data before allowing it to be sent to a back-end script. This is most true for email addresses as they are often the only point of contact between yourself and the user and are important to get right. The below function makes use of a regular expression to confirm the email is of a valid format.
If you are unsure of what a regular expression is be sure to read this article on wikipedia as well as the AS3 documentation for the RegExp type. Be sure to also check out Validating Various Input Data in Flash.
11. Space Removal
In the same way you often need to format data being sent from Flash, you sometimes need to format data being received. This function takes a string and removes all spaces from it. Here we use the split method of the String class to split the string into an Array and then call the join method of the Array class to convert it back to a string. This is useful when dealing with a string you know cannot contain spaces such as an email address, URL or phone number. This returns a new string, leaving the input string unchanged.
If you are wondering why we do not use a regular expression here, it is simply because this method executes faster.
12. Slugify
Wikipedia describes a slug as
part of a URL which identifies a page using human-readable keywords
A slug is usually a few words with each word separated by a delimiting character, usually an underscore or hyphen. A slugified version of the string "10 Tips to a Better Life!" would be "10-tips-to-a-better-life".
This function takes an arbitrary string and converts in for use as a slug. We use two regular expressions to accomplish this, the first is used to remove any character which is not a word character, space or hyphen, the second replaces any spaces with a single hyphen. Finally we convert the string to lowercase. This returns a new string, leaving the input string unchanged.
If you're wondering where this might be used in the context of Flash it is particularly useful with SWFAddress for creating SEO friendly URLs for the discreet sections of your app.
13. Strip http://
or https://
From a String, Optionally Removing www.
This function takes a string and returns a copy with all occurrences of http://
and https://
removed. The string, "Please visit http://example.com to find out more" would convert to "Please visit example.com to find out more". Generally this is more compact as the http:// is redundant when you are obviously referring to a URL.
Optionally, this function can also strip the www
subdomain by passing true as the second parameter. It's set to false by default as example.com and www.example.com could theoretically contain different websites.
14. Strip HTML Markup
If there is ever a time when you load text from an external source containing unwanted HTML markup use the following function. This uses a regular expression to strip all HTML tags from the input string. The string "<strong>Click <a href='http://example.com'>here</a> to find out more</strong>" would simply be converted to "Click here to find out more.". This returns a new string, leaving the input string unchanged.
15. Strip XML Namespaces
Loading XML, specifically from 3rd party sources can sometimes prove problematic when the XML makes use of namespaces. This snippet will take an XML object and strip all namespace declarations and prefixes. This snippet leaves the input XML unchanged and returns a new XML object.
It is worth mentioning that namespaces exist for a reason and removing them may cause problems. This is usually only an issue when combining multiple XML files together from different sources. I personally have never experienced this but is still worth being wary of.
Conclusion
I hope you found these 15 snippets useful. Writing functional, reusable code can be a great habit to get into. I personally believe looking at other people's code snippets is a great way of learning tips and tricks you may not of thought of before.
To check out some more snippets head over to the Actionscript section at Snipplr and if you have any of your own AS3 snippets you'd like to share, add them to Snipplr and leave the url in the comments!
If you enjoyed this post please check out my site at ahrooga.com for more Flash related articles.
Comments