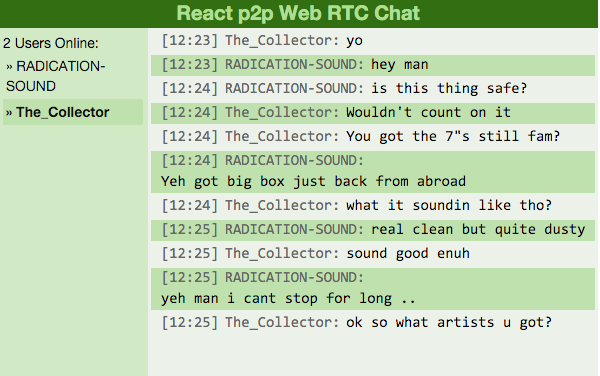
JSX is similar to a mix of XML and HTML. You use JSX within React code to easily create components for your apps. JSX transforms into JavaScript when React compiles the code.
The beauty of React is that you can create reusable code and easily structure your app from a component-based mindset. Finally, the gap between mocking up wireframes of semantically formed ideas and implementing them has never been closer.
Your First Taste of JSX
Here is an example of JSX being used to render HTML:
var div = <div className="foo" />; ReactDOM.render(div, document.getElementById('example'));
To create a component, just use a local variable that starts with an upper-case letter, e.g.:
var MyComponent = React.createClass({/*...*/}); var myElement = <MyComponent someProperty={true} />; ReactDOM.render(myElement, document.getElementById('example'));
Note: There are reserved words in JSX, as it is essentially JavaScript after all—so keywords such as class
and for
are discouraged as attribute names. Instead, React components expect the React property names such as className
and htmlFor
, for example.
Nesting Tags
Specify children inside your JSX as so:
var User, Profile; // You write in JSX: var app = <User className="vip-user"><Profile>click </Profile></User>; // What will get outputted in JS: var app = React.createElement( User, {className:"vip-user"}, React.createElement(Profile, null, "click") );
Testing JSX
Use the Babel REPL to test out JSX.
Sub-Components and Namespaces
Form creation is easy with JSX and sub-components, for example:
var Form = FormComponent; var App = ( <Form> <Form.Row> <Form.Label htmlFor='login' /> <Form.Input name='login' type='text' /> </Form.Row> <Form.Row> <Form.Label htmlFor='password' /> <Form.Input name='pass' type='password' /> </Form.Row> </Form> );
To make this work, you must create the sub-components as attributes of the main component:
var FormComponent = React.createClass({ ... }); FormComponent.Row = React.createClass({ ... }); FormComponent.Label = React.createClass({ ... }); FormComponent.Input = React.createClass({ ... });
Expressions
To use some JavaScript to create a result for use in an attribute value, React just needs you to wrap it in {}
curly braces like so:
// You write in JSX: var myUser = <User username={window.signedIn ? window.username : ''} />; // Will become this in JS: var myUser = React.createElement( User, {username: window.signedIn ? window.username : ''} );
You can also just pass a boolean value for form attributes such as disabled
, checked
and so on. These values can also be hardcoded if you like by just writing plain HTML.
// Make field required <input type="text" name="username" required />; <input type="text" name="username" required={true} />; // Check by default <input type="checkbox" name="rememberMe" value="true" checked={true} />; // Enable a field <input type="text" name="captcha" disabled={false} />
Spread Attributes
When you want to set multiple attributes, ensure you do this on declaration of your component and never after. Later declaration becomes a dangerous anti-pattern that means you could possibly not have the property data until much later in execution.
Add multiple properties to your component as so, using ES6’s new ...
spread operator.
var props = {}; props.username = username; props.email = email; var userLogin = <userLogin {...props} />; ``` You can use these prop variables multiple times. If you need to override one of the properties, it can be done by appending it to the component after the `...` spread operator, for example: ```js var props = { username: 'jimmyRiddle' }; var userLogin = <userLogin {...props} username={'mickeyFinn'} />; console.log(component.props.username); // 'mickeyFinn'
Comments in JSX
You can use both the //
and /* ... */
multi-line style comments inside your JSX. For example:
var components = ( <Navigation> {/* child comment, put {} around */} <User /* multi line comment here */ name={window.isLoggedIn ? window.name : ''} // Here is a end of line comment /> </Navigation> );
Common Pitfalls With JSX
There are a few things that can confuse some people with JSX, for example when adding attributes to native HTML elements that do not exist in the HTML specification.
React will not render any attributes on native HTML elements that are not in the spec unless you add a data-
prefix like so:
<div data-custom-attribute="bar" />
Also, the rendering of HTML within dynamic content can get confusing due to escaping and the XSS protection that React has built in. For this reason, React provides the dangerouslySetInnerHTML
.
<div dangerouslySetInnerHTML={{__html: 'Top level » Child'}} />
An Example Chat Application
You may have seen the app Gitter. It’s a real-time web chat application that’s mainly aimed at developers. Many people are using it to discuss their projects on GitHub, due to its easy integration with GitHub and being able to create a channel for your repository.
This type of application can easily be created with React by implementing the WebRTC
API to create a p2p chat in the browser. For this we will be using the PeerJs
and socket.io
modules for Node.
To give you a clear idea of the architecture of the application, here is a basic low-level UML diagram:

ChatServer receives signal messages with PeerJS, and each client will use it as a proxy for taking care of the NAT traversal.
We will start the app from the ground up to give you a good feel of how to create a React application. First create a new directory for your application, and inside it make a package.json
.
{ "name": "react-webrtc-chat", "version": "0.0.0", "description": "React WebRTC chat with Socket.io, BootStrap and PeerJS", "main": "app.js", "scripts": { "start-app": "node app.js", "start": "npm run start-app" }, "keywords": [ "webrtc", "react" ], "license": "MIT", "dependencies": { "express": "~4.13.3", "peer": "~0.2.8", "react": "~0.12.2", "react-dom": "^0.14.1", "socket.io": "~1.0.6" }, "devDependencies": { "babel-preset-react": "^6.0.14", "babelify": "^7.1.0", "browserify": "^12.0.1" } }
This package.json
file is used by npm
in order to easily configure your application. We specify our dependencies: express
, a web framework that we will use to serve our app; peer
, the peerjs server we will use for signalling; socket.io
which will be used for polling and the webRTC implementation. react-bootstrap
and bootstrap
are packages for using Twitter’s CSS framework to style our app.
We will also need some additional packages, and for that we will use bower
.
Create a bower.json
and add the following:
{ "name": "react-webrtc-chat", "main": "app.js", "version": "0.0.0", "ignore": [ "**/.*", "node_modules", "bower_components", "public/lib", "test", "tests" ], "dependencies": { "react": "~0.12.2", "jquery": "~2.1.4", "eventEmitter": "~4.2.7", "peerjs": "~0.3.14", "bootstrap": "~3.3.5" } }
With this configuration, we bring down react
, jQuery
, bootstrap
, eventEmitter
for firing events and the peerJS
Client library for wrapping the WebRTC.
Finally, specify where to install this by setting the .bowerrc
file:
{ "directory": "src/lib" }
From here, just sit back and install your dependencies via:
$ bower install && npm install
Once this command has finished, you will see a new directory node_modules
and src/lib
. These contain the modules ready for use.
Now create an app.js
in the main directory alongside your package.json
, etc. This will be the main entry point of your application.
//Configure our Services var express = require('express'), PeerServer = require('peer').PeerServer, events = require('./src/Events.js'), app = express(), port = process.env.PORT || 3001; //Tell express to use the 'src' directory app.use(express.static(__dirname + '/src')); //Configure the http server and PeerJS Server var expressServer = app.listen(port); var io = require('socket.io').listen(expressServer); var peer = new PeerServer({ port: 9000, path: '/chat' }); //Print some console output console.log('#### -- Server Running -- ####'); console.log('Listening on port', port); peer.on('connection', function (id) { io.emit(events.CONNECT, id); console.log('# Connected', id); }); peer.on('disconnect', function (id) { io.emit(events.DISCONNECT, id); console.log('# Disconnected', id); });
This will simply create an Express server, making the src/
files we just got with bower
now accessible via HTTP. Then a socket.io
instance was created to listen on the expressServer
object. This is used for polling and facilitating the next step for the PeerServer
, which will actually do the WebRTC chat part.
To configure a PeerServer
, all you need to do is specify the port
and path
the server will run on, and then start configuring events with the .on
method. We are using a separate file named Events.js
to specify our app’s events.
peer.on('connection', function (id) { io.emit(events.CONNECT, id); console.log('# Connected', id); });
Here we use the events.CONNECT
event to specify when a user has connected to our app. This will be used by the states of our view components to update their displays in real time.
To do this, we need to create the Server for our peer-to-peer connections to proxy through.
Create a file in src/Server.js
and add the following:
/* global EventEmitter, events, io, Peer */ 'use strict'; function ChatServer() { EventEmitter.call(this); this._peers = {}; } ChatServer.prototype = Object.create(EventEmitter.prototype); ChatServer.prototype.onMessage = function (cb) { this.addListener(events.MSG, cb); }; ChatServer.prototype.getUsername = function () { return this._username; }; ChatServer.prototype.setUsername = function (username) { this._username = username; }; ChatServer.prototype.onUserConnected = function (cb) { this.addListener(events.CONNECT, cb); }; ChatServer.prototype.onUserDisconnected = function (cb) { this.addListener(events.DISCONNECT, cb); }; ChatServer.prototype.send = function (user, message) { this._peers[user].send(message); }; ChatServer.prototype.broadcast = function (msg) { for (var peer in this._peers) { this.send(peer, msg); } }; ChatServer.prototype.connect = function (username) { var self = this; this.setUsername(username); this.socket = io(); this.socket.on('connect', function () { self.socket.on(events.CONNECT, function (userId) { if (userId === self.getUsername()) { return; } self._connectTo(userId); self.emit(events.CONNECT, userId); console.log('User connected', userId); }); self.socket.on(events.DISCONNECT, function (userId) { if (userId === self.getUsername()) { return; } self._disconnectFrom(userId); self.emit(events.DISCONNECT, userId); console.log('User disconnected', userId); }); }); console.log('Connecting with username', username); this.peer = new Peer(username, { host: location.hostname, port: 9000, path: '/chat' }); this.peer.on('open', function (userId) { self.setUsername(userId); }); this.peer.on('connection', function (conn) { self._registerPeer(conn.peer, conn); self.emit(events.CONNECT, conn.peer); }); }; ChatServer.prototype._connectTo = function (username) { var conn = this.peer.connect(username); conn.on('open', function () { this._registerPeer(username, conn); }.bind(this)); }; ChatServer.prototype._registerPeer = function (username, conn) { console.log('Registering', username); this._peers[username] = conn; conn.on('data', function (msg) { console.log('Message received', msg); this.emit(events.MSG, { content: msg, author: username }); }.bind(this)); }; ChatServer.prototype._disconnectFrom = function (username) { delete this._peers[username]; };
This is the main guts of the application. Here we configure the ChatServer
object with all of its functions.
First we use socket.io
to establish signalling of a new user connected via the events.CONNECT
as so:
ChatServer.prototype.connect = function (username) { var self = this; this.setUsername(username); this.socket = io(); this.socket.on('connect', function () { self.socket.on(events.CONNECT, function (userId) { if (userId === self.getUsername()) { return; } self._connectTo(userId); self.emit(events.CONNECT, userId); console.log('User connected', userId); });
Then, to connect to the PeerServer, we use the following:
this.peer = new Peer(username, { host: location.hostname, port: 9000, path: '/chat' });
We then listen for events via the on
method:
this.peer.on('open', function (userId) { self.setUsername(userId); }); this.peer.on('connection', function (conn) { self._registerPeer(conn.peer, conn); self.emit(events.CONNECT, conn.peer); });
We also have our JSX inside components in the components/chat
directory. Take your time to look through all of them in the repository. I will be focusing on the ChatBox
component for now:
/** @jsx React.DOM */ 'use strict'; var ChatBox = React.createClass({ getInitialState: function () { return { users: [] }; }, componentDidMount: function () { this.chatProxy = this.props.chatProxy; this.chatProxy.connect(this.props.username); this.chatProxy.onMessage(this.addMessage.bind(this)); this.chatProxy.onUserConnected(this.userConnected.bind(this)); this.chatProxy.onUserDisconnected(this.userDisconnected.bind(this)); }, userConnected: function (user) { var users = this.state.users; users.push(user); this.setState({ users: users }); }, userDisconnected: function (user) { var users = this.state.users; users.splice(users.indexOf(user), 1); this.setState({ users: users }); }, messageHandler: function (message) { message = this.refs.messageInput.getDOMNode().value; this.addMessage({ content: message, author : this.chatProxy.getUsername() }); this.chatProxy.broadcast(message); }, addMessage: function (message) { if (message) { message.date = new Date(); this.refs.messagesList.addMessage(message); } }, render: function () { return ( <div className="chat-box" ref="root"> <div className="chat-header ui-widget-header">React p2p Web RTC Chat</div> <div className="chat-content-wrapper row"> <UsersList users={this.state.users} username={this.props.username} ref="usersList"></UsersList> <MessagesList ref="messagesList"></MessagesList> </div> <MessageInput ref="messageInput" messageHandler={this.messageHandler}> </MessageInput> </div> ); } });
This class takes usage of the ChatServer
we created before, utilising it as a proxy for the ChatBox
component.
The components and libraries are finally all rendered on the page with index.html
and served via node express.
To start the app, run npm start
and point your browser to http://localhost:3001
to take a look at the chat.
Going Live on Heroku
Serving from the cloud is really easy with Heroku. Sign up for a free account and then you can install the heroku
toolbelt on to your system. Read more about getting Heroku
set up at the Heroku Dev Center.
Now that you have Heroku
available, log in and create a new project like so:
$ git clone [email protected]:tomtom87/react-p2p-chat.git $ cd react-p2p-chat $ heroku create Creating sharp-rain-871... done, stack is cedar-14 http://sharp-rain-871.herokuapp.com/ | https://git.heroku.com/sharp-rain-871.git Git remote heroku added
Here you will get a random name from Heroku and your app URL—in our example it is http://sharp-rain-871.herokuapp.com/
. Heroku also creates a git repo for this app.
Now it’s as simple as pushing your code to heroku:
$ git push heroku master
Once the push is finished, you will be able to start your web service with the following:
$ heroku ps:scale web=1
Now just visit the URL provided, or as a shortcut use the open
command as so:
$ heroku open
Conclusions
You’ve learned how to create JSX components and interface them with React, with a detailed example of the chat application. Take some time to go through the code and see how React and the components/chat
directory are working.
Combined with the deployment to Heroku
, you can start hacking and creating your own React
apps for the cloud!
Comments