Have you ever wanted to learn how to make your applications more social with Facebook? It's much easier than you think!
In this tutorial, we'll be building an application that reads and publishes data to and from Facebook using Facebook's Graph API. Interested? Join me after the jump!
A Short Introduction to the Open Graph Protocol
The Open Graph protocol enables any web page to become a rich object in a social graph. For instance, this is used on Facebook to allow any web page to have the same functionality as any other object on Facebook.
While many different technologies and schemas exist and could be combined together, there isn't a single technology which provides enough information to richly represent any web page within the social graph. The Open Graph protocol builds on these existing technologies and gives developers one thing to implement. Developer simplicity is a key goal of the Open Graph protocol which has informed many of the technical design decisions.
In a nutshell, the Open Graph Protocol turns any web page into an object in a huge graph. Each object, at least in Facebook's graph objects, can have many other objects linked to it. For example, a Facebook Page
can have multiple Post
objects, which are posts made by that page. In turn, each Post
object can have multiple Comment
objects attached to it, referring to comments written by people on the post. This relationship between graph objects is the basis for Facebook's Graph API, which in turn allows us to do CRUD operations on these objects.
In this tutorial, we'll be learning how to use and integrate the Facebook Graph API into an application. We'll also learn how to use data from the Graph API to do operations like logging in a user via their Facebook account. Ultimately, we'll be creating a small application than allows people to create and read posts from a Facebook Page they're managing, similar to HootSuite or TweetDeck.
Step 1: Create a Facebook Application
The first thing you should do when you're planning to use the Facebook Graph API is to create a Facebook application. This doesn't necessarily mean that we'll be putting the application on Facebook (although we can); we just need a Facebook application (specifically an APP ID
and APP SECRET
) to access the API.
Open http://developers.facebook.com and click on the Apps
link in the navigation bar.
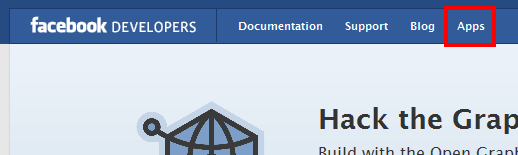
Facebook's Developer Site
You'll be prompted to log in (if you're not) and allow the Developer
application to access your account. Just click on Allow
and you'll be redirected to the Developer App
homepage.
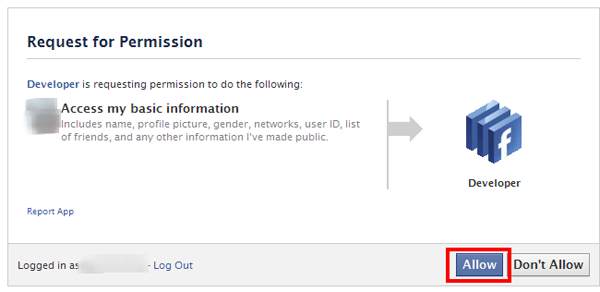
Allow the Developer application access
On the Developer App homepage, click on Create New App
in the upper right corner of the page.
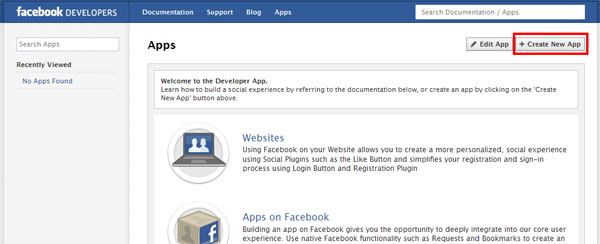
Developer App homepage
You'll be greeted with a modal window asking for an App Display Name
and App Namespace
. Provide anything you want here, but for the purposes of this tutorial, I'll be using Nettuts+ Page Manager
and nettuts-page-manager
respectively. Click on Continue
.
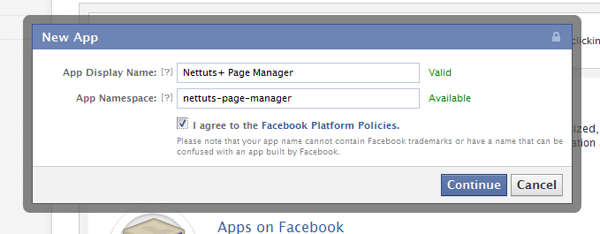
Birth of the Nettuts+ Page Manager
After some obligatory captcha checking, you'll be redirected to your newly-minted application's page. Here you'll see the APP ID
and APP SECRET
that we need. Copy and paste these values somewhere for later use.
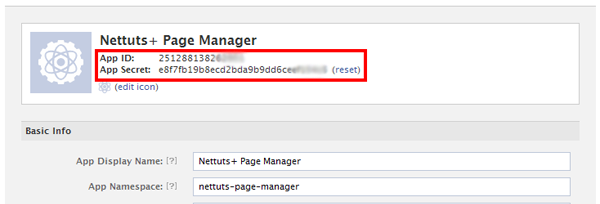
The APP ID and APP SECRET
When that's done, go to the lower part of the page and click on the “Website” box, and below it should appear a form that asks for a Site URL
. Since I'm just using my local machine to build the application, I'll use http://localhost/nettuts/pagemanager
. When you're done, click on the Save Changes
button below.
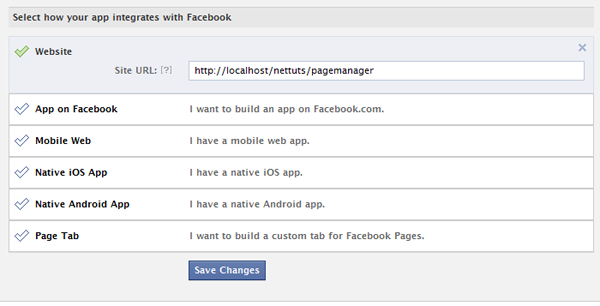
Nettuts+ Page Manager Settings
Step 2: Download and Set Up Facebook's PHP SDK
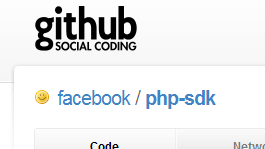
Facebook's GitHub page
Our next task is to download and set up Facebook's PHP SDK. The best location to get it would be on Facebook's GitHub page, since this is where the latest and greatest version of the PHP SDK will be.
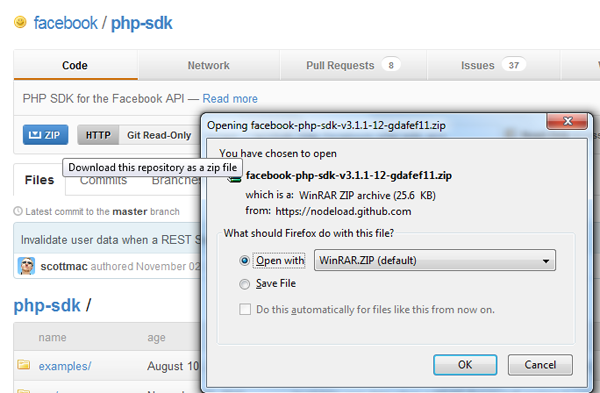
Downloading Facebook's PHP SDK
Point your browser to https://github.com/facebook/php-sdk and click on the “ZIP” button. This should prompt a download for the latest version of the SDK. Save it anywhere you like.
Now, extract this into PHP's include_path
to make it accessible by any application. Alternatively, if you're just using this for one application, extract it inside the application's folder — just make sure to take note where, since we'll have to include facebook.php
in our code later.
Step 3: Read from Facebook via the Graph API
Let's start creating our project and using the Facebook Graph API to read information from Facebook. For starters, create an index.php
file where a user can log in via Facebook. The index.php
file should contain the following code:
<!DOCTYPE html> <html> <head> <title>Nettuts+ Page Manager</title> <link rel="stylesheet" href="css/reset.css" type="text/css" /> <link rel="stylesheet" href="css/bootstrap.min.css" type="text/css" /> <script src="js/jquery.min.js"></script> <style> body { padding-top: 40px; } #main { margin-top: 80px; text-align: center; } </style> </head> <body> <div class="topbar"> <div class="fill"> <div class="container"> <a class="brand" href="/">Nettuts+ Page Manager</a> </div> </div> <div id="main" class="container"> <a href="connect.php" class="btn primary large">Login via Facebook</a> </div> </body> </html>
If you're wondering, reset.css
is just your standard stylesheet reset, and bootstrap.min.css
is Twitter Bootstrap. I've also added jQuery into the mix to make it easier to do client-side stuff. Now, if you refresh the page, it should look something like this:
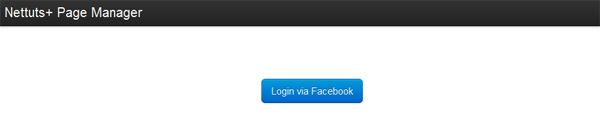
Nettuts+ Page Manager First Run
Now let's create our connect.php
file, which will enable us to connect a user's Facebook account and the pages that he or she manages. Let's start by including the Facebook library we downloaded earlier. Instantiate it using the APP ID
and APP SECRET
:
//include the Facebook PHP SDK include_once 'facebook.php'; //instantiate the Facebook library with the APP ID and APP SECRET $facebook = new Facebook(array( 'appId' => 'REPLACE WITH YOUR APP ID', 'secret' => 'REPLACE WITH YOUR APP SECRET', 'cookie' => true ));
The $facebook
variable can now be used to make API calls to Facebook on behalf of the application.
- The
appID
setting tells Facebook which application we're using. - The
secret
setting “authenticates” our API calls, telling Facebook that they came from someone who owns the application. This shouldnever
be shown to the public, which is why it's named the “Application Secret.” - The
cookie
setting tells the library to store the user's session using cookies. Without it, we won't be able to know whether the user is logged in via Facebook or not.
Now, we check if the current user has already allowed access to the application. If not, the application has to redirect them to Facebook's “Allow Permissions” page.
//Get the FB UID of the currently logged in user $user = $facebook->getUser(); //if the user has already allowed the application, you'll be able to get his/her FB UID if($user) { //do stuff when already logged in } else { //if not, let's redirect to the ALLOW page so we can get access //Create a login URL using the Facebook library's getLoginUrl() method $login_url_params = array( 'scope' => 'publish_stream,read_stream,offline_access,manage_pages', 'fbconnect' => 1, 'redirect_uri' => 'http://'.$_SERVER['HTTP_HOST'].$_SERVER['REQUEST_URI'] ); $login_url = $facebook->getLoginUrl($login_url_params); //redirect to the login URL on facebook header("Location: {$login_url}"); exit(); }
Essentially, this is all that happens here:
- The application makes a simple API call to get the user's Facebook User ID (also known as
FB UID
) via the$facebook->getUser()
method. - If the
$user
variable has a value, it means that the user has already allowed basic permissions for the application. - If not, then it means the user hasn't allowed the application permissions yet, and the application needs to redirect to Facebook's permissions page to get the necessary permissions.
-
It then generates a
Login URL
, which is where the application should redirect the user to show Facebook's permissions page for the application. ThegetLoginUrl()
method takes in the following parameters:-
scope
- this is a comma-delimited list of permissions the application needs -
fbconnect
- this should be1
to tell Facebook that the application will be using Facebook to authenticate the user -
redirect_uri
- this is the page Facebook redirects to after the user has gone through the Facebook permissions page
You can read more about the
getLoginUrl()
method here: https://developers.facebook.com/docs/reference/php/facebook-getLoginUrl/ -
- Afterwards, the application redirects the user to the
Login URL
and the user sees Facebook's permissions page.
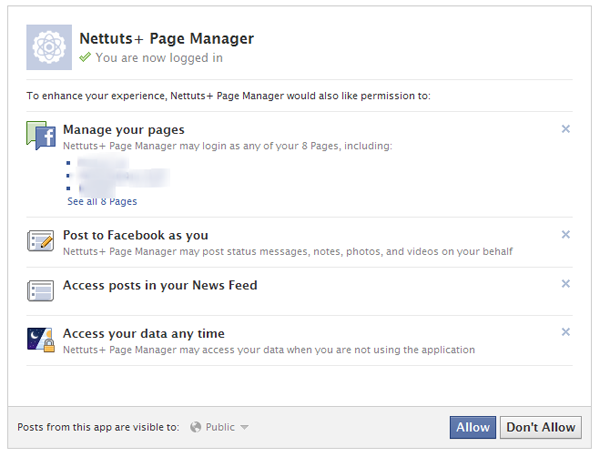
Facebook's Permissions Page
Facebook Permissions
Let's take a minute to talk about Facebook permissions. Similar to when you install an application on your Android phone, Facebook permissions are various levels of access a Facebook application can do on behalf of a user. For example, if we wanted access to user's email address, we can add the email
permission to the scope
setting we use to generate the login URL.
Remember, with great power comes great responsibility, so make sure to only use your permissions for good, not for evil.
It's important that your application only asks a user for permissions that it actually needs. If you only need to authenticate a user via Facebook, you don't even need to ask any permissions at all! Remember, with great power comes great responsibility, so make sure to only use your permissions for good, not for evil.
In the app, we use the following permissions:
-
publish_stream
- allows the application to publish updates to Facebook on the user's behalf -
read_stream
- allows the application to read from the user's News Feed -
offline_access
- converts theaccess_token
to one that doesn't expire, thus letting the application make API calls anytime. Without this, the application'saccess_token
will expire after a few minutes, which isn't ideal in this case -
manage_pages
- lets the application access the user's Facebook Pages. Since the application we're building deals with Facebook Pages, we'll need this as well.
There are a lot more permissions Facebook requires for different things. You can read all about them in Facebook's documentation for permissions.
Going back to the application, now that the user has allowed the permissions required by the application, we can do some API calls to Facebook! Place this inside the if-else
block from the code above:
<pre> //start the session if needed if( session_id() ) { } else { session_start(); } //get the user's access token $access_token = $facebook->getAccessToken(); //check permissions list $permissions_list = $facebook->api( '/me/permissions', 'GET', array( 'access_token' => $access_token ) ); </pre>
The first thing we do is get the $access_token
for the user that the application just authenticated. This is crucial, as the application will need this access token for almost everything we do. To get it, we use the getAccessToken()
method. The $access_token
acts as a 'password' for the user. It's always unique for every user on every application, so make sure to store this when needed.
Afterwards, we can see how to make API calls to Facebook using the api()
method. This method takes in the following parameters:
-
$graph_path
- this is the Facebook graph path, which is essentially the “URL” to the open graph object we want to access. This can be any graph object on Facebook, like aPost
(e.g. '/<post_id>'), aComment
(e.g. '/<comment_id>'), or even aUser
('/me' is a shortcut for the current user whom the$access_token
belongs to. It can also be '/<user_name>' or '/<fb_uid>', but the$access_token
you're usingmust
have access to that user, or you'll only be able to get the user's public information). -
$method
- this is the kind of method you want to do. It's usuallyGET
when you're trying to “get” information, andPOST
when you're trying to “post” or update information andDELETE
if you want to remove information. If you're not sure, the Graph API documentation tells you which method to use for specific API calls. -
$params
- these are the parameters that come with your API request. Usually forGET
methods you only need to supply the user's$access_token
. ForPOST
methods though, you'll also need to provide other parameters. For example, if you want to post a new status update, you'll provide the status update message here.
Alternatively, we can also use the api()
method to execute FQL (Facebook Query Language) queries
, which lets us get data via SQL-style language. This is great for retrieving information not available in the Graph API, as well as running multiple queries in one call. For example, we can get a user's name and other details through this FQL API call:
$fql = 'SELECT name from user where uid = ' . $fb_uid; $ret_obj = $facebook->api(array( 'method' => 'fql.query', 'query' => $fql, ));
We won't need this for this tutorial, but it's good to keep this in mind when you come across something the Graph API can't get.
Now that we have the list of permissions the user has allowed for the application, we need to check if the user gave the application all the necessary ones. Since Facebook's permissions page allows users to revoke certain permissions, we need to make sure that all of them have been allowed to make sure the application works. If the user has revoked one of the permissions, we'll redirect them back to the permissions page.
//check if the permissions we need have been allowed by the user //if not then redirect them again to facebook's permissions page $permissions_needed = array('publish_stream', 'read_stream', 'offline_access', 'manage_pages'); foreach($permissions_needed as $perm) { if( !isset($permissions_list['data'][0][$perm]) || $permissions_list['data'][0][$perm] != 1 ) { $login_url_params = array( 'scope' => 'publish_stream,read_stream,offline_access,manage_pages', 'fbconnect' => 1, 'display' => "page", 'next' => 'http://'.$_SERVER['HTTP_HOST'].$_SERVER['REQUEST_URI'] ); $login_url = $facebook->getLoginUrl($login_url_params); header("Location: {$login_url}"); exit(); } }
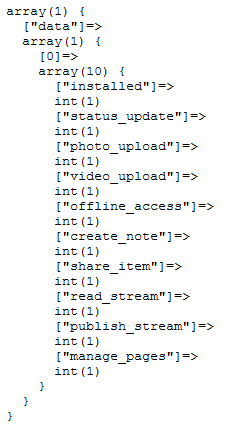
var_dump()
of the $permissions_list
variable
When that's done, it means we're all set and we'll be able to run the application without any problems. Let's start by doing another API call, this time to retrieve the list of Facebook Pages the user has administrative rights to, and saving these into a session variable. Afterwards, we'll redirect the user to the manage.php
page, where the page management code will be.
//if the user has allowed all the permissions we need, //get the information about the pages that he or she managers $accounts = $facebook->api( '/me/accounts', 'GET', array( 'access_token' => $access_token ) ); //save the information inside the session $_SESSION['access_token'] = $access_token; $_SESSION['accounts'] = $accounts['data']; //save the first page as the default active page $_SESSION['active'] = $accounts['data'][0]; //redirect to manage.php header('Location: manage.php');
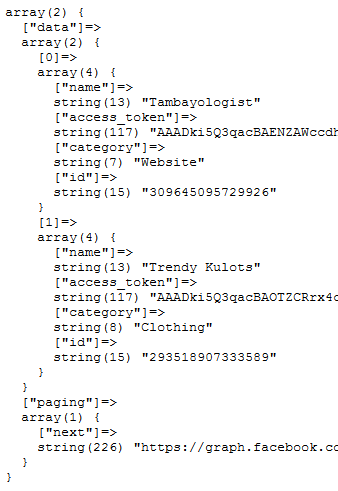
var_dump()
of the $accounts
variable
The /me/accounts
graph path gives us a list of the pages that a user has administrative rights to. This returns an array of all of the pages, plus specific $access_token
s for each of these pages. With those $access_token
s, we'll be able to do API calls on behalf of the Facebook Page as well!
Save these in the $_SESSION
array and redirect to the manage.php
page.
Let's start working on our manage.php
file. Remember that we've saved the user's $accounts
list into the $_SESSION
array, as well as set the first account on the list as the default active page. Let's GET
that account's news feed and display it:
//include the Facebook PHP SDK include_once 'facebook.php'; //start the session if necessary if( session_id() ) { } else { session_start(); } //instantiate the Facebook library with the APP ID and APP SECRET $facebook = new Facebook(array( 'appId' => 'APP ID', 'secret' => 'APP SECRET', 'cookie' => true )); //get the news feed of the active page using the page's access token $page_feed = $facebook->api( '/me/feed', 'GET', array( 'access_token' => $_SESSION['active']['access_token'] ) );
Again, the application does the intialization of the Facebook library, and then another api()
call, this time to /me/feed
. Take note that instead of using the user's access token
, we use the active page's access token
(via $_SESSION['active']['access_token']
). This tells Facebook that we want to access information as the Facebook Page and not as the user.
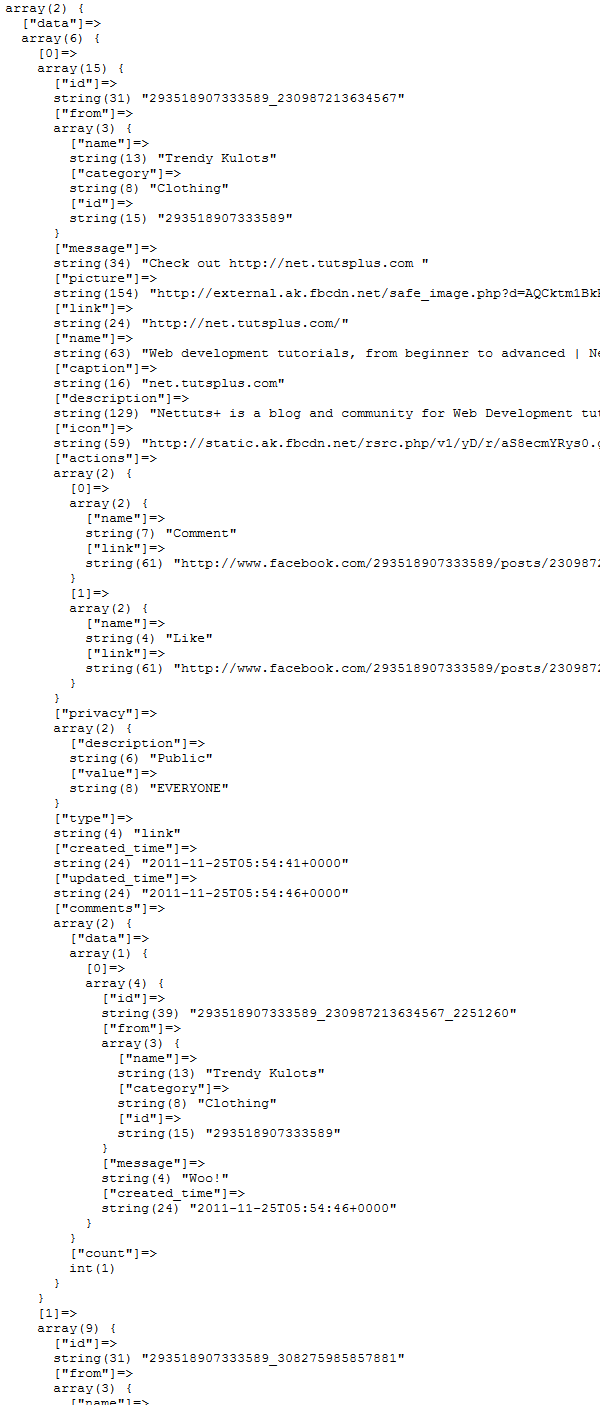
var_dump()
of the $page_feed
variable
Wow, that's a lot of information about a Facebook Post
. Let's dissect a single Facebook Post
object and see what it's made of.
The Facebook Post
Object
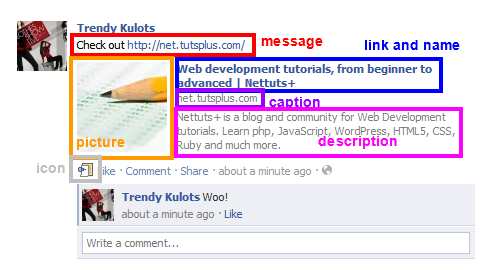
Facebook Post Cheat Sheet
-
id
- this is thePost
's ID -
from
- contains information about the user who posted the message -
message (red)
- the message component of the post -
picture (orange)
- a link to the photo attached to the post -
name (blue)
- the “title” of the Facebook post -
link (also blue)
- the link to where thename
goes to when clicked -
caption (purple)
- a few words to describe the link -
description (pink)
- more than a few words to describe the link -
icon (grey)
- a link to the icon image used -
actions
- Facebook actions you can do to the post, such asLiking
it orCommenting
on it. -
privacy
- the privacy of the post -
type
- the type of the post. A post can be astatus
,link
,photo
orvideo
. -
created_time
- the time when the post was created -
updated_time
- the time when the post was updated -
comments
- comments on the post
It would be wise to keep a copy of the cheat sheet above, since we'll be using that again when we publish Post
objects to Facebook.
Moving on, let's display the news feed in a more pleasing way. Add the following HTML below the PHP code:
<!DOCTYPE html> <html> <head> <title>Nettuts+ Page Manager</title> <link rel="stylesheet" href="css/reset.css" type="text/css" /> <link rel="stylesheet" href="css/bootstrap.min.css" type="text/css" /> <script src="js/jquery.min.js"></script> <style> body { padding-top: 40px; background-color: #EEEEEE; } img { vertical-align: middle; } #main { text-align: center; } .content { background-color: #FFFFFF; border-radius: 0 0 6px 6px; box-shadow: 0 1px 2px rgba(0, 0, 0, 0.15); margin: 0 -20px; padding: 20px; } .content .span6 { border-left: 1px solid #EEEEEE; margin-left: 0; padding-left: 19px; text-align: left; } .page-header { background-color: #F5F5F5; margin: -20px -20px 20px; padding: 20px 20px 10px; text-align: left; } </style> </head> <body> <div id="main" class="container"> <div class="content"> <div class="page-header"> <h1> <img width="50" src="http://graph.facebook.com/<?php echo $_SESSION['active']['id']; ?>/picture" alt="<?php echo $_SESSION['active']['name']; ?>" /> <?php echo $_SESSION['active']['name']; ?> <small><a href="http://facebook.com/profile.php?id=<?php echo $_SESSION['active']['id']; ?>" target="_blank">go to page</a></small> </h1> </div> <div class="row"> <div class="span10"> <ul id="feed_list"> <?php foreach($page_feed['data'] as $post): ?> <?php if( ($post['type'] == 'status' || $post['type'] == 'link') && !isset($post['story'])): ?> <?php //do some stuff to display the post object ?> <?php endif; ?> <?php endforeach; ?> </ul> </div> <div class="span6"> <h3>Post a new update</h3> </div> </div> </div> </div> </body> </html>
We make use of a simple Graph API trick here: the Picture
Graph API object. Basically, you can take the Graph path of any User
or Page
object (e.g. http://graph.facebook.com/293518907333589
), add /picture
in the end and you'll get a 50x50 photo of that object. For example:
https://graph.facebook.com/293518907333589/picture
Becomes...
Demo Picture Graph API Object
Going back, when we refresh the manage.php
page now, it should look something like this:
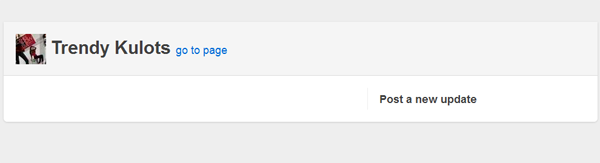
Add this inside the <?php foreach($page_feed['data'] as $post): ?>
to display the feed from the page:
<?php if( ($post['type'] == 'status' || $post['type'] == 'link') && !isset($post['story'])): ?> <li> <div class="post_photo"> <img src="http://graph.facebook.com/<?php echo $post['from']['id']; ?>/picture" alt="<?php echo $post['from']['name']; ?>"/> </div> <div class="post_data"> <p><a href="http://facebook.com/profile.php?id=<?php echo $post['from']['id']; ?>" target="_blank"><?php echo $post['from']['name']; ?></a></p> <p><?php echo $post['message']; ?></p> <?php if( $post['type'] == 'link' ): ?> <div> <div class="post_picture"> <?php if( isset($post['picture']) ): ?> <a target="_blank" href="<?php echo $post['link']; ?>"> <img src="<?php echo $post['picture']; ?>" width="90" /> </a> <?php else: ?> <?php endif; ?> </div> <div class="post_data_again"> <p><a target="_blank" href="<?php echo $post['link']; ?>"><?php echo $post['name']; ?></a></p> <p><small><?php echo $post['caption']; ?></small></p> <p><?php echo $post['description']; ?></small></p> </div> <div class="clearfix"></div> </div> <?php endif; ?> </div> <div class="clearfix"></div> </li> <?php endif; ?>
When you refresh the page again, it should look something like this:
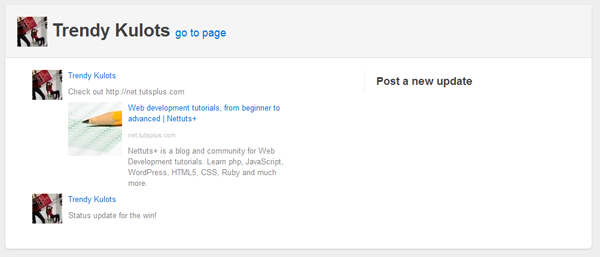
Looks good, right? We'll have to thank the Twitter Bootstrap
CSS for that!
Now, let's add a top navigation bar to help us switch from one page to another. Add the following HTML after the <body>
tag and before the <div id="main" class="container">
tag:
<div class="topbar"> <div class="fill"> <div class="container"> <a class="brand" href="/">Nettuts+ Page Manager</a> <ul class="nav secondary-nav"> <li class="dropdown" data-dropdown="dropdown"> <a class="dropdown-toggle" href="#">Switch Page</a> <ul class="dropdown-menu"> <?php foreach($_SESSION['accounts'] as $page): ?> <li> <a href="switch.php?page_id=<?php echo $page['id']; ?>"> <img width="25" src="http://graph.facebook.com/<?php echo $page['id']; ?>/picture" alt="<?php echo $page['name']; ?>" /> <?php echo $page['name']; ?> </a> </li> <?php endforeach; ?> </ul> </li> </ul> </div> </div> </div>
Don't forget to load the dropdown JavaScript library from Twitter Bootstrap's JavaScript page. You can download it here.
<script src="js/bootstrap-dropdown.js"></script> <script> $('.topbar').dropdown() </script>
Lastly, let's create the switch.php
file, where we'll set another page as the active page:
<?php session_start(); $page_id = $_GET['page_id']; foreach($_SESSION['accounts'] as $page) { if( $page['id'] == $page_id ) { $_SESSION['active'] = $page; header('Location: manage.php'); exit(); } } exit(); ?>
Refresh the manage.php
page again and you should see something like this:
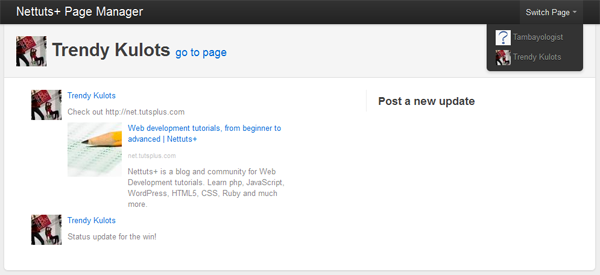
And now, by using our dropdown switcher, we're able to switch pages.
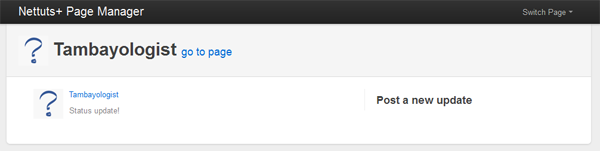
Step 4: Publish to Facebook via the Graph API
Now we're ready to publish new updates to our page! First of all, let's create the HTML form where we can set what we'll publish. Add this below the <h3>Post a new update</h3>
HTML:
<img src="post_breakdown.png" alt="Facebook Post Cheat Sheet" width="340" /><br /> <form method="POST" action="newpost.php" class="form-stacked"> <label for="message">Message:</label> <input class="span5" type="text" id="message" name="message" placeholder="Message of post" /> <label for="picture">Picture:</label> <input class="span5" type="text" id="picture" name="picture" placeholder="Picture of post" /> <label for="link">Link:</label> <input class="span5" type="text" id="link" name="link" placeholder="Link of post" /> <label for="name">Name:</label> <input class="span5" type="text" id="name" name="name" placeholder="Name of post" /> <label for="caption">Caption:</label> <input class="span5" type="text" id="caption" name="caption" placeholder="Caption of post" /> <label for="description">Description:</label> <input class="span5" type="text" id="description" name="description" placeholder="Description of post" /> <div class="actions"> <input type="submit" class="btn primary" value="Post" /> <input type="reset" class="btn" value="Reset" /> </div> </form>
Refresh to see how it looks:
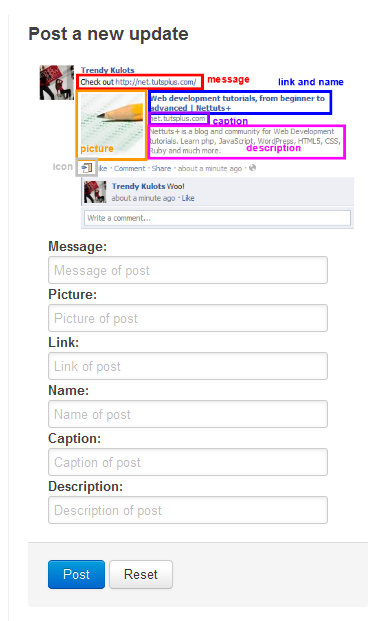
Loving Twitter Bootstrap's default form styles
The Post
cheat sheet should come in handy when we're figuring out what to put in the fields. Now, let's create the newpost.php
file where we'll actually use the Facebook Graph API to publish to the page's feed.
Start by creating the file and initializing the Facebook library like we did for the other pages:
<?php //include the Facebook PHP SDK include_once 'facebook.php'; //start the session if necessary if( session_id() ) { } else { session_start(); } //instantiate the Facebook library with the APP ID and APP SECRET $facebook = new Facebook(array( 'appId' => 'APP ID', 'secret' => 'APP SECRET', 'cookie' => true )); ?>
Afterwards, let's get all of the content we received from the POST
request:
//get the info from the form $parameters = array( 'message' => $_POST['message'], 'picture' => $_POST['picture'], 'link' => $_POST['link'], 'name' => $_POST['name'], 'caption' => $_POST['caption'], 'description' => $_POST['description'] );
Let's not forget to add the $access_token
for the page to the parameters:
//add the access token to it $parameters['access_token'] = $_SESSION['active']['access_token'];
Now that we have our $parameters
array, let's build and send our Graph API request!
//build and call our Graph API request $newpost = $facebook->api( '/me/feed', 'POST', $parameters );
Take note of what difference the $method
can make. A GET
API call to /me/feed
will return the news feed of that particular object, but a POST
API call to /me/feed
will post a new status update to the object.
To publish a new Post
object to Facebook, the application needs to make a call to the /<page_id or /me>/feed
graph path, and it needs to supply the following in the $parameters
array:
-
access_token
- the access token of the account we are publishing for -
message
- the message to publish -
picture
(optional) - a link to the photo of the post -
name
(optional) - the “title” of the post -
link
(optional) - a link to where thename
will go to when clicked -
caption
(optional) - a few words to describe the link/name -
description
(optional) - more than a few words to describe the link/name
You can see that the only parameters that are required are the access_token
and the message
parameters. By providing only these two, we can publish a simple status message, like so:
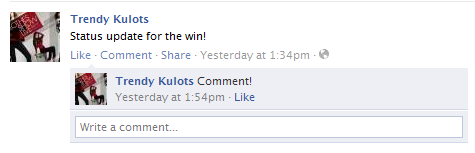
Status Update Example
Everything else is optional. However, if you provide a name
, link
, caption
, or decription
, you have to provide all four. As for picture
, if a parameter value is not provided or not accessible, the post's picture will be blank.
Finally, let's try publishing to Facebook using the application! Go back to the manage.php
page and fill up the form, then press Post
:
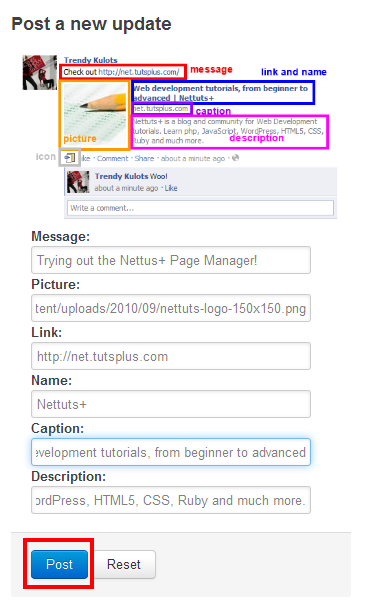
Afterwards, you should be redirected back to the manage.php
page, but there should be a new post on the feed!
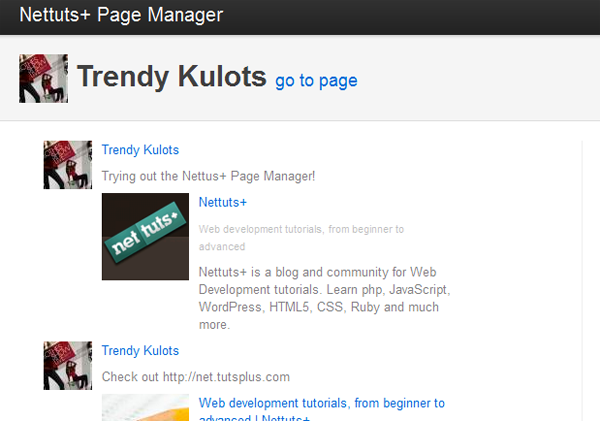
Publishing to Facebook successful!
You can also check the Facebook Page itself. You should see the new post there:
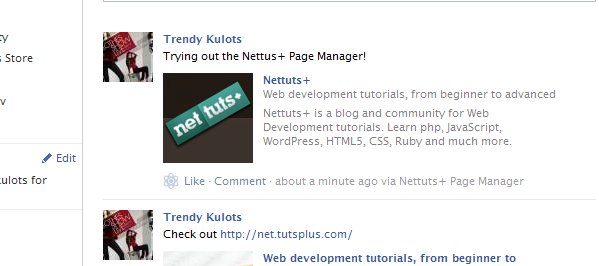
Publishing to Facebook Page
Conclusion
By now, you should already have a clear grasp on how to use the Facebook Graph API to both read and publish to Facebook. In this tutorial, we only cover the basics — there's a whole lot more you can do using the Graph API. Stuff like real-time updates
, which lets you subscribe to events that happen to your application's users (e.g. when a user changes his/her profile photo) or the Insights
Graph object, which lets you get the statistics of an application, page, or domain the user has access to.
Of course, the best resource for learning more about the Graph API is undoubtedly Facebook's Graph API documentation, but I also suggest taking a look at FBDevWiki, which is a third-party hosted wiki for Facebook Development documentation.
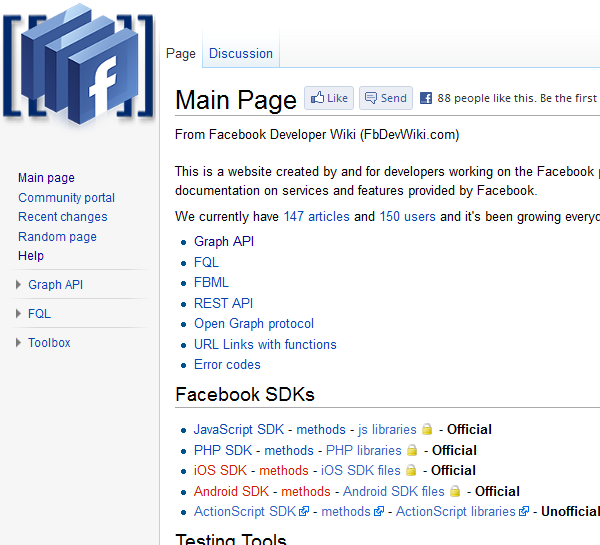
FBDevWiki.com
Additionally, there's also a special Facebook version of StackOverflow you can visit at http://facebook.stackoverflow.com. The questions here are all about Facebook and Facebook Development, so it's well worth visiting if you need any help or have any questions on the subject.
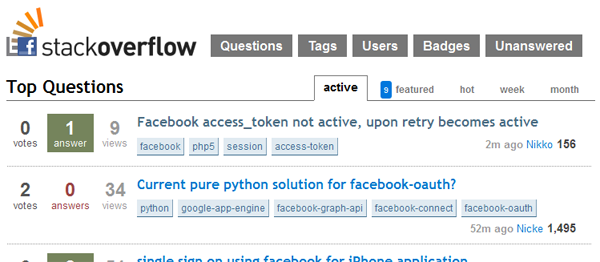
Facebook StackOverflow
Hopefully, you've learned from this tutorial how easy it is to use the Facebook Graph API
to make your applications more social.
Have you used the Facebook Graph API in a previous project, or are you planning to use it now? Tell me all about it in the comments below and thank you so much for reading!
Comments