In this tutorial, you'll learn about virtual environments. You'll learn about the importance of using virtual environments in Python and how to get started with using virtual environments.
What Is a Virtual Environment?
A virtual environment is a tool to maintain separate space for a project with its dependencies and libraries in one place. This environment is specific to the particular project and doesn't interfere with other projects' dependencies.
For example, you can work on project X which is using version 1.0 of library Z and also maintain project Y which is using version 2.0 of library Z.
How Do Virtual Environments Work?
The virtual environment tool creates a folder inside the project directory. By default, the folder is called venv
, but you can custom name it too. It keeps Python and pip executable files inside the virtual environment folder. When the virtual environment is activated, the packages installed after that are installed inside the project-specific virtual environment folder.
Getting Started With VirtualEnv
First, make sure you have pip
installed on your system. You can install pip
using the following command:
sudo apt-get install python-pip python-dev build-essential
Using pip
, install the virtual environment tool.
pip install virtualenv
To get started with using virtualenv
, you need to initialize and activate it. Let's start by creating a new Python project directory PythonApp
.
mkdir PythonApp
Navigate to the project directory PythonApp
and initialize the virtual environment by typing the following command:
virtualenv PythonAppVenv
The above command will set up the virtual environment for the project PythonApp
.
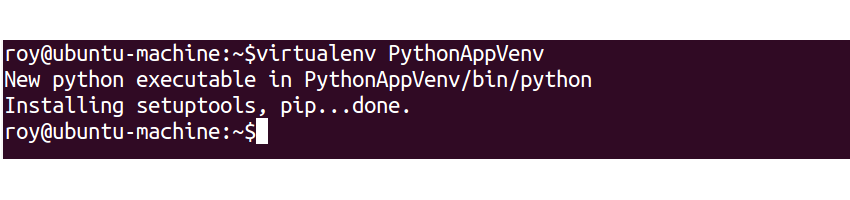
It creates a folder called PythonAppVenv
inside the project directory PythonApp
. It keeps the Python and pip executables inside the virtual environment folder. Any new packages installed for the project after virtual environment activation are placed inside the virtual environment folder. Here is the folder structure:
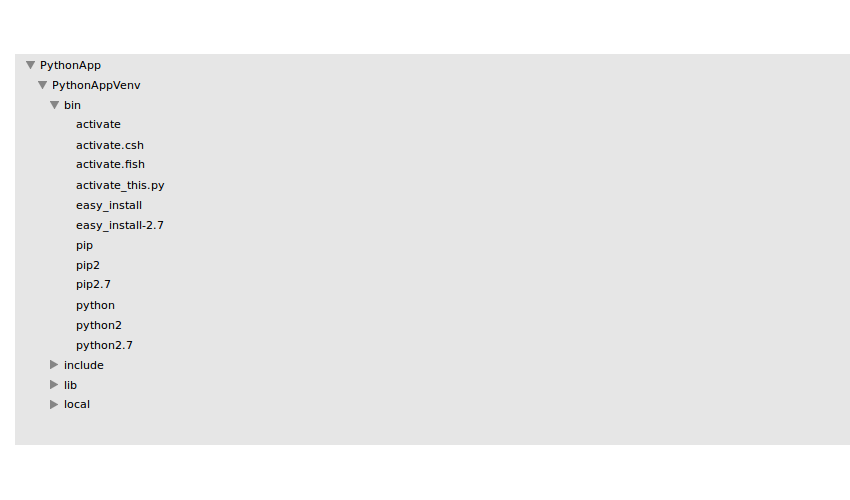
To get started with using the virtual environment, you need to activate it using the following command:
source PythonAppVenv/bin/activate
Once activated, you should be able to see the PythonAppVenv
name on the left side of the name prompt.

Let's try to install a new package to the project PythonApp
.
pip install flask
The new package should get installed in the virtual environment folder. Check the virtual environment folder inside lib/python2.7/site-packages
and you should be able to find the newly installed flask
package. You can learn more about Flask on the project page.
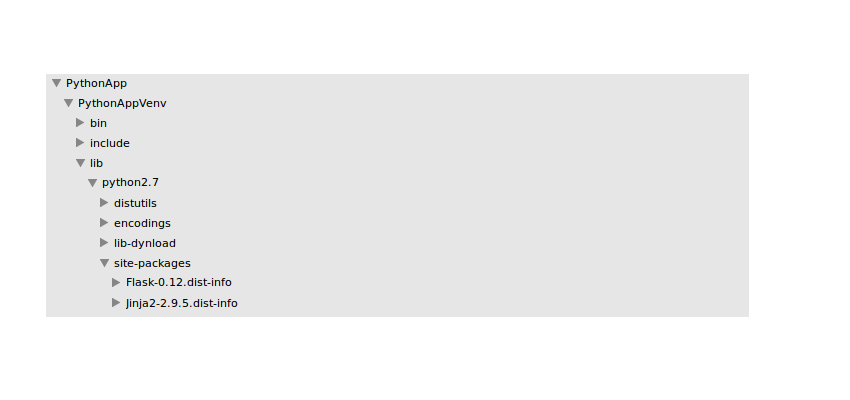
Once you are done with the virtual environment, you can deactivate it using the following command:
deactivate
Easier to Track Packages
While working with Python programs, you install different packages required by the program. You keep working, and the list of packages installed keeps on piling up. Now the time arrives when you need to ship the Python code to the production server. Oops... You really don't know what packages you have installed for the program to work.
All you can do is to open the Python program and check for all the packages that you have imported in your program and install them one by one.
A virtual environment provides an easier method to keep track of the packages installed in the project. Once you have activated the virtual environment, it provides the facility to freeze the current state of the environment packages.
You can achieve this by using the following command:
pip freeze > requirements.txt
The above command creates a file called requirements.txt
which has details about the packages with versions in the current environment. Here is how it looks:
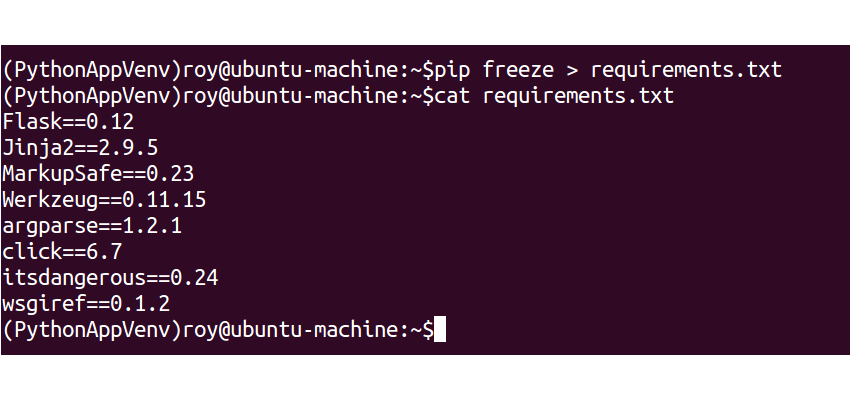
Now this file would be really helpful for deploying the project on a different platform since all the project dependencies are already at your disposal in the requirements.txt
file. To install the project dependencies using the requirements.txt
file, execute the following command:
pip install -r requirements.txt
virtualenvwrapper
to Make Things Easier
The virtualenv
tool is really a boon for developers. But it gets really complicated when you have to deal with more than one virtual environment. To manage multiple virtual environments, there is an extension to the virtualenv
tool called virtualenvwrapper
.
virtualenvwrapper
is a wrapper around the virtualenv
tool which provides the functionality to manage multiple virtual environments.
Let's get started by installing virtualenvwrapper
using pip.
pip install virtualenvwrapper
Once you have installed virtualenvwrapper
, you need to set the working directory where the virtual environments will be stored. Execute the following command to set the working directory for virtualenvwrapper
:
export WORKON_HOME=.virtualenvs
The above command sets the working directory for virtualenvwrapper
to the .virtualenvs
folder in the home directory.
You can either source the virtualenvwrapper
commands to run from the terminal or add the virtualenvwrapper
commands to the .bashrc
.
source /usr/local/bin/virtualenvwrapper.sh
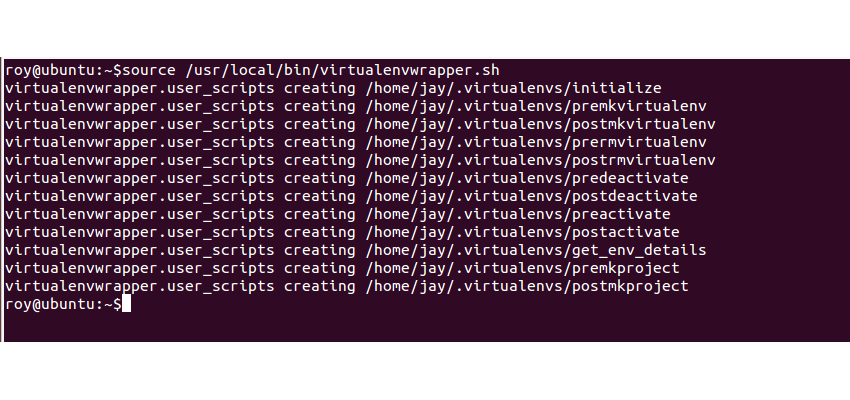
Now the commands will be accessible in the current terminal by pressing the Tab key. Create a new project folder called PythonProject
. Navigate to the project directory. Earlier, when you used virtualenv
, you first created the virtual environment and then activated it. Using virtualenvwrapper
, you can complete both of these tasks using a single command.
mkvirtualenv PythonVenv
The above command creates the virtual environment and then activates it.
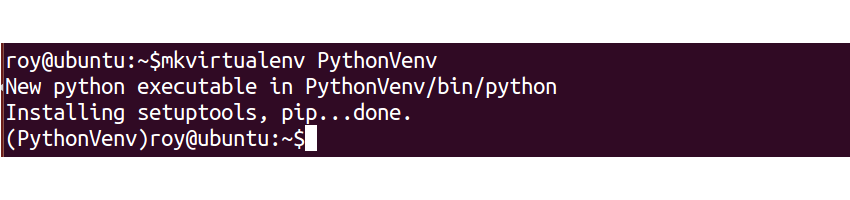
To deactivate the virtual environment, you need to type in the deactivate
command.
deactivate
Now suppose in certain scenarios you need to switch between the different virtual environments you are working in. virtualenvwrapper
provides a workon
method to switch virtual environments. The command to switch the virtual environment is:
workon PythonV
In the above command, PythonV
is the name of the virtual environment. Here is an image where the workon
command is shown in action:
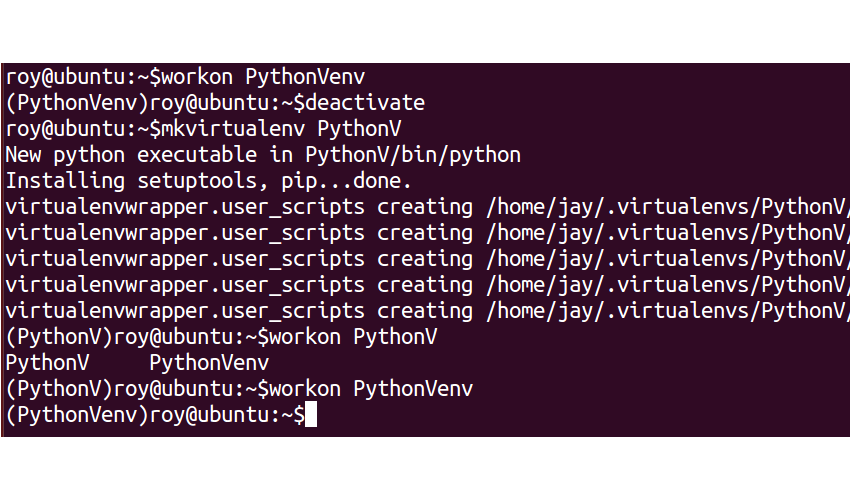
virtualenvwrapper
also provides a command to list the virtual environments in your environment.
ls $WORKON_HOME
The above command displays a list of virtual environments that exist in the environment.
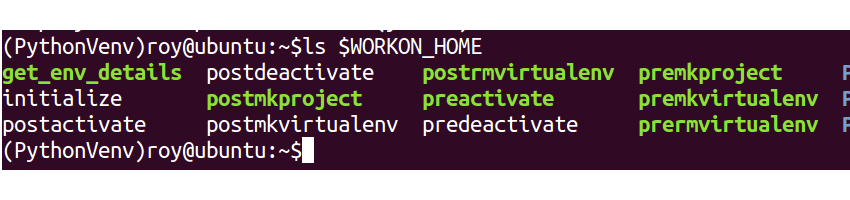
To remove an existing virtual environment, you can use the rmvirtualenv
command.
rmvirtualenv PV
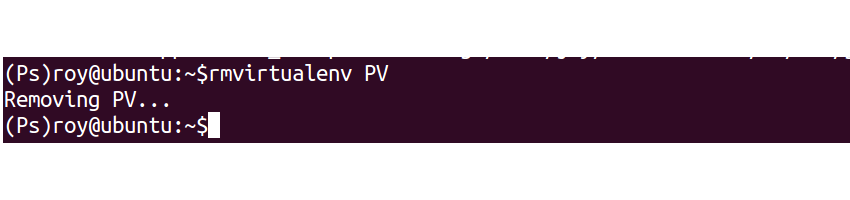
There is a command which creates a project directory and its associated virtual environment. Navigate to the terminal and execute the following command:
mkproject NewPro
The above command should create the project and its associated virtual environment.
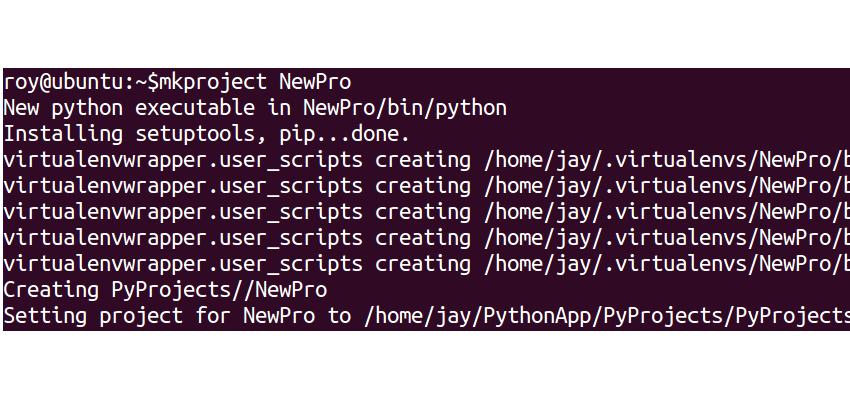
There are a few more commands that you can use in virtualenvwrapper
. You can find the list of commands available by typing the following command:
virtualenvwrapper
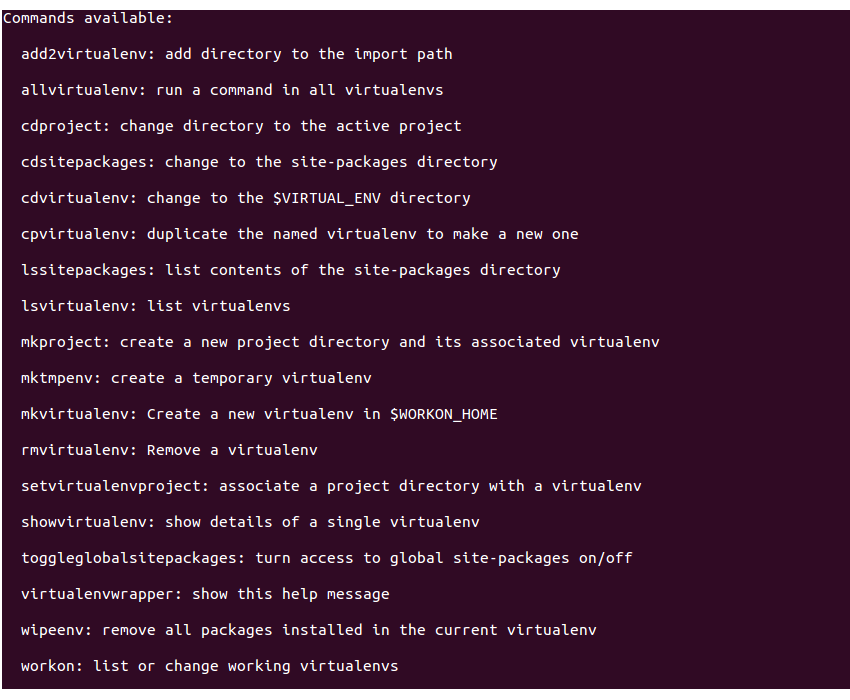
Wrapping It Up
In this tutorial, you saw how to get started with using virtual environments in Python. You learnt the importance of using virtual environment and how it works. You also had a look at virtualenvwrapper
, a wrapper in the virtualenv
tool for managing multiple virtual environments.
Have you ever used virtual environments in Python? Do let us know your thoughts in the comments below.
Comments