To say that JavaScript is on the rise in web development would be an understatement. In fact, years ago, famed programmer Jeff Atwood coined Atwood's Law in which he stated:
Any application that can be written in JavaScript, will eventually be written in JavaScript.
At the time of writing this article, there are so many JavaScript frameworks and libraries that it's overwhelming to know where to start, especially if you're a beginner.
And I know, much of what we publish here is geared towards those who already have experience in writing web applications or doing something in web development. But that's that not the target audience for this article.
Instead, this is being written specifically for those of you who have never (or barely) written a line of JavaScript, and want to learn more about the language and understand what's out there. Furthermore, we want to cover how it's used and what to expect from it.
In short, if you're a seasoned professional, then this article isn't for you; however, if you're curious about getting into JavaScript but aren't sure where to start, then perhaps this primer will help set you in the right direction.
We've also built a comprehensive guide to help you learn JavaScript, whether you're just getting started as a web developer or you want to explore more advanced topics. Check out:
JavaScript Defined
You've likely heard JavaScript referred to as "a client-side scripting language", which is another way of saying that it's a programming language that runs in a web browser.
Alternatively, Wikipedia defines it this way:
JavaScript is a high-level, dynamic, untyped, and interpreted programming language. It has been standardized in the ECMAScript language specification.
All of the above is true (with varying degrees of complexity), but it's also worth noting that JavaScript can run on the server-side, too. That's getting ahead of ourselves, though. Instead, let's first talk about some of the points above and we'll come to talk about server-side JavaScript later in the article.
- High-Level. When a programming language is high-level, it's considered one that's built without needing to know finer details about the underlying computer. You don't have to manage memory, you don't have to know what type of processor is running, and you don't have to deal with things like pointers (such as in languages like C or Assembly).
-
Dynamic. Languages that are dynamic allow developers to extend certain aspects of the language by adding new code or introducing new objects (such as a
Post
object) while the program is running versus needing to compile the program. This is a powerful feature of JavaScript. -
Untyped. If you have any programming experience, then you've likely come across certain types of languages that require you to declare the type of variable with which you're working. For example, perhaps your variable will store a
string
or aboolean
. In JavaScript, this is not necessary. Instead, you simply declare a variable with thevar
keyword. - Interpreted. When a language is a compiled language, the code you write is converted into an executable binary that you can distribute to others. In Windows, these files are known as EXE files. On OS X, these are often programs you download from the App Store or that you drag into your Applications directory. JavaScript is interpreted, meaning that there is no compiler. Instead, the code is interpreted (as is PHP), so there is an intermediary piece of software called the interpreter that sits between the code you've written and the computer to translate the instructions back and forth.
- Standardized. JavaScript is standardized (its official name being ECMAScript) which means that any browser that implements the standard will offer the same features as any other browser. Were it not standardized then Chrome may provide some features that Edge does not and vice versa.
Now that we've covered the attributes of the language, we can discuss certain aspects and nuances about the language.
Though all of the above is important, it's also essential to know how the language works (especially if you've worked with other languages) so that you don't go into development with pre-conceived ideas as to how it might work or how it should work.
Instead, I'd rather cover how it does work so that you can get started writing code and understand exactly what it is that you're doing.
About the Language
Above all else, JavaScript is an object-oriented programming language, but it likely differs a bit from what you usually see (if you've previously used an object-oriented programming language).
JavaScript is what's called a prototypal language. This means that all of the objects in JavaScript, like String
, are based on prototypes.
This allows us, as developers, to add additional functionality to the objects through the use of prototypal inheritance:
Prototype-based programming is a style of object-oriented programming in which behaviour reuse (known as inheritance) is performed via a process of cloning existing objects that serve as prototypes.
I'd argue that if you've never worked with an object-oriented language before, then you may have an advantage at this point because you have no conceptual model to shift in order to think about how this works.
If, on the other hand, you have worked in these types of languages then I think it's worth distinguishing how prototypal inheritance differs from classical inheritance:
- In classical inheritance, we, as developers, will write a class. Multiple objects can be created from this single class. Furthermore, we can then write another class that inherits from this class and then create instances of those classes. In this situation, subclasses are sharing code with their base class. So when you create an instance of a subclass, you're getting the functionality of both the subclass and the parent class.
- In prototypal inheritance, there's no such thing as classes. Instead, you simply define an object and introduce whatever functionality is necessary. When you want to add functionality to an existing object, you do so by adding it to the object's prototype. If you attempt to call a method on an object such as
Number
then it will first look for the method on that object. If it doesn't find it, then it will move up the chain until it finds the method (which may live on the baseObject
).
Finally, and perhaps the most important thing to note, is that when you make a change to an object via its prototype, then it's accessible to everyone who uses that object (at least within the context of your environment).
It's really powerful, it's really cool, but it also takes a slight shift in thinking if you're not used to working in an environment like that.
How Do We Use JavaScript?
In terms of how we actually put JavaScript to use, it ultimately depends on what your goals are. At one point, working with JavaScript meant that you needed to "make something happen" on a web page. It was meant to control the behavior.
This could be introducing an element, removing (or hiding) an element, or things like that. Then the web advanced a little bit and browsers were able to make asynchronous calls to the server, handle the response, and then change the state of the page based on this response.
All of this is achieved via Ajax. If you're reading this, you're likely familiar with the term. If you're not, you can think of it as a way for JavaScript to make a call to the server hosting the page and then handle the response it receives all without reloading the page.
But it's matured even beyond that.
Google has developed a highly sophisticated JavaScript parsing engine known as V8, and other browsers are working to provide optimal JavaScript performance, as well.
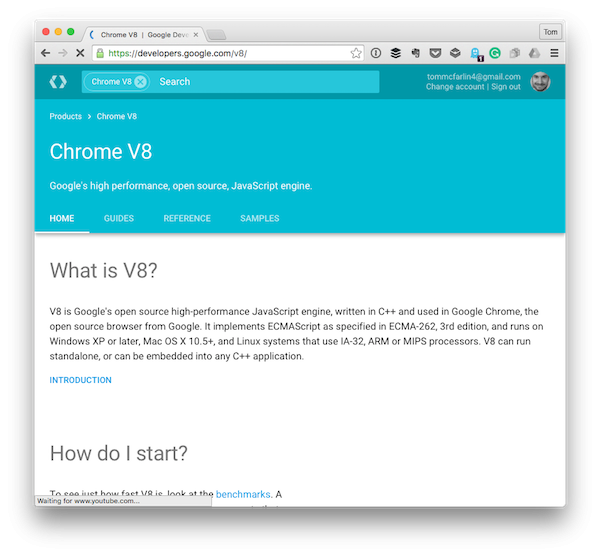
In fact, we're now able to write JavaScript on the server using tools like Node.js. Furthermore, we're even able to build hybrid applications that run on our mobile devices. This means we're able to build solutions for our phones, our tablets, and our desktop computers through the use of JavaScript.

And this is coming from a language that was once used as a way to animate things on a screen. All of this to say is that if you're new to JavaScript, don't underestimate it.
"What Should I Expect From the Language?"
All of the above is interesting to read, and it's fun to see what we're able to do, but from a purely practical perspective, what can we expect from the JavaScript language?
Regardless of whether you're new to the language or you're looking to learn a new language when you've come from another background, you've got a level of expectations as to what the language can offer.
And though we've talked about how the language works from an internal perspective, we haven't really talked about the objects that are available in the language, let alone APIs. To be honest, covering the APIs and built-in functions in the language would be an article all its own.
But covering its built-in objects? That's something we can review before ending this article:
- Object. The base object from which all other objects inherit some of their basic functionality.
- Function. Since JavaScript is truly object-oriented, this means that everything is an object, including functions. So when you create a new function, you're creating a reference to an object with a Function type. And functions have properties that you can inspect during runtime (such as the arguments passed into it).
-
Boolean. This object serves as an object wrapper for a boolean value. In many languages, booleans are a data type that's either
true
orfalse
. In JavaScript, you can still work with those values, but they are to be understood as objects. -
Number. In many programming languages, there are primitive types such as
float
,int
,double
, and so on. In JavaScript, there's only a number type, and it too is an object. - Date. Working with dates in programming is never fun, especially when you introduce timezones. I can't say that JavaScript will solve all of your problems as it relates to timezones, but it can make it a bit easier working with dates (all the way from year and month down to day, hour, minute, and second).
- String. Nearly every programming language has a primitive string data type. JavaScript isn't much different except that, as you'd expect, the string is an object with properties of its own.
Remember that all of the types that you see above are objects with properties (and functions) of their own that you can call. This doesn't mean that you need to call constructors to instantiate your variables. That is, you can create strings and booleans and numbers like this:
var example_string = 'Hello world!'; var example_boolean = true; var example_number = 42;
But, ultimately, they are still objects.
To be clear, these are the basic objects. There are far more advanced objects that are worth exploring, especially if you're going to be working with error handling, various types of collections beyond Arrays, and so on.
If you're interested in reading more about these, then I highly recommend checking out this page in the Mozilla Developer Network.
What Libraries and Frameworks Are Available?
If you've been keeping up with the various frameworks, libraries, and other tools that exist in the JavaScript economy, then you're by no means behind on just how vibrant the economy has become.
But this article is targeting those who are looking to get started with JavaScript. Now that you have a basic understanding of how the language is structured and how it works, it's time to look at the libraries and frameworks offered to help ease web and/or application development.
- jQuery is a library that aims to provide a cross-browser API that allows you to "write less, do more."
- Angular is a JavaScript framework that aims to make building single-page applications easier.
- React is a JavaScript library for building user interfaces.
- Backbone aims to give structure to web applications through the use of models, collections, and views.
- Ember.js is another framework for "creating ambitious web applications".
- And more.
This is far from a complete list of what's available, but it is a start, and it's a handful of options that those getting familiar with JavaScript should at least be aware of, even if you don't do any work with them.
And as you begin to learn JavaScript and start to pick up some of these tools, you may find just how popular some of them are when it comes to some of your very own favorite applications.
Learning JavaScript
As you've come to expect, Envato is all for "teaching skills to millions worldwide". So what would a post like this be if it didn't include links to some of our more popular JavaScript articles and courses?
- Quiz: JavaScript ES6, Do You Know the Right Tool for the Job?
-
Keeping Promises With JavaScript
-
Creating Single Page Applications With WordPress and Angular.js
-
The Genius of Template Strings in ES6
-
JavaScript ES6 Fundamentals
-
Testing Angular Directives
-
JavaScript for Windows 10 Universal Apps
All of these resources are ideal for getting started with JavaScript and adding it to your repertoire of web development skills.
Conclusion
When it comes to web development, JavaScript is here to stay. Though you may not use what's considered to be "vanilla JavaScript" and opt for one of the many libraries and/or frameworks that are available, JavaScript is a language almost every web developer should know.
Of course, not everyone works on the front-end. Some are purely server-side developers; some are purely client-side developers. Nonetheless, we all have to work together to make sure the various parts of our applications are communicating with one another.
To that end, it's at least important to understand how data from the client-side is sent to the server-side via JavaScript, and how it's processed on the server-side and then returned to the client-side to be used in whatever manner.
Don't be so quick to write off JavaScript just because you're not a front-end developer. Odds are, someone you're working with is using it and will need your work to tie parts of the application together.
Granted, this article is just scratching the surface. As I said at the beginning, the purpose of the article is to explain what JavaScript is, how it's used, and what to expect from it, particularly for those who are just getting started with the language.
JavaScript has become one of the de-facto languages of working on the web. It’s not without it’s learning curves, and there are plenty of frameworks and libraries to keep you busy, as well. If you’re looking for additional resources to study or to use in your work, check out what we have available in the Envato marketplace.
If you've enjoyed this article, you can also check out my courses and tutorials on my profile page, and, if you're interested, you can read more articles specifically about WordPress and WordPress development on my blog.
Comments